NestJs REST API with MongoDB #4 - Authentication, Login/Sign Up, assign JWT and more
Summary
TLDRThis tutorial video guides viewers through implementing authentication in a REST API using Nest.js. It covers setting up the Passport library, creating authentication modules, and generating JWT tokens for user login and registration. The video also demonstrates how to validate user credentials and handle errors. Viewers are encouraged to watch the next video for securing routes and additional authentication features.
Takeaways
- π In previous videos, we created a simple REST API, added search, pagination, and handled errors.
- π This video focuses on implementing authentication in our API with two parts: creating login and register routes and providing JWT tokens.
- π The next video will cover authorizing users and protecting routes from unauthenticated access.
- π οΈ We use Nest.js for our API and configure authentication with the Passport library.
- π¦ Several packages need to be installed, including nest.js/passport and JWT modules.
- π§ We create an auth module, controller, and service, and configure Passport and JWT in the auth module.
- πΎ The user schema is defined with fields for email and password, ensuring email uniqueness and adding timestamps.
- π JWT configuration includes setting secret and expiration time using environment variables.
- π Auth service handles user registration with hashed passwords and returns a JWT token.
- π§ͺ Validation checks are performed during login, and JWT tokens are generated and returned upon successful login.
- π Detailed steps include installing necessary packages, setting up schemas, configuring modules, and creating routes for login and signup.
- π¬ The next video will continue with protecting routes using JWT strategy and saving the user in the book.
Q & A
What is the main focus of the video?
-The video focuses on implementing authentication in a REST API using Nest.js, including creating login and register routes and issuing JWT tokens to users.
Why is the Passport library used in Nest.js for authentication?
-Nest.js uses the Passport library because it is a popular library for Node.js used for authentication, and it integrates well with Nest.js through the 'nestjs/passport' package.
What are the packages needed to be installed for implementing authentication in the video?
-The packages needed include 'passport', 'nestjs/passport', '@nestjs/jwt', and 'bcryptjs' for hashing passwords.
How does the video guide the creation of the authentication module?
-The video instructs the viewer to use the 'nest g module auth' command to generate the authentication module and then proceed to create a controller and service for it.
What is the purpose of creating a 'schemas' folder and a 'user.schema.ts' file?
-The 'schemas' folder and 'user.schema.ts' file are created to define the user's data structure, including fields like email and password, and to ensure that the email is unique.
Why is the 'bcryptjs' package used in the video?
-The 'bcryptjs' package is used for hashing passwords before saving them to the database, which is a security measure to protect user data.
How does the video handle the creation of DTOs (Data Transfer Objects) for signup and login?
-The video demonstrates creating 'signup.dto.ts' and 'login.dto.ts' files to define the data structure for the signup and login requests, including validation rules for the email and password fields.
What is the role of the JWT token in the authentication process shown in the video?
-The JWT token is issued to the user upon successful signup or login, serving as a secure way to authenticate the user's identity for subsequent requests.
How does the video ensure the security of the JWT token?
-The video sets an expiry time for the JWT token and uses a strong secret key defined in the environment variables to sign the token, enhancing its security.
What is the next step after the authentication implementation covered in the video?
-The next step, as mentioned in the video, is to authorize users and protect routes from unauthenticated users in the subsequent video.
How can viewers access the source code and further learning materials mentioned in the video?
-Viewers can find the source code and additional learning materials, such as a Udemy course link, in the description of the video.
Outlines
π Introduction to API Authentication Implementation
The video begins with an introduction to the task at hand, which is to implement authentication in a previously developed REST API. The speaker outlines a two-part video series, with the current part focusing on setting up the authentication process, including login and registration routes, and issuing JWT tokens to users. The second part will cover user authorization and route protection. Nest.js is highlighted as the framework of choice, utilizing the Passport library for authentication, with specific packages to be installed for the task.
π Setting Up Passport and JWT for Authentication
The speaker proceeds to demonstrate the setup of the Passport and JWT modules for authentication within the Nest.js framework. This includes installing necessary packages, configuring the auth module to use Passport with JWT strategy, and setting up environment variables for the JWT secret and expiry time. The config service is used to access these variables, and the user schema is registered to integrate with the authentication process.
