#8 Type Conversion in Java
Summary
TLDRThis video script delves into the intricacies of data types, type conversion, and casting in programming. It explains that variables are immutable in their type but can be assigned values of different types, leading to implicit or explicit conversions. The script uses byte and integer types to illustrate how data can be lost during narrowing conversions and how widening conversions are allowed. It also introduces the concept of casting, where data types are explicitly converted, and type promotion, where operations on smaller data types are automatically promoted to larger types to prevent data loss. The tutorial is practical, guiding viewers through code examples and the impact of these concepts in programming.
Takeaways
- 😀 Variables in programming must have a name and a type, such as int, float, double, char, boolean, and string.
- 🔄 You cannot change the type of a variable once it's declared, but you can assign values of different types to it, depending on compatibility.
- ❌ Assigning a value from a larger data type (like int) to a smaller one (like byte) without explicit conversion will result in a compilation error.
- ✅ Implicit conversion (widening) is allowed when assigning a smaller data type value to a larger one, as the larger type can accommodate the smaller one.
- ⚙️ Explicit conversion (narrowing) is done through casting, where you manually convert a value from one type to another, potentially losing data if the target type has a smaller range.
- 🚫 Not all types can be implicitly or explicitly converted, such as assigning a character value to a boolean, due to their incompatible nature.
- 📉 When converting from float to int, the decimal part of the float is truncated, and only the integer part is retained.
- 🔀 Type promotion occurs automatically during operations when the result exceeds the range of the original data type, such as multiplying two byte values that exceed the byte range, and the result is promoted to an int.
- 💡 Understanding type conversion and casting is crucial for more advanced programming concepts, including object-oriented programming (OOPs).
- 💻 The video script provides a practical guide on how to handle different data types and perform conversions in Java, with examples and potential pitfalls.
Q & A
What are the two essential characteristics of a variable?
-Every variable needs a name and a type.
What is the range of values that a byte variable can hold?
-A byte variable can hold values from -128 to 127.
Can you change the type of a variable in Java?
-No, you cannot change the type of a variable in Java, but you can assign values of different types to variables through type conversion or casting.
What is the difference between type conversion and type casting?
-Type conversion is an automatic process where the type of a value is changed implicitly, while type casting is an explicit process where you manually convert a value from one type to another using a cast.
What happens when you assign a value that is outside the range of a byte variable?
-When assigning a value outside the range of a byte variable, Java uses the modulus operator to find the remainder of the division of the value by 256, effectively wrapping around the byte's range.
Why can't you assign an integer value directly to a byte variable if it's outside the byte's range?
-Assigning an integer value directly to a byte variable that is outside the byte's range will result in a loss of data and is not allowed because it can cause overflow or underflow, leading to incorrect values.
How do you convert an integer value to a byte in Java?
-You can convert an integer value to a byte in Java by explicitly casting the integer value to a byte using the syntax `(byte)integerValue`.
What is the result of converting a floating-point number to an integer in Java?
-When converting a floating-point number to an integer in Java, the decimal part of the number is truncated, and only the integer part is retained.
What is type promotion?
-Type promotion is the automatic conversion of a smaller data type to a larger one during arithmetic operations to prevent data loss. For example, when multiplying two byte values that result in a number exceeding the byte range, Java promotes the operation to use integer arithmetic.
How can you perform arithmetic operations involving different data types without causing errors?
-To perform arithmetic operations involving different data types without causing errors, you can use type casting to convert the smaller data type to a larger one before the operation, ensuring that the result fits within the range of the larger type.
