How to write code with few bugs?
Summary
TLDRThis video script discusses strategies for writing code with minimal bugs, emphasizing the importance of understanding automated testing, including unit, integration, and visual regression testing. It highlights the significance of the testing pyramid and the need for a comprehensive approach to software delivery, from development to production. The speaker shares real-world examples to illustrate common pitfalls and suggests using feature flagging and blue-green deployment to mitigate risks. The script concludes by advocating for simplicity in code to reduce complexity and potential bugs.
Takeaways
- 🔍 **Understand Automated Testing**: The importance of automated testing, including unit, integration, and visual regression testing, is emphasized to ensure each layer of the application works as expected.
- 📚 **Learn the Testing Pyramid**: Familiarize yourself with the testing pyramid to understand the different levels of testing and how they are applied in your specific tech stack.
- 🛠️ **Know Your Stack's Tools**: Gain knowledge of the tools commonly used in your tech stack for effective bug detection and management at various levels.
- 🦟 **Bugs are Unintended Behaviors**: Recognize that bugs are simply unintended behaviors that can affect user experience, and understanding this can help in identifying potential failure points.
- 🔗 **Environment Synchronization**: Ensure that updates across different environments are synchronized to prevent inconsistencies that can lead to production issues.
- 🚫 **Prevent Temporary Outages**: Utilize strategies like feature flagging and blue-green deployment to avoid temporary outages during updates.
- 🔄 **Continuous Learning**: Acquire a full-stack understanding to effectively manage the software delivery process and mitigate potential production issues.
- 🛡️ **Implement Fail-Safes**: Apply necessary safeguards such as health checks and redundancies to handle potential failures in production.
- 🔄 **Rollbacks and Redundancies**: Prepare for the possibility of bugs by having rollback procedures and redundancy systems in place.
- 📈 **Simplicity in Code**: Write simple and stable code to reduce complexity and the likelihood of bugs, especially in the delivery process.
- 🌐 **Consider Global Issues**: Be aware of potential issues like character encoding that can affect users globally and ensure your application is prepared for such scenarios.
Q & A
What is the primary focus of the discussion in the video script?
-The primary focus of the discussion is on the best approaches to write code with very few bugs, emphasizing the importance of understanding automated testing and the testing pyramid.
Why is automated testing crucial in reducing bugs in software development?
-Automated testing is crucial because it helps to ensure that each layer of the application is working as expected, thus preventing unintended behavior that affects the user experience.
What is the testing pyramid and why is it important?
-The testing pyramid is a concept that illustrates the different levels of testing in software development, from unit tests at the base to UI tests and end-to-end tests at the top. It's important because it helps developers understand the general tools and patterns used to deal with bugs at different levels.
What is an example of a production issue mentioned in the script?
-An example of a production issue mentioned is when a developer updated the base URL of an application without coordinating with the operations team, leading to a broken load balancer and incorrect routing, which caused the entire application to fail.
Why did the QA environment not help in the scenario described with the developer updating the base URL?
-The QA environment did not help because the issue was related to the front-end routing and the developer did not consider how to synchronize the updates of the routing with the production environment, leading to a temporary outage.
What is feature flagging and how can it help in reducing bugs?
-Feature flagging is a technique that allows developers to enable or disable features without deploying new code. It helps in reducing bugs by allowing for a safer deployment process, such as blue-green deployments, where a new version of the code can be tested before fully replacing the old one.
What is the significance of understanding the entire delivery process from code writing to production?
-Understanding the entire delivery process is significant because it allows developers to identify potential points of failure and implement necessary fail-safes, thus reducing the likelihood of bugs in production.
What is the importance of writing simple code to minimize bugs?
-Writing simple code is important because it reduces unnecessary complexity in the delivery process, making it easier to manage and less prone to introducing bugs.
Can you provide an example of a common mistake that leads to bugs, as mentioned in the script?
-An example of a common mistake is when developers forget to consider the execution environment or logical errors, such as not properly importing code libraries into a Docker container, leading to a broken container build.
What strategies are suggested for dealing with bugs in production?
-Strategies suggested include using feature flagging, implementing redundancies like multiple availability zones, running end-to-end tests in production, and ensuring that there are checks and health monitors for running code.
Why is it recommended to have more than one availability zone when dealing with cloud providers?
