Adding JWT Authentication & Authorization in ASP.NET Core
Summary
TLDRIn this tutorial, Nick demonstrates how to integrate JSON Web Token (JWT) authentication and authorization into an ASP.NET Core application. He showcases the process using a sample movies API, explaining the importance of distinguishing between authentication and authorization. Nick guides viewers through adding the necessary NuGet package, configuring token validation parameters, and securing endpoints with the [Authorize] attribute. He also covers creating policies for role-based access control, using claims for authorization, and enhancing the developer experience with Swagger support for JWT.
Takeaways
- 😀 Nick introduces a tutorial on integrating JSON Web Token (JWT) authentication and authorization in an ASP.NET Core application.
- 🔒 The video focuses on the implementation of JWT, assuming viewers already understand the concept of JWTs and 'jot'.
- 📚 Nick references other in-depth videos on JWTs and suggests checking the description for more resources.
- 🎬 The demonstration uses a sample 'Movies API' to illustrate the process of adding JWT support.
- 🛠️ To begin, Nick shows how to add the 'Microsoft.AspNetCore.Authentication.Bearer' NuGet package for JWT support.
- 🔑 Configuration involves setting up authentication and authorization services, and using the 'AddBearer' method to configure token validation parameters.
- 📝 It's important to validate the token's issuer, audience, expiration, and signature to ensure its validity and security.
- 💡 For security, keys used for token generation and validation should be stored securely, not hard-coded in settings.
- 🛑 The order of middleware matters: authentication should come before authorization in the pipeline.
- 🚫 Without proper authentication, unauthorized access to the API would be unrestricted, which is demonstrated with the Movies API.
- 📝 Nick discusses two approaches for allowing public access to certain actions: using the 'AllowAnonymous' attribute or removing the 'Authorize' attribute from specific actions.
- 👮♂️ Authorization policies can be created based on claims within the JWT to restrict access, such as allowing only admins to delete movies.
- 🛠️ Custom attributes like 'RequiresClaimAttribute' can be used for fine-grained control over claims-based authorization.
- 📝 The video also covers how to integrate JWT authentication with Swagger, ensuring that endpoints can be tested with proper authentication.
Q & A
What is the main topic of the video by Nick?
-The main topic of the video is how to add JSON Web Token (JWT) authentication and authorization support in an ASP.NET Core application.
Why is Nick not explaining what a JSON Web Token is in this video?
-Nick is not explaining what a JSON Web Token is because he already has a separate video on that topic, and he wants to focus on the implementation in ASP.NET Core specifically.
What is the purpose of the 'Movies API' shown in the video?
-The 'Movies API' is used as an example to demonstrate how to implement JWT authentication and authorization in an ASP.NET Core application.
What does Nick demonstrate with the 'Movies API' before adding JWT support?
-Nick demonstrates creating, listing, updating, and deleting movies in the API, showing that initially, there is no authentication or authorization required to perform these actions.
What NuGet package does Nick recommend to add JWT support?
-Nick recommends adding the 'Microsoft.AspNetCore.Authentication.JwtBearer' NuGet package to implement JWT support.
What is the difference between authentication and authorization as explained in the video?
-Authentication is the process of verifying that someone is who they claim to be, while authorization is the process of verifying that someone has access to what they claim to have access to.
How does Nick suggest storing the JWT secret key for security purposes?
-Nick suggests storing the JWT secret key securely in a service designed to store tokens, such as Azure Key Vault or AWS Secrets Manager, rather than hardcoding it in the settings.
What are the minimum token validation parameters that Nick mentions should be specified?
-The minimum token validation parameters mentioned are validating the issuer, audience, ensuring the token is not expired, and validating the signature.
How does Nick demonstrate passing a JWT to authenticate a request?
-Nick demonstrates passing a JWT by setting the 'Authorization' header in Postman with the value 'Bearer ' followed by the actual token.
What are the two approaches Nick discusses for allowing anonymous access to certain actions in the API?
-The two approaches are using the 'AllowAnonymous' attribute on specific actions to override the controller-based behavior, or removing the 'Authorize' attribute from the controller and applying it individually to actions that require it.
How does Nick explain creating a policy for role-based authorization?
-Nick explains creating a policy by using the 'AddPolicy' method in the 'AuthorizationOptions', defining a name for the policy, and specifying the required claim name and value using a 'PolicyBuilder'.
What is the alternative method to policies for claim-based authorization that Nick presents?
-The alternative method is creating a custom attribute called 'RequiresClaimAttribute' that can be used to validate claims directly on actions or controllers without defining a policy.
How does Nick address adding Swagger support for JWT authentication?