Outlines
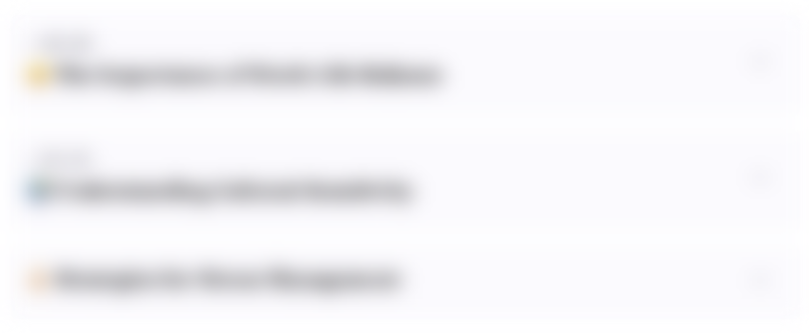
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
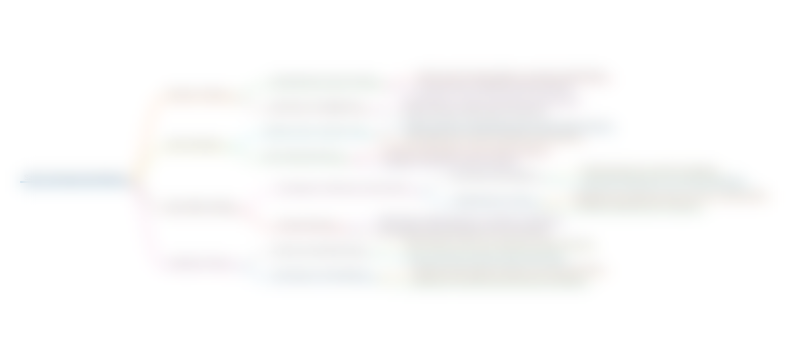
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
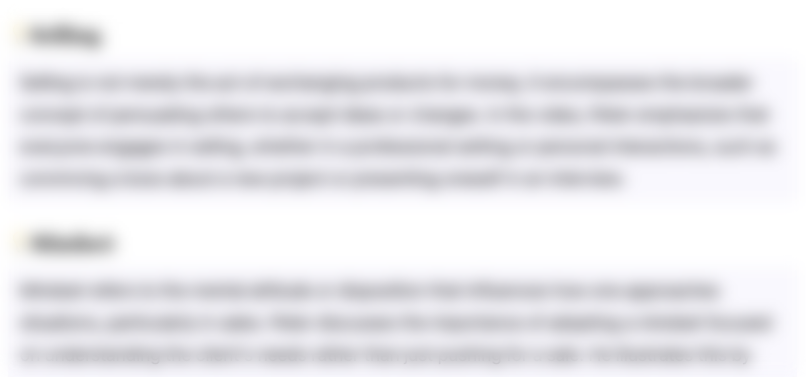
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
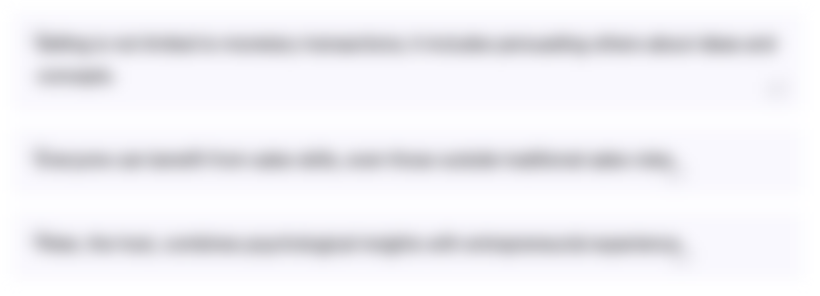
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
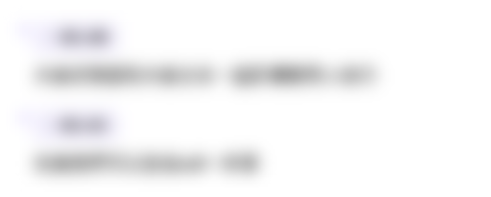
