COS 333: Chapter 7, Part 1
Summary
TLDRIn the previous lecture, we completed our discussion on data types. This lecture begins with an introduction to expressions and assignment statements, focusing primarily on arithmetic expressions, overloaded operators, and type conversions. Key topics include the evaluation order of operators and operands, operator precedence, and associativity rules. The lecture also covers implementation details in Ruby, Scheme, Common Lisp, and conditional expressions in C-based languages. Additionally, it discusses operand evaluation, operator overloading, and type conversions, concluding with common errors in expressions and their implications.
Takeaways
- 📚 The lecture introduces Chapter 7 focusing on expressions and assignment statements, emphasizing the importance of understanding details specific to different programming languages.
- 🧠 Expressions are the fundamental means of specifying computations in programming languages, including arithmetic and logical tests, with evaluation order depending on operator precedence and associativity rules.
- 🔢 Arithmetic expressions are central to programming, with operators and operands defining the computation, and the use of parentheses to modify evaluation order.
- 🔄 The design of arithmetic expressions in programming languages involves considerations of operator precedence, associativity, operand evaluation order, and the allowance of user-defined operator overloading.
- 🕵️♂️ Operator overloading, both built-in and user-defined, can aid readability but may also lead to confusion and errors if not implemented carefully.
- 🔄 Type conversions, both narrowing and widening, are crucial for mixed mode expressions, with the latter generally being safer due to the preservation of value magnitude.
- 🛑 Errors in expressions can arise from type checking, coercion, inherent arithmetic limitations, and computer arithmetic limitations like overflow and underflow.
- 🌐 The script touches on how different programming languages handle expressions, such as Ruby implementing all operators as methods, allowing for overrides, and Scheme treating operators as function calls.
- 📈 The importance of operand evaluation order is highlighted, noting that it can affect the efficiency of a program and the potential for side effects.
- 📝 Assignment statements, which are central to imperative programming languages, model the pipelining concept of the von Neumann architecture, connecting memory and the processing unit.
- 🔍 The upcoming lecture will continue the discussion on expressions, covering relational and boolean expressions, short circuit evaluation, and conclude with assignment statements.
Q & A
What is the main focus of the lecture on chapter 7?
-The lecture on chapter 7 primarily focuses on expressions and assignment statements, including a detailed discussion on arithmetic expressions, operator overloading, and type conversions.
Why is it important to pay attention to details when discussing expressions in different programming languages?
-It is important to pay attention to details because specific programming languages may implement certain features differently, which can affect how expressions are evaluated and behave in those languages.
What are the two main kinds of expressions mentioned in the script?
-The two main kinds of expressions mentioned are arithmetic expressions, which perform mathematical computations, and relational and boolean expressions, which allow for constructing logical tests and dealing with their outcomes.
How does the von Neumann architecture relate to imperative programming languages?
-Imperative programming languages are closely modeled on the von Neumann architecture, which features a shared memory for both instructions and data, and a pipeline connecting memory and the processing unit, reflecting the central role of assignment statements and variables in these languages.
What is the significance of operator precedence in evaluating arithmetic expressions?
-Operator precedence defines the order in which adjacent operators of different precedence levels are evaluated in an arithmetic expression, ensuring that operations are performed in a structured and predictable manner.
What are the typical associativity rules for binary operators in programming languages?
-The typical associativity rules for binary operators use left-to-right associativity, meaning that operations at the same precedence level are performed from left to right, except for power operators which usually have right-to-left associativity.
How does Ruby implement its operators differently from other programming languages?
-In Ruby, all operators are implemented as methods, which allows for the possibility of overriding them by the programmer, thus changing how the operators behave, unlike in languages such as C++ or Java.
Why is operator overloading considered both beneficial and problematic in programming languages?
-Operator overloading can be beneficial as it allows for more natural expressions of operations, especially with user-defined data structures. However, it can also be problematic as it may lead to a loss of readability, difficulty in understanding the operator's purpose without knowing the operand types, and potential for nonsensical operations if not implemented carefully.
What are the two types of type conversions discussed in the script, and how do they differ in safety?
-The two types of type conversions are narrowing conversions, which are considered unsafe because they can result in a loss of value magnitude, and widening conversions, which are generally safe as they preserve the value's magnitude, though potentially with some loss of accuracy.
Why might a programming language choose to use widening conversions over narrowing conversions in expressions?
