Class Inheritance | Godot GDScript Tutorial | Ep 17
Summary
TLDRThis tutorial episode explores class inheritance in Godot's GDScript, explaining the concept of deriving classes to share attributes and methods. It clarifies that multiple inheritance is not supported in GDScript. The script demonstrates how to use the 'extends' keyword, the roles of base and derived classes, and the importance of constructors for variable assignment and method overriding. It showcases practical examples, including creating animal subclasses with unique behaviors, and emphasizes inheritance's role in promoting cleaner, more efficient code reuse.
Takeaways
- ๐ Class inheritance in Godot allows for creating a hierarchy of classes that share attributes and methods.
- โ Godot does not support multiple inheritance, meaning a class can only inherit from one other class.
- ๐ To inherit from a class, use the 'extends' keyword followed by the class name or a file path enclosed in double quotes.
- ๐ The terms 'base class' and 'superclass' are synonymous, referring to the class whose properties are inherited.
- ๐ด The 'derived class' or 'subclass' is the class that inherits properties from the base class, such as the 'horse' class inheriting from the 'animal' class.
- ๐ซ A subclass cannot have a member variable with the same name as one in the superclass; use the constructor to assign or change values from the superclass.
- ๐ก The power of inheritance lies in reusing code and making small tweaks to subclasses, reducing the amount of code needed for each class.
- ๐ Inheritance enables overriding functions in subclasses, allowing specific implementations of methods provided by the superclass.
- ๐ Godot supports multiple layers of inheritance, where a class can inherit from another, which in turn inherits from another, and so on.
- ๐ถ Using constructors and class objects with inheritance can save time and lead to cleaner code, especially when deriving many classes from a base class.
- ๐ ๏ธ The video script provides practical examples, such as the 'animal', 'horse', and 'frog' classes, to illustrate inheritance concepts and their application.
Q & A
What is class inheritance in the context of programming?
-Class inheritance is a mechanism where a new class, known as the derived or subclass, is based on an existing class, known as the base or superclass. The subclass inherits attributes and methods from the superclass, creating a hierarchy of classes that share a set of functionalities.
Is multiple inheritance allowed in Godot's GDScript?
-No, in Godot's GDScript, multiple inheritance is not allowed. A class can only inherit from one other class or file.
How do you implement inheritance in GDScript?
-In GDScript, inheritance is implemented using the 'extends' keyword followed by the class name or a file path wrapped in double quotes, which turns it into a string.
What is the difference between a base class and a derived class?
-A base class, also known as a superclass, is the class from which properties are inherited. A derived class, also known as a subclass, is the class that inherits properties from the base class.
Why can't a subclass have a member variable with the same name as one in the superclass?
-A subclass cannot have a member variable with the same name as the superclass to avoid conflicts and ensure that the subclass does not unintentionally override or shadow the superclass's variable.
How can a subclass change the value of a member variable inherited from the superclass?
-A subclass can change the value of an inherited member variable by using the constructor method to assign or change the value of the variable from the subclass to the superclass.
What is the purpose of using inheritance in programming?
-Inheritance is used to promote code reuse, reduce redundancy, and create a hierarchical structure of classes. It allows for cleaner code and easier definition of classes with similar functionalities but with additional or modified behaviors.
Can functions be overridden in GDScript?
-Yes, in GDScript, you can override functions from the superclass in the subclass to provide a specific implementation of a method that is already provided by the superclass.
What does it mean to override a function in a subclass?
-Overriding a function in a subclass means providing a new implementation for a method that already exists in the superclass, allowing the subclass to have its own specific behavior for that method.
Can you have multiple layers of inheritance in GDScript?
-Yes, GDScript allows for multiple layers of inheritance. A class can inherit from another class, which in turn can inherit from another, creating a chain of inheritance where a subclass can access methods and variables from all its ancestor classes.
What are some tips for using inheritance effectively in GDScript?
-Effective use of inheritance in GDScript includes using it for cleaner code, reusing code from a base class, and making small tweaks for individual subclasses. It's also important to use constructors and class objects to pass down values and change them as needed.
