#8 Type Conversion & Coercion in JavaScript
Summary
TLDRIn this JavaScript tutorial by Davin Rady, the concept of type conversion and type coercion is explored. The video explains how data types like numbers, strings, and booleans can be explicitly converted using language-specific functions. It also delves into implicit type coercion, where JavaScript automatically changes data types during operations, leading to potential bugs if not carefully managed. The tutorial covers practical examples, such as converting numbers to strings for storing zip codes and telephone numbers, and discusses the importance of being vigilant with these operations to avoid unexpected results.
Takeaways
- 😀 The video discusses type conversion and type coercion in JavaScript, explaining the difference between the two concepts.
- 🔢 Data types in JavaScript include numbers, strings, booleans, and the need to convert data between these types for various operations.
- 💡 Type conversion is explicit, where developers use specific functions like `String()` or `Number()` to change data types.
- 📝 Type coercion is implicit, where JavaScript automatically changes data types to perform operations, such as when adding a number and a string.
- 👀 The `typeof` operator is used to check the data type of a variable in JavaScript.
- 📌 Storing data like zip codes or phone numbers as strings is recommended when no calculations are needed.
- 🔄 The plus (`+`) operator can perform both addition and string concatenation, depending on the types of operands involved.
- 🤔 JavaScript's type coercion can sometimes lead to unexpected results and bugs if not handled carefully.
- 👍 JavaScript is appreciated for its flexibility in understanding the developer's intent and performing type coercion to meet that intent.
- 🚫 However, JavaScript's automatic type coercion can also be frustrating when it overrides the developer's intended operations.
- 🔍 The video suggests using functions like `parseInt()` to convert strings to integers, especially when the string contains non-numeric characters at the start.
Q & A
What is the main topic of the video?
-The main topic of the video is JavaScript type conversion and type coercion.
Why is type conversion necessary in programming?
-Type conversion is necessary when you want to change the data format, for example, converting a number to a string or vice versa, to meet specific requirements such as storing a zip code as a string.
What is the purpose of the 'typeof' operator in JavaScript?
-The 'typeof' operator is used to print the type of data of a variable in JavaScript.
How can you convert a number to a string in JavaScript?
-You can convert a number to a string in JavaScript by using the String function and passing the number as an argument, like String(num).
What happens when you try to perform an operation with a number and a string using the plus (+) operator?
-When using the plus (+) operator with a number and a string, JavaScript performs type coercion and treats the operation as string concatenation, converting the number to a string.
How does JavaScript handle type coercion with the subtraction operator?
-With the subtraction operator, JavaScript converts the string to a number if possible, allowing the operation to proceed as a numerical subtraction.
What is the result of converting a non-zero number to a boolean in JavaScript?
-In JavaScript, converting a non-zero number to a boolean results in true, as all non-zero numbers are considered truthy values.
What is the result of converting zero to a boolean in JavaScript?
-In JavaScript, converting zero to a boolean results in false, as zero is considered a falsy value.
How can you convert a string that represents a number into an actual number in JavaScript?
-You can use the parseInt function to convert a string that represents a number into an actual number in JavaScript.
What does the unary plus (+) operator do when used with a string in JavaScript?
-The unary plus (+) operator attempts to convert the string into a number in JavaScript.
What are some of the falsy values in JavaScript?
-In JavaScript, falsy values include 0, null, undefined, NaN, '', and false.
What is the difference between type conversion and type coercion in JavaScript?
-Type conversion is the explicit action of a developer to convert data from one type to another using functions like String(), Number(), or Boolean(). Type coercion is an implicit action performed by JavaScript where the engine changes the type of data during an operation to make it compatible, such as when adding a number and a string.
