Write Python Code Properly!
Summary
TLDRThis video tutorial focuses on the essential aspects of Python coding according to PEP 8, the Python style guide. It covers key conventions such as line length, indentation, naming conventions for variables, constants, modules, functions, classes, and exceptions. The presenter also discusses auto-formatting tools like Black, spacing rules, quotation mark usage, parameter naming, import statements, string operations, and best practices for comments and exception handling. The aim is to improve code readability and consistency, even though adherence to PEP 8 is not mandatory for code correctness.
Takeaways
- 📘 The video discusses the importance of adhering to PEP 8, the Python style guide, for writing consistent and readable Python code.
- 🛠 It's not incorrect to deviate from PEP 8, but doing so can lead to inconsistency, especially in large organizations.
- 🔗 A link to PEP 8 is provided in the video description, and it's authored by Guido van Rossum, Python's creator.
- ⚙️ The video recommends using an auto-formatter like 'black' to automatically adhere to PEP 8 guidelines, saving time and effort.
- 📝 PEP 8 suggests a maximum line length of 79 characters, with the 80th character being where the line should wrap.
- 🔑 For indentation, PEP 8 recommends using four spaces, avoiding tabs, to prevent potential errors and maintain consistency.
- 🔡 When naming variables, functions, and methods, snake_case should be used, while classes and exceptions should follow PascalCase.
- 🔠 Constants should be in all capitals with underscores separating words, and are typically placed at the top of a Python file.
- 📑 The video emphasizes the importance of consistent styling, such as the use of quotation marks, over strict adherence to every rule.
- 🚫 It advises against using wildcard imports (e.g., 'from module import *') as it does not clearly define what is being used from the module.
- ➡️ For string operations, using methods like `startswith` or `endswith` is preferred over slicing for checking prefixes or suffixes.
Q & A
What is the main focus of the YouTube video?
-The main focus of the YouTube video is to show the proper way to write Python code by following the Python conventions and the most important parts of the PEP 8 style guide.
Who wrote the PEP 8 style guide?
-PEP 8 was written by Guido van Rossum, the inventor and creator of Python.
Why is it important to follow PEP 8 guidelines in a large organization?
-Following PEP 8 guidelines in a large organization ensures code consistency, which can prevent some people from being upset about inconsistencies in how Python code is written.
What is one of the main rules in PEP 8 regarding line length?
-One of the main rules in PEP 8 is that any line in Python code cannot exceed 80 characters.
What tool does the video recommend for auto-formatting Python code in VS Code?
-The video recommends using 'black', a popular formatter for Python, for auto-formatting code in VS Code.
How should variables be named according to PEP 8?
-Variables should be named using snake_case, which means separating words with underscores and using all lowercase letters.
What is the naming convention for constants in Python?
-Constants should be named using all uppercase letters and snake_case.
How should functions be named according to PEP 8?
-Functions should be named using snake_case, similar to variables.
What is the correct way to name classes in Python?
-Classes should be named using PascalCase, which means capitalizing the first letter of each word without underscores.
What should be the first parameter name in instance methods and class methods?
-The first parameter in instance methods should be named 'self', and in class methods, it should be named 'cls'.
How should top-level functions and classes be spaced in a Python file?
-Top-level functions and classes should be separated by two blank lines.
What is the PEP 8 guideline for spacing around operators?
-PEP 8 recommends having spaces around operators, with the same number of spaces on each side of the operator.
How should default values for parameters in functions be formatted?
-Default values for parameters should have no spaces around the equal sign.
What is the recommended way to import modules in Python?
-Modules should be imported at the top of the file, each on a separate line, and using 'import *' is discouraged.
How should strings be enclosed in Python, according to PEP 8?
-Strings can be enclosed in single or double quotation marks, but consistency should be maintained throughout the code.
What is the preferred method for checking if a variable is 'None'?
-The preferred method is using 'is None' rather than '== None'.
What is the correct way to check if a string starts or ends with a specific substring?
-The correct way is to use the '.startswith()' and '.endswith()' methods instead of string slicing.
