#32 Custom Route Constraint | Understanding Routing | ASP.NET Core MVC Course
Summary
TLDRIn this tutorial, the instructor demonstrates how to create and use a custom route constraint in ASP.NET Core to accept only alphanumeric usernames. The video walks through creating a class that implements the IRouteConstraint interface, explaining the match method, and applying a regular expression to validate the route parameter. The process includes registering the custom constraint in the Program.cs file and testing different inputs to ensure the constraint works. Finally, the instructor provides an assignment to create additional custom constraints for month values (1-12) and date formats, encouraging viewers to extend their learning.
Takeaways
- 😀 Learn how to create and use a custom (user-defined) route constraint in ASP.NET Core.
- 😀 A user-defined route constraint helps ensure route parameters match specific criteria (e.g., alphanumeric values).
- 😀 The first step in creating a custom route constraint is to define a route pattern using the 'endpoint.mapGet' method.
- 😀 ASP.NET Core has built-in constraints like 'Alpha', but it lacks a built-in constraint for alphanumeric values, which can be addressed with a custom constraint.
- 😀 A custom route constraint is created by implementing the 'IRouteConstraint' interface and its 'Match' method.
- 😀 The 'Match' method checks if the route parameter (e.g., 'username') matches specific conditions, using tools like regular expressions.
- 😀 Regular expressions are used to validate whether the route parameter contains only alphanumeric characters, preventing special characters.
- 😀 After creating the custom constraint class, it needs to be registered in the 'Program.cs' file before building the application using 'AddRouting' and 'ConstraintMap'.
- 😀 To apply the custom constraint, it is referenced in the route definition (e.g., '{username:alphanumeric}') within the routing setup.
- 😀 The script demonstrates how to test the constraint by passing various route values (e.g., alphanumeric, alphabetic, numeric) and checking if the constraint is applied correctly.
Q & A
What is a user-defined route constraint in ASP.NET Core?
-A user-defined route constraint, also known as a custom route constraint, is a custom validation rule applied to route parameters in ASP.NET Core to restrict the types of values a route parameter can accept. It allows developers to define their own conditions for route parameter values beyond the built-in constraints.
How do you create a custom route constraint in ASP.NET Core?
-To create a custom route constraint in ASP.NET Core, you need to create a class that implements the `IRouteConstraint` interface. This class should provide an implementation for the `Match` method, where you can define the logic for validating route parameter values (e.g., using regular expressions).
What is the role of the `Match` method in the `IRouteConstraint` interface?
-The `Match` method in the `IRouteConstraint` interface is responsible for validating whether the route parameter values match the defined constraint. It takes several parameters, including the HTTP context, route, route parameters, and request direction, and it returns `true` if the value is valid or `false` otherwise.
What are the five parameters of the `Match` method?
-The five parameters of the `Match` method are: `HttpContext` (provides context of the incoming request), `IRouter` (the route on which the constraint is applied), `routeKey` (the name of the route parameter being checked), `values` (a dictionary of route parameter names and their values), and `routeDirection` (an enum indicating whether the request is incoming or a URL is being generated).
What is the role of the `RouteDirection` enum in the `Match` method?
-The `RouteDirection` enum specifies whether the request is incoming (when a URL is being processed) or if a URL is being generated. In the case of custom route constraints, `RouteDirection` is typically set to `IncomingRequest`, meaning the constraint checks an incoming URL request.
What is the purpose of using regular expressions (regex) in the custom route constraint?
-Regular expressions are used in the custom route constraint to validate the format of the route parameter value. For example, to ensure the value is alphanumeric (letters and numbers), a regex pattern is created to match the required format, rejecting any special characters or invalid values.
How do you register a custom route constraint in an ASP.NET Core application?
-To register a custom route constraint, you need to use the `ConstraintMap` feature in the `Program.cs` file. You add the custom constraint to the service container using `services.AddRouting()` and then map the custom constraint to a key (e.g., 'alphanumeric') using `options.ConstraintMap`. This makes the application aware of the custom route constraint.
What is the role of the `Program.cs` file in using custom route constraints?