π User Registration and Password Hashing
The video script details the creation of a user registration process. This involves setting up a user schema with email and password fields, ensuring the email is unique. The speaker uses bcryptjs for password hashing before saving the user to the database. After successful registration, the system assigns a JWT token to the user. The process includes creating DTOs (Data Transfer Objects) for sign up and login, and demonstrating the validation of user input.
π User Login Route Creation and Testing
The speaker moves on to creating a login route, which involves validating user credentials and issuing a JWT token upon successful authentication. The login DTO is created with email and password fields. The script describes how to check if the user exists and validate the password using bcrypt's compare function. If the credentials are correct, a token is issued; otherwise, an unauthorized exception is thrown. The speaker tests the login route using Postman, demonstrating the process and expected outcomes.
π Conclusion and Future Steps in Authentication
In conclusion, the speaker summarizes the successful creation of sign up and login routes, including the assignment of JWT tokens to users. The script mentions plans for the next video, which will focus on protecting routes from unauthenticated users and saving user data in a book (likely a typo for 'database'). The speaker invites viewers to ask questions in the comments and provides a link to a related Udemy course and source code in the video description.
Mindmap
Keywords
π‘REST API
π‘Authentication
π‘JWT
π‘Nest.js
π‘Passport
π‘Schema
π‘Mongoose
π‘Bcrypt
π‘Environment Variables
π‘DTO (Data Transfer Object)
π‘Unauthorized Exception
Highlights
Introduction to implementing authentication in a REST API using Nest.js
Overview of two-part video series on authentication, starting with login and registration routes
Explanation of using passport library for authentication in Nest.js applications
Instructions on installing necessary packages for authentication with Nest.js
Creation of an authentication module using Nest.js CLI commands
Generation of controller and service without spec files for the auth module
Configuration of passport in the auth module and setup for login and sign up routes
Creation of a user schema with Mongoose for handling user data and timestamps
Use of unique property to ensure email uniqueness in the user schema
Registration of user schema and setup of passport with JWT strategy
Installation and setup of JWT module for token generation
Configuration of JWT secret and expiry time using environment variables
Implementation of user registration service with password hashing
Use of bcrypt for secure password hashing before saving to the database
Creation of DTOs (Data Transfer Objects) for sign up and login processes
Validation of user input using decorators in DTOs for email and password
Development of the sign up endpoint in the auth controller
Testing the sign up route using Postman and observing validation responses
Demonstration of successful user creation and JWT token generation
Explanation of JWT token contents using jwt.io to inspect the token
Setup of the login route with validation of user credentials
Testing the login route and verifying the correct assignment of JWT tokens
Upcoming continuation of the series focusing on protecting routes and saving users in a book
Invitation for questions and reference to a Udemy course for further learning
Transcripts
welcome back to this channel so in our
previous videos we have created a simple
rest API we have added the search
pagination and we have handled our
errors and now in this video we will
implement the authentication uh in our
API now there are two parts of this
video in the first part we will add the
authentication we will create our login
route register route and we will give
the JWT token to the user and in our
next video we will authorize our users
we will protect our routes from
unauthenticated users so make sure to
watch all uh the videos so in this video
we will implement the authentication so
I am on the documentation of nest.js you
can see that here under security we have
authentication so nest.js uses passport
Library if you don't know password is a
very popular library for node.js that is
used for authentication so nest.js uses
the passport package so you have to also
use that and you can see here we have a
package that is nest.js slash passport
that is configured for the nest.js API
so we have to use these packages
so if you scroll down we have to install
all these packages in order to work I
simply copy it from here copy that and I
go back and let's install all these
packages so I simply paste it here and
let's install them let's go back and
copy this as well and install it
okay so our packages are installed
successfully now I have to create our
module for authentication so I type here
Nest G module and the name of the module
is going to be auth so I will generate
the module
now let's create our controller and
service for the module so I type here
Nest G
controller auth dash dash no spec so
that it will not create any testing file
we will handle the testing in our
upcoming videos so I will create my
controller
then I will create my service file here
and that is it now let's work on our
application
so first of all let's configure our