Outlines
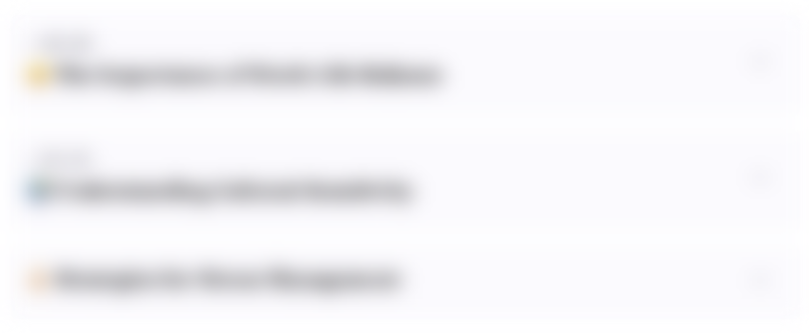
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
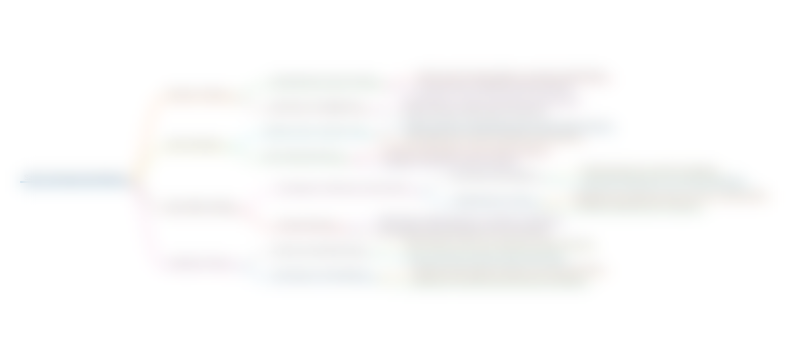
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
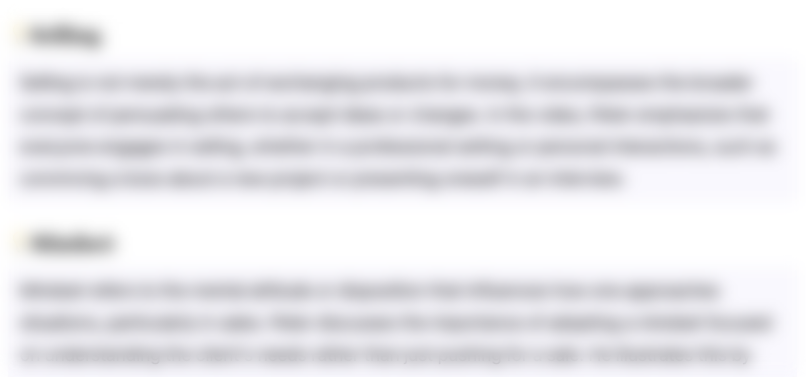
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
