Dynamic Memory Allocation In C | C Memory Management Explained | C For Beginners | Simplilearn
Summary
TLDRThis educational video by Simply Learn introduces memory management in C programming. It explains the difference between static and dynamic memory allocation, emphasizing the flexibility of the latter for runtime memory needs. The video covers functions like malloc, calloc, realloc, and free from the stdlib.h library, illustrating how they allocate, initialize, resize, and deallocate memory blocks. Practical examples and syntax are provided, along with a simple program demonstration, ensuring viewers grasp the concepts for efficient memory usage in C.
Takeaways
- ๐ Memory management in C involves strategies for allocating and deallocating memory during the program's execution.
- ๐ There are two primary methods for memory allocation in C: static memory allocation and dynamic memory allocation.
- ๐ง Static memory allocation assigns memory to variables at compile time with a fixed size that cannot be changed.
- ๐พ Dynamic memory allocation occurs at runtime, allowing for more flexibility as memory can be allocated and deallocated as needed.
- ๐ The C standard library provides functions like `malloc`, `calloc`, `realloc`, and `free` for dynamic memory management.
- ๐ The `malloc` function is used to allocate a single block of memory, and it returns a pointer to the beginning of the block.
- ๐ The `calloc` function allocates memory for an array, initializing all elements to zero, and requires two arguments: number of elements and size of each element.
- ๐ The `realloc` function is used to resize a previously allocated block of memory without losing the original data.
- ๐๏ธ The `free` function is essential for deallocating memory that is no longer needed, making it available for other programs to use.
- ๐ป The video includes a practical demonstration of using these functions, highlighting the importance of checking for memory allocation success and proper memory deallocation.
Q & A
What is memory management in C programming?
-Memory management in C programming refers to the process of allocating and deallocating memory during runtime to manage the memory more efficiently. It involves allocating memory dynamically to variables whose size is not known until runtime.
What are the two ways to allocate memory in C?
-The two ways to allocate memory in C are static memory allocation and dynamic memory allocation. Static allocation happens during compile time, while dynamic allocation occurs during runtime.
How does static memory allocation differ from dynamic memory allocation?
-In static memory allocation, the memory is allocated during compile time and the size is fixed, whereas in dynamic memory allocation, memory is allocated during runtime and its size can change as needed.
What functions are provided by C for dynamic memory allocation?
-C provides the functions malloc(), calloc(), realloc(), and free() for dynamic memory allocation. These functions are defined in the stdlib.h header file.
What is the purpose of the malloc() function in C?
-The malloc() function is used to allocate a block of memory of a specified size. It returns a pointer to the beginning of the block, which can be used to access the allocated memory.
How does the calloc() function differ from the malloc() function?
-The calloc() function is similar to malloc() but it allocates memory for an array of elements, initializing all of them to zero. It takes two arguments: the number of elements and the size of each element.
What is the role of the realloc() function in memory management?
-The realloc() function is used to resize a previously allocated block of memory. It can be used to either increase or decrease the size of the memory block without losing the existing data.
Why is the free() function necessary in C?
-The free() function is necessary to deallocate memory that was previously allocated by malloc(), calloc(), or realloc(). It helps in returning the memory back to the heap for reuse by other programs, thus preventing memory leaks.
What happens if malloc() fails to allocate memory?
-If malloc() fails to allocate memory, it returns a null pointer. It's important to check the return value to ensure that memory allocation was successful before using the allocated memory.
What is the significance of checking the return value of memory allocation functions?
-Checking the return value of memory allocation functions is significant because it allows the programmer to handle errors, such as memory allocation failure, gracefully. It prevents the program from using uninitialized memory, which can lead to crashes or undefined behavior.