-Having more than one availability zone is recommended to provide redundancy and increase the reliability of the application, ensuring that if one zone goes down, the application can still function in another zone.
Outlines
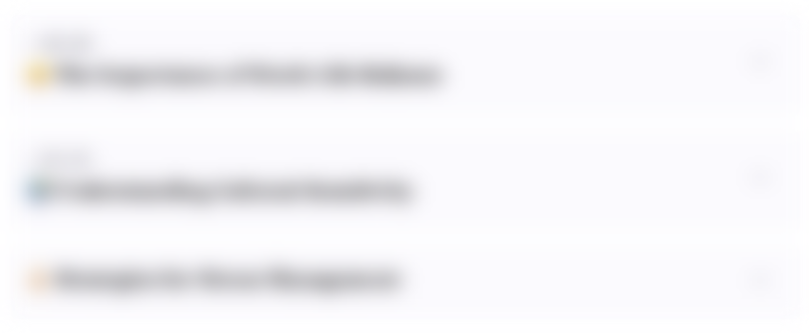
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
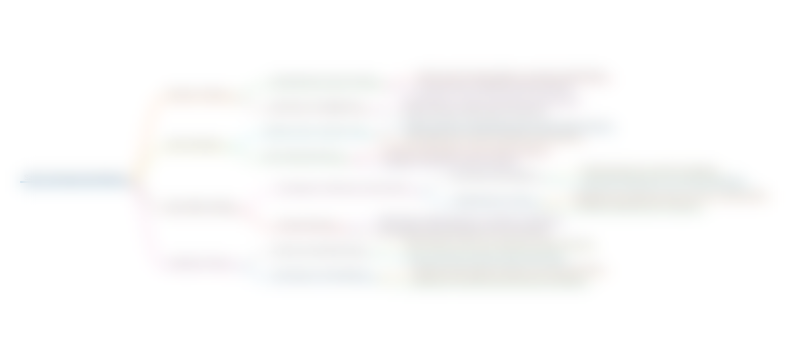
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
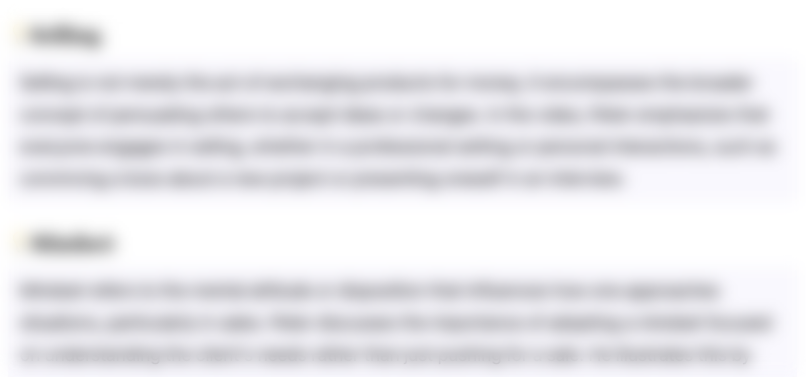
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
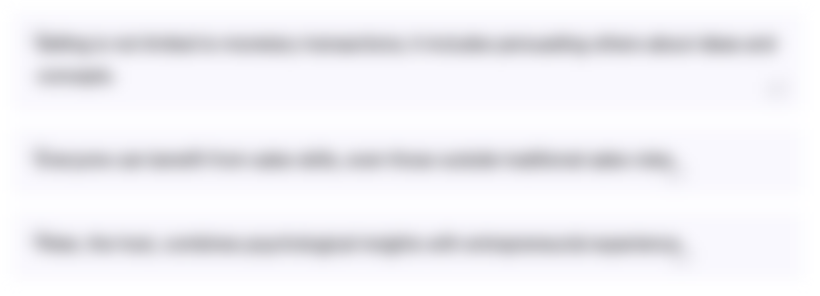
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
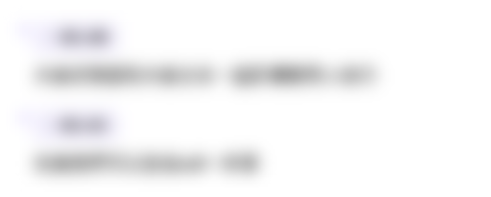
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)