React Naming Conventions You should follow as a Junior Developer - clean-code
Summary
TLDRThis video script offers essential React naming conventions for developers, focusing on clean, readable, and maintainable code. It covers directory and file structure, naming for pages, components, hooks, variables, and more. The script emphasizes using PascalCase for pages and components, camelCase for files and variables, and underscores for constants. It also advises on descriptive function and hook names, and leveraging linters to enforce consistent naming conventions, ensuring a harmonious codebase for team collaboration.
Takeaways
- ๐ The script emphasizes the importance of a well-structured directory for React projects, with main folders like 'src', 'public', and specific files for configurations.
- ๐ Inside the 'src' folder, there should be organized sub-folders such as 'assets', 'pages', 'components', 'hooks', 'services', 'store', 'typings', and 'utils' for better code maintenance and readability.
- ๐ For 'pages', use PascalCase for folder and file names to highlight their importance as the main entry points of the application.
- ๐ฆ Components should be reusable and kept in their respective folders, with an 'index' file as the main entry point for each component.
- ๐ Hooks should be named descriptively and prefixed with 'use' to indicate their purpose and to follow React's naming convention.
- ๐ Functions and variables should have descriptive names to convey their functionality at a glance, using camelCase for file names and variables outside of the 'pages' folder.
- ๐ For props, use PascalCase and define them using interfaces for clarity and reusability across components.
- ๐ When naming interfaces or types, avoid starting with an 'I' prefix as it is no longer necessary with TypeScript, and use PascalCase instead.
- ๐ Constants or global variables should be in ALL_UPPERCASE with underscores to indicate their unchanging nature.
- ๐ฎโโ๏ธ Use linters with specific rules like 'camelcase' and 'typescript-eslint' to enforce naming conventions and maintain code quality.
- ๐ The script suggests that following these naming conventions contributes to cleaner, more readable, and maintainable React code.
Q & A
What are the essential React naming conventions discussed in the video?
-The video discusses several React naming conventions, including using PascalCase for pages and components, camelCase for file names and variables, and specific hooks naming with 'use' prefix. It also covers the structure of folders and files within a React project.
Why is the 'src' folder important in a React project?
-The 'src' folder is important as it contains all the source code for the application. It is the main directory where developers write and organize their code.
What is the purpose of the 'public' folder in a React project?
-The 'public' folder contains assets that are publicly available throughout the web server, such as images, fonts, and other static files that can be accessed by users or other websites.
What are the main folders typically found inside the 'src' directory of a React project?
-The main folders inside the 'src' directory include 'assets', 'pages', 'components', 'hooks', 'services', 'store', 'typings', and 'utils'.
Why should pages be named using PascalCase in a React project?
-Pages should be named using PascalCase because they are very important and serve as the main entry points for the application. PascalCase makes it clear and easy for developers to identify these key files.
What is the recommended naming convention for file names outside the 'pages' folder?
-For file names outside the 'pages' folder, camelCase is recommended as it is easier to read and write, and it is the conventional standard in JavaScript and React projects.
How should components be organized within a React project?
-Components should be organized in folders, with each folder representing a single component. Inside each component folder, there can be multiple files related to that component, including an 'index' file that serves as the main entry point.
What is the naming convention for functions in a React project?
-Functions should be named using camelCase and should be descriptive to indicate their purpose. For example, 'calculateTotalWithVat' clearly indicates that the function calculates a total including VAT.
Why is it important to use interfaces for props in a React component?
-Using interfaces for props is important because it enforces a contract for the data that components expect to receive, making the code more maintainable and less prone to errors.
What is the recommended naming convention for TypeScript interfaces?
-TypeScript interfaces should be named using PascalCase, without the traditional 'I' prefix, as it is no longer necessary and can make the interface name too specific.
How can a linter help enforce good naming conventions in a React project?
-A linter can enforce good naming conventions by setting rules that check for correct case usage, such as camelCase for variables and PascalCase for interfaces and components. It can prevent code from being committed if it does not adhere to these standards.
