C++ Inheritance: constructors and destructors in base and derived classes [3]
Summary
TLDRIn this video, Professor Hank explains constructors and destructors in C++ within the context of inheritance. He covers the order of execution for base and derived class constructors and destructors, demonstrating how the base class constructor is invoked before the derived class constructor, and vice versa for destructors. Hank also shows how to pass arguments from the derived class to the base class constructor using an initializer list, and discusses inheriting constructors from the base class to simplify the derived class code. The video provides a thorough yet clear explanation, making these important C++ concepts more accessible.
Takeaways
- 😀 The base class constructor executes first, followed by the derived class constructor when objects are created in inheritance.
- 😀 The destructor order is the opposite: the derived class destructor is called first, then the base class destructor.
- 😀 Constructors can be invoked from the derived class to initialize the base class members using the ': base(x, y)' syntax.
- 😀 A base class constructor can be inherited by the derived class using the 'base::base' syntax, eliminating the need to write a separate constructor in the derived class.
- 😀 The default constructor of the base class initializes private variables 'A' and 'B' to zero, while the overloaded constructor allows passing values to initialize them.
- 😀 The base class constructor can be invoked in the derived class constructor using arguments passed from the derived class.
- 😀 You can add extra variables to the derived class constructor and use them in combination with the base class constructor for further initialization.
- 😀 The 'print' function in the base class prints the values of 'A' and 'B' for testing purposes, showing how object data can be accessed.
- 😀 Dynamic memory allocation in the main function can trigger the constructor and destructor executions, allowing us to see the order of execution in practice.
- 😀 The syntax for calling a constructor from the base class within the derived class allows for flexibility in passing parameters and initializing base class data members.
- 😀 If the derived class does not need its own constructor functionality, it can simply inherit constructors from the base class for easier code management.
Q & A
What is the order of execution for constructors and destructors in C++ inheritance?
-In C++ inheritance, the order of execution is as follows: The base class constructor is executed first, followed by the derived class constructor. For destructors, the order is reversed: the derived class destructor is executed first, followed by the base class destructor.
How can a derived class invoke the base class constructor?
-A derived class can invoke the base class constructor by using an initialization list in its own constructor. This involves calling the base class constructor and passing the required arguments to it, for example: 'BaseClassName(arguments);'.
What is the purpose of the 'print' function in the base class?
-The 'print' function in the base class is used to display the values of the private variables 'A' and 'B'. It helps for testing and verifying the values of these variables after creating an object.
What does dynamic memory allocation with 'new' do in the script?
-Using 'new' in the script creates a dynamic instance of the derived class, allowing for the allocation of memory on the heap. This enables the triggering of both the constructor and destructor when the object is created and deleted, respectively.
How does the derived class constructor pass arguments to the base class constructor?
-The derived class constructor passes arguments to the base class constructor through an initialization list. It calls the base class constructor with the arguments, and these arguments are used to initialize the base class's member variables.
Can the derived class constructor have additional functionality besides invoking the base class constructor?
-Yes, the derived class constructor can perform additional functionality, such as initializing its own member variables or adding extra logic. The base class constructor is invoked first, followed by the derived class constructor's body.
What happens if the derived class does not have its own constructor?
-If the derived class does not have its own constructor, it will inherit the constructor from the base class. The constructor of the base class will be used to initialize the derived class object, as long as the derived class does not need any specific initialization.
Why might a derived class choose to inherit the base class constructors?
-A derived class might inherit the base class constructors if it does not require additional initialization beyond what the base class constructor provides. This simplifies the code and reduces the need for redundant constructor definitions.
What is the role of the destructor in both the base and derived classes?
-The destructor is responsible for cleaning up resources when an object is destroyed. In the context of inheritance, the base class destructor is executed after the derived class destructor, ensuring proper cleanup of resources in the correct order.
How does the script demonstrate the use of constructors and destructors in C++ inheritance?
-The script demonstrates constructors and destructors in C++ inheritance by first creating a base class with constructors and a destructor. It then defines a derived class that calls the base class constructor and has its own destructor. The order of execution is shown when an object is created and deleted using dynamic memory allocation.