-The `Program.cs` file is used to configure and register custom route constraints in the application. You register the route constraint using `ConstraintMap` in the `ConfigureServices` method, which makes ASP.NET Core aware of the custom constraint and ensures that it can be applied to routes.
What happens if a route parameter does not match the custom route constraint?
-If a route parameter does not match the custom route constraint, the route does not match, and ASP.NET Core will redirect to the default route, indicating that the requested URL is not found or is invalid.
Can you create multiple custom route constraints for different use cases?
-Yes, you can create multiple custom route constraints for different use cases. For example, one constraint can be created to validate alphanumeric values for a username, while another can validate month values between 1 and 12, or a date format. Each constraint can be applied to different routes as needed.
Outlines
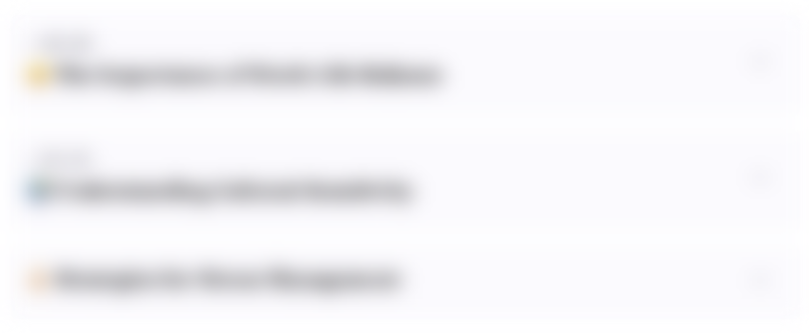
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
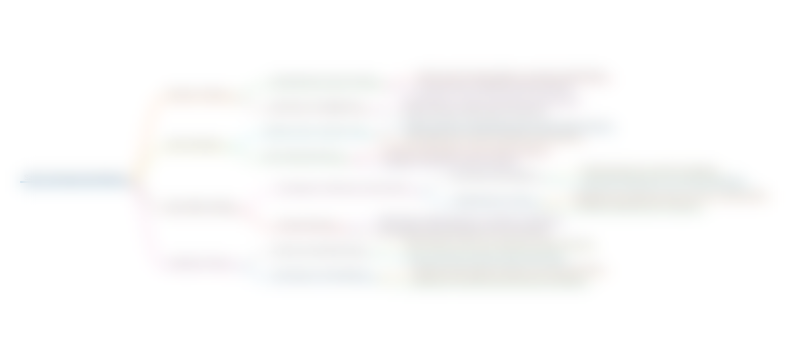
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
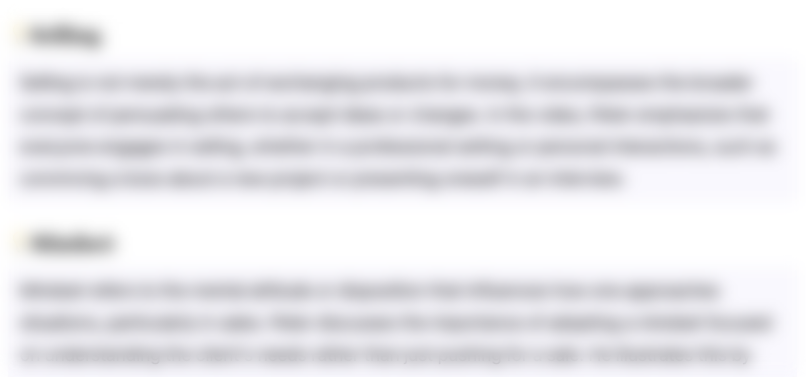
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
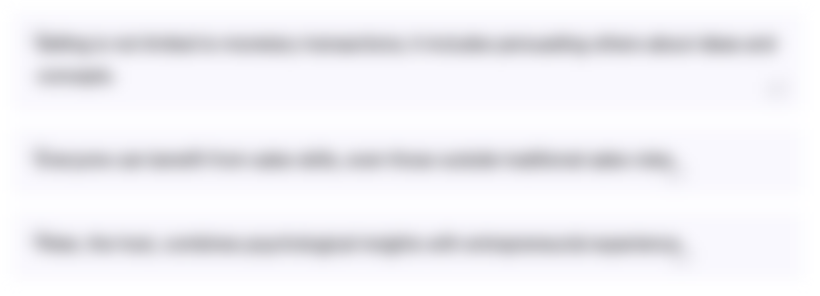