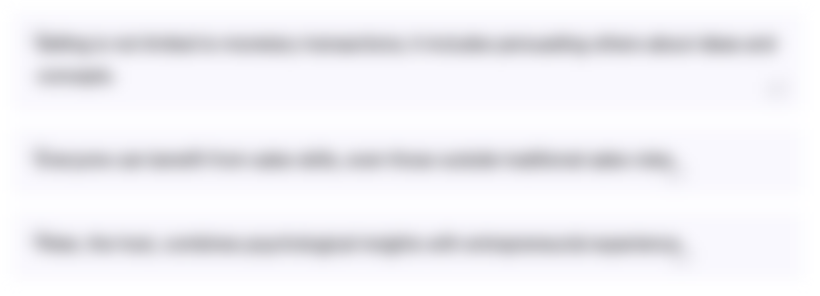
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
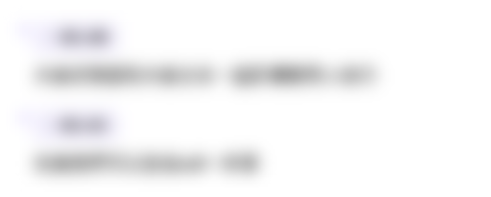
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
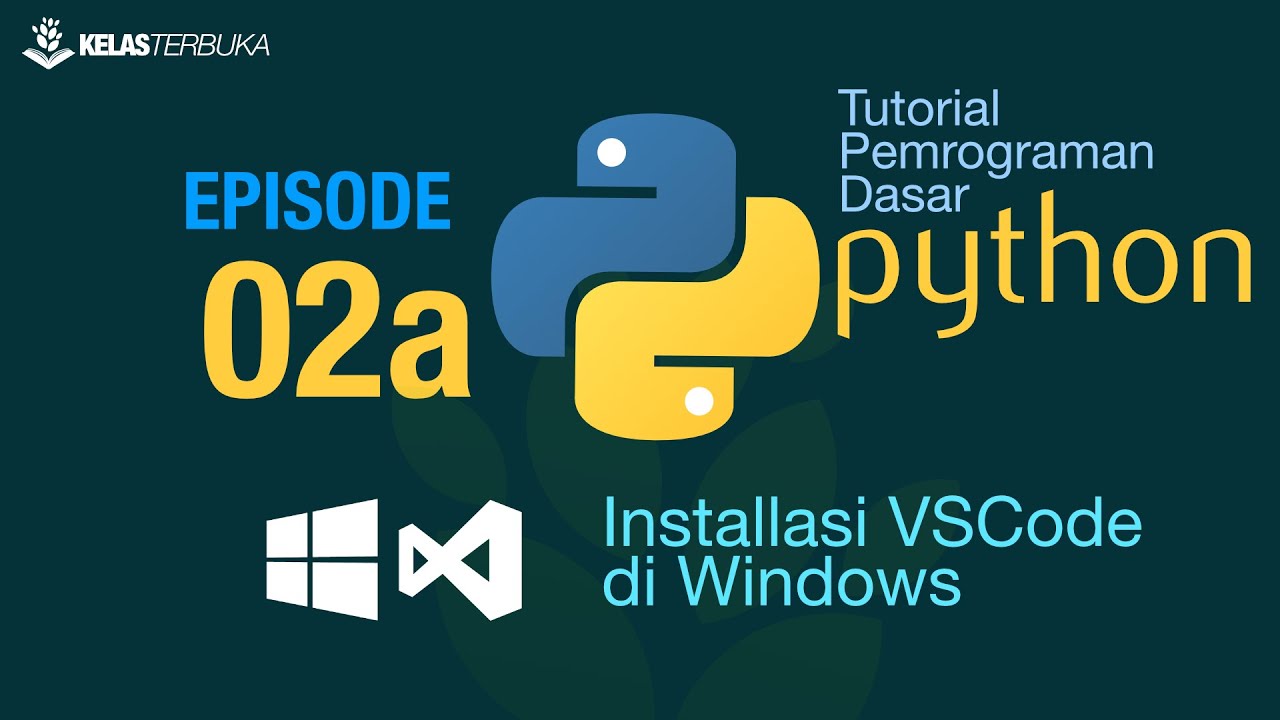
Belajar Python [Dasar] - 02a - Installasi Python dan VS Code di Windows
