Coding Encrypted Chat in Python
Summary
TLDRIn this tutorial video, the host guides viewers through the process of creating an encrypted chat application in Python. Utilizing the RSA library for encryption and core Python modules like socket and threading, the video demonstrates how to establish a secure communication channel that prevents eavesdropping on network traffic. The host also illustrates the effectiveness of encryption using Wireshark to compare clear text and encrypted message transmission, emphasizing the importance of keeping private keys secure for maintaining message confidentiality.
Takeaways
- 😀 The video is a tutorial on creating an encrypted chat in Python.
- 🔒 It emphasizes the importance of encryption for secure communication, preventing third-party eavesdropping.
- 📚 The tutorial uses the RSA library for encryption, which is an external module in addition to Python's core modules like socket and threading.
- 🔑 The concept of asymmetric encryption is introduced, explaining the use of a public key for encryption and a private key for decryption.
- 🔄 The video demonstrates creating a public-private key pair at the start of the session, which is not typical for long-term use cases.
- 💼 The public key can be shared openly, while the private key must remain confidential to ensure message security.
- 🔗 The script involves a client-server model with a choice for the user to either host or connect to a chat session.
- 🤖 The script uses threading to handle both sending and receiving messages simultaneously in separate threads.
- 📡 The tutorial includes a practical demonstration of network traffic using Wireshark to contrast encrypted versus unencrypted messages.
- 🛑 The video shows that without encryption, messages can be easily read by sniffing network traffic, but with encryption, the content is secure.
- 🎓 The tutorial concludes with a demonstration of the encrypted chat in action and a comparison using Wireshark, highlighting the security benefits of encryption.
Q & A
What is the main topic of the video?
-The video is about building an encrypted chat application in Python.
Why is encryption important in chat applications?
-Encryption is important to ensure that the communication between clients is secure and cannot be easily intercepted or read by third parties.
What is the difference between encrypted and unencrypted network communication?
-Encrypted communication means that the messages are encoded and can only be decoded by the intended recipient using a private key, making it secure against eavesdropping. Unencrypted communication sends messages in clear text, which can be easily read by anyone who can access the network traffic.
What tool is used in the video to demonstrate the difference between encrypted and unencrypted network traffic?
-Wireshark is used to capture and analyze network traffic to show the difference between encrypted and unencrypted messages.
What is the RSA library used for in this context?
-The RSA library is used for implementing asymmetric encryption, which involves a public key for encrypting messages and a private key for decrypting them.
How does asymmetric encryption with RSA work?
-In asymmetric encryption, there are two keys: a public key that can be shared with anyone and is used to encrypt messages, and a private key that is kept secret and is used to decrypt messages. The private key can only decrypt messages that were encrypted with the corresponding public key.
What are the two main functions that handle message sending and receiving in the chat application?
-The two main functions are the sending messages function and the receiving messages function, both of which run in separate threads to handle communication with the client.
How are the keys exchanged between clients in the encrypted chat application?
-The keys are exchanged by sending the public key of one client to the other client over the established connection. The public key is then used to encrypt messages that can only be decrypted with the recipient's private key.
What is the significance of using a private key and a public key in the encryption process?
-The private key is used to decrypt messages that were encrypted with the corresponding public key. It is kept secret to ensure that only the intended recipient can decrypt and read the messages. The public key can be shared openly and is used to encrypt messages for the recipient.
How does the video demonstrate the effectiveness of the encryption in the chat application?
-The video demonstrates the effectiveness of encryption by showing the inability to read the content of encrypted messages when using Wireshark to sniff network traffic, as opposed to the clear text messages that can be easily read when encryption is not used.
