All about Firebase Authentication 🔥 | Login & Signup | Jetpack Compose
Summary
TLDRThis video tutorial guides viewers on integrating Firebase authentication into an Android application. It covers creating a login and signup page, setting up seamless navigation between screens, and using a shared ViewModel to manage authentication status. The tutorial also demonstrates how to connect the app to Firebase, handle user registration, login, and logout processes, ensuring a smooth user experience with real-time UI updates.
Takeaways
- 🚀 The video tutorial covers integrating Firebase Authentication into an Android application, guiding through the creation of a login and signup page.
- 📱 It introduces the process of setting up a new Android Studio project with an empty activity and configuring it for Firebase integration.
- 🔒 The tutorial explains the concept of a ViewModel in Android, which is used to manage authentication status across different screens.
- 📝 It details the creation of methods within the ViewModel to handle authentication checks, login, signup, and logout functionalities.
- 🔄 The script discusses navigating between screens in the application, using a navigation controller to manage the flow.
- 📚 The importance of adding Firebase Authentication library to handle user authentication is emphasized, abstracting the complexity from the application code.
- 🔗 The process of connecting the Android application to Firebase and enabling email and password authentication in the Firebase console is outlined.
- 🛠️ The tutorial provides step-by-step instructions for designing the UI for login and signup pages, including input fields for email and password, and buttons for user actions.
- 🔑 The script explains how to implement the login and signup functionality by calling Firebase methods and handling the response to update the UI accordingly.
- ❗️ It highlights the importance of error handling during the authentication process, showing messages to the user when authentication fails.
- 🔄 The tutorial concludes with the implementation of the sign-out functionality, demonstrating how to return the user to the login page upon successful logout.
Q & A
What is the main topic of the video?
-The main topic of the video is integrating Firebase authentication into an Android application, including creating login and signup pages.
What are the three screens mentioned for the application architecture?
-The three screens mentioned are the signup page, homepage, and login page.
What is the purpose of the 'AuthViewModel' in the application?
-The 'AuthViewModel' is used to manage authentication status, provide methods for checking login status, logging in, signing up, and signing out, and to handle navigation between different screens based on the authentication state.
How does the application check if a user is already logged in?
-The application checks if a user is already logged in by using the 'checkAuthStatus' method in the 'AuthViewModel', which checks the current user state from Firebase authentication.
What is the role of the 'NavController' in the navigation flow?
-The 'NavController' is responsible for navigating between different screens of the application based on the user's authentication state and interactions, such as signing in or signing up.
How does the video script guide the implementation of the login page?
-The script guides the implementation of the login page by detailing the creation of UI elements like email and password input fields, a login button, and a text button to navigate to the signup page.
What is the significance of the 'AuState' sealed class in the ViewModel?
-The 'AuState' sealed class is used to represent different authentication states such as authenticated, unauthenticated, loading, and error, which helps in updating the UI accordingly.
How is the sign-up process implemented in the application?
-The sign-up process is implemented by calling the 'signup' method in the 'AuthViewModel' with the user's email and password, which then interacts with Firebase authentication to create a new user account.
What is the purpose of the 'signOut' method in the ViewModel?
-The 'signOut' method is used to sign out the current user by calling Firebase's sign-out function, which then updates the authentication state to unauthenticated, prompting navigation back to the login page.
How does the script handle user input validation for the login and signup processes?
-The script checks if the email and password fields are empty before attempting to log in or sign up. If they are empty, it prevents the login or signup process and can display an error message to the user.
What additional feature is implemented to enhance the user experience during the login and signup processes?
-The script implements a feature that disables the login and signup buttons while the authentication process is in the 'loading' state, preventing multiple submissions and providing a better user experience.