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
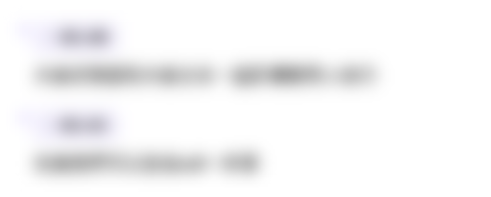
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
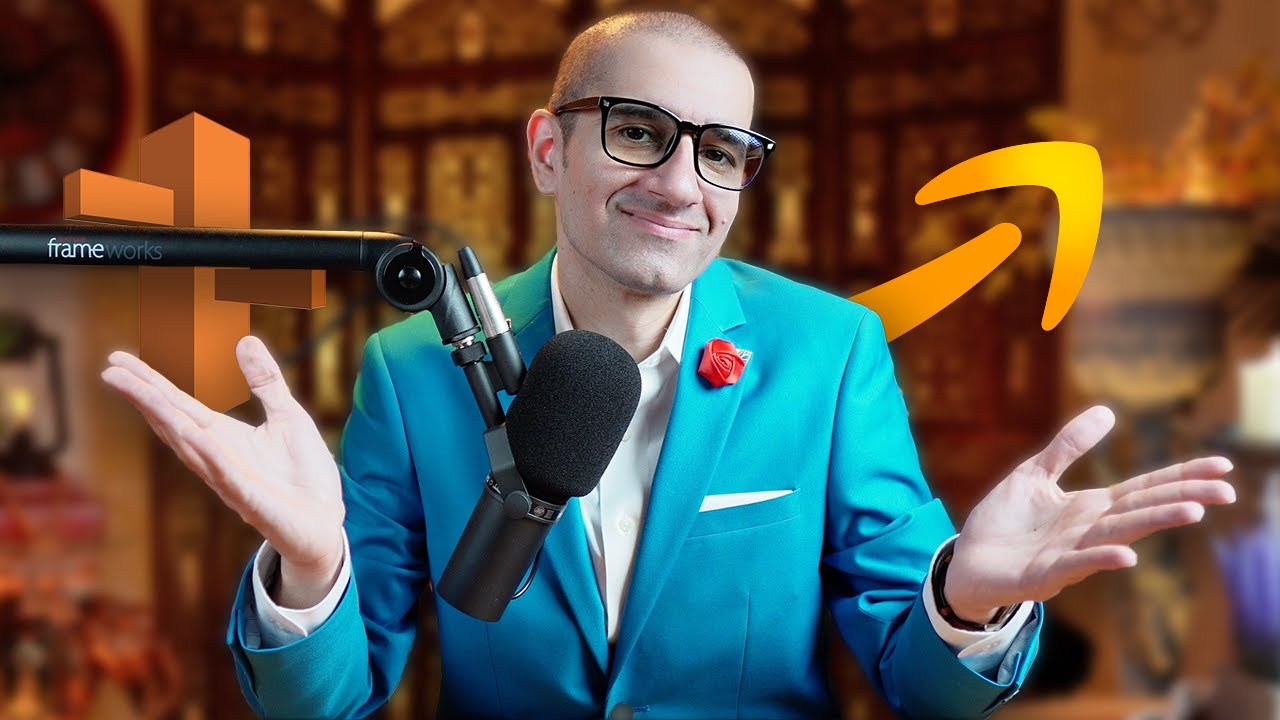
How To: Custom Domain Name In Elastic Beanstalk (2 Min) | AWS | Using Route 53 Routing

useContext Hook | Mastering React: An In-Depth Zero to Hero Video Series
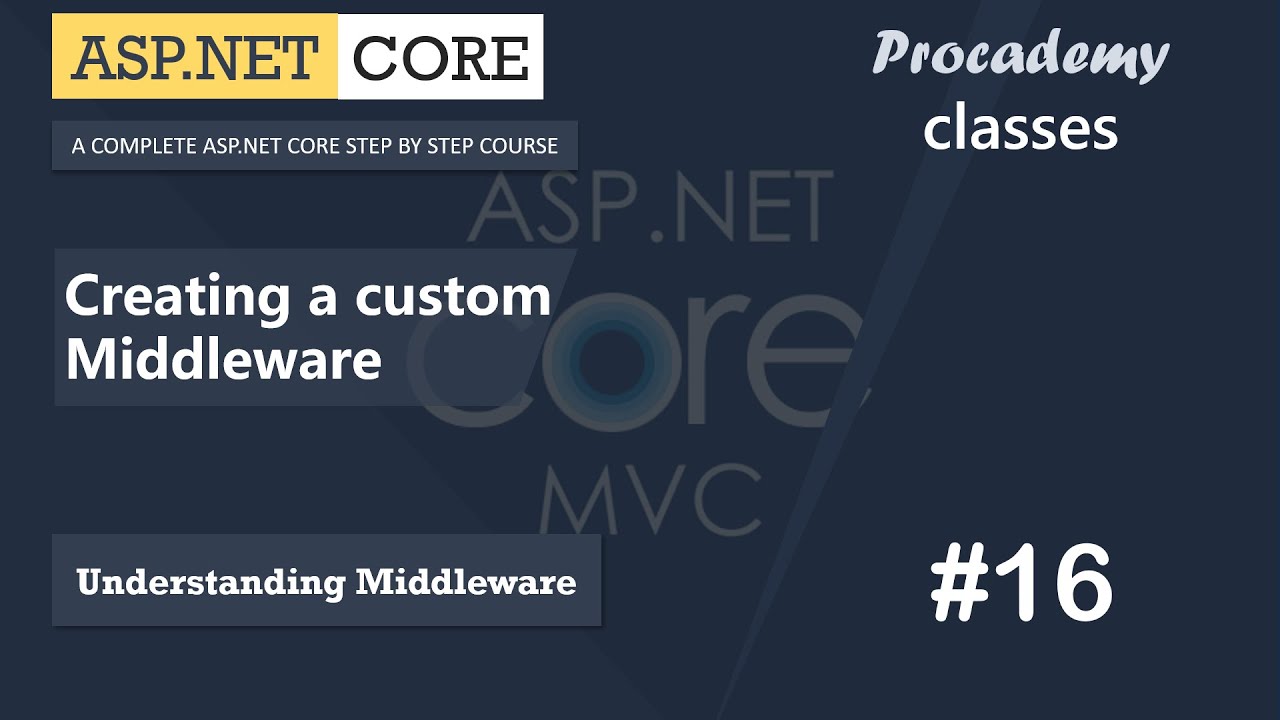