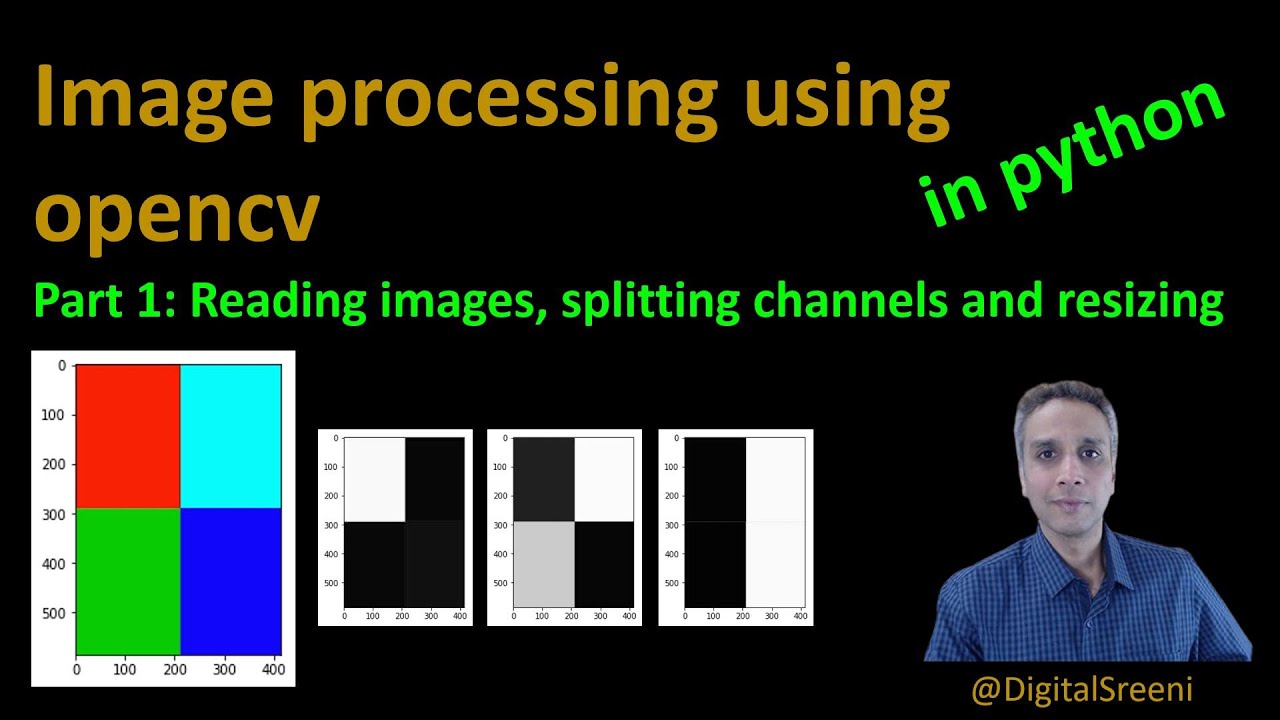
25 - Reading Images, Splitting Channels, Resizing using openCV in Python
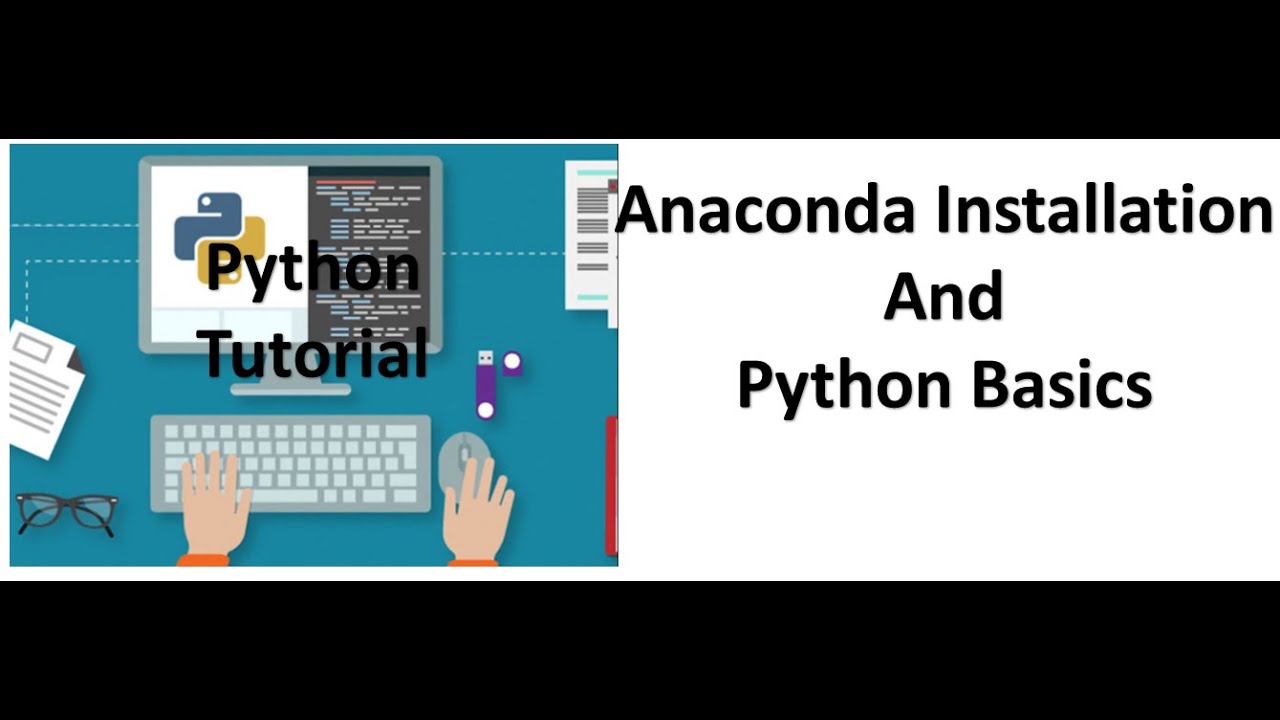
Tutorial 1- Anaconda Installation and Python Basics

Python Syntax - Everything you need to know!