Outlines
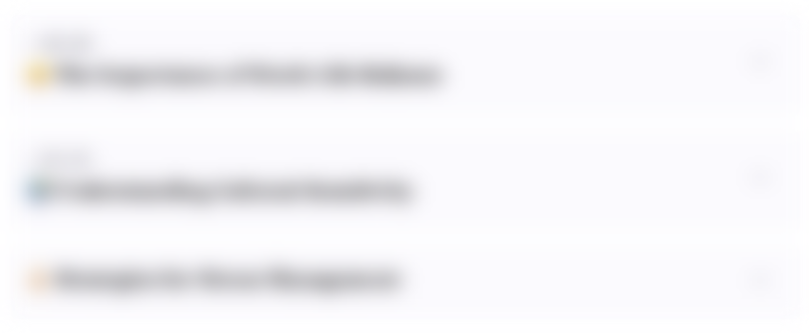
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
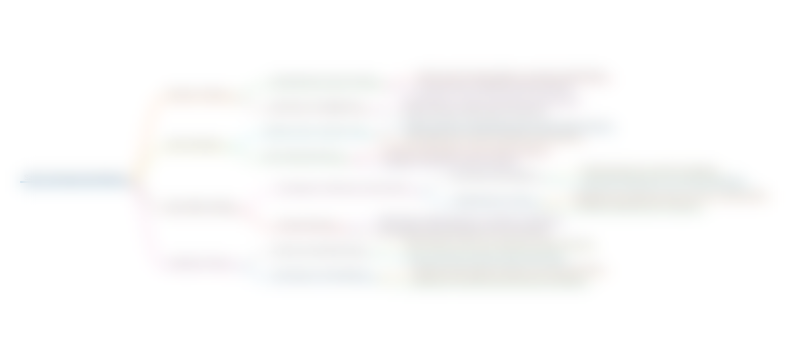
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
