Pointers and Dynamic Memory in C++ (Memory Management)
Summary
TLDRThis video tutorial explores the concept of pointers in C++, focusing on their use in dynamically allocating memory on the heap. It explains the importance of managing memory to prevent memory leaks and the creation of garbage. The video introduces the 'new' keyword for memory allocation and the 'delete' keyword for deallocation, highlighting the need to avoid dangling pointers. It also touches on the role of the standard template library in handling dynamic memory allocation efficiently.
Takeaways
- 💾 Dynamic Memory Allocation: The video discusses how to use pointers to refer to memory allocated during program execution, known as dynamically allocated memory or the heap.
- 🔍 Memory Layout: It explains the different areas of memory in a program, including code/text area, static/global area, and the stack for local variables and return addresses.
- 🔑 The Role of 'new': The keyword 'new' is introduced as a way to allocate memory on the heap and also to obtain the address of the allocated memory.
- 👉 Pointers and Memory Address: Pointers are used to hold the addresses of dynamically allocated memory, allowing access and manipulation of the allocated space.
- 🔄 Reassignment and Memory Leak: The video warns about the risk of creating garbage (unreachable memory) by not deallocating memory before reassigning a pointer to new heap memory.
- 🗑️ Deallocation with 'delete': The keyword 'delete' is used to free up memory that was previously allocated on the heap, preventing memory leaks.
- 🚫 Dangers of Dangling Pointers: After deallocating memory with 'delete', the pointer becomes a dangling pointer if not reassigned or set to null, which can lead to undefined behavior if used.
- 🔄 Reassignment Best Practices: It's advised to set pointers to null after deleting the memory they point to, to avoid dangling pointers and potential crashes.
- 📈 Managing Memory with STL: The video suggests that using the Standard Template Library (STL) can help manage dynamic memory allocation and deallocation, reducing the need for direct use of 'new' and 'delete'.
- 🚨 Common Sources of Garbage: The script highlights that forgetting to deallocate memory before reassignment and not deallocating memory within functions are common ways to create garbage.
Q & A
What is dynamically allocated memory?
-Dynamically allocated memory refers to memory that is allocated during the program execution, as opposed to static allocation which happens at compile time. This memory is also known as the heap or free store.
Why is it important to manage the heap in C++?
-In C++, it is the programmer's responsibility to manage the heap because the language does not automatically handle the allocation and deallocation of memory from this area. Proper management prevents memory leaks and ensures efficient use of resources.
How does the 'new' keyword work in C++?
-The 'new' keyword in C++ is used to allocate memory dynamically at runtime. It returns the address of the allocated memory, which can be stored in a pointer to reference the allocated space.
What is the significance of the address returned by the 'new' operator?
-The address returned by the 'new' operator is significant because it allows programmers to reference the dynamically allocated memory using a pointer. This address is where the allocated memory resides in the heap.
What is the purpose of the 'delete' keyword in C++?
-The 'delete' keyword is used to deallocate memory that was previously allocated using the 'new' keyword. It frees up the memory on the heap, making it available for other allocations and preventing memory leaks.
Why is it necessary to avoid creating garbage in memory?
-Creating garbage refers to having inaccessible memory on the heap that cannot be referenced or used. Accumulating garbage can lead to memory exhaustion, which might cause the program to crash or run out of heap space.
What is a dangling pointer and why should it be avoided?
-A dangling pointer is a pointer that points to memory that has been deallocated or is no longer valid. It should be avoided because attempting to access or manipulate memory through a dangling pointer can lead to undefined behavior and program crashes.
Why should a pointer be set to null after deleting the memory it points to?
-Setting a pointer to null after deleting the memory it points to is a good practice because it indicates that the pointer no longer references any valid memory. This can help prevent the pointer from being used inadvertently, thus avoiding potential errors.
How can the standard template library in C++ help manage memory?
-The standard template library (STL) in C++ provides container classes and algorithms that manage memory automatically. By using STL containers like vectors, maps, and lists, programmers can often avoid direct use of 'new' and 'delete', thus reducing the risk of memory management errors.
What is the potential consequence of not deallocating memory within a function in C++?
-If memory is allocated within a function using 'new' and not deallocated with 'delete' before the function ends, the memory will remain on the heap as garbage after the function's execution. This can lead to memory leaks and eventually cause the program to run out of memory.
