Node Auth Tutorial (JWT) #5 - Mongoose Validation
Summary
TLDRThis video script discusses enhancing user experience by creating custom error messages for a web application. It covers using Mongoose validation errors and custom error handling to provide users with specific feedback on form submission errors, such as invalid emails or passwords that don't meet criteria. The tutorial also introduces the use of a third-party package 'validator' for email validation and demonstrates how to handle errors in a centralized manner to return a JSON object containing relevant error messages. Additionally, it touches on the importance of hashing passwords using Mongoose hooks to ensure security.
Takeaways
- π The script discusses the importance of handling errors in web development to provide clear feedback to users when they submit incorrect data through a form.
- π Custom error messages are created for different types of validation errors to improve user experience by informing them what needs to be corrected.
- π€ Mongoose, a MongoDB object modeling tool, is used to define validation rules and custom error messages for user input fields such as email and password.
- π A 'validate' property is added to the email field to ensure that the input is a valid email address using a third-party validation package called 'validator'.
- π The 'isEmail' function from the 'validator' package is used to validate the email address within the custom validation function in the Mongoose schema.
- π The script mentions the use of Mongoose hooks to hash passwords before storing them in the database, emphasizing the security best practice of not storing plain text passwords.
- π The process involves creating a function to handle and evaluate errors, which checks the error object to determine the type of validation error and constructs a useful response for the user.
- π The 'handleErrors' function logs the error message and error code, which helps in identifying the type of validation error encountered during user input submission.
- π The script covers how to access and utilize the 'errors' object within the error message to extract specific validation error messages and paths for further processing.
- π The 'object.values' method is used to iterate over the errors and extract the necessary information to update an 'errors' object that will be sent back to the user.
- π Lastly, the script discusses handling the 'duplicate key error', which occurs when a user tries to register with an email that already exists in the database, by providing a custom error message.
Q & A
What is the main issue with the current post request error handling in the script?
-The main issue is that the error handling provides a generic error message regardless of the specific error, which doesn't offer additional information to the user on what needs to be corrected.
Why is it important to create custom error messages for different types of errors in a web application?
-Custom error messages are important because they inform the user specifically what went wrong, allowing them to correct the issue more efficiently rather than guessing what the problem might be.
What is the purpose of using Mongoose validation in the script?
-Mongoose validation is used to enforce data integrity and to provide specific error messages when the data does not meet the defined conditions, such as required fields or minimum password length.
How does the script customize error messages for the 'required' field in Mongoose schema?
-The script customizes error messages by using an array where the first element is the validation condition (e.g., true for required) and the second element is the custom error message to be displayed if the condition fails.
What additional property is added to the email field in the Mongoose schema to ensure it contains a valid email address?
-The 'validate' property is added to the email field with a function that uses a third-party validation package to check if the input is a valid email address.
What package does the script use for validating email addresses, and how is it installed?
-The script uses the 'validator' package for email validation, which is installed using the command 'npm install validator'.
How does the script handle the error object caught during user creation in order to provide more useful feedback to the user?
-The script evaluates the error object to determine the type of error and then creates a JSON object with specific error messages for the email and password fields, which is then sent back to the user.
What is the significance of the 'handleErrors' function in the script?
-The 'handleErrors' function is significant as it processes the error object, extracts the relevant error messages, and returns a structured JSON object containing those messages, which can be used to inform the user of specific validation errors.
How does the script differentiate between different types of validation errors in the 'handleErrors' function?
-The script checks for the presence of 'user validation failed' in the error message and then iterates over the values of the 'errors' object within the error to extract and assign the appropriate error messages to the email and password properties.
What is the final step in the 'handleErrors' function after processing the errors?
-The final step is to return the 'errors' object containing the specific error messages for the email and password, which can then be sent back to the user as a JSON response.
How does the script handle the scenario where an email is not unique during user registration?
-The script checks for a specific error code ('11000') which indicates a duplicate key error. If this error code is present, it sets a custom error message indicating that the email is already registered.