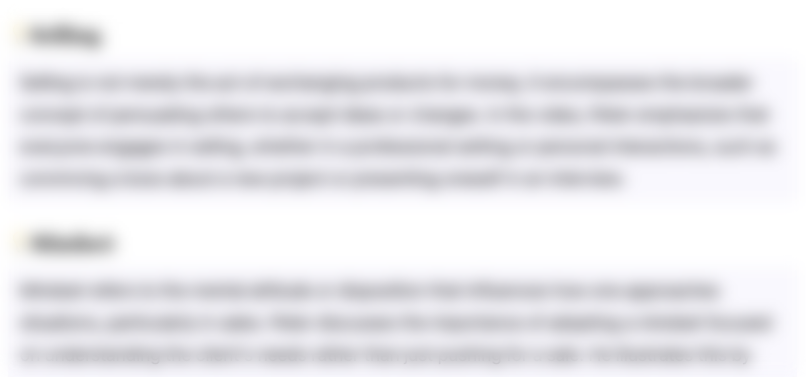
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
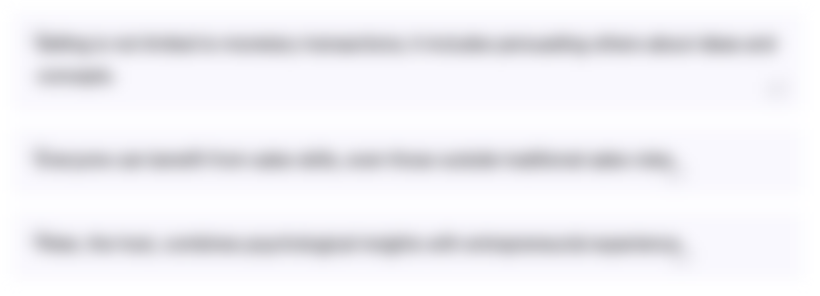
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
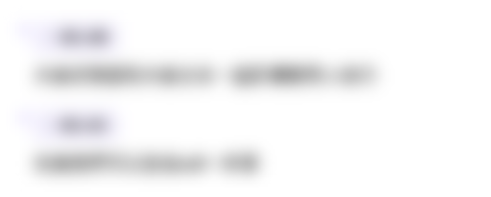