Outlines
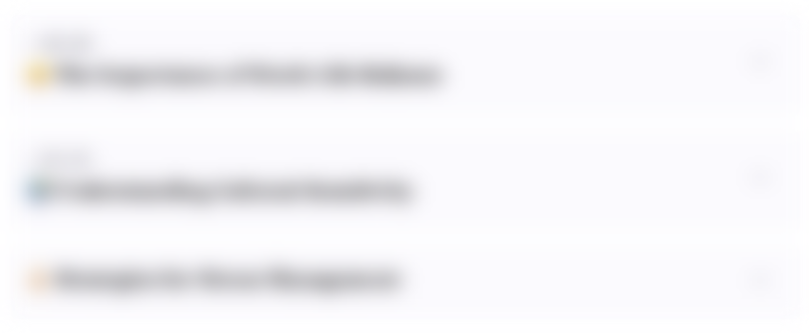
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
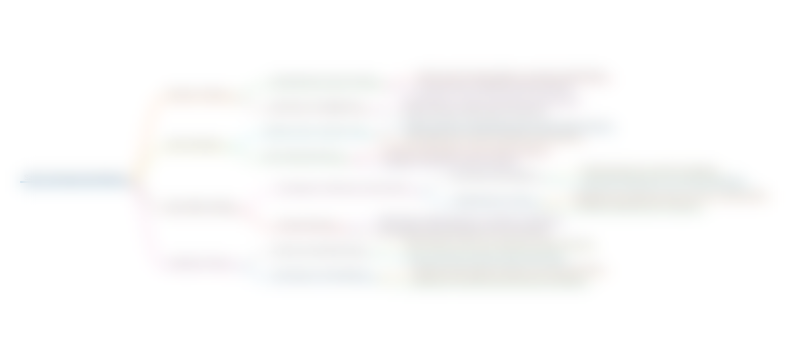
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