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Variable Basics (Updated) | Godot GDScript Tutorial | Ep 1.1

Lec-6: Typecasting in Python 🐍 with Execution | Python Programming 💻

C_80 Void Pointer in C | Detailed explanation with program
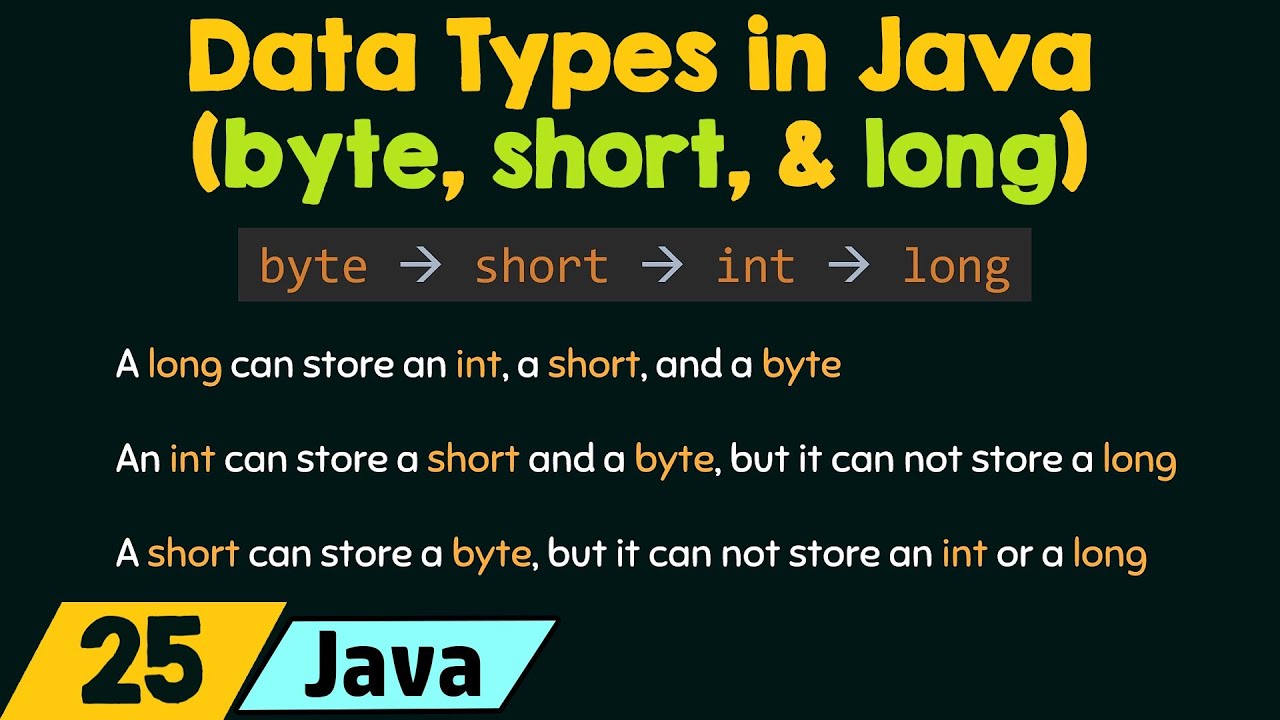
The byte, short, and long Data Types in Java
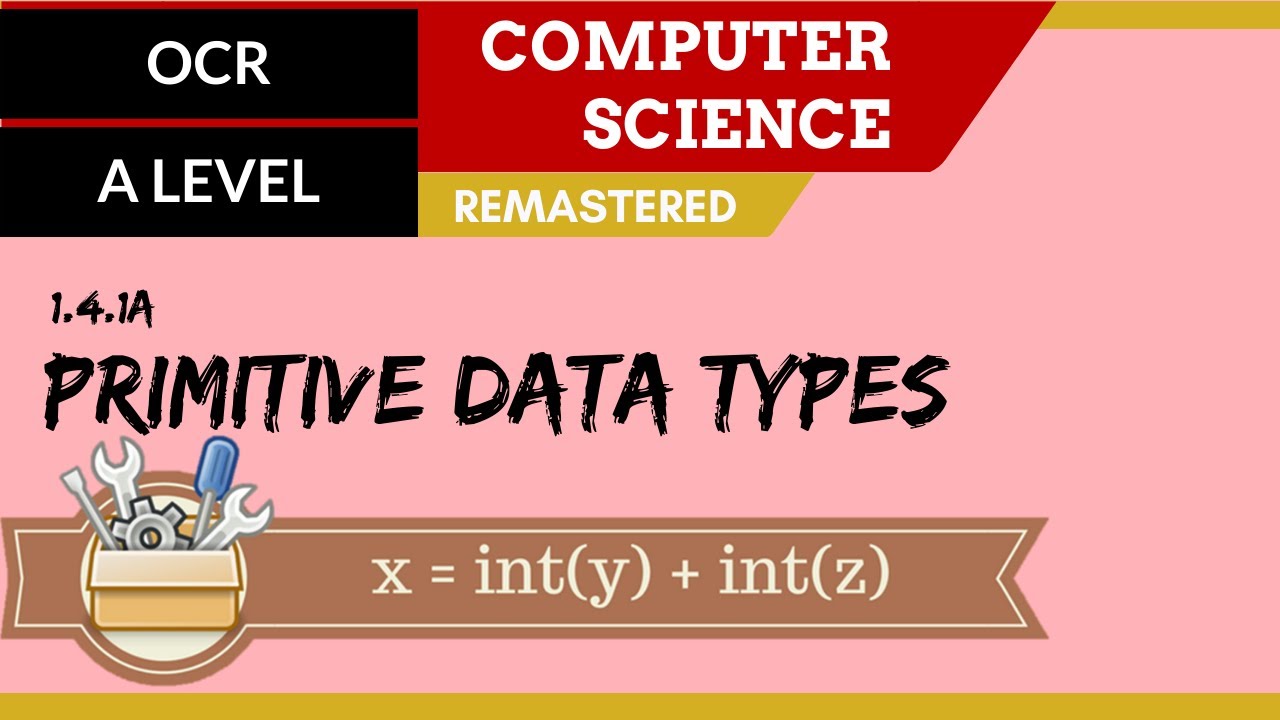
72. OCR A Level (H046-H446) SLR13 - 1.4 Primitive data types

02 - Expressions B - Python for Everybody Course
5.0 / 5 (0 votes)