While Loops | Godot GDScript Tutorial | Ep 07
Summary
TLDRThis tutorial episode delves into 'while loops', a fundamental control flow statement in programming that executes code repeatedly based on a boolean condition. It explains the structure of a while loop, the potential for infinite loops, and distinguishes between three types: fake (appears infinite but exits due to a break statement), intended (used in applications like game development for continuous operation), and unintended (resulting from an oversight that can freeze an application). The episode aims to educate viewers on the importance of loop management to avoid unintended infinite loops.
Takeaways
- ๐ A 'while loop' is a control flow statement that executes code repeatedly based on a boolean condition.
- ๐ The 'while' loop includes a test expression that evaluates to either true or false, dictating whether the loop continues or exits.
- ๐ A flowchart is an effective way to visualize the operation of a while loop, showing the decision point and the loop's continuation or termination.
- ๐ If the test expression always evaluates to true without a way to exit, it results in an 'infinite loop', which is a loop that repeats indefinitely.
- ๐ฎ There are three types of infinite loops: 'fake infinite loops', 'intended infinite loops', and 'unintended infinite loops'.
- ๐ก A 'fake infinite loop' appears endless but actually contains a break statement that will exit the loop, making it run only once.
- ๐ฎ An 'intended infinite loop' is used intentionally, such as in a game where the script runs on every frame until the user exits.
- โ ๏ธ An 'unintended infinite loop' is a mistake where the programmer forgets to include an exit condition, which can freeze the application.
- ๐ ๏ธ Understanding the different types of infinite loops is crucial for preventing application crashes and ensuring smooth code execution.
- ๐ The script emphasizes the importance of proper loop management to avoid unintended infinite loops and the potential for application freezing.
Q & A
What is a while loop in programming?
-A while loop is a control flow statement that allows code to be executed repeatedly based on a given boolean condition.
What is the basic structure of a while loop?
-A while loop consists of the keyword 'while' followed by a test expression that evaluates to either true or false. If the test is true, the code block within the loop is executed.
What happens if the test expression in a while loop evaluates to true?
-If the test expression evaluates to true, the code within the loop is executed, and then the loop returns to the test expression to check its value again.
What occurs when the test expression in a while loop evaluates to false?
-If the test expression evaluates to false, the loop is exited, and the program continues with the code following the loop.
Can you explain the concept of an infinite loop?
-An infinite loop, sometimes called an endless loop, is a piece of code that lacks a functional exit, causing it to repeat indefinitely.
What are the three types of infinite loops mentioned in the script?
-The three types of infinite loops are fake infinite loops, intended infinite loops, and unintended infinite loops.
What is a fake infinite loop?
-A fake infinite loop gives the impression of being infinite, but it contains a break statement or some condition that will eventually cause it to exit.
What is an intended infinite loop?
-An intended infinite loop is a loop where the programmer intentionally does not want the loop to end, such as in a game loop that runs until the user decides to exit.
What is an unintended infinite loop?
-An unintended infinite loop occurs when a programmer accidentally forgets to include a condition to exit the loop, which can lead to the application freezing.
How can an infinite loop be problematic in a coding environment?
-An unintended infinite loop can cause an application to freeze or crash, as it will continuously execute without a way to stop.
Can you provide an example of an intended infinite loop in GDScript?
-An example of an intended infinite loop in GDScript is when the play button is pressed, creating a loop that runs every script on every frame until the user exits the game.
