Pointers and dynamic memory - stack vs heap
Summary
TLDRThis script delves into the architecture of memory, explaining the four segments of a program's memory: the instruction, global variable, stack, and heap segments. It clarifies the fixed nature of the first three and the dynamic, expandable nature of the heap, crucial for large data storage and flexible memory management. The tutorial covers the use of dynamic memory in C and C++ through functions like malloc, calloc, realloc, free, and operators new and delete, emphasizing the importance of manual memory management to prevent leaks and overflow.
Takeaways
- 🧠 Memory is a crucial resource in computing, and understanding its architecture is vital for programmers.
- 🏗️ A program's memory is typically divided into four segments: instruction, global variables, function calls and local variables (stack), and heap.
- 📚 The text segment holds the program's instructions, while the global segment stores static or global variables accessible throughout the application's lifetime.
- 📈 The stack segment manages function calls, local variables, and has a fixed size that does not grow during the application's runtime.
- 🔄 The heap segment is a flexible memory pool that can grow and shrink during the application's lifetime, used for dynamic memory allocation.
- 📝 In the provided C program example, the 'main' function and other functions like 'Square of Sum' and 'Square' demonstrate how memory is allocated and managed during execution.
- 🔑 The call stack is a LIFO (Last In, First Out) structure where the most recent function call is at the top and execution pauses until it returns.
- 🚫 Stack overflow occurs when the call stack exceeds its reserved memory, leading to a program crash, often due to infinite recursion.
- 🛠️ Dynamic memory allocation using the heap allows for more flexibility in memory usage, but requires manual management to avoid memory leaks.
- 🔄 In C, memory on the heap is allocated with 'malloc' and deallocated with 'free', while in C++, 'new' and 'delete' operators are used for the same purpose.
- 🔒 It's important for programmers to be cautious with heap memory to prevent overuse and ensure proper deallocation when memory is no longer needed.
Q & A
What are the four segments of memory in a typical architecture?
-The four segments of memory in a typical architecture are the text segment for storing instructions, the global variable segment for static or global variables, the stack for function calls and local variables, and the heap for dynamic memory allocation.
What is the purpose of the stack in memory management?
-The stack is used to store information about function calls, including local variables and where to return after the function finishes executing. It is allocated during runtime and has a fixed size that does not grow during the application's execution.
Why is the heap different from the other memory segments?
-The heap is different because its size can vary during the lifetime of the application. Unlike the stack, the heap does not have a set rule for allocation or deallocation, and a programmer can control the memory usage from the heap as needed.
What is the relationship between the call stack and the stack segment of memory?
-The call stack is a concept that represents the order of function calls during program execution. The stack segment of memory is the physical area where the call stack is implemented, storing local variables and function call information.
What is the potential issue with using a large amount of memory on the stack?
-Using a large amount of memory on the stack can lead to stack overflow if the call stack grows beyond the reserved memory for the stack, causing the program to crash.
How does dynamic memory allocation with the heap differ from memory allocation on the stack?
-Dynamic memory allocation with the heap allows for flexible memory usage where the size can grow and shrink as needed, and the programmer has control over when to allocate and deallocate memory. Memory on the stack is automatically allocated and deallocated with function calls and has a fixed size.
What are the four functions used for dynamic memory allocation in C?
-The four functions used for dynamic memory allocation in C are malloc, calloc, realloc, and free.
What is the purpose of the malloc function in C?
-The malloc function is used to allocate a specified amount of memory on the heap and returns a pointer to the starting address of the allocated block.
What is the significance of the free function in C?
-The free function is used to deallocate memory that was previously allocated with malloc, preventing memory leaks and unnecessary consumption of memory resources.
What are the two operators used for dynamic memory allocation in C++?
-The two operators used for dynamic memory allocation in C++ are new and delete, which are type-safe and simplify memory allocation and deallocation compared to C's malloc and free.
Why is it important to free memory allocated on the heap?
-It is important to free memory allocated on the heap to avoid memory leaks and to ensure efficient use of memory resources. Unlike the stack, memory on the heap does not get automatically deallocated when the function that allocated it completes.