Outlines
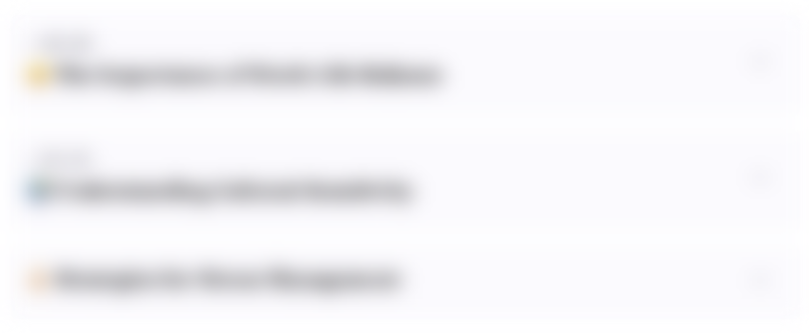
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
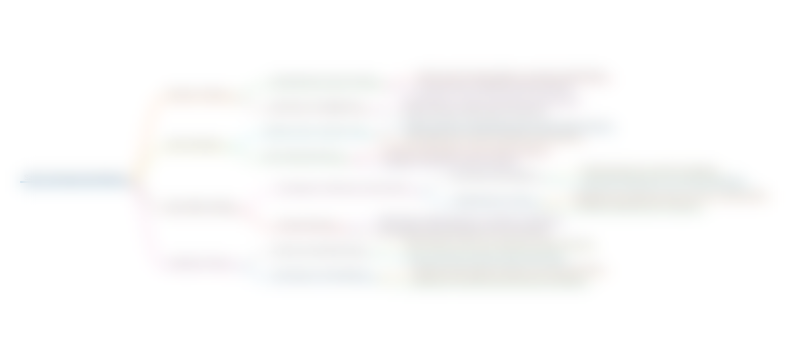
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
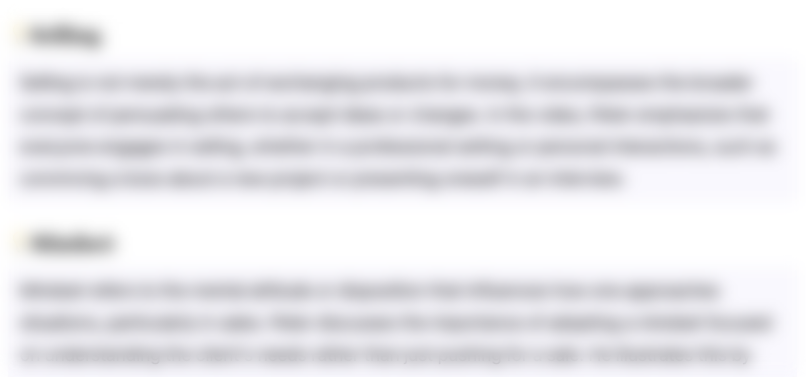
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
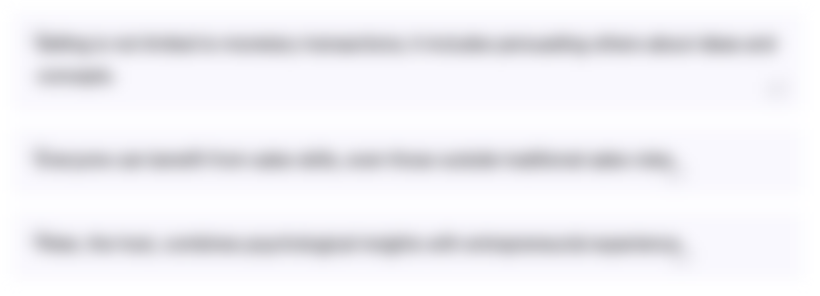