-Widening conversions are generally preferred over narrowing conversions because they are safe as they preserve the magnitude of the original value, even if some accuracy might be lost, whereas narrowing conversions can result in a significant change or loss of the original value.
What are some of the errors that can arise due to the limitations of arithmetic in expressions?
-Errors due to the limitations of arithmetic in expressions can include division by zero, which results in an undefined value, and overflow or underflow, which occur when a computation produces a value that is outside the representable range of the type.
Outlines
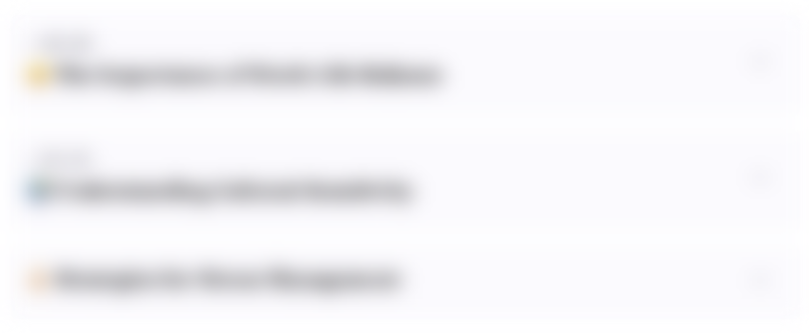
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
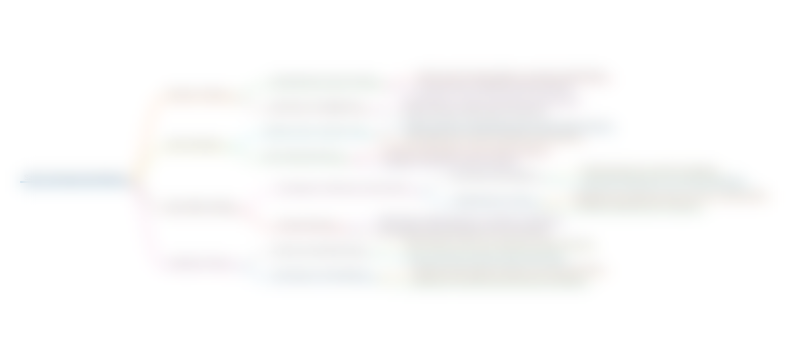
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