passport in our auth module and then we
will create our two routes for the login
and sign up okay
so I go to source and the auth we have
this auth module.ts and here we have to
configure our
um and let's actually before that create
our schema so in the auth create a new
folder that is called schemas create a
new file
uh I call it user dot schema dot TS
let's create our schema first I close
this one so here first of all I use here
schema and I will
pass the option that time stamps to true
oops so that it will add the time
automatically when we will create our
user so you export here
a class I have discussed all about these
in my previous video If you haven't
watched that you can watch that also as
well then it will make more sense now
let's add our Fields here so I add here
at prop decorator
in which we can pass further options for
these fields but I will leave it empty
string and then we have here
two fields one is the email and one is
the
password
so in the props I will simply type here
some options like unique
to make sure that the email is unique
and if the email is not unique we will
simply
send back
duplicate
email entered like this simply say this
one and then we have to export here so
export
const user schema
so I use a schema Factory
dot create for class and pass in here my
user okay so now our schema is ready
here long let's
um actually close this one go to our
auth module now let's register our
schema as well and also set up our
passport here so I create here Imports
array
in that I will first of all set up here
the Mongoose module
dot for feature and in that we have to
pass the array of our models so the
first is going to be user
and schema is going to be the user
schema like this and now we have to
configure our password module as I have
explained before nest.js uses the
password for the authentication so I use
here passport uh password module and I
have to import that from nest.js slash
passport that we have installed before
okay then register okay now in the
register we have to pass here one option
that is default strategy that with
strategy we want to use and we want to
use the JWT strategy okay so now we have
registered our password module now let's
uh register our JWT module so JWT
uh module and I think so
I have to install that as well so I type
here npmi at nest.js slash JWT let's
install this package also okay so
package is installed successfully now I
type here password module I have to
import that from uh nest.js diesel
Beauty dot register async so we will use
here register async we can use here uh
the simple register function but we have
to also use the config variable inside
it so that's why we have to use here
register async so let me show you how it
will work
now here we have first of all inject our
config service because we have to use
here two config variables one is a DWT
secret and one is the JWT expired if I
go to my EnV file let's register here
first of all
JWT
expire
and also JWT
Secret
so make sure that you put here a strong
secret I will use here my name
accordingly the bus and then the JWT
expire so this is the expiry time of the
token so I type here like three days now
the expiry time of our jw3 token is
going to be three days so I will Define
all my two variables here close it now
we have to use that here and before that
we have to inject our config service so
I use here inject
on the inject I will use here config
service and if you don't use here like
this you will not be able to access here
process.env.jwt secret it will throw
undefined so we have to use that in this
way we have to inject our config service
then we have to use data function that
is called use Factory
and now
in that we will get access to the config
so the config and type is going to be
config service
like this
now let's
return from here an object in that we
have to pass two things first of all the
secret
okay now we have defined security number
EMV file so I will use here config
which is this one
that will give us access to the EnV
variables so dot get
and I use here JWT
underscore
secret and one more
that is the uh
sign in options and that is going to be
JWT
JWT
expires I think so
expires
save it so this is the variable that we
have to use and let's also give here
type that is going to be
oops
string and this is going to be also
string
but it can be a number also because if
user only pass here like 5 or 6 or 10
like for the minutes or
um other numbers so we have to allow
that also
so it is going to be either a string or
number
and if you have to actually cut it from
here create here an object in that we
will have to pass here expires in and
then we will use like this Simply Save
it and now it will set up our password
module JWT module and also we have
registered our user schema here simply
we have to register our password module
like this pass and here our default
stats data is going to be JWT then we
will use here our JWT module we have to
register it so I will inject my config
service because I want to access my
config variable inside it so I have to
pass here two things first of all the
secret which is going to be this and
then in the sign options I have to pass
the expiry date of that token which is
this one that we have defined in our
config file now let's remove this one
and go to our
here in the service and create our
routes for the login and sign up so here
first of all in the auth service I will