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
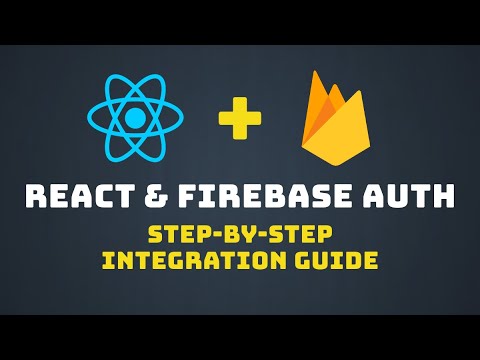
Setting Up Firebase Auth with React: Step-by-Step Tutorial
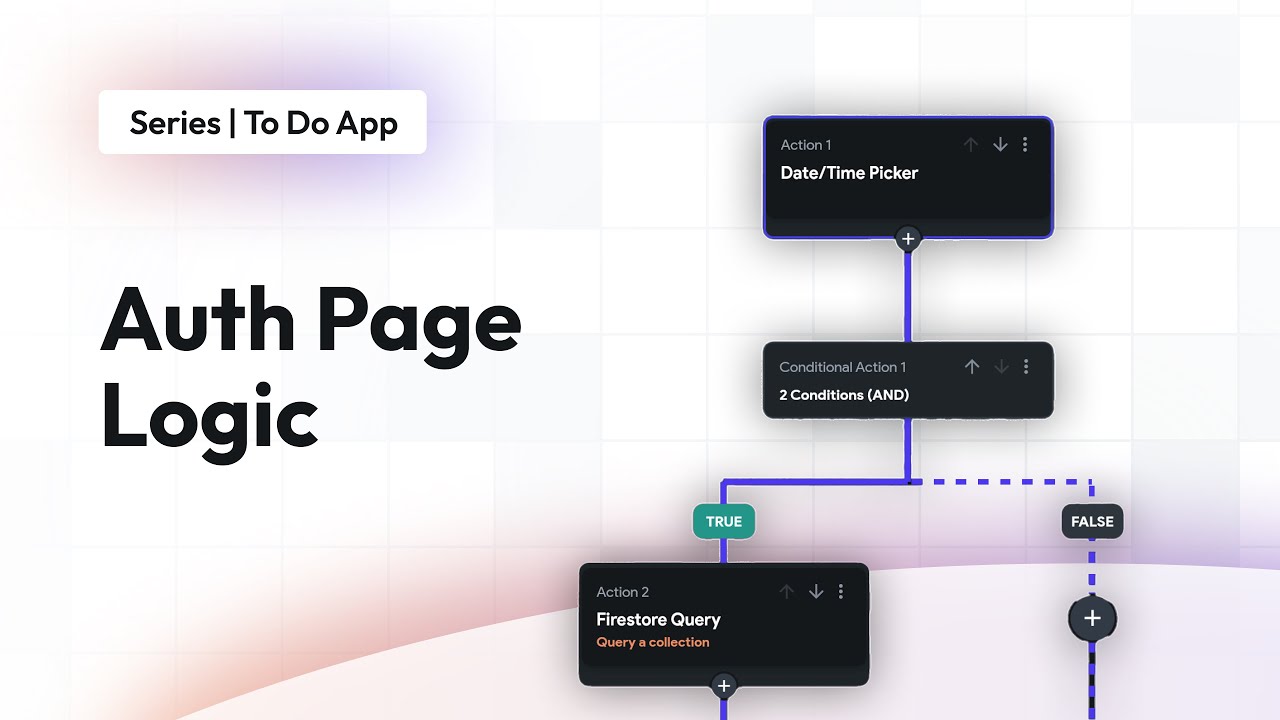