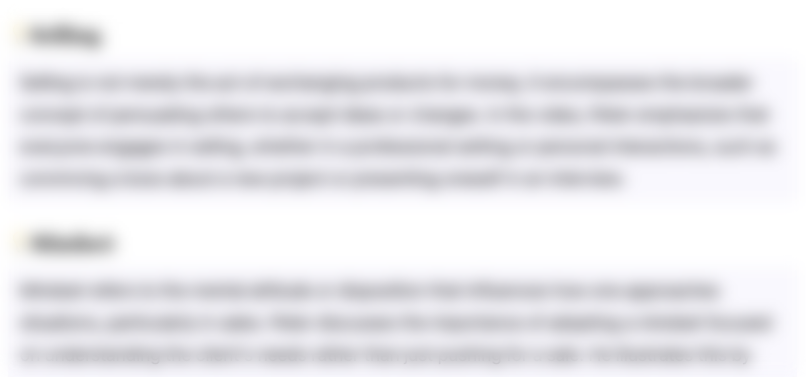
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
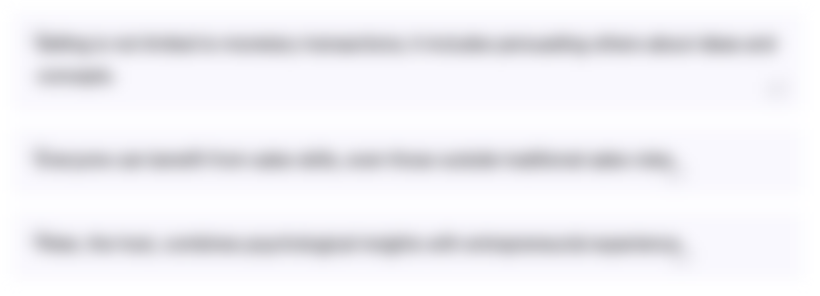
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
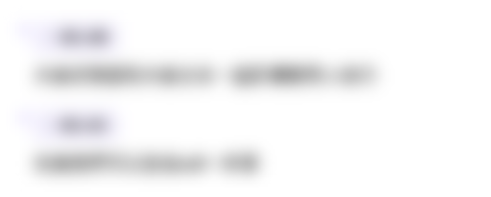