-Nick explains creating a 'Swagger' directory with specific configuration files to define security schemes and requirements, and then registering these configurations in the 'Program.cs' to enable JWT authentication in Swagger UI.
Outlines
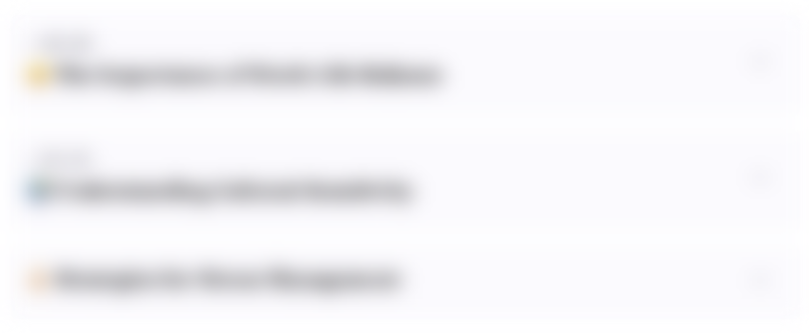
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
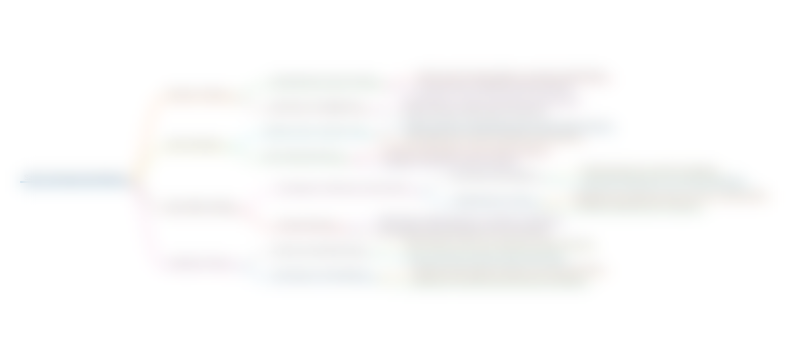
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