Outlines
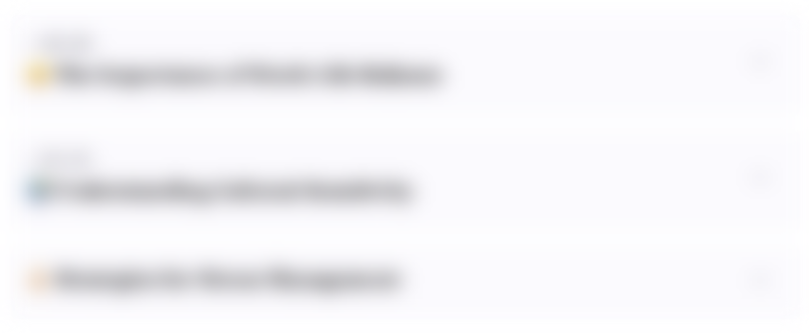
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
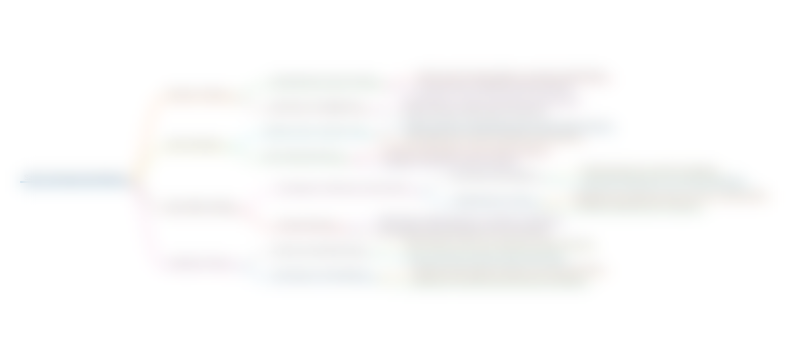
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
