Destructors in a Class | C++ Object Oriented Tutorial
Summary
TLDRIn this C++ programming tutorial by Anil, viewers are introduced to destructors, the counterpart to constructors. Destructors are special member functions executed when an object goes out of scope or is deleted, ensuring proper resource cleanup. The tutorial demonstrates creating a 'Human' class with both a constructor and destructor, using the 'new' keyword to create an object and 'delete' to deallocate its memory, illustrating the sequence of constructor and destructor calls. Anil emphasizes the importance of destructors in managing memory and their restrictions, such as no parameters or return values, before concluding with a reminder to subscribe for more educational content.
Takeaways
- π The script is a C++ programming tutorial focused on destructors.
- π¨ Destructors in C++ are special member functions that execute when an object goes out of scope or is deleted.
- π Destructors are the opposite of constructors, which are called when an object is created.
- π The destructor is declared with the `~` symbol followed by the class name and has no parameters or return values.
- π·ββοΈ The tutorial demonstrates creating a class named 'human' with both a constructor and a destructor.
- π¬ The destructor is used to execute statements before the object's memory is released.
- π The scope resolution operator can be used to define the destructor outside the class.
- ποΈ Demonstration includes creating an object with the 'new' keyword and deleting it to trigger the destructor.
- π When an object is created, the constructor is called, and when it's destroyed, the destructor is called.
- π οΈ The tutorial includes a practical example of creating and deleting an object to illustrate the use of destructors.
- π The presenter encourages viewers to subscribe for more tutorials and ends with a reminder for the next session.
Q & A
What is the purpose of a destructor in C++?
-A destructor in C++ is a special member function of a class that is executed whenever an object of its class goes out of scope or when the delete expression is applied to a pointer to the object. It is used to release the memory allocated for the object and perform any necessary cleanup before the object is destroyed.
How is a destructor different from a constructor in C++?
-A constructor is a special member function that is called when a new object of a class is created, while a destructor is called when an object is about to be destroyed. Unlike constructors, destructors cannot take parameters and cannot return any values.
What symbol is used to declare a destructor in C++?
-The tilde symbol (~) is used to declare a destructor in C++, followed by the class name and a pair of parentheses.
Can a destructor be defined outside the class using the scope resolution operator?
-Yes, a destructor can be defined outside the class using the scope resolution operator, which is the double colon (::).
What happens when an object is created using the 'new' keyword in C++?
-When an object is created using the 'new' keyword in C++, the constructor of the class is called to initialize the object, and memory is allocated for it.
What is the role of the 'delete' keyword in C++?
-The 'delete' keyword in C++ is used to deallocate the memory that was previously allocated for an object using the 'new' keyword. It also calls the destructor of the object.
What is the correct syntax for defining a destructor in a C++ class?
-The correct syntax for defining a destructor in a C++ class is to use the tilde symbol (~), followed by the class name, a pair of parentheses, and then the body of the destructor enclosed in curly braces.
When is the destructor called for an object created within a function scope?
-The destructor for an object created within a function scope is called when the function execution is completed and the object goes out of scope.
Can destructors have parameters or return values?
-No, destructors in C++ cannot have parameters and cannot return any values. They are meant for cleanup and releasing resources.
What is the significance of the 'see out and' statement used in the destructor example?
-The 'see out and' statement in the destructor example is likely a placeholder for a more meaningful action or message that should be executed or displayed when the destructor is called. It serves as a demonstration of where such actions would occur.
How can you observe the effect of a destructor in a C++ program?
-You can observe the effect of a destructor in a C++ program by creating an object, running the program to see the constructor message, then deleting the object or allowing it to go out of scope and observing the destructor message.
Outlines
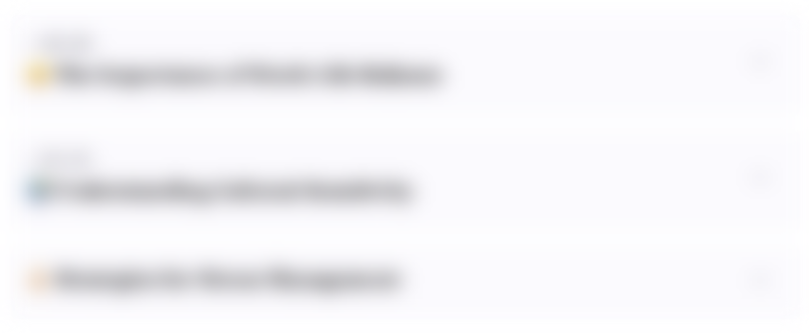
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
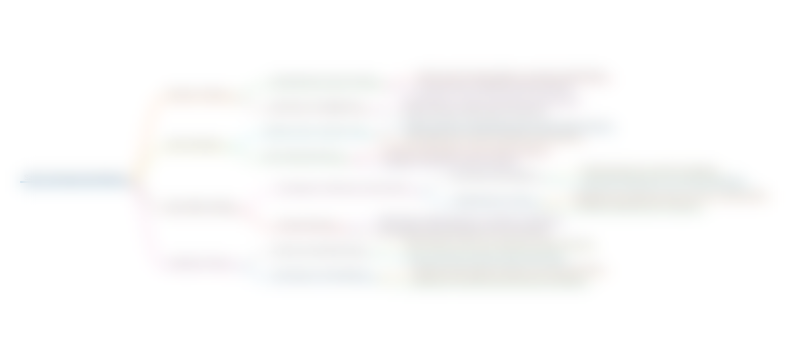
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
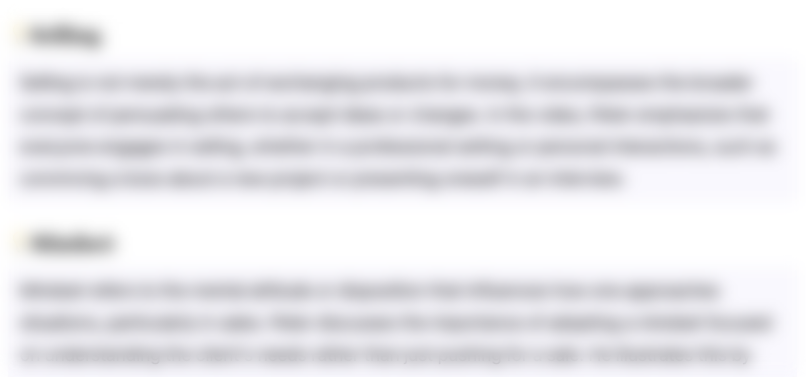
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
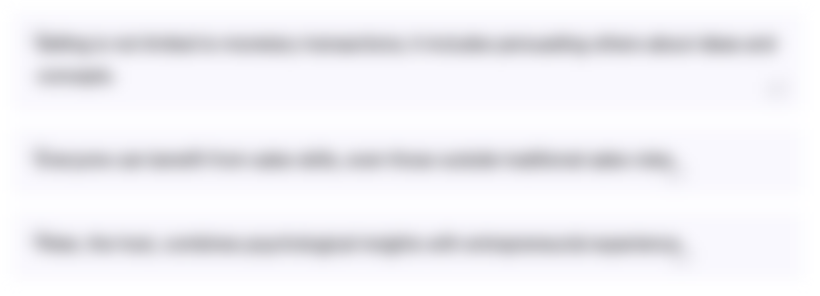
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
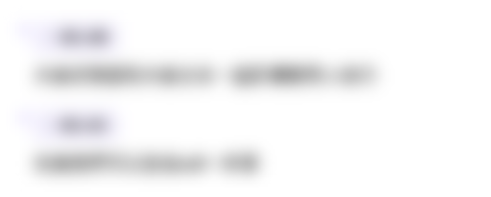
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)