Outlines
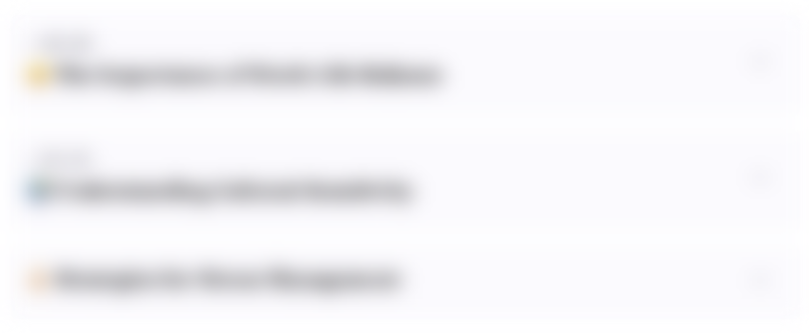
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
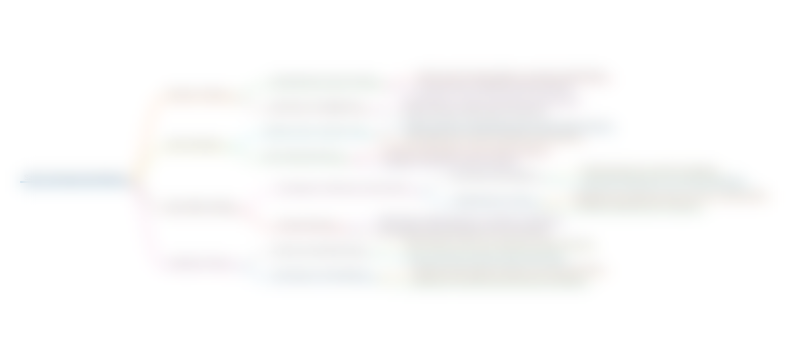
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
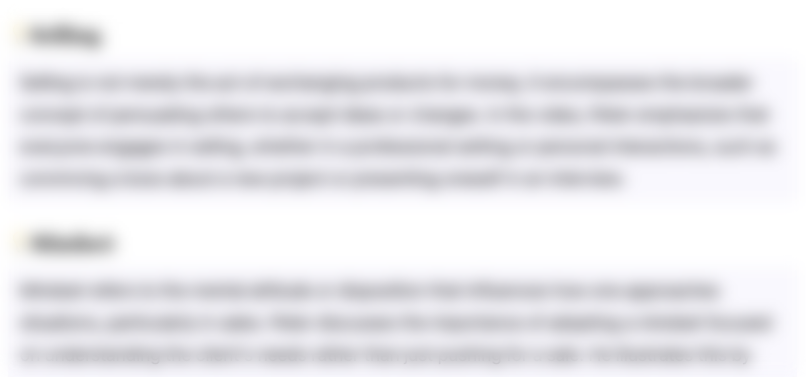
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
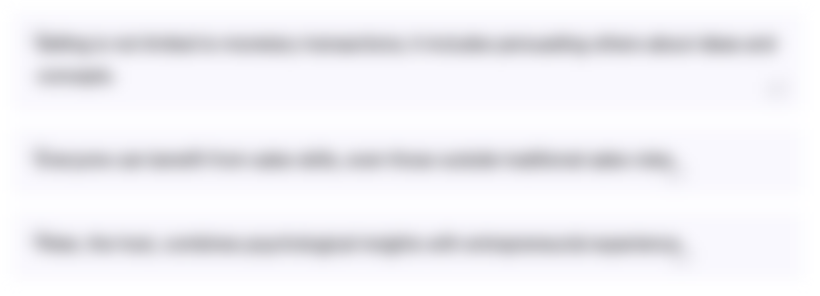
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
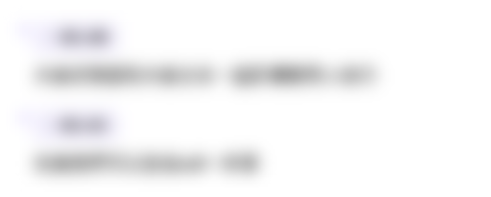
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)