C_63 Strings in C-part 2 | Read a String using scanf and gets function
Summary
TLDRThe video script is an in-depth discussion on strings in the C programming language. It covers the basics of what a string is, how to declare and initialize strings, and the importance of null characters. The script explains compile-time data, the use of functions like 'scanf' and 'gets', and their differences, emphasizing the risks of buffer overflow. It also demonstrates how to read a string using 'scanf' and the advantages of using both functions. The video aims to educate viewers on the correct practices to avoid common pitfalls when handling strings in C.
Takeaways
- 😀 The video discusses the basics of strings in the C programming language, including how to declare, initialize, and read strings.
- 📝 It explains that strings in C are arrays of characters and emphasizes the importance of the null terminator character at the end of a string.
- 💡 The video covers the use of the 'scanf' function to read strings from the user input, including the format specifier '%s'.
- 🔍 It demonstrates how to use the 'gets' function to read a string, but also mentions its deprecation due to security risks.
- 👍 The script highlights the difference between character arrays and strings, and the need to allocate memory for strings.
- 🚫 The video warns against buffer overflow, a common security vulnerability when dealing with strings and arrays.
- 🔑 It shows how to use the 'printf' function with '%s' to print strings, and the importance of handling memory correctly.
- 🔄 The script touches on the concept of memory allocation for strings and the potential risks of overwriting memory.
- 🛠️ It provides an example of how to enter a string using 'scanf' and 'gets' functions and the associated risks.
- 📚 The video mentions the importance of understanding computer memory when working with strings to avoid unsafe practices.
- ✅ Finally, it suggests that using 'fgets' is a safer alternative to 'gets' and hints at covering more about 'fgets' in a separate video.
Q & A
What is the main topic of the video script?
-The main topic of the video script is discussing strings in C programming, including how to declare, initialize, and read strings at runtime.
What is the difference between character array and string as mentioned in the script?
-The script explains that a character array is just an array of characters, while a string is a character array that is null-terminated, indicating the end of the string.
How does the script define a string in C?
-In the script, a string is defined as a character array that is null-terminated, which means it ends with a null character ('\0').
What is the purpose of the null character in a string?
-The null character ('\0') in a string serves as an indicator to signal the end of the string, which is important for functions that process strings.
What is the role of the 'scanf' function in reading strings as discussed in the script?
-The 'scanf' function is used to read strings at runtime. However, it's mentioned that 'scanf' should not be used with '%s' to read strings due to the risk of buffer overflow.
What is buffer overflow and why is it a concern in the script?
-Buffer overflow is a situation where more data is written to a block of memory, or buffer, than it can hold, which can lead to data corruption and security vulnerabilities. The script highlights this as a risk when using 'scanf' to read strings.
What alternative to 'scanf' is suggested in the script for reading strings?
-The script suggests using 'fgets' as an alternative to 'scanf' for reading strings, as it is safer and can prevent buffer overflow.
How does the script explain the use of 'fgets' to read strings?
-The script explains that 'fgets' can be used to read an entire line, including spaces, until a newline character is encountered, making it a safer choice for string input.
What is the significance of the 'gets' function mentioned in the script?
-The 'gets' function is mentioned as a deprecated function that was used to read strings. It is highlighted as unsafe due to its potential to cause buffer overflow and is no longer recommended for use.
How should one declare a string in C according to the script?
-The script suggests declaring a string in C by specifying the type as 'char' and initializing it with a set of characters enclosed in double quotes, followed by a null character at the end.
What is the importance of understanding memory allocation when working with strings in C?
-Understanding memory allocation is crucial when working with strings in C to avoid issues like buffer overflow and to ensure that the correct amount of memory is allocated for the string data.
Outlines
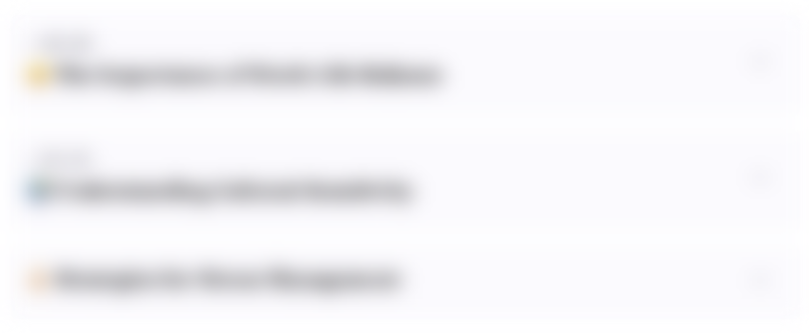
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