Outlines
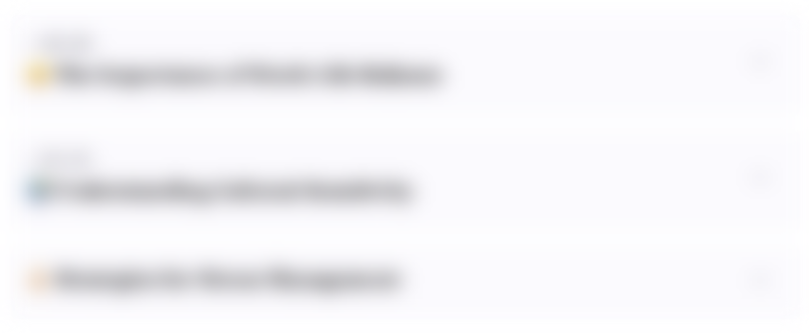
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
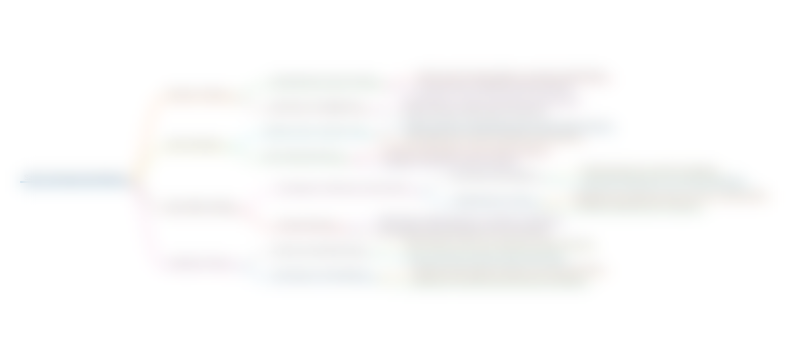
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
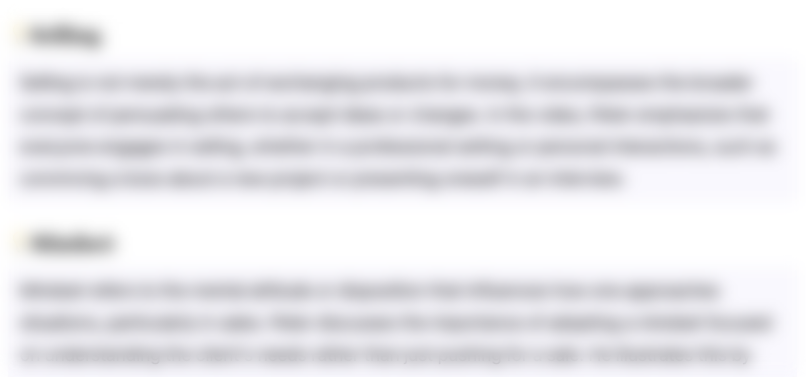