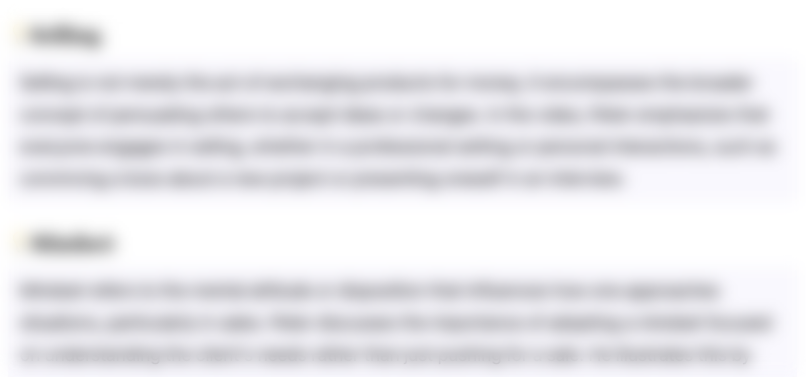
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
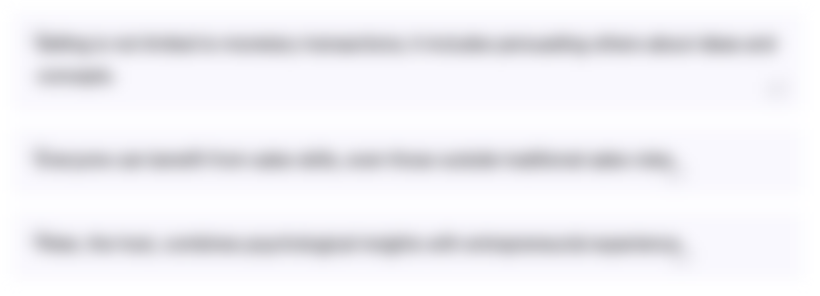
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
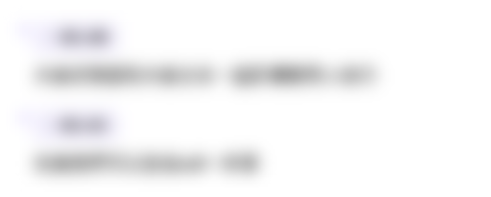