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
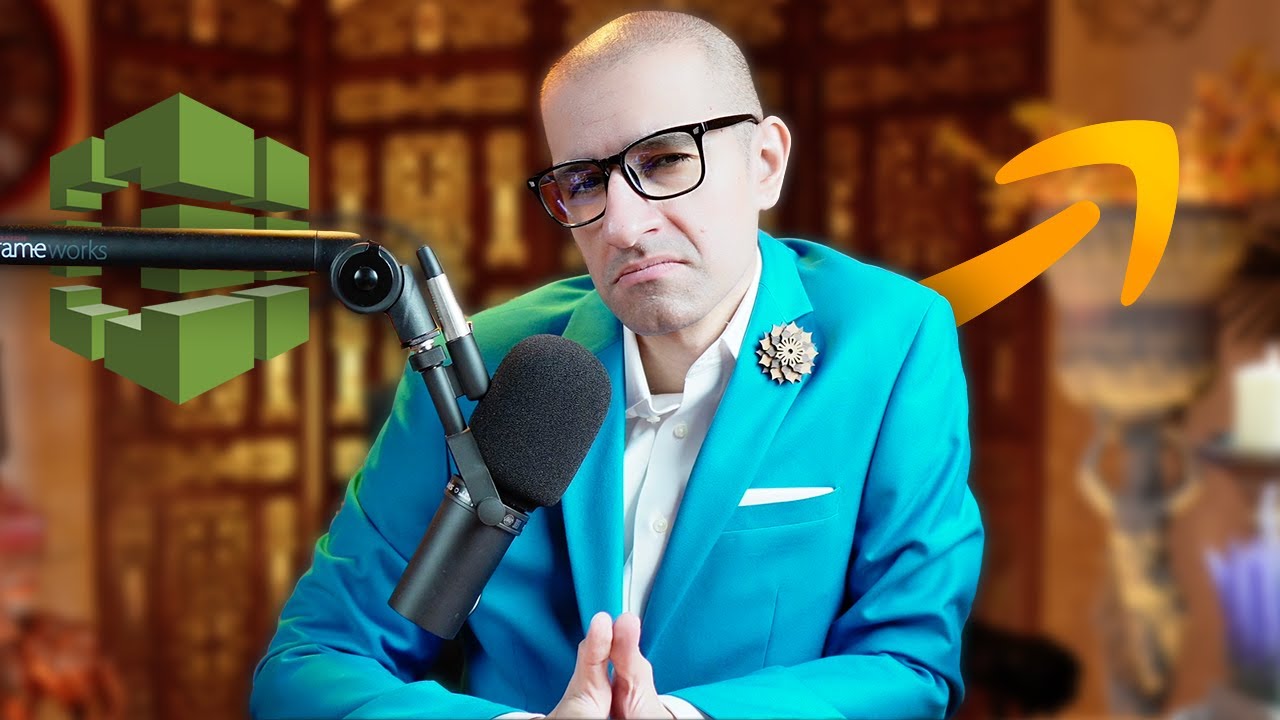
How To Deploy Serverless SAM Using Code Pipeline (5 Min) | Using AWS Code Build & Code Commit
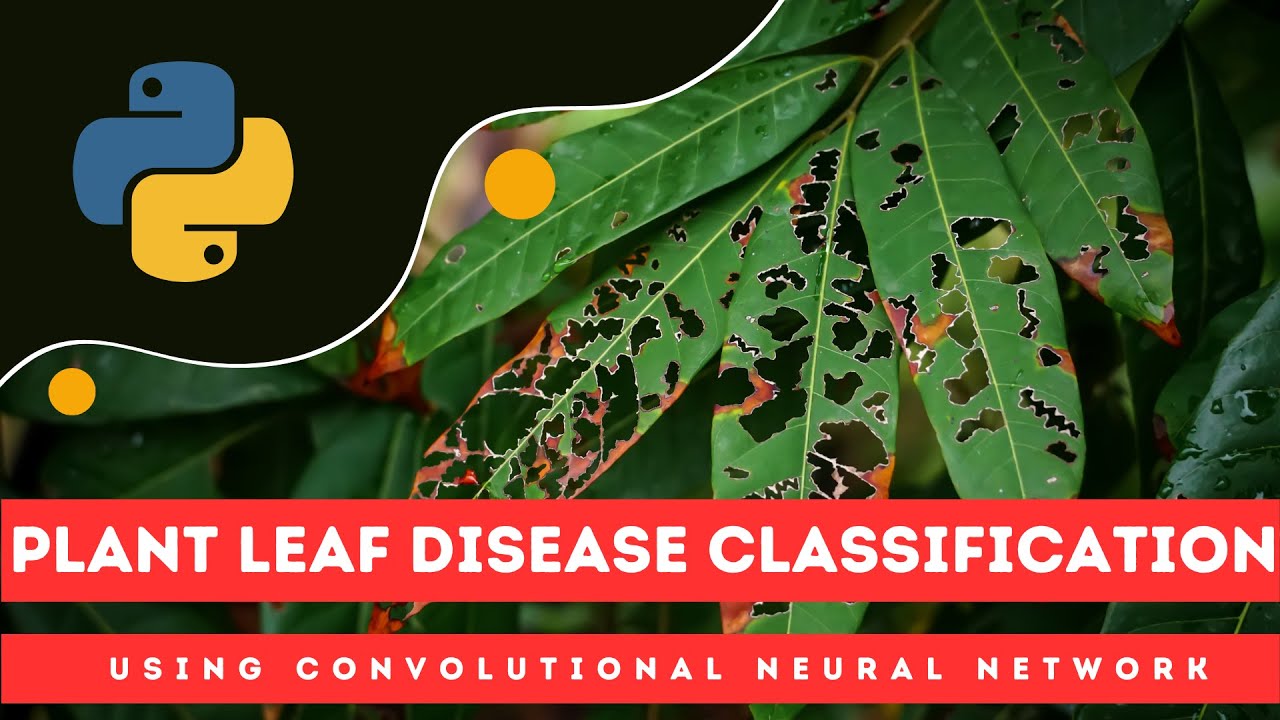