create my Constructor we have to inject
our model so
we'll simply type here add
inject
model and I have to pause here the user
dot name okay and then I will call it
private user model that is going to be
model and type is going to be user okay
and I have to import the model from
Mongoose like this
and then here after the Constructor I
have to register my user so I type here
async
I call it sign up
and in that I have to pass Here sign up
dto that I will create in a minute
and
I have to first of all get
name
email and then the password
from the sign up dto okay and then I
have to Hash my password before saving
any database so I have to also install
one more package that is B grip JS npm I
B grip
Js
I will install it first of all
and then I type here const hashed
password is going to be a weight
B Crypt
dot hash and we have to also import that
so import all As
B grip from
B group Js so B grip dot hash
um now in that I have to pass in here
the password
then this old value type here 10 which
is also a default value so now we have
here our hash password we have to save
the user in the database so simply type
here const user is going to be await
this dot user model dot create
and in that I will simply pass in here
name
email and then the password that is
going to be hashed password like this
okay and now you can also return the
user from here but I have to assign JWT
token to the user so that's why I will
use here A Sign function that will
assign uh JW token to that user how we
can do that first of all we have to use
here
create here a variable so private that
is going to be JWT service
and the type is going to be jwp service
that I have to import from nas JS JWT
okay I have to put in here comma
and now I will simply type your const I
have to create a token so token is going
to be this dot JWT service DOT sign so
the sign function help us to generate
our jwd token so on this sign we have to
pass the payload so what is the payload
payload is the data that we want to save
in the token so we want to save the ID
of the user so type here ID and the
value is going to be user dot underscore
ID okay now we have to return the token
from here isomer type here return
token okay and the return type of this
is going to be
I type here promise oops
and
that is going to be an object
that contains token and type is going to
be string
okay
and let's actually first of all create
our signup dto I go to the auth
create here dto
and if you don't know about video and
all this stuff I have discussed all that
in my previous videos make sure to watch
those videos if you don't know so I
created a new file that is sign
up dot dto.ts so I actually just go to
the book dto to save some time and copy
uh this from here
copy
put that here I don't need here
this part
not in here this
so the first thing is going to be the
name of the user so that is going to be
string and it is not empty then we have
here the email that is going to be is
email decorator and then I pass in here
um the option
I type here message
please enter
correct email and this is going to be
password
and I can also Define one more decorator
here that is minimum length so main
length I type here 6 that minimum length
of the password is going to be 6. I
remove here is number from here and also
this enum
and that is
sign up dto Simply Save this one
and also I will simply copy that from
here create a new dto for the login so
login dot dto.ts put that here and here
we need here only email and the password
just simply remove this one and that is
it and this is
uh login video Simply Save this one we
have created our dtos I close this one
this one and here I give the type that
is going to be
sign up dto okay so now let's create our
controller for this signup I go to my
auth.controller.ts and then here I will
create my Constructor
and in that I will simply create add
here my
private variable that is going to be
auth service if you don't know private
variable can only be accessed inside a
class if you don't know about the o so
that's why we create here private
variable so that it can only be accessed
inside the class okay so auth service
and type is going to be auth service
like this and then that is going to be a
post
request
I type here slash sign up so it is going
to be slash auth slash sign up and then
here
I name the function
and
from the body I have to get my sign up
dto
okay so sign up
dto
and then
it will return a promise
and it
object we have token type is going to be
string
and in that I will simply return this
dot auth service DOT sign up and pass in
here the sign up dto
sign up dto
like this and oops that is only sign
update you
okay so now we will pass the sign up dto
which is the body in the sign up and it
will HD password to save this on the
database and assign the token to the
user now let's test our route so I
restart my server here and
[Music]
okay so there is not any error let's go
to the postman
I simply copy this from here this is
going to be a post request that is going
to be slash auth slash
sign up in the body row and let me type
here the data
and here you can see that I have passed
here incorrect email and the password is
also just five characters and if I click
on send from here you will see that
please enter correct email address and
password must be longer or equal to 6
characters if I type here at
now I will get only password error so I
type here 6.