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
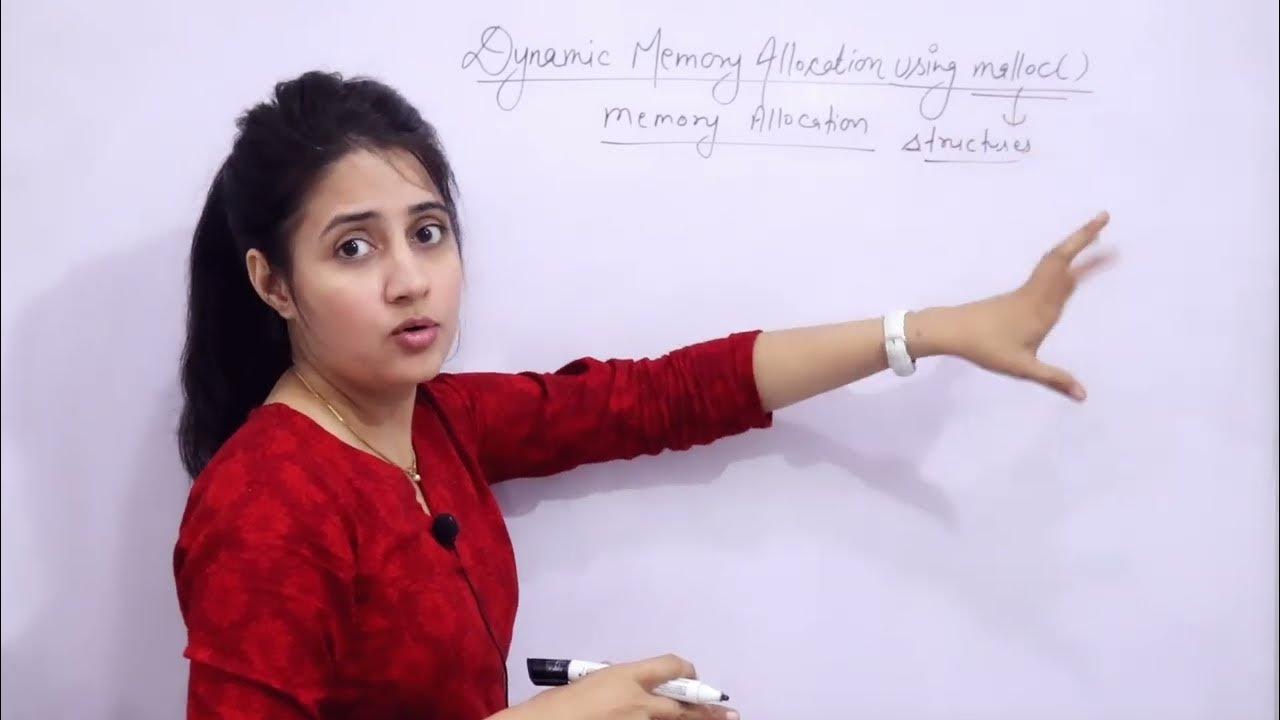
C_133 Dynamic Memory Allocation using malloc() | C Language Tutorials
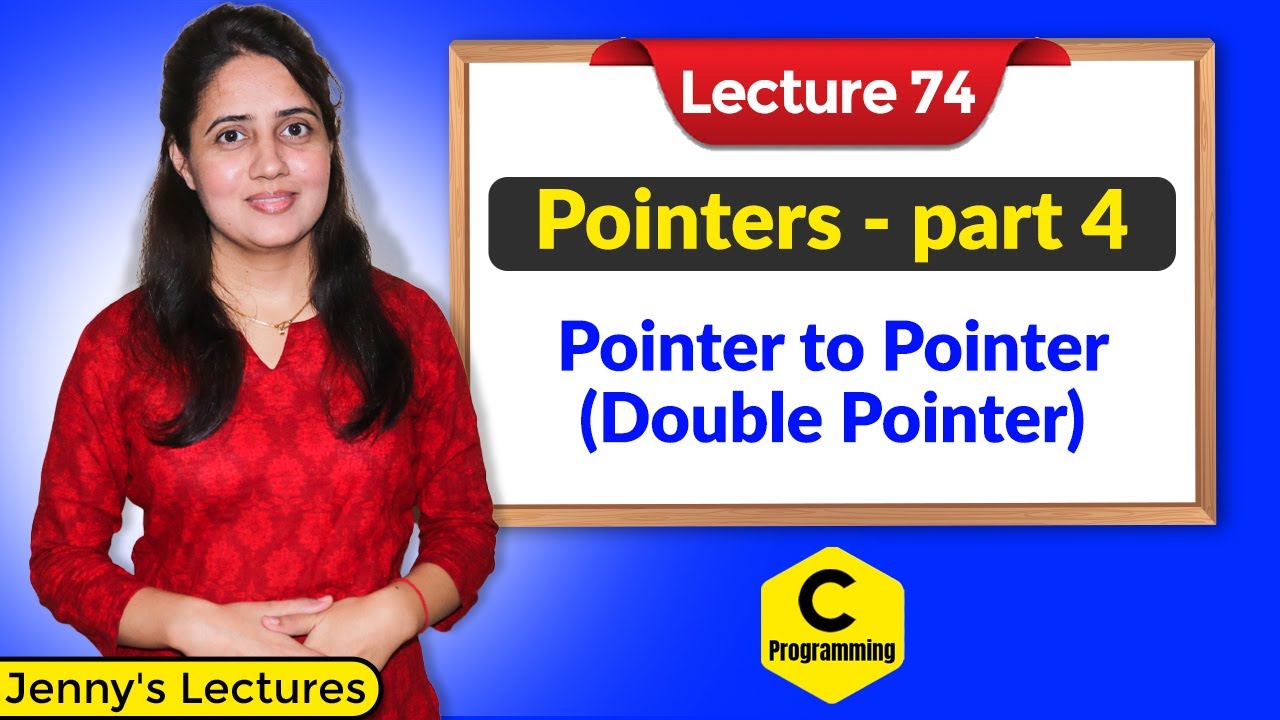