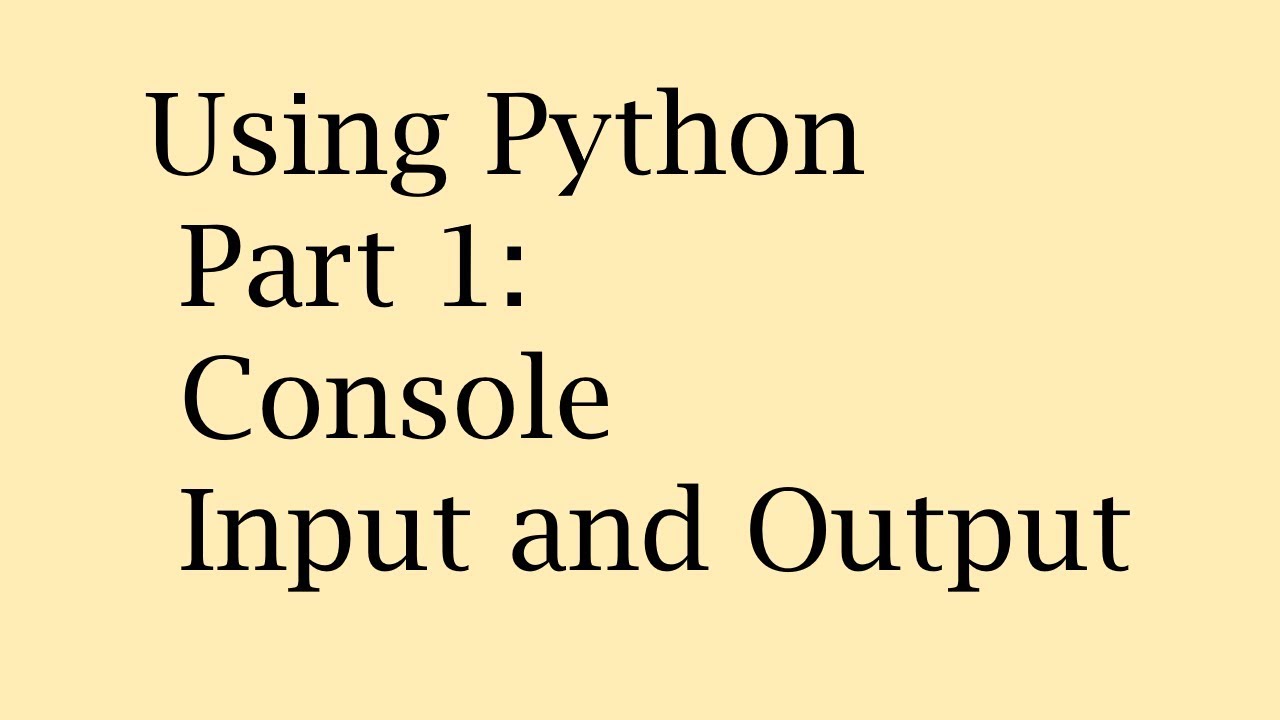
Using Python: Part 1: Console Input and Output

Belajar Python [Dasar] - 02b - Installasi Python dan VS Code di MacOS
5.0 / 5 (0 votes)