Auth Page Logic | To Do App | FlutterFlow for Beginners
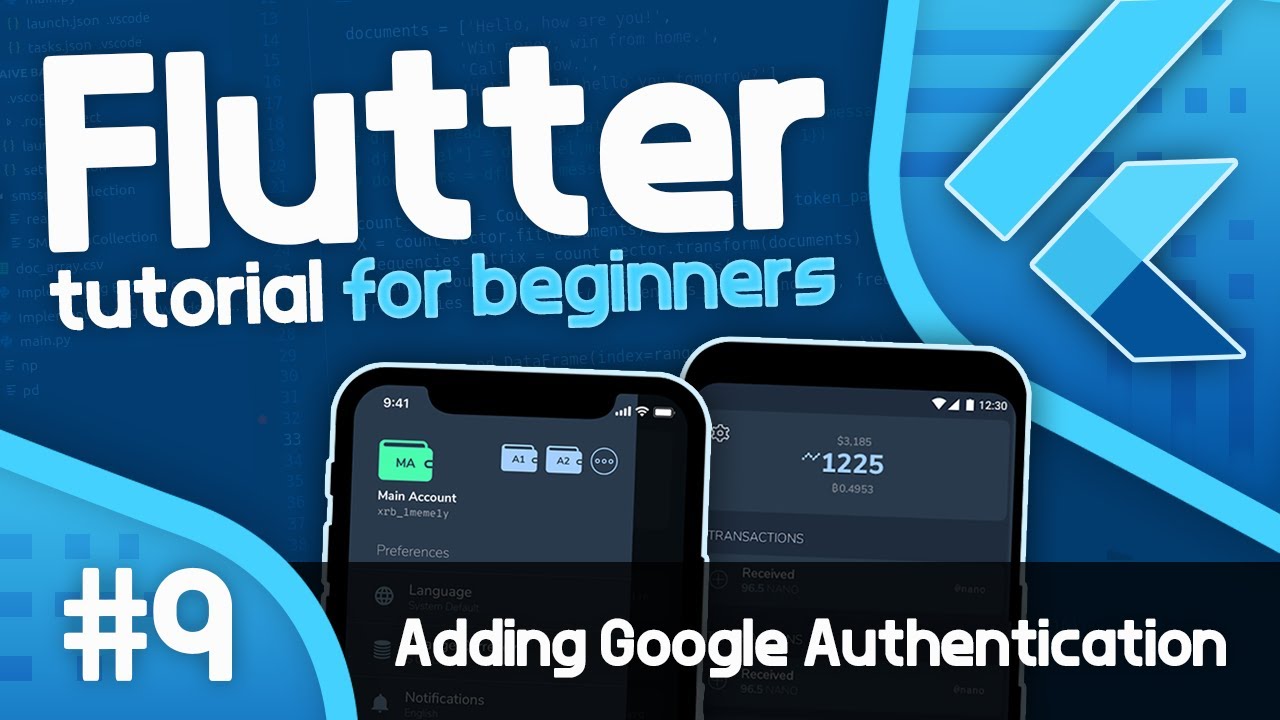
Flutter Tutorial For Beginners #9 - Adding Google Authentication with Firebase
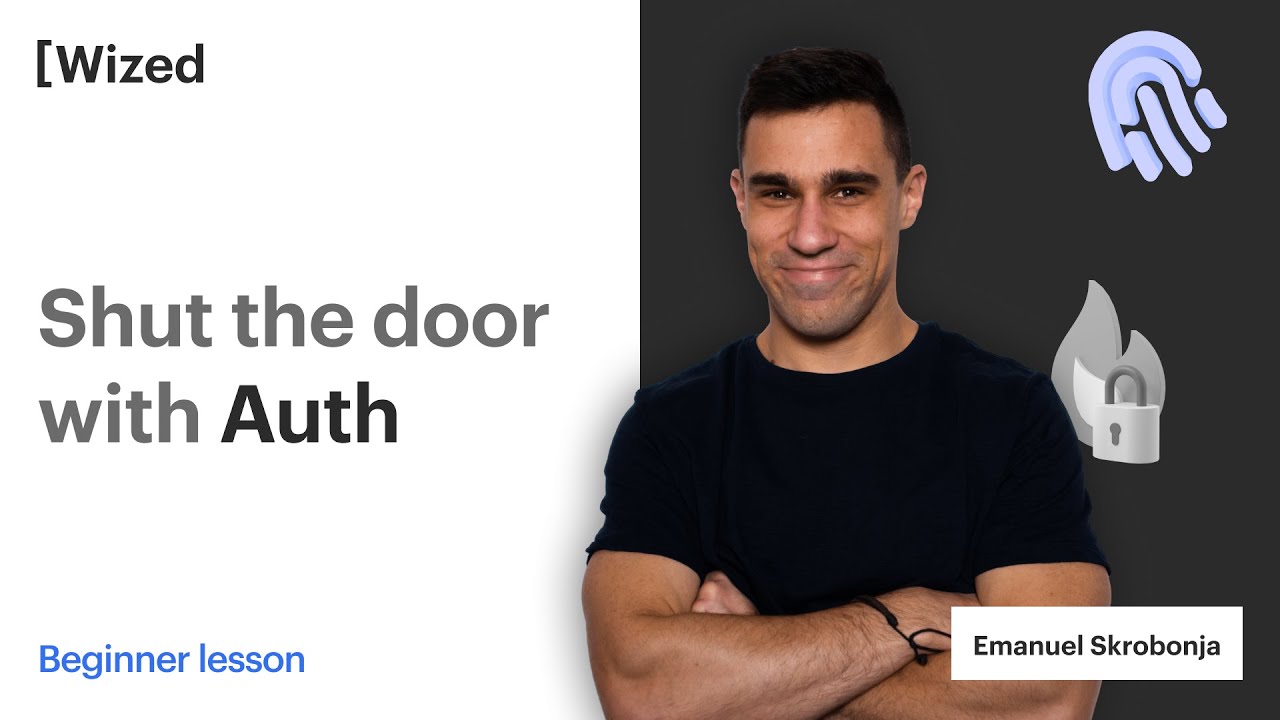