C_74 Pointers in C- part 4 | Pointer to Pointer (Double Pointer)
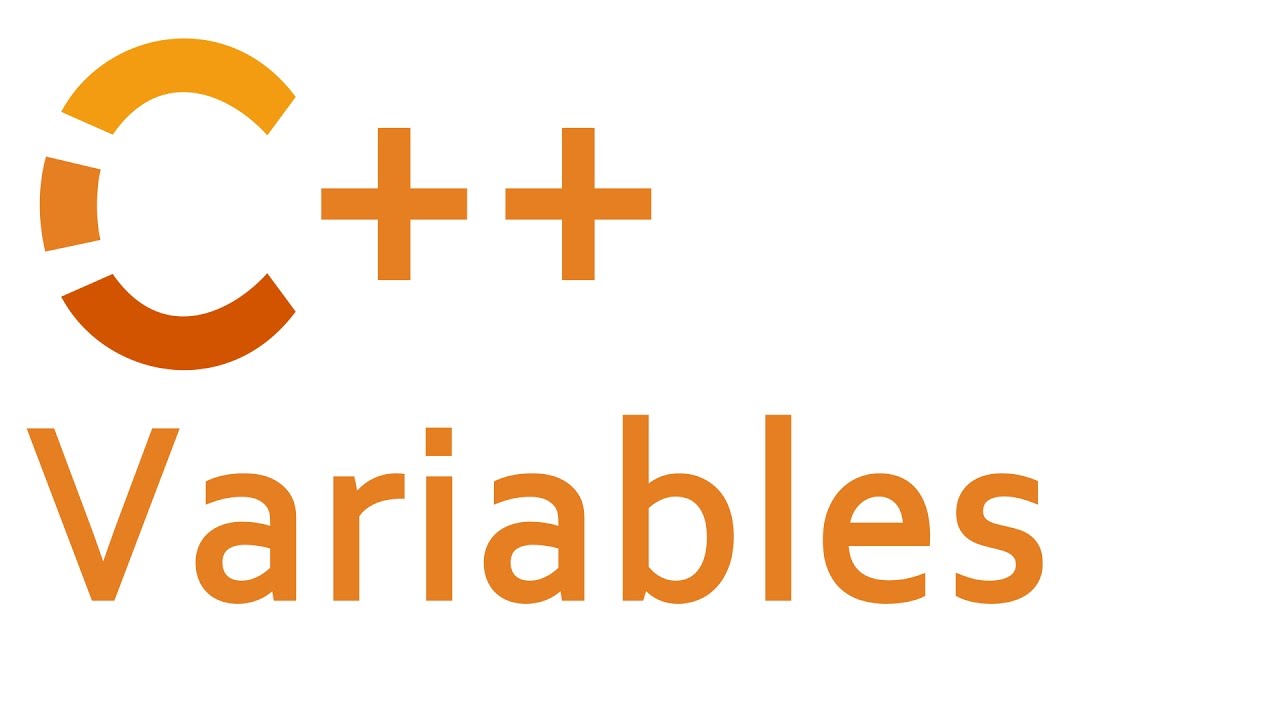
Variables in C++
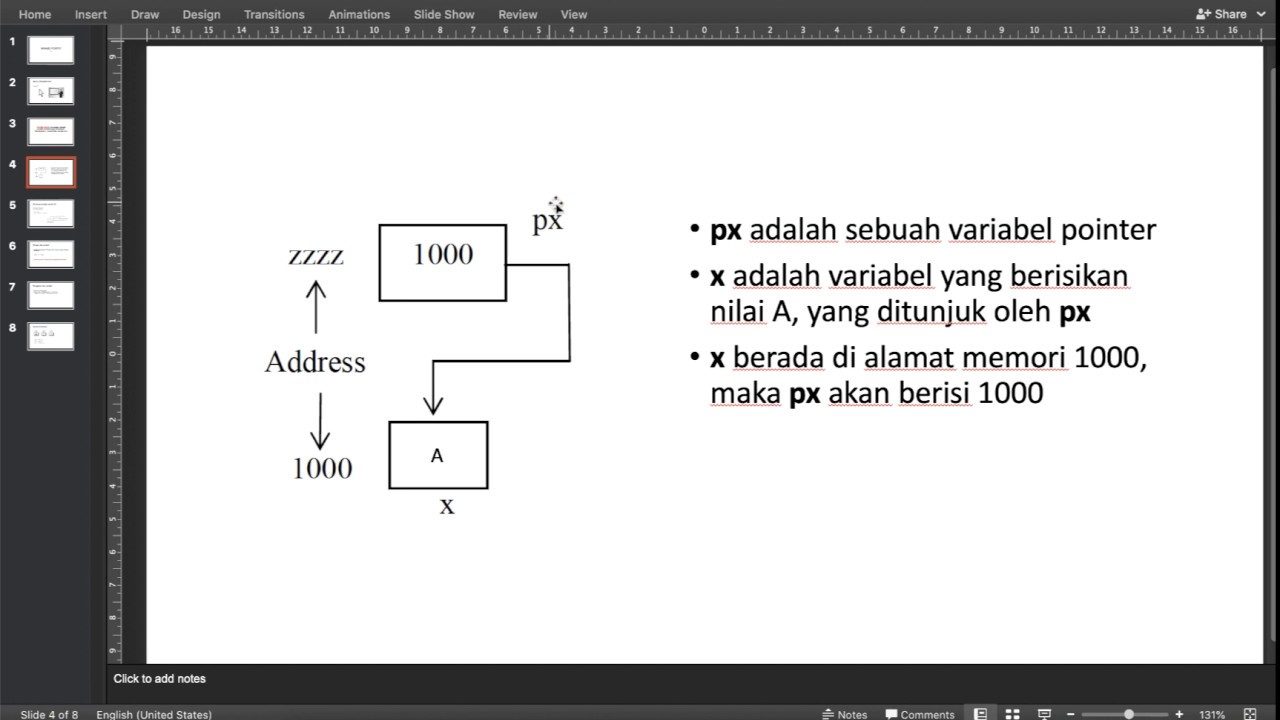
Variabel Pointer
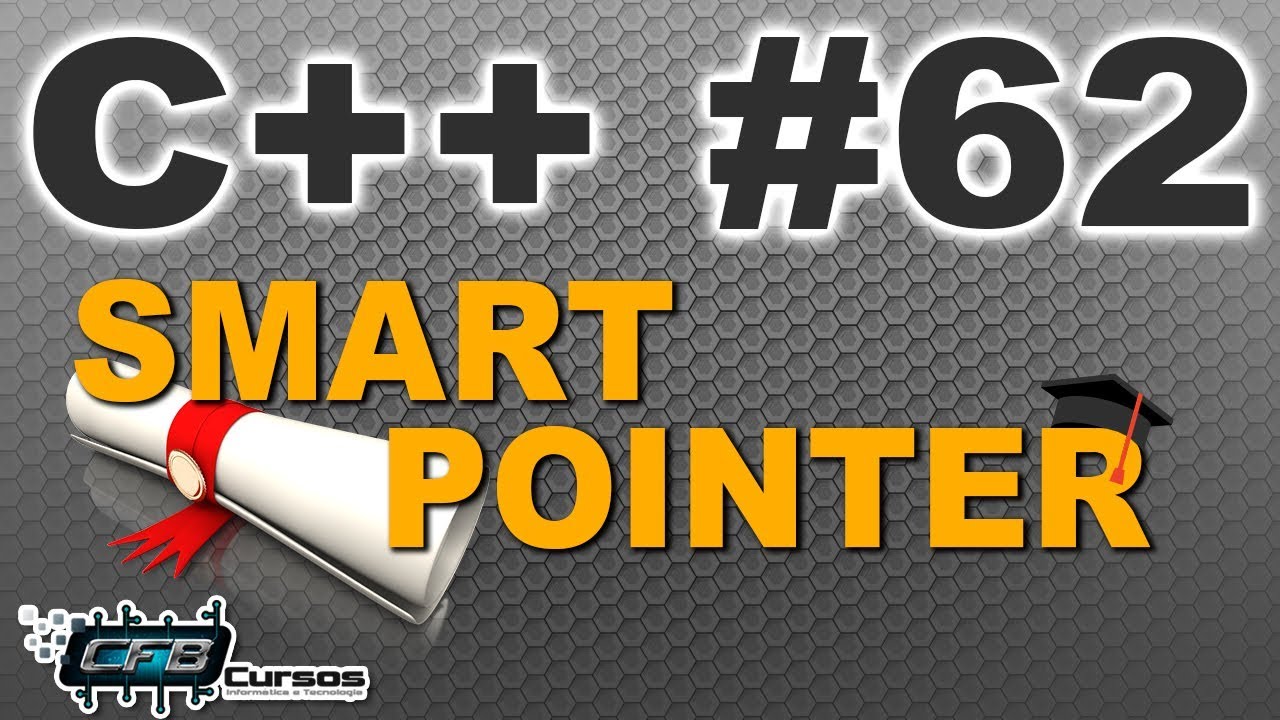