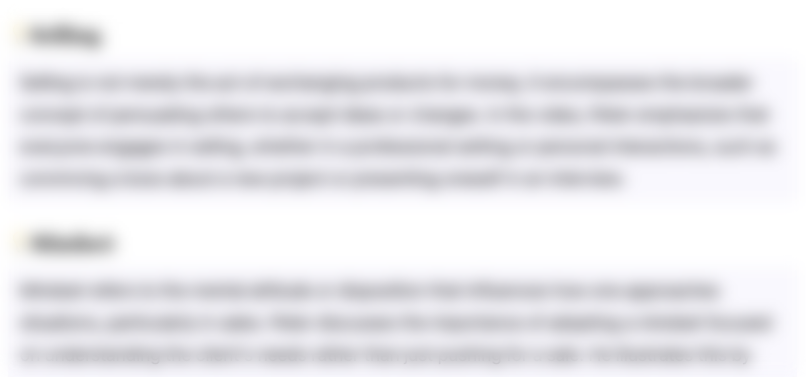
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
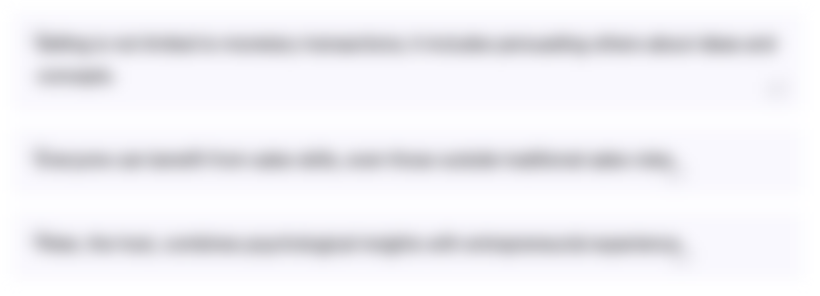
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
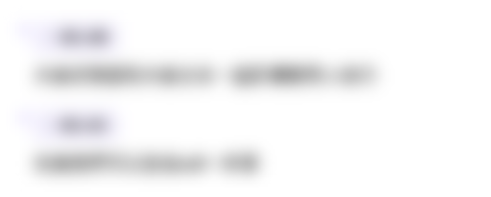
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
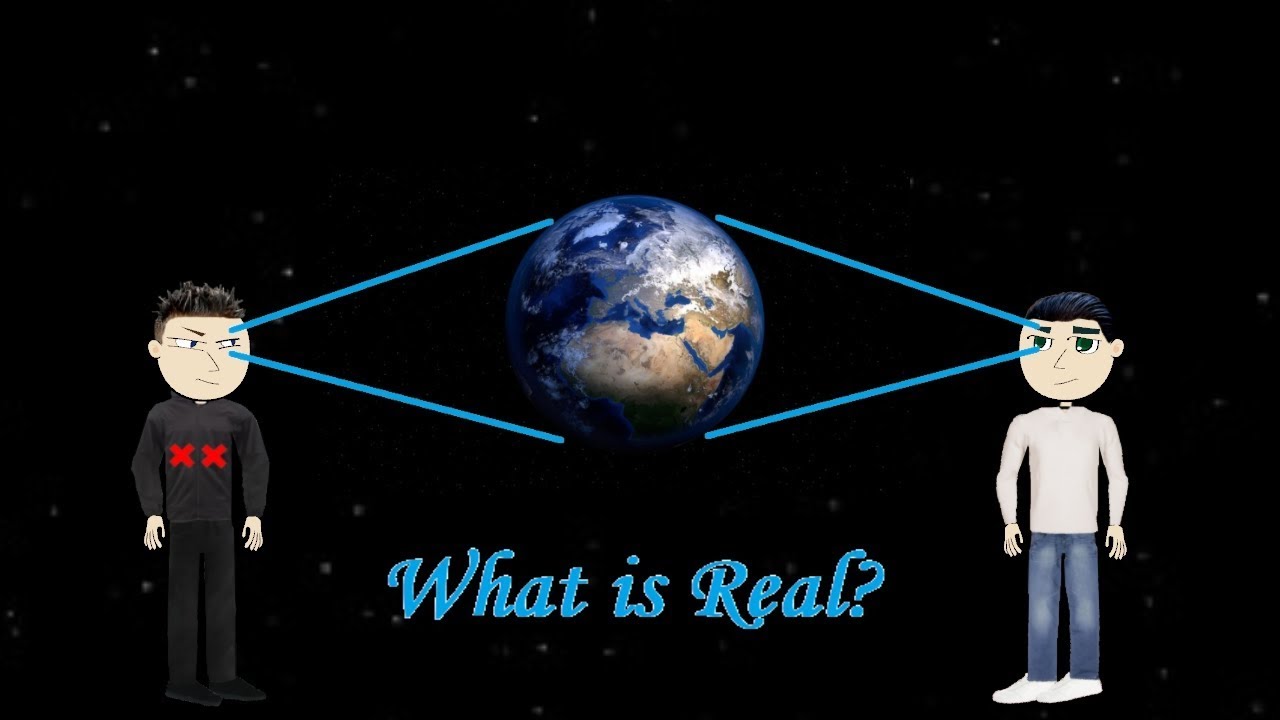
Knowledge of the External World (Direct Realism, Indirect Realism & Idealism)