Outlines
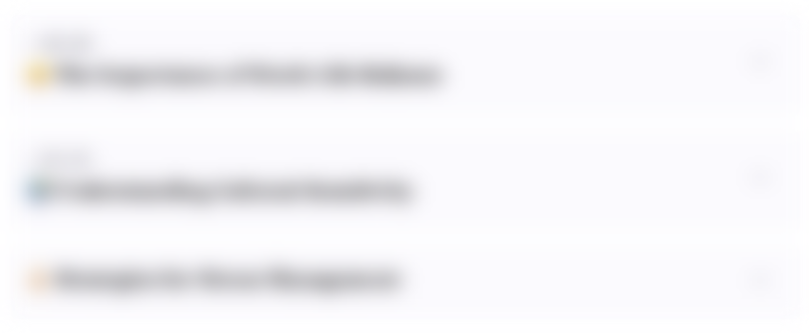
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
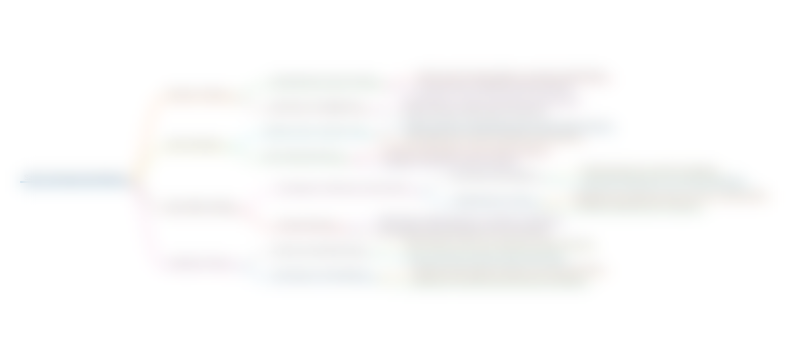
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
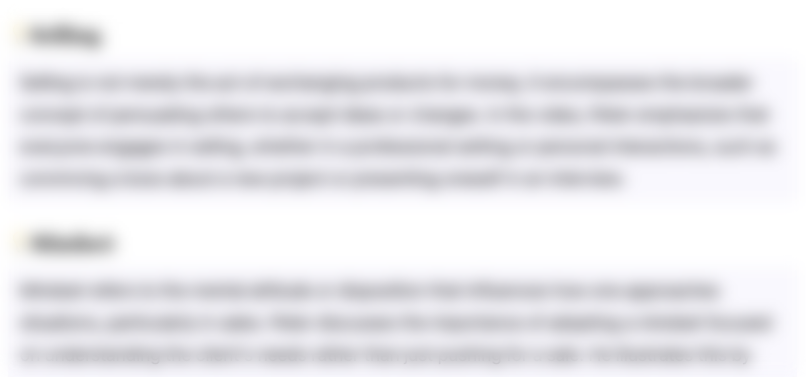
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
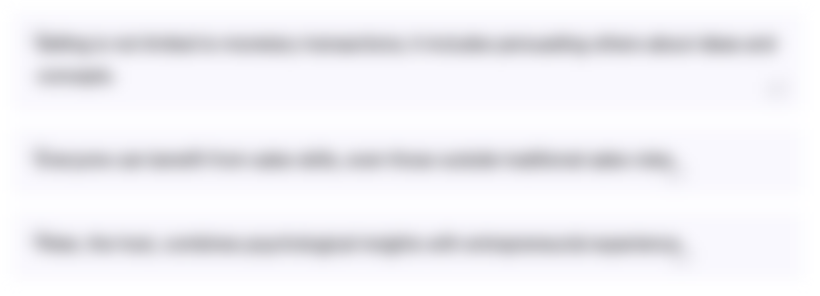
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
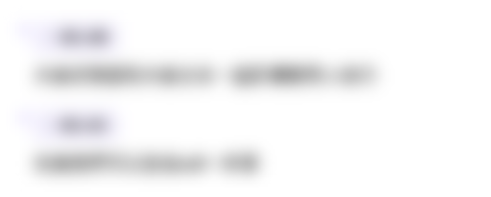
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)