Plant Leaf Disease Detection Using CNN | Python
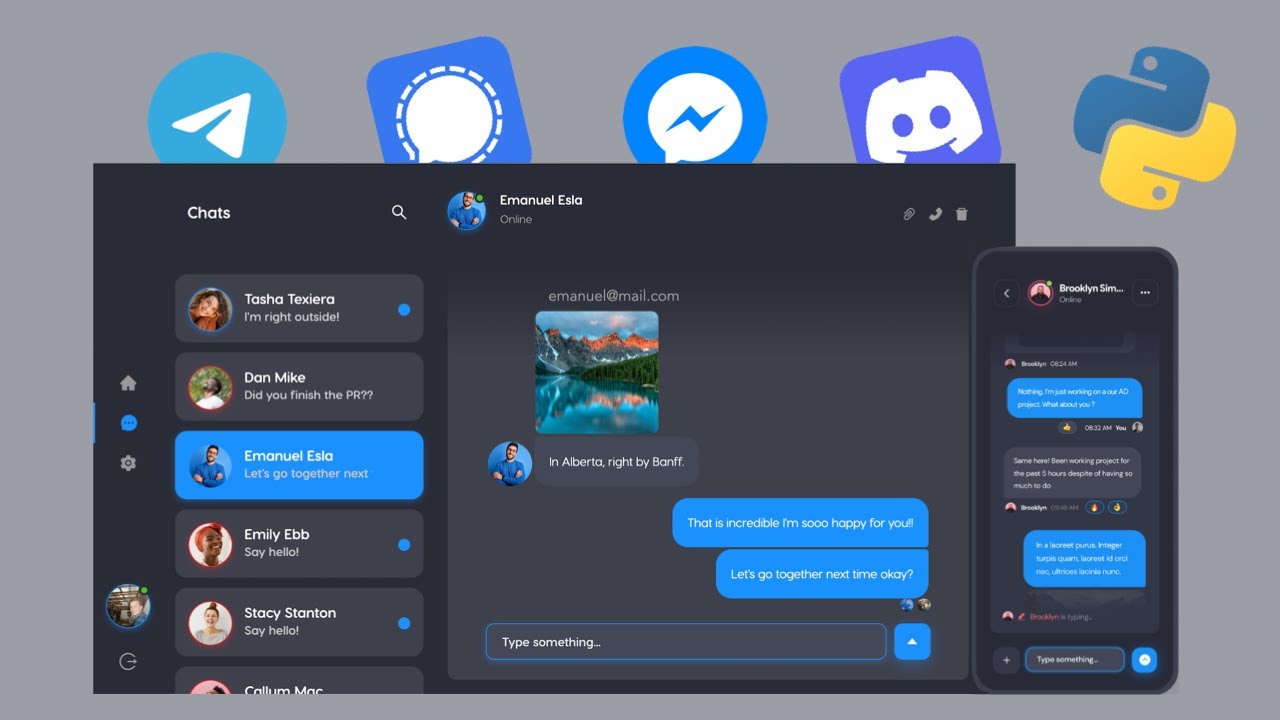
Build a 🔥 Chat App with FastAPI and React JS (👾 Discord Clone)

Chat With Documents Using ChainLit, LangChain, Ollama & Mistral 🧠