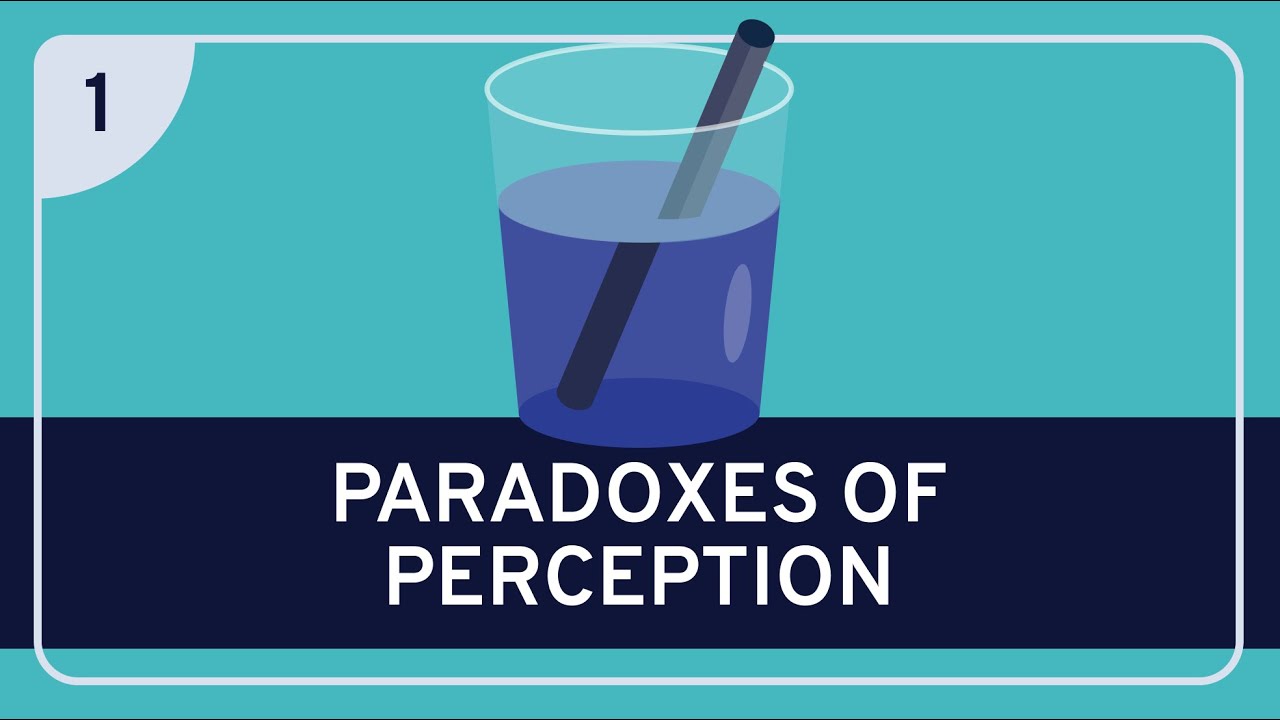
PHILOSOPHY - Epistemology: Paradoxes of Perception #1 (Argument from Illusion) [HD]
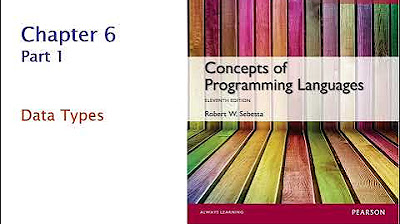
COS 333: Chapter 6, Part 1
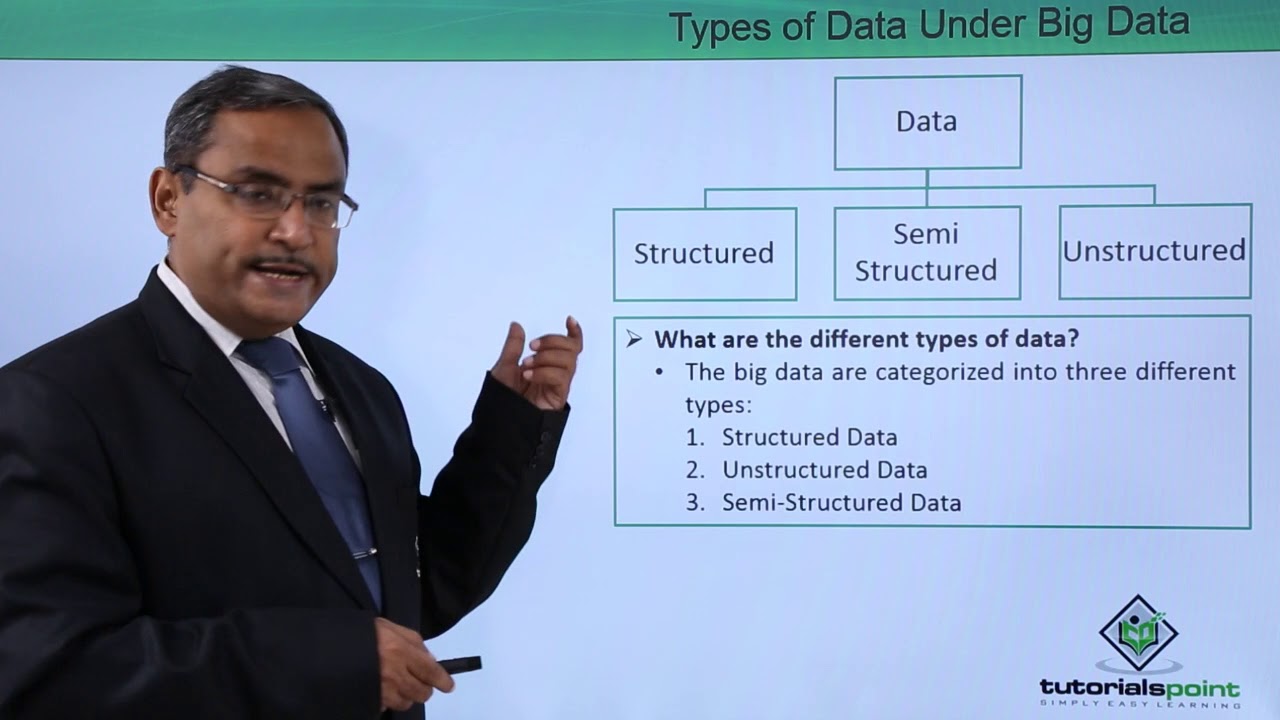
Types of Data Under Big data
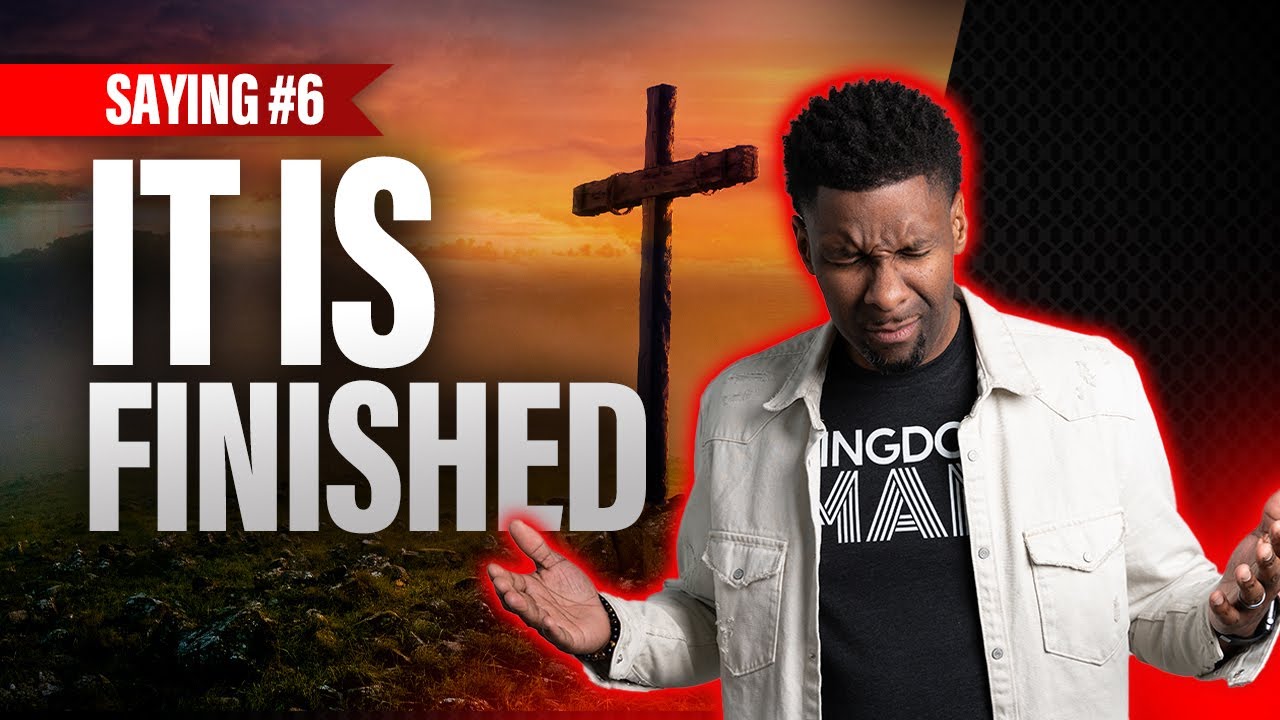
"It Is Finished!" | Saying #6 (7 Last Words of Jesus)

Are Entities, Aliens and Ghosts ticklish?
5.0 / 5 (0 votes)