Outlines
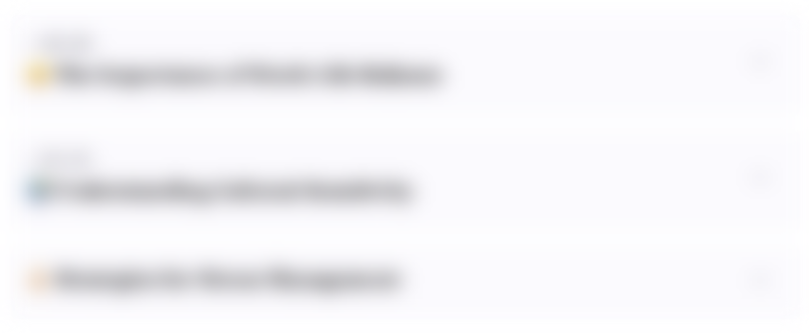
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
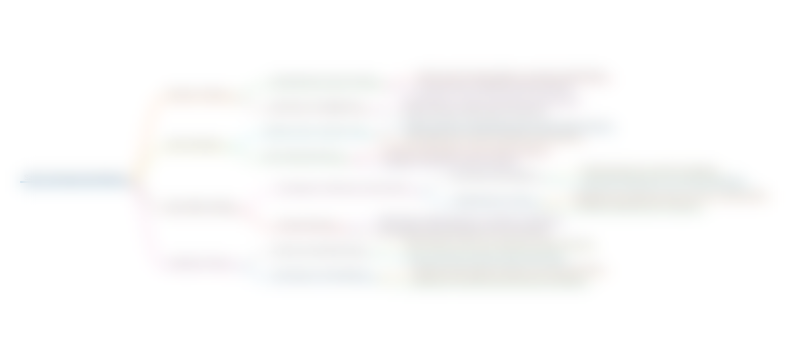
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
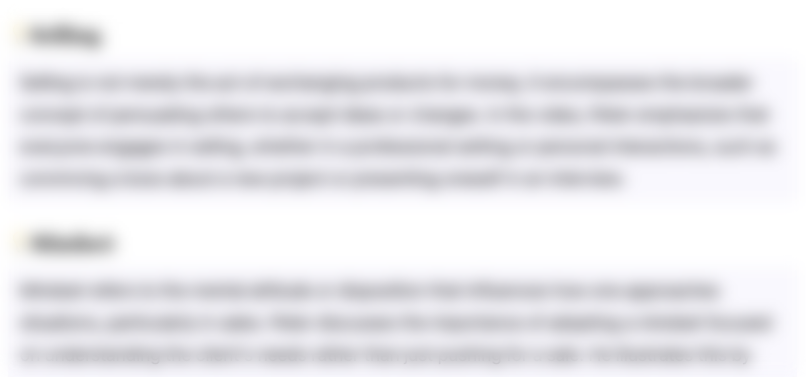
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
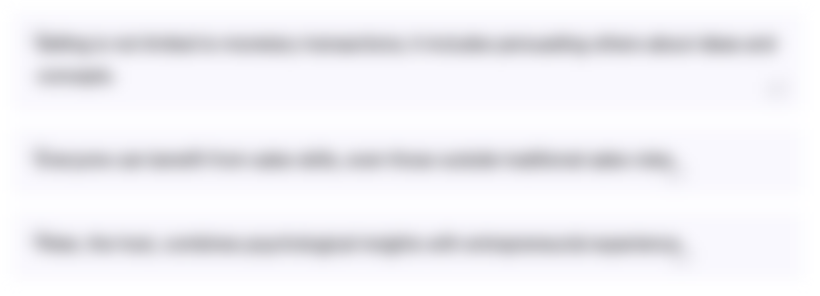
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
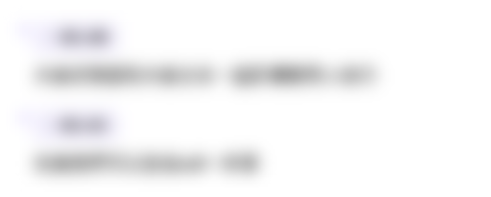
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)