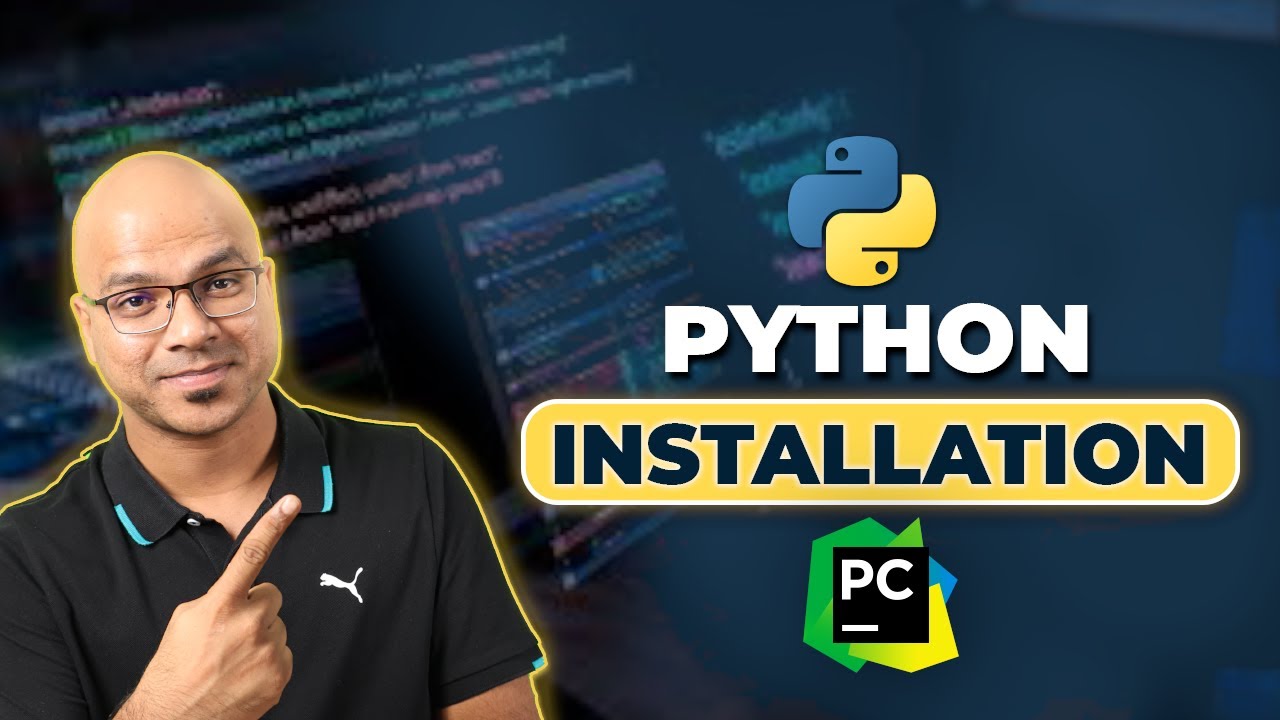
#2 Python Tutorial for Beginners | Python Installation | PyCharm
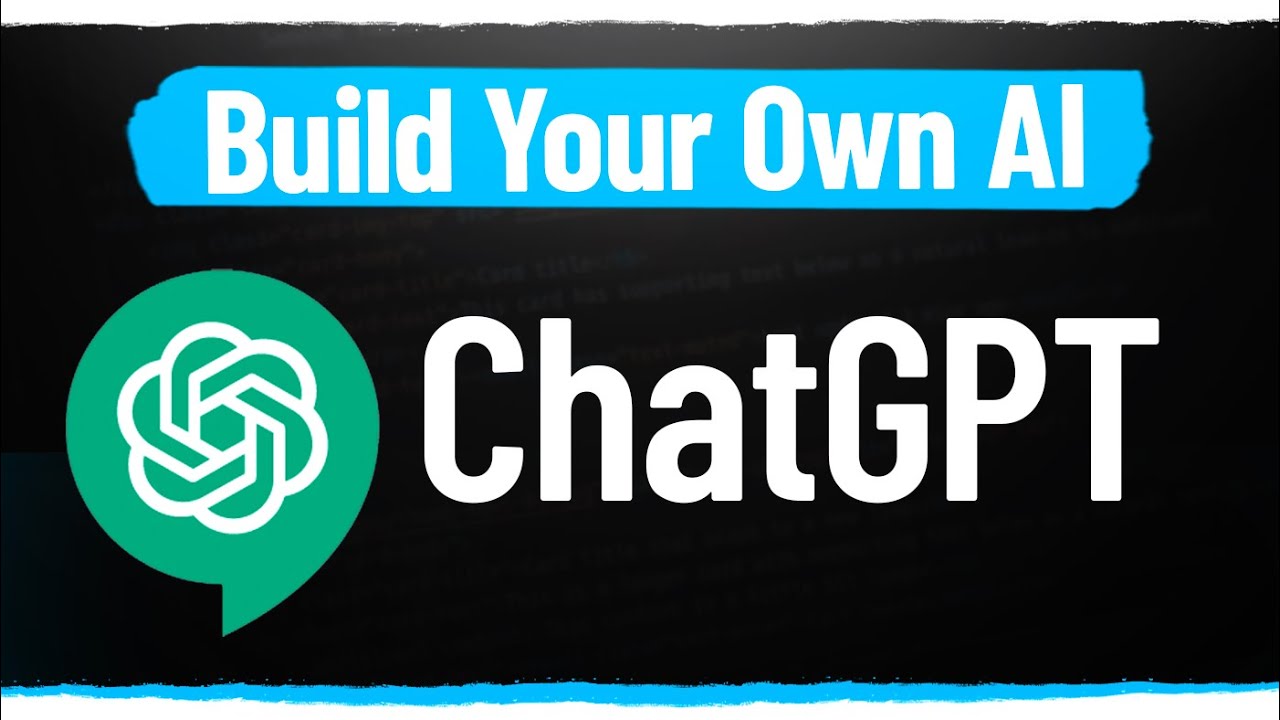
How To Build Your Own AI With ChatGPT API
5.0 / 5 (0 votes)