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
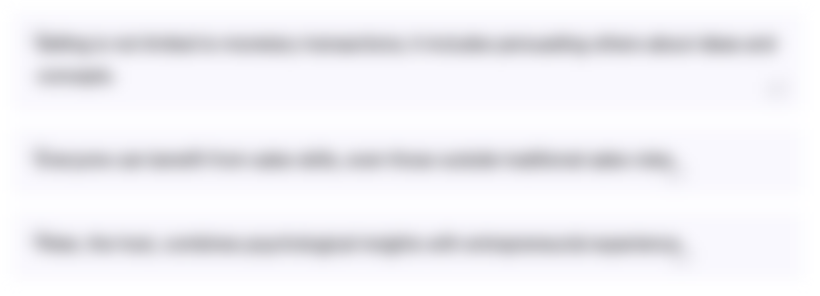
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
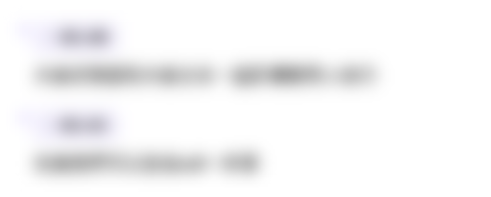
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

The 'while' Statement in C++
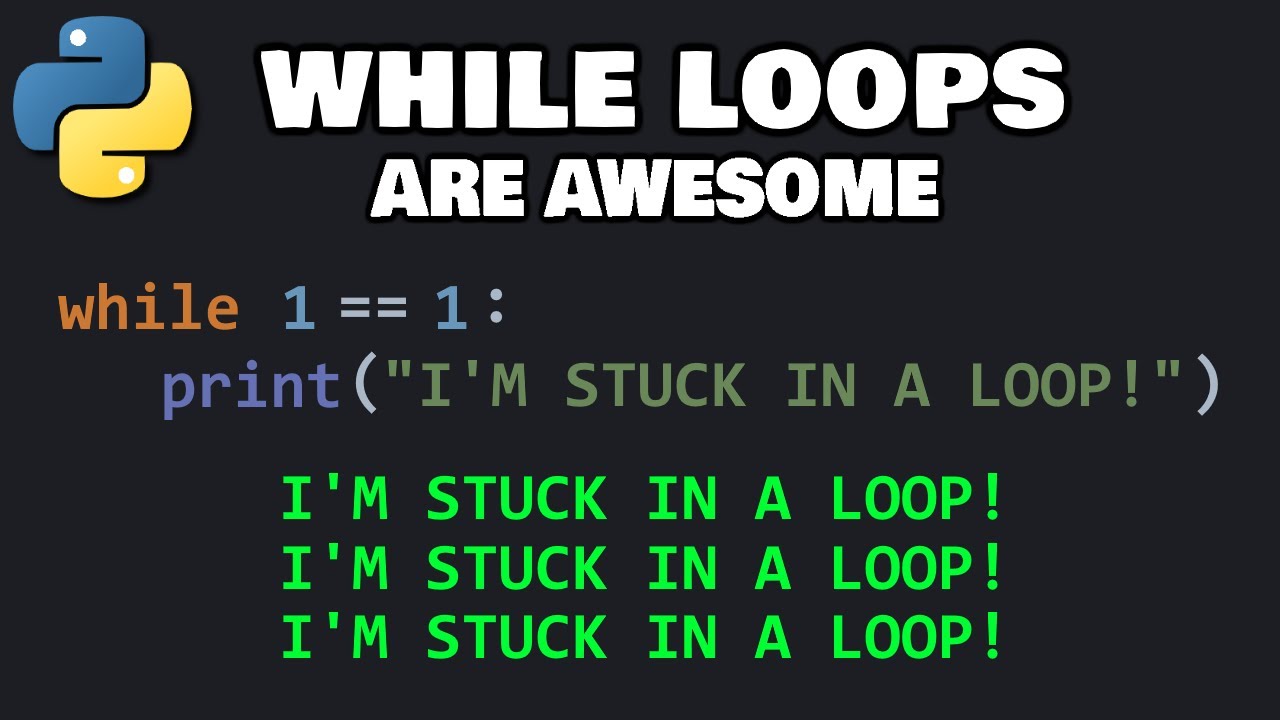
While loops in Python are easy โพ๏ธ
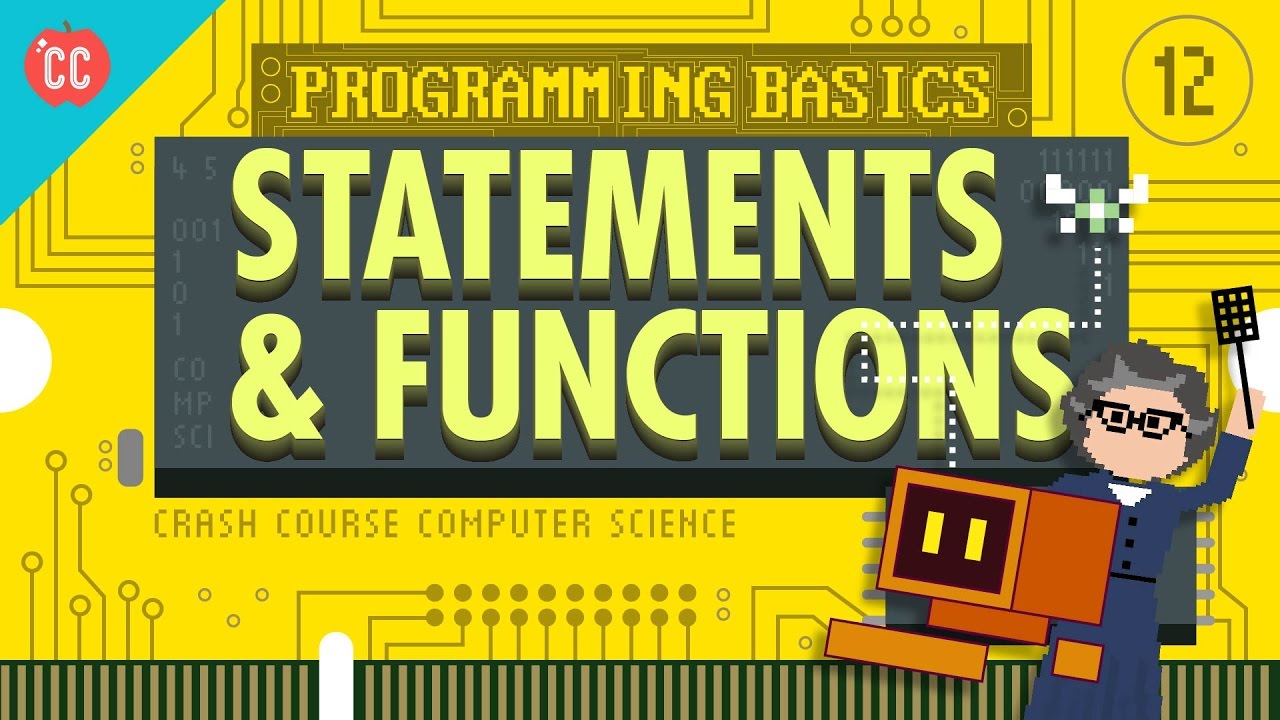
Programming Basics: Statements & Functions: Crash Course Computer Science #12