#16 Creating a Custom Middleware | Understanding Middleware | ASP.NET Core MVC Course
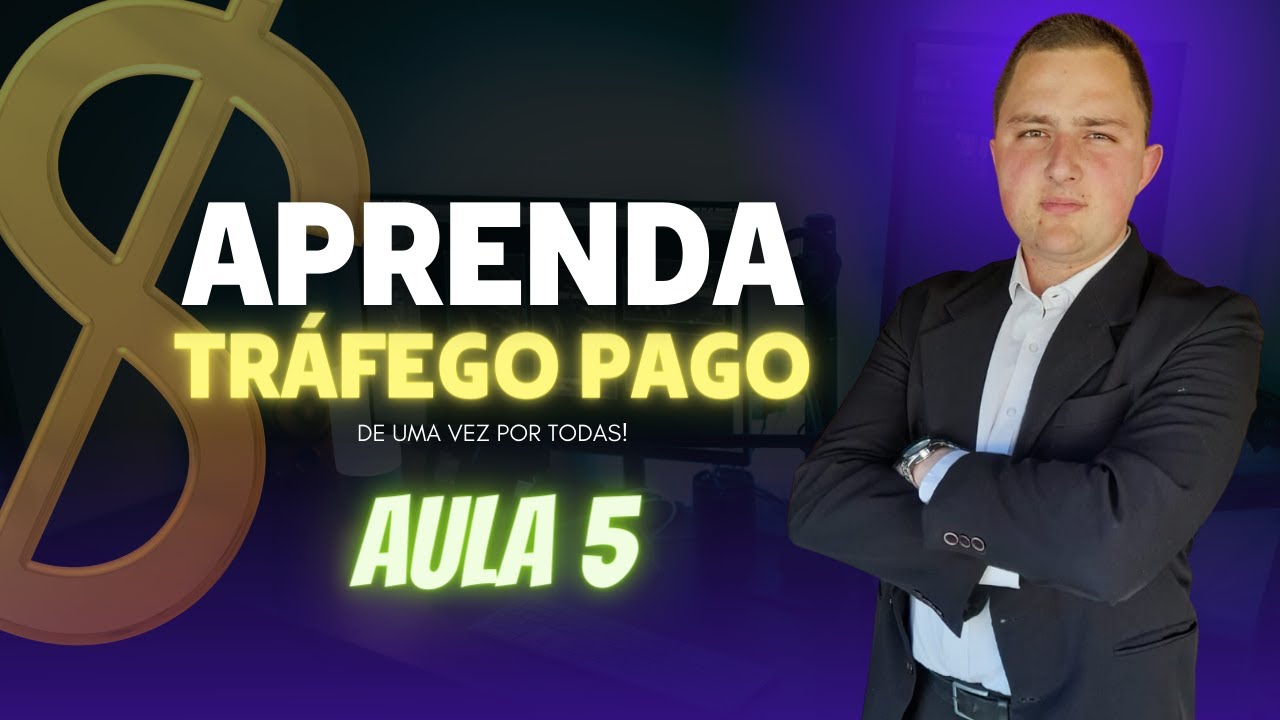
Aula 05 - Biblioteca de públicos
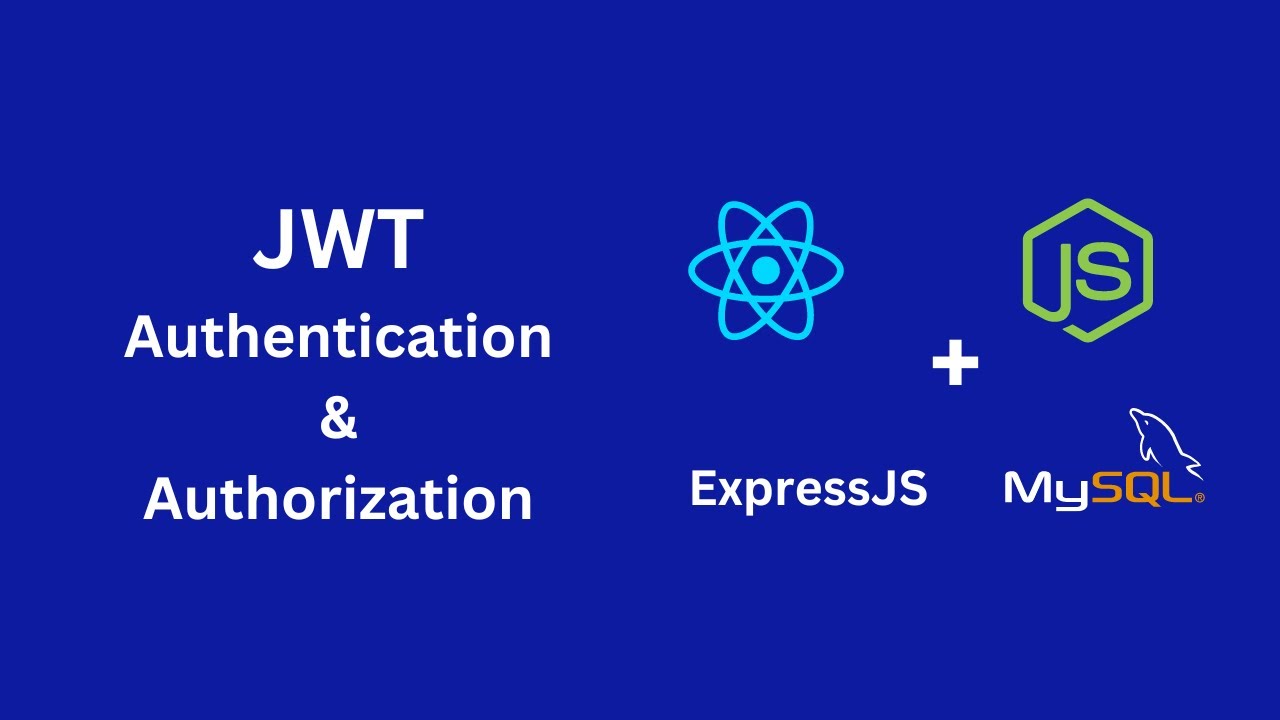
JWT Authentication with Node.js, React, MySQL | Node JS Authentication With JSON Web Token
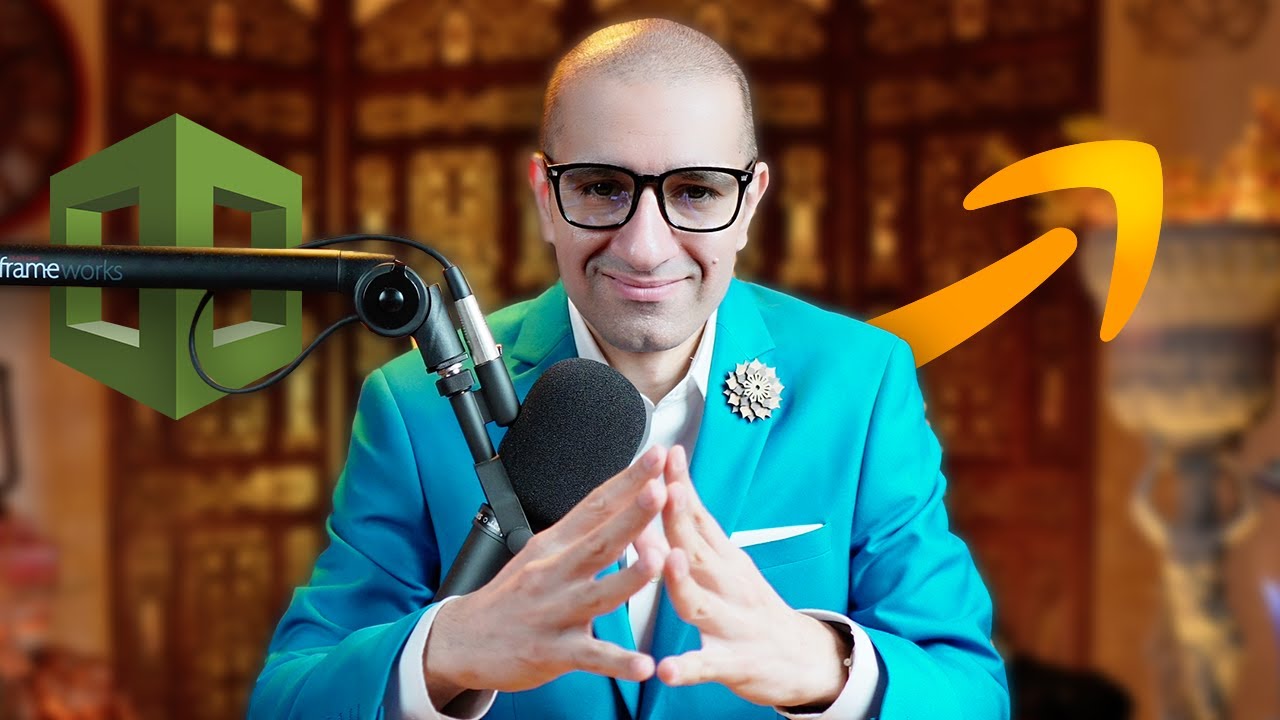
How To Host S3 Static Website With Custom Route 53 Domain (4 Min) | AWS | Set Alias To S3 Endpoint
5.0 / 5 (0 votes)