Curso de C++ #62 - Map - Smart Pointer / Ponteiro Inteligente - C++11 - (C++ Moderno)
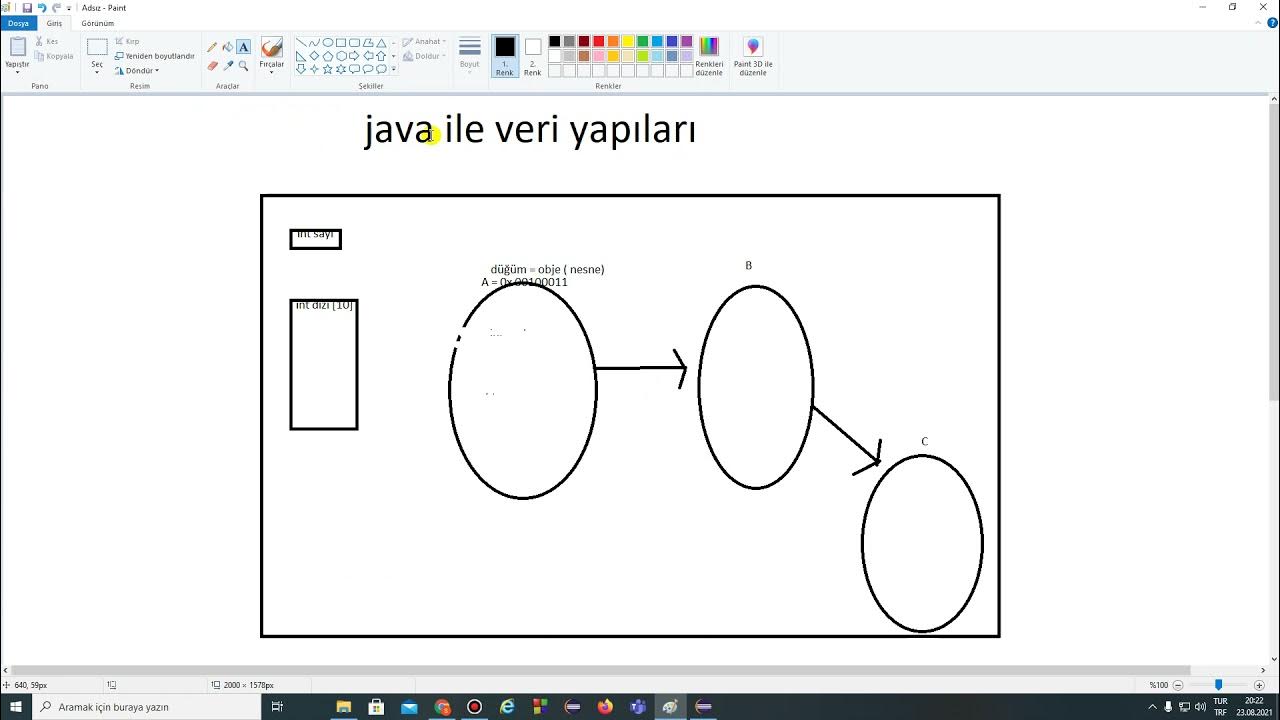
2- düğüm oluşturma , değer atama, düğümleri birbirine bağlama
5.0 / 5 (0 votes)