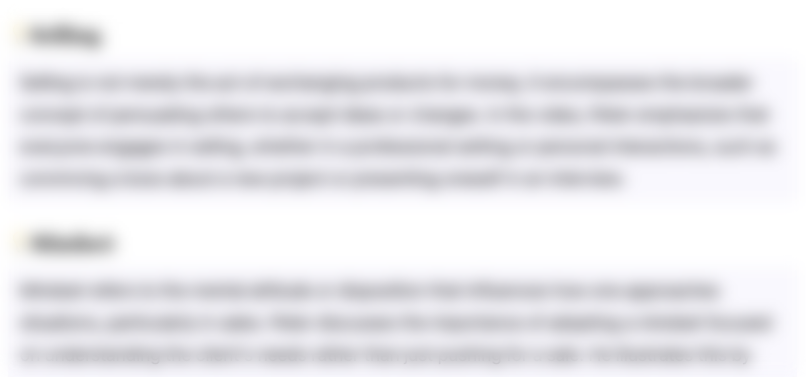
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
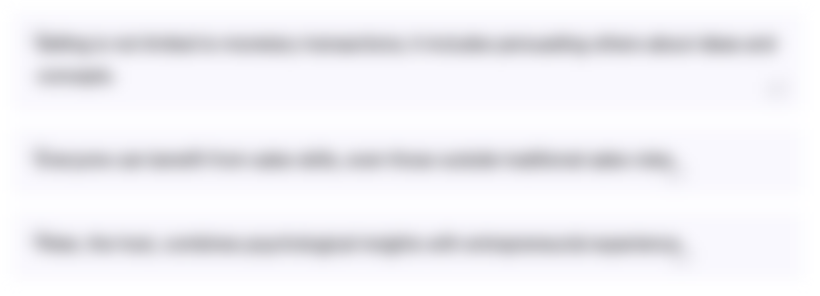
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
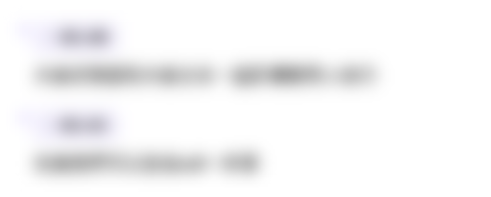
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Policy, AuthorizationRequirement & AuthorizationHandler | ASP.NET Core Identity & Security | Ep 8
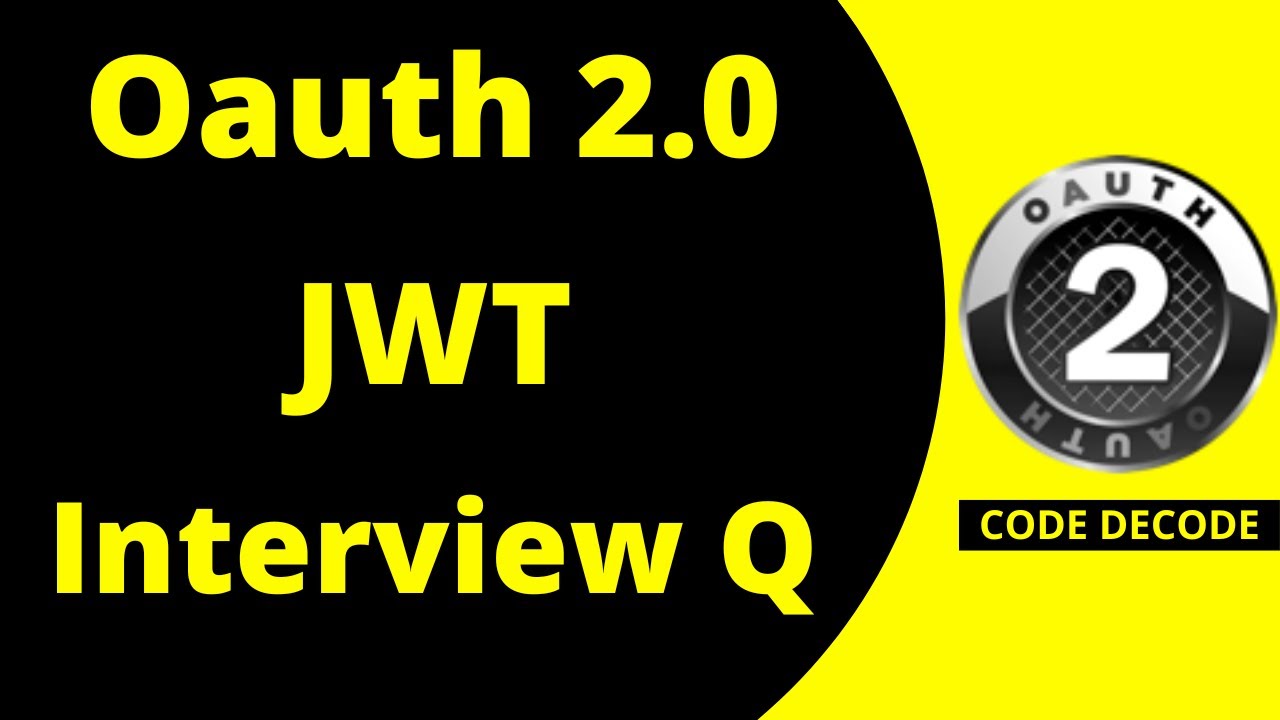