Outlines
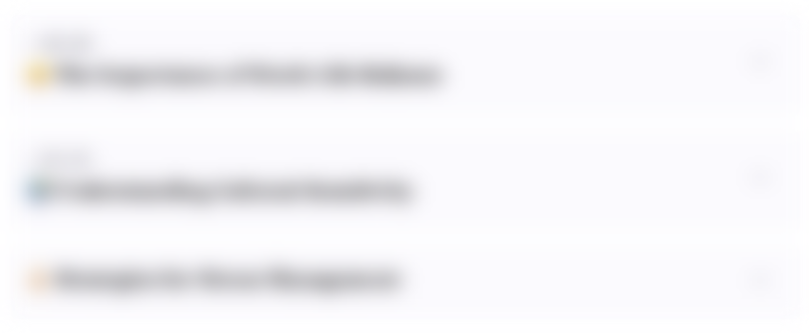
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
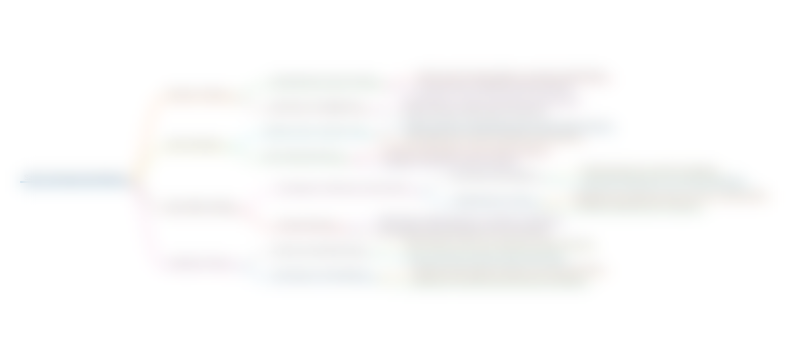
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
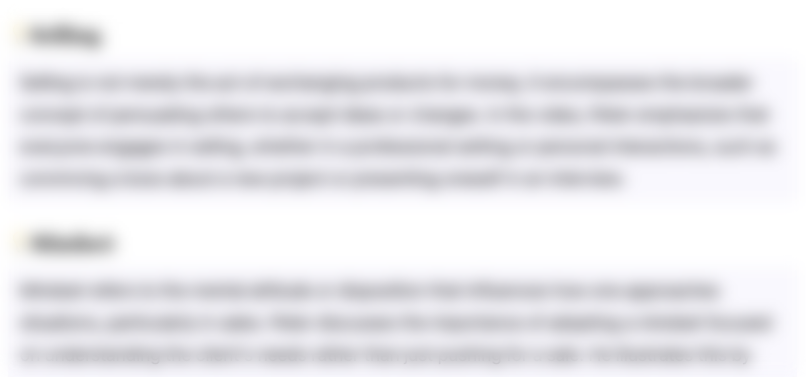
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
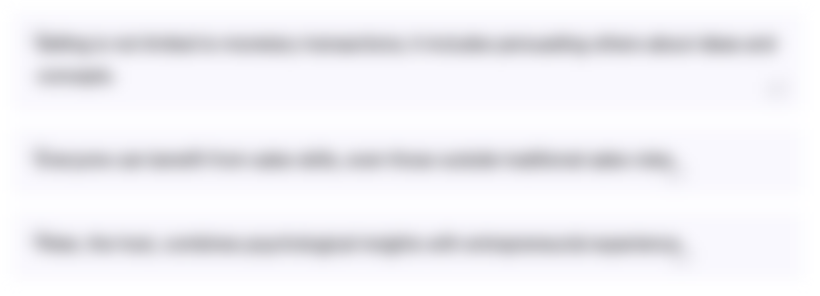
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
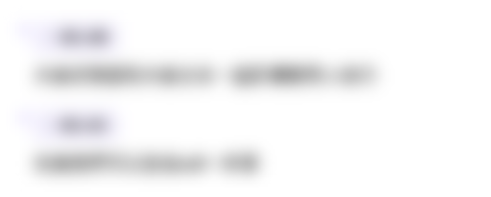
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)