so validation is working properly now if
I click on send from here you can see
that we get back here our token that
this means that user is created
successfully and this is 201 created if
I go to a website that is JWT dot IO
here we can actually see what is inside
the token so I simply go back uh and
copy this token from here
and here if I paste it you can see that
here we have the ID of the user here
okay so our token is created
successfully and we have successfully
saved our user also now let's create our
second route for the login
so I go back in the controller
sorting the service and then here
type here async login now in the login I
have to pass here login detail that will
contain email and the password of the
user so dto that is going to be
login dto and it will also return this
token
okay
and now first of all I need to get here
the
email and then the password from the
login dto
and now we have to first of all check
that if the user exists or not so I
simply type here const user is equal to
await this dot user model dot find one
and in that we will pass
the email so we'll check that if the
user exists with this email or not and I
check that if
not user we will simply throw new
unauthorized exception
and it is going to be invalid
invalid email or
password
okay and if the user exists with that
email now we have to validate the
password so I type here const is
password
matched I will use here bcrypt
bcrypt has a function that is called
compare in the compare we have to pass
first of all the password that user has
passed in the body so I type here the
password
then the second password which is saved
in the database which is the hashed
password so that is going to be user Dot
password okay again I checked that if
not password match then we will throw
this unauthorized exception
otherwise we have to assign the token so
I simply copy this from here and if the
email is ranked password is correct then
we will give back the token to the user
like this
Simply Save It Now go to alt controller
and I copy this from here
and let's make it get pass in here login
I also have to import it
that is going to be login
and that is
login
um
Dot Login okay and now if I simply save
it now let's test it out if I go to my
Postman
and I copy this from here
that is going to be login and the body
row
um I pass this
so if I type here wrong password like
this click on oops I have to remove this
name from here click on send you can see
that invalid email or password if I type
here correct password but wrong email
send then invalid email or password and
if I remove this from here click on send
you can see that we get back here our
token
and if I copy it from here go back and
put that here you can see that we have
our ID of the user did open so now we
have successfully created a two routes