Oauth2 JWT Interview Questions and Answers | Grant types, Scope, Access Token, Claims | Code Decode

Secure Your Microservices with Keycloak | OAuth2 & JWT | Spring Cloud Gateway
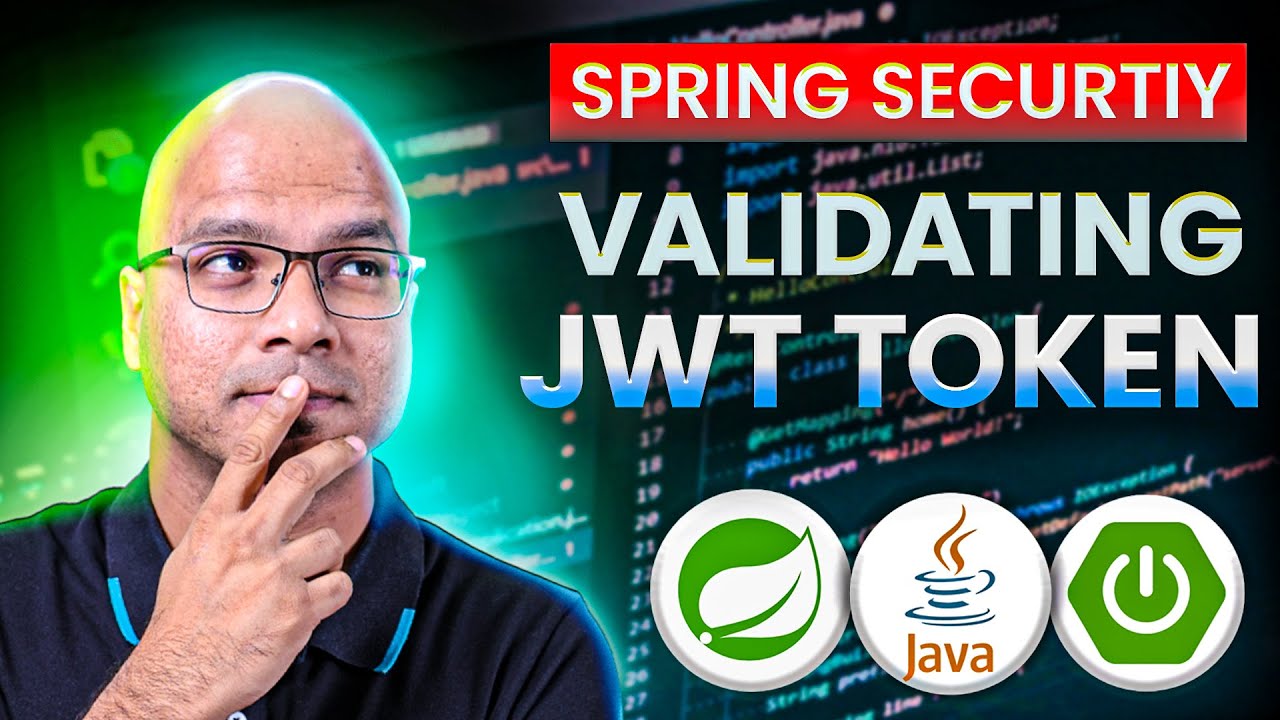
#38 Spring Security | Validating JWT Token

Learn JWT in 10 Minutes with Express, Node, and Cookie Parser
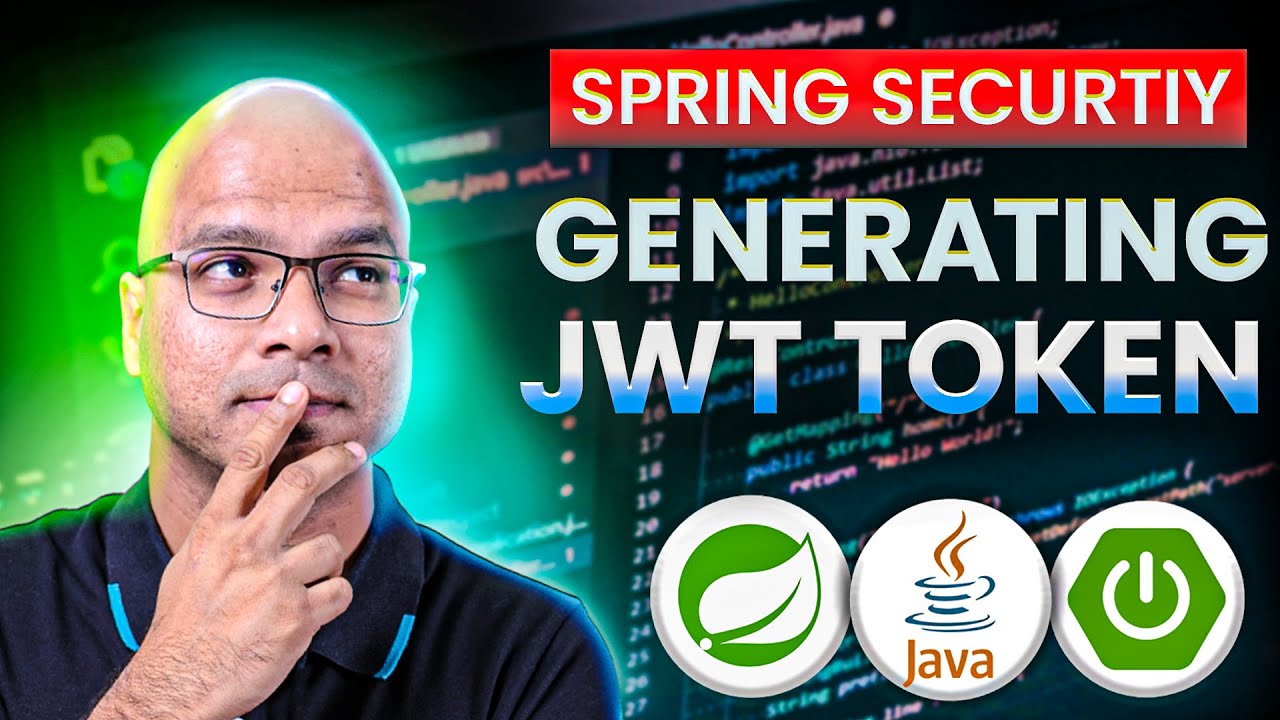
#37 Spring Security | Generating JWT Token
5.0 / 5 (0 votes)