Outlines
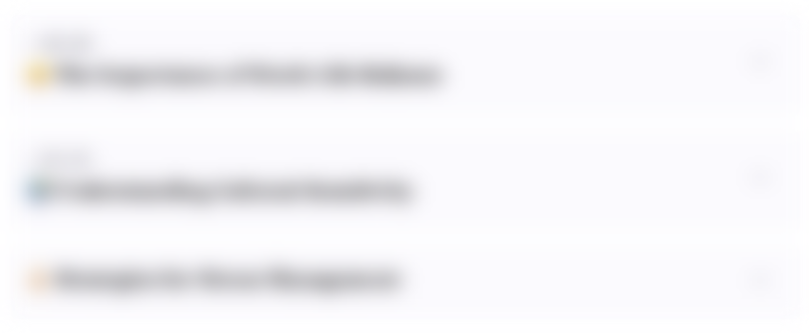
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
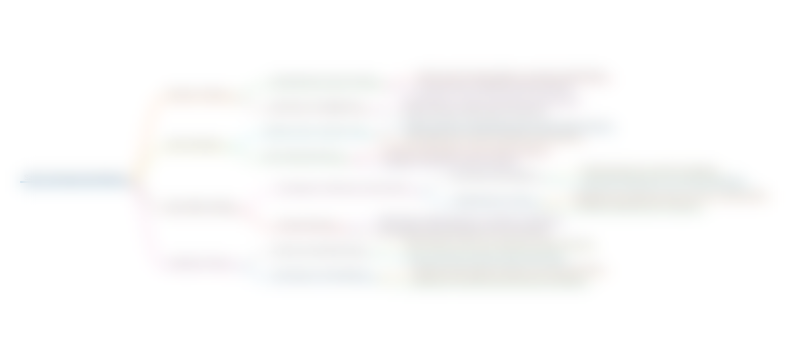
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
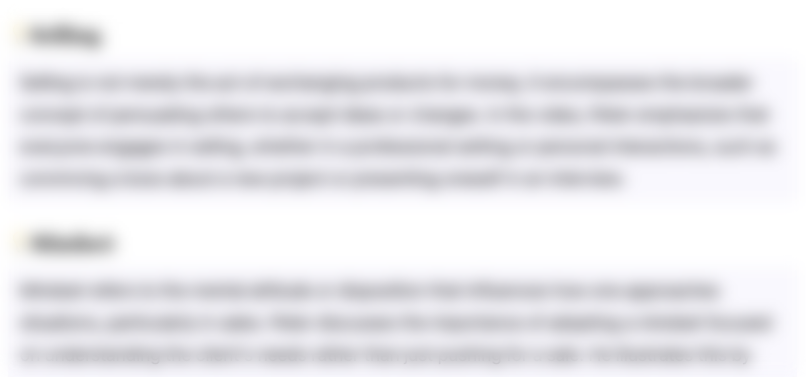
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
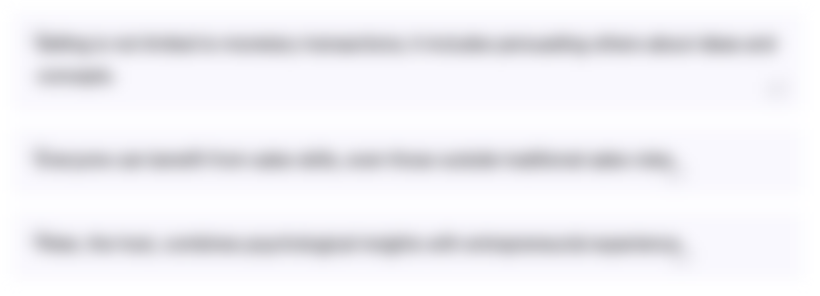
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
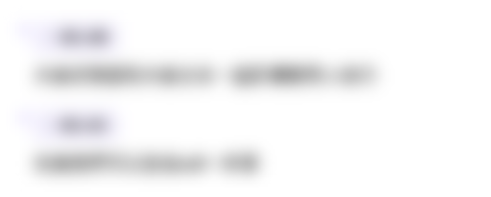
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)