one is for sign up and one is for the
login and we are also assigning the JWT
token to the user and in my next video I
will I will basically protect my routes
from the unauthenticated users and also
I will save the user uh in the book okay
so I will handle that in my next video I
hope that you understand if you have any
question you can definitely post your
question in the comment section and if
you want to check out my udemy course
about nestls I will also add the link in
the description of this video
so you can also find the source code in
the description of this video now in my
next video I will continue with the
authentication and protect my routes
from the unauthenticated users we will
create our JWT strategy
okay and then we also save our user in
the book all right so I will see you in
the next video
Browse More Related Video

Learn JWT in 10 Minutes with Express, Node, and Cookie Parser
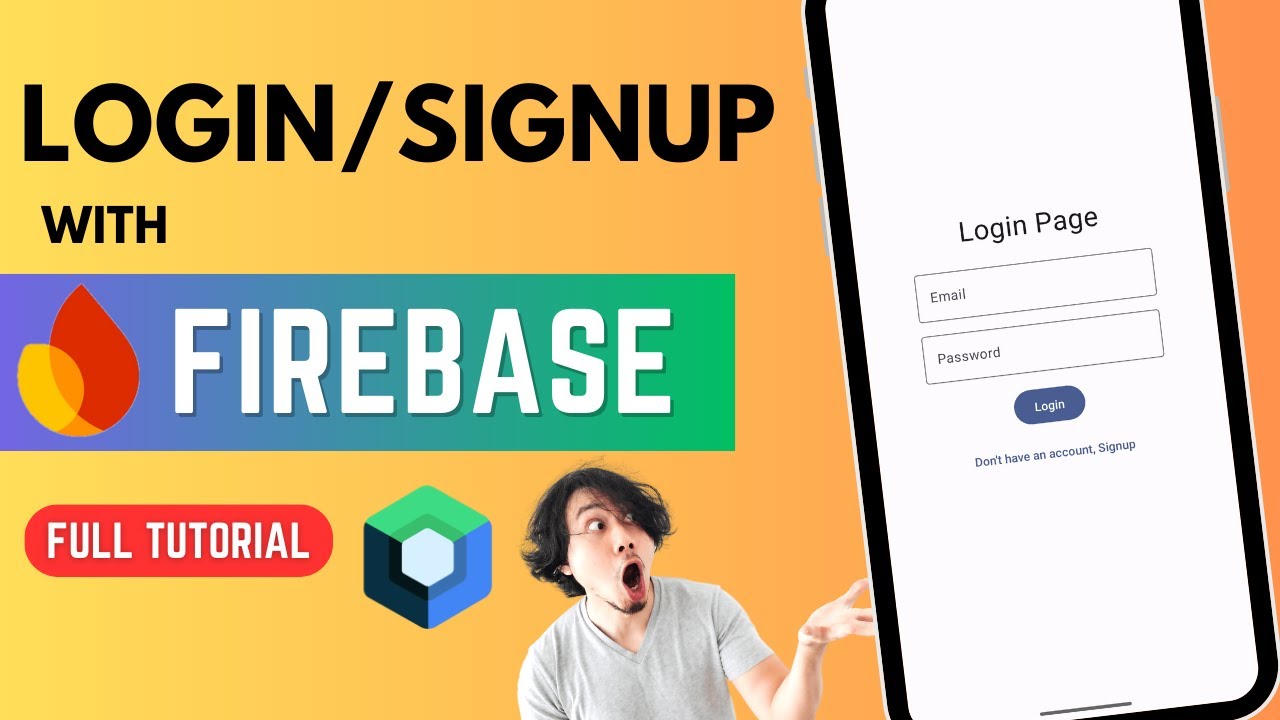
All about Firebase Authentication π₯ | Login & Signup | Jetpack Compose

Adding JWT Authentication & Authorization in ASP.NET Core
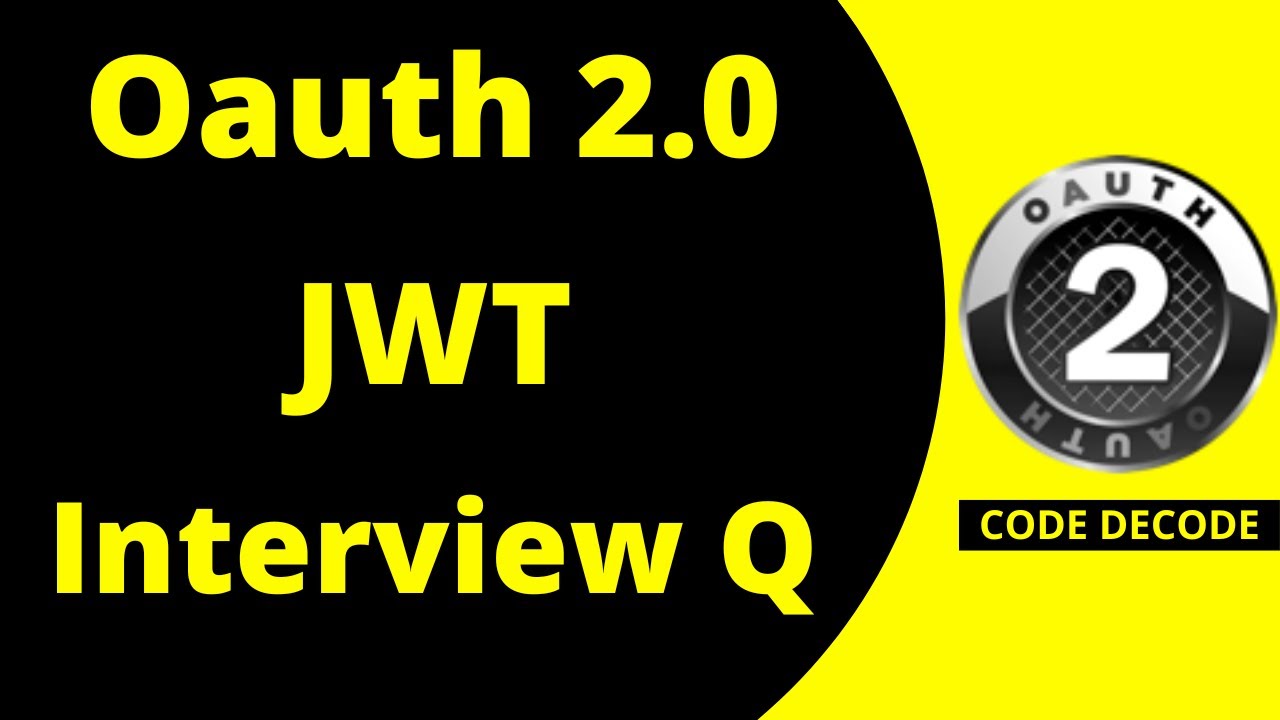
Oauth2 JWT Interview Questions and Answers | Grant types, Scope, Access Token, Claims | Code Decode
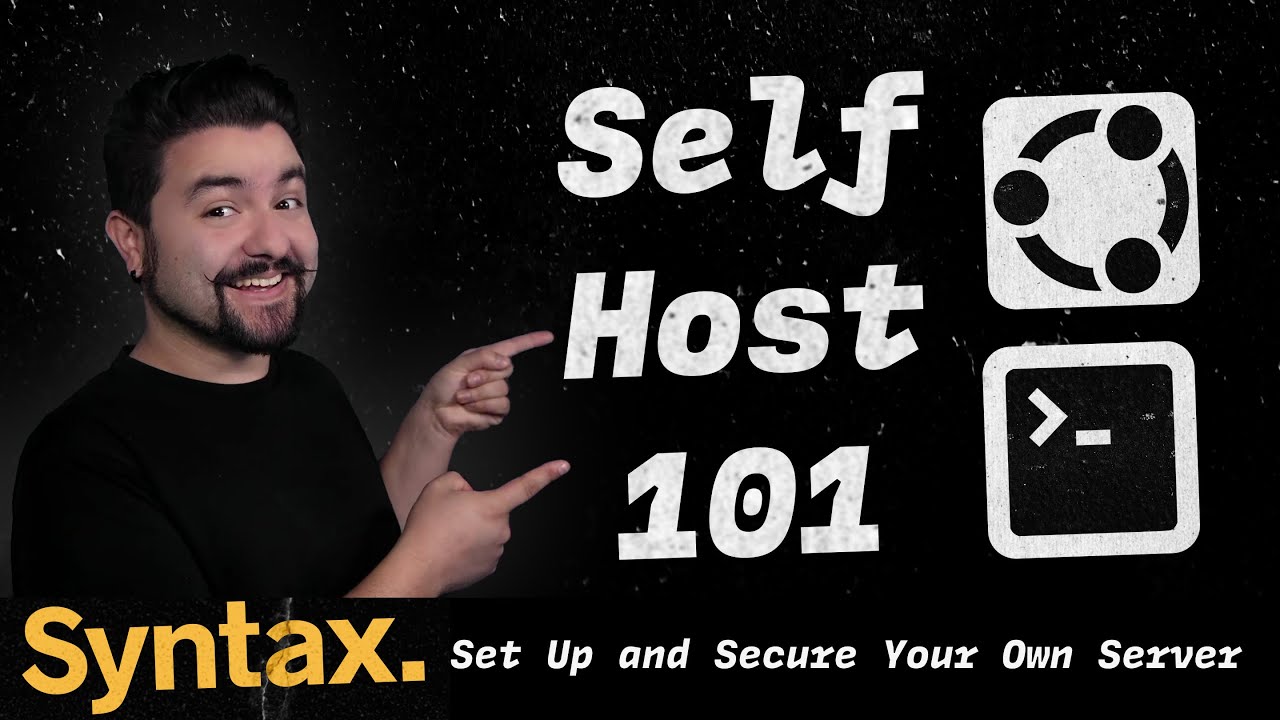
Self Host 101 - Set up and Secure Your Own Server

Build an API SaaS using Next.js, Prisma, Stripe in under an hour (Get paid for your API)
5.0 / 5 (0 votes)