Outlines
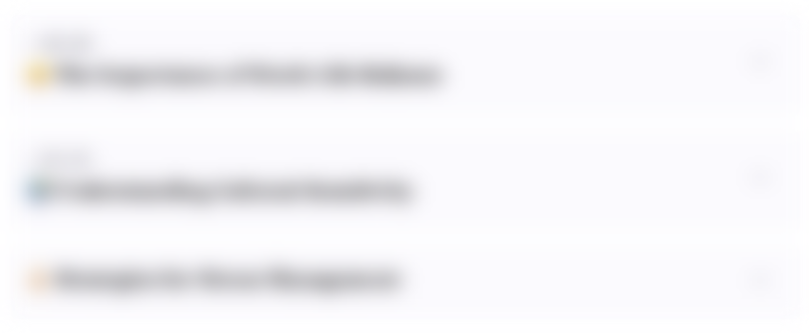
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
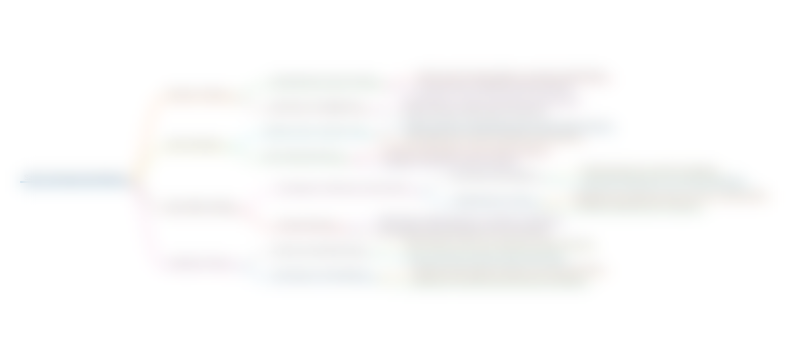
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
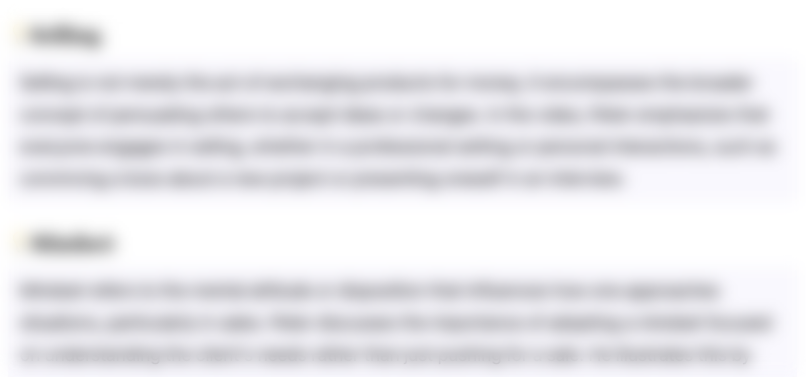
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
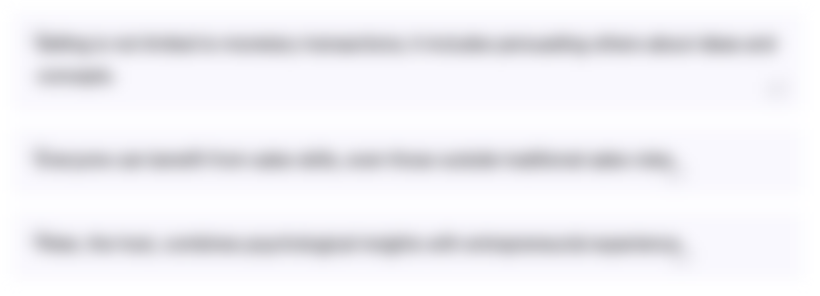
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
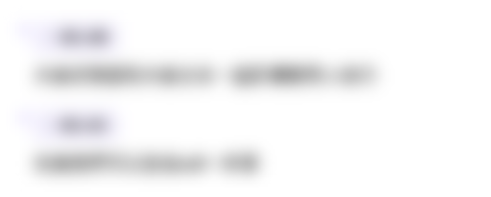
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)