[Legacy] Use Firebase for Auth in Wized
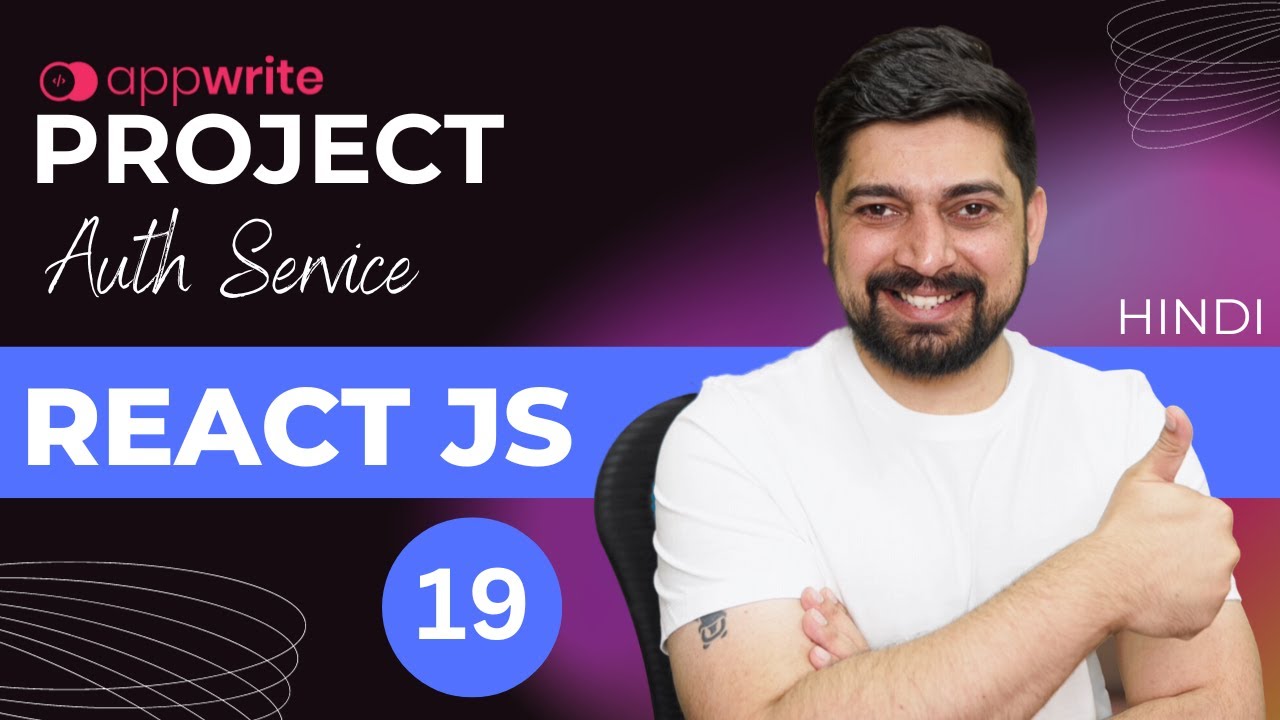
Build authentication service with appwrite
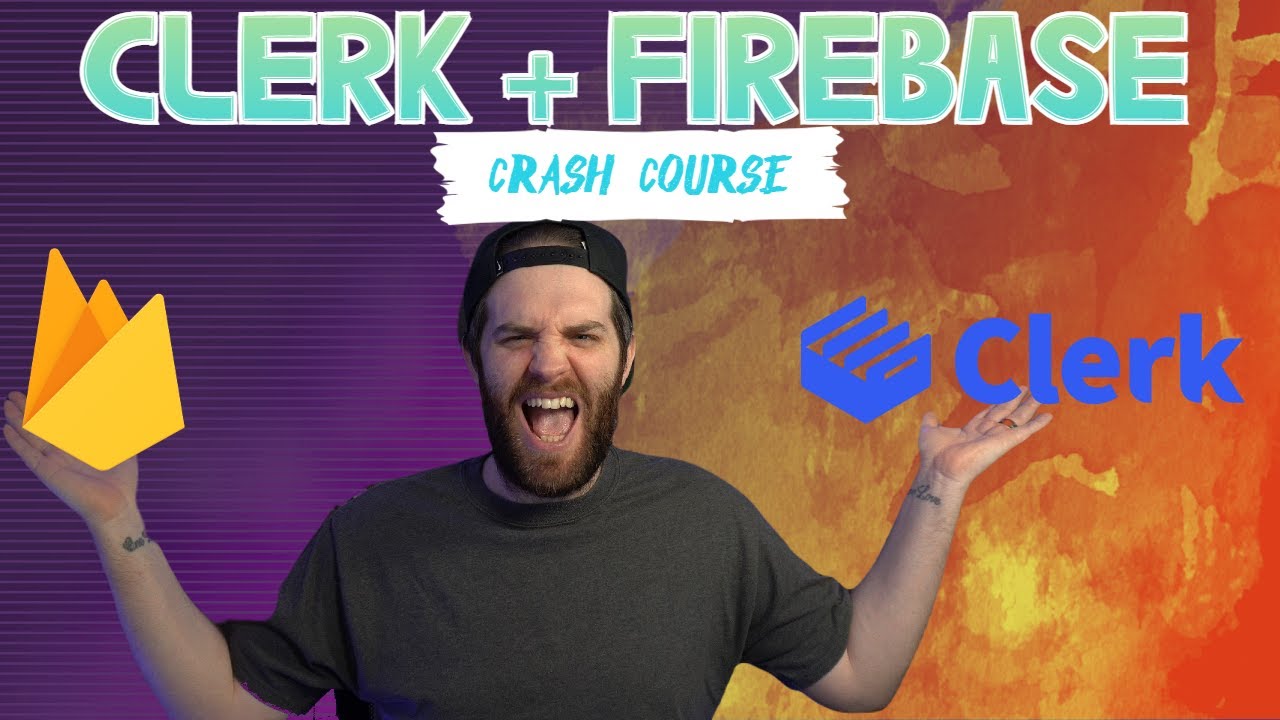
Clerk with Firebase Crash Course
5.0 / 5 (0 votes)