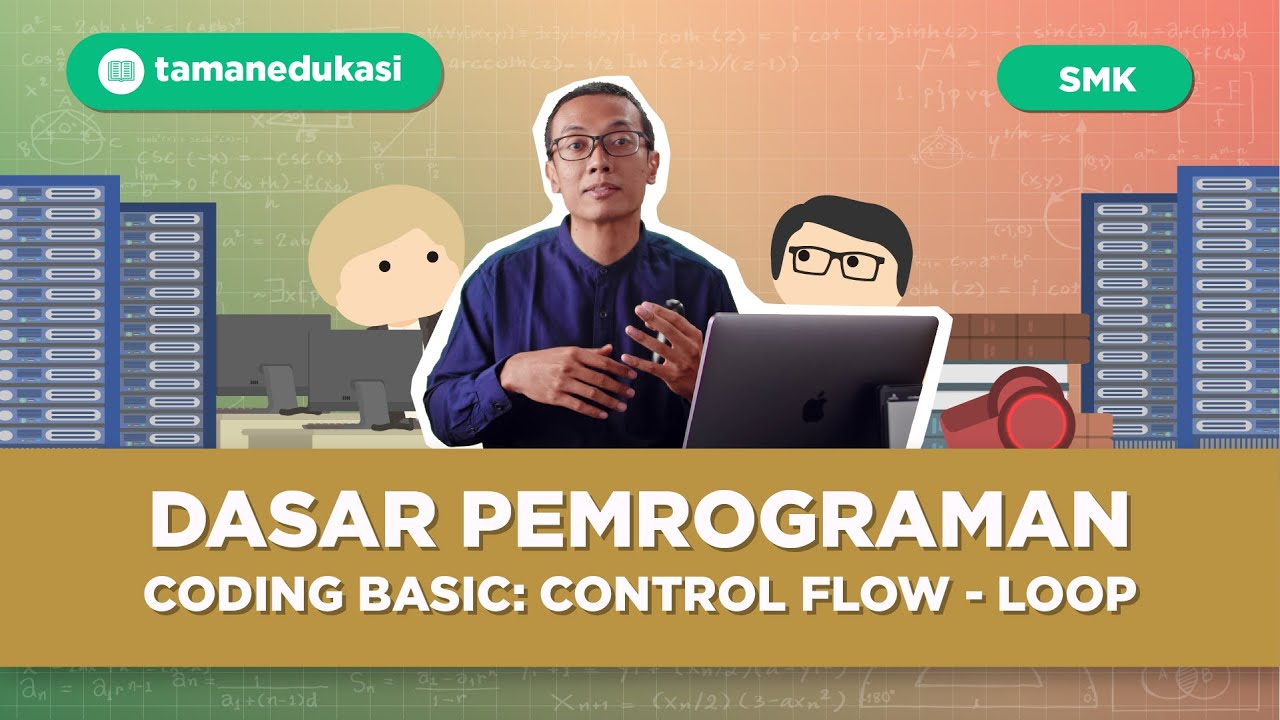
Mengenalย Salah Satu Jenis Alur Kendali yaitu: Loop / Pengulangan
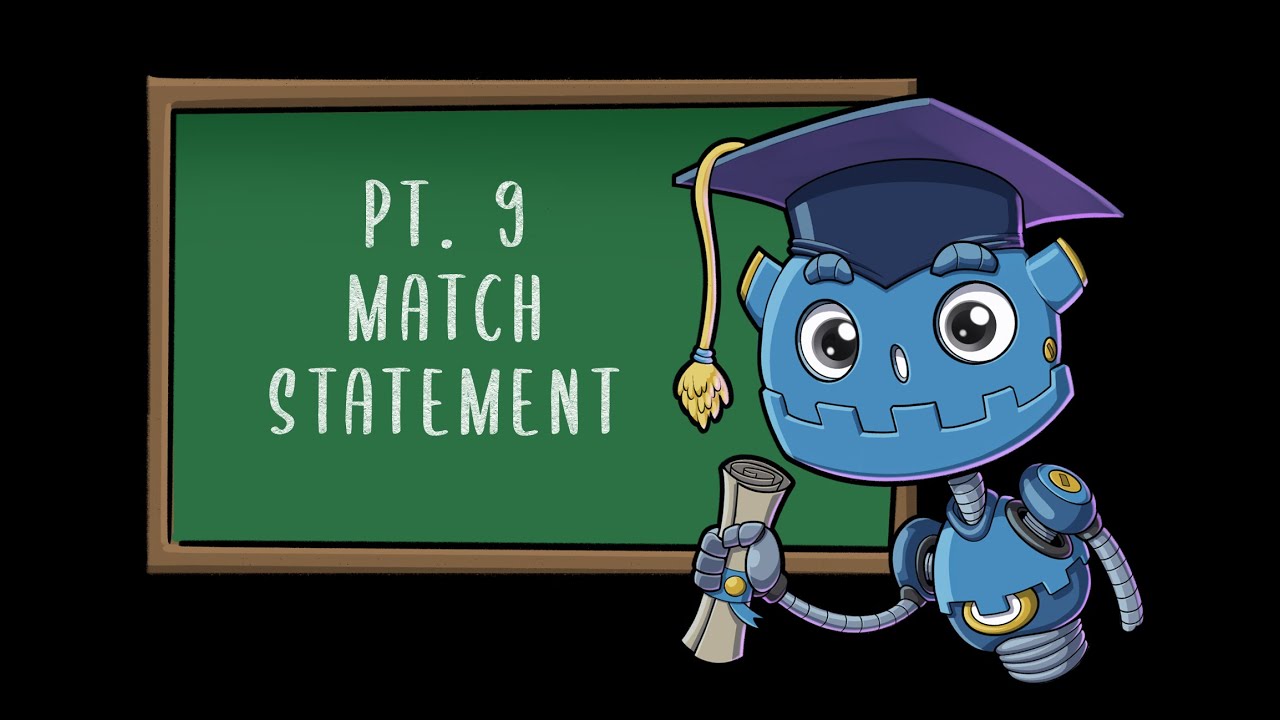
Match Statements Pt 1 | Godot GDScript Tutorial | Ep 09

โC++ Final Review Loop Quiz 5 Questions
5.0 / 5 (0 votes)