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
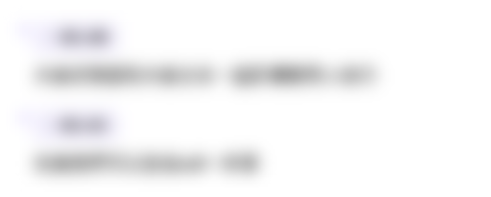
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
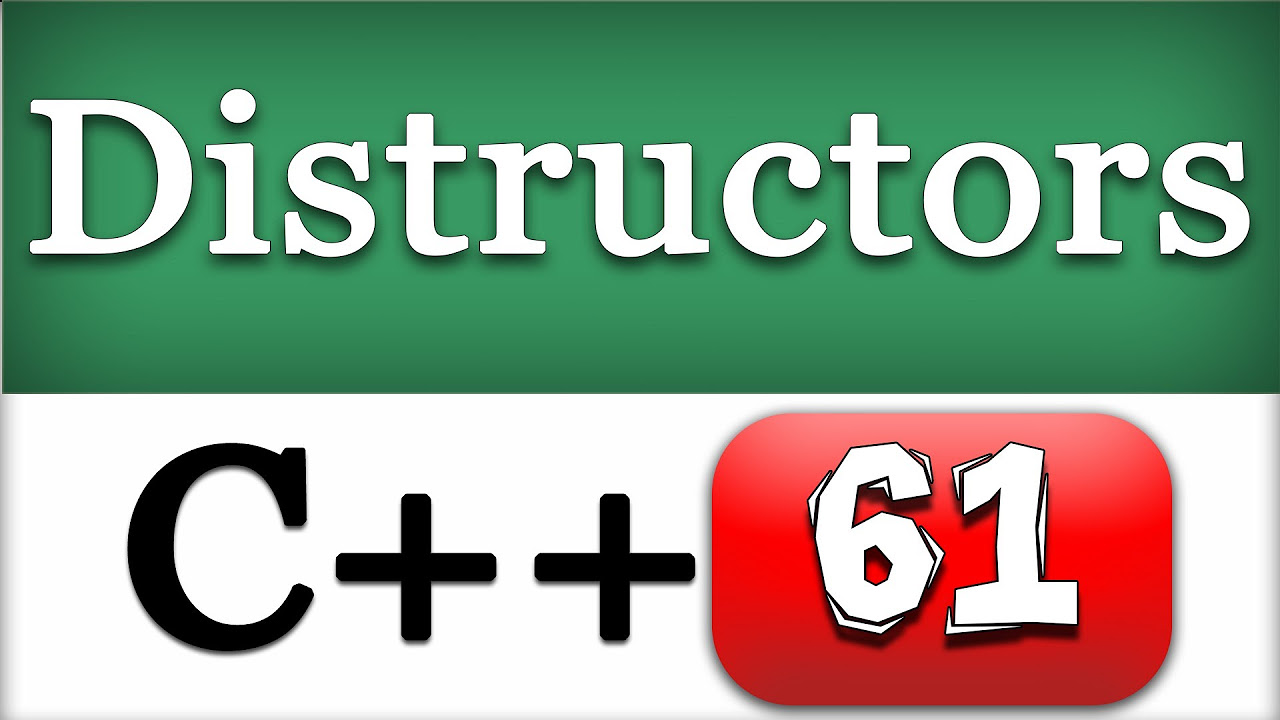
Destructors in a Class | C++ Object Oriented Tutorial

COS 333: Chapter 11, Part 1
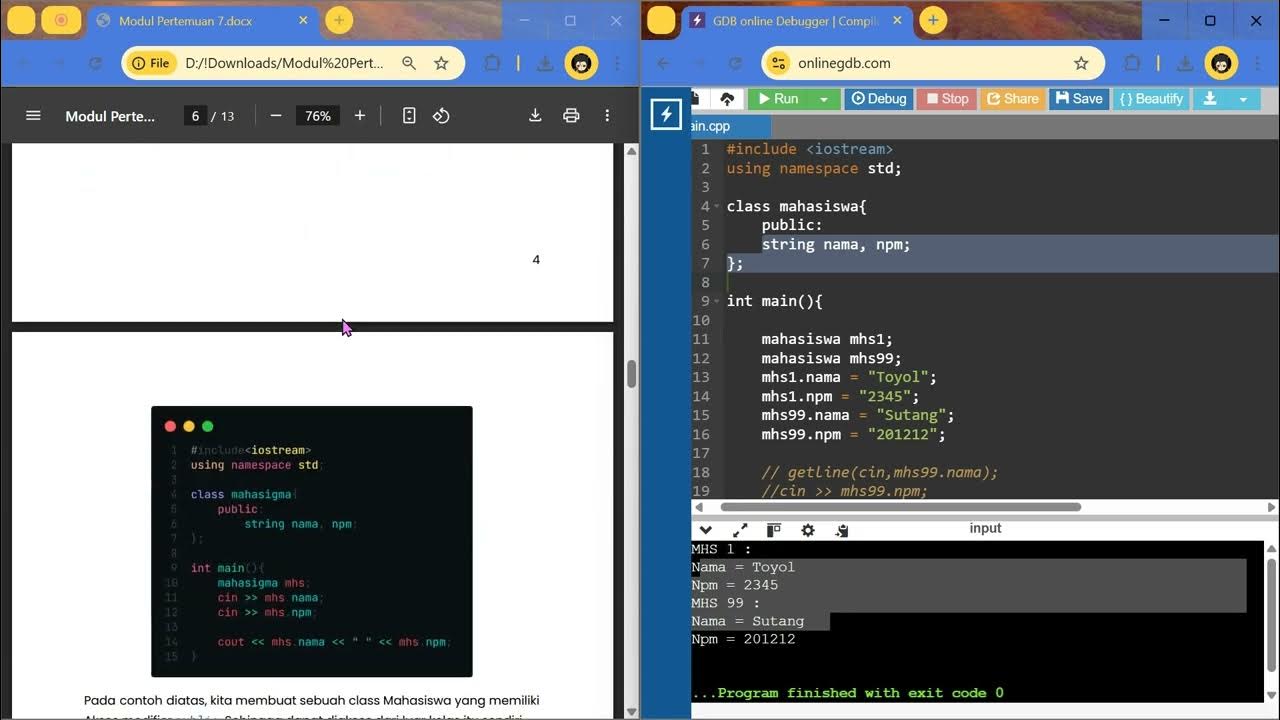
Pertemuan 7 Pemrograman Terstruktur 2025

JAVA TECHNICAL QUESTION AND ANSWERS FOR INTERVIEW PART III (Classes & Objects)

Destructors in Programming: Practical Demonstration
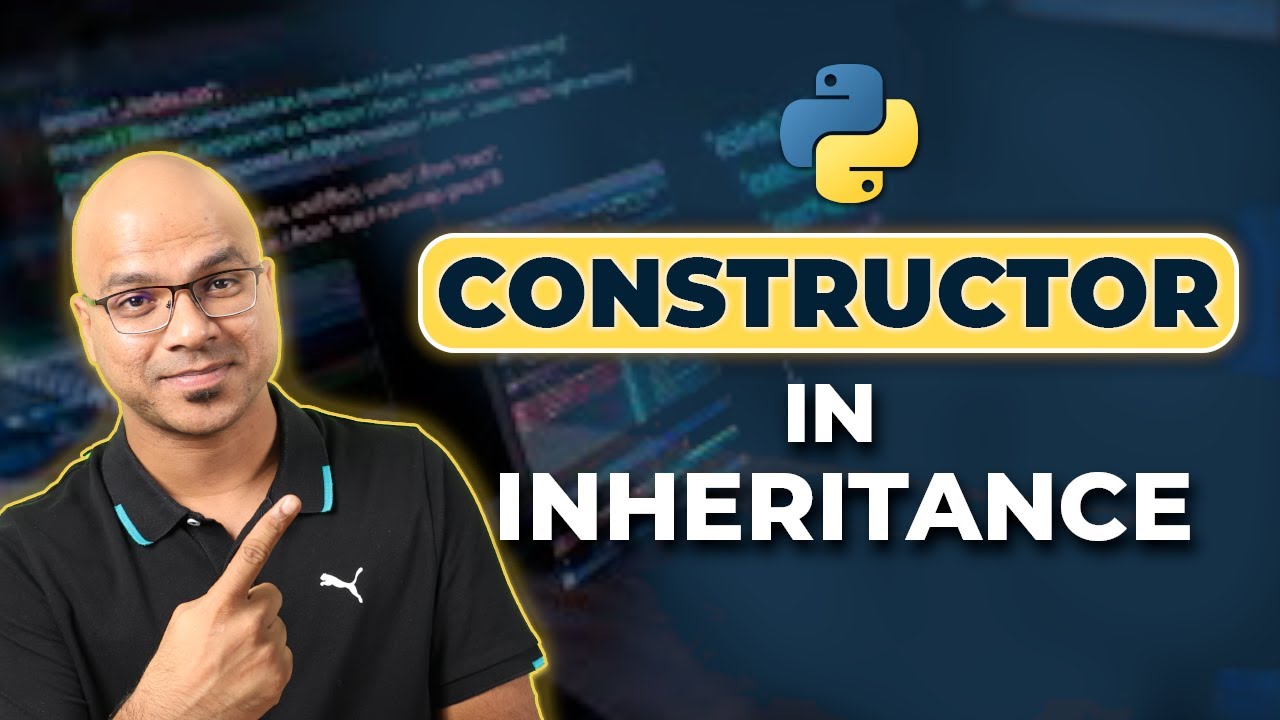
#56 Python Tutorial for Beginners | Constructor in Inheritance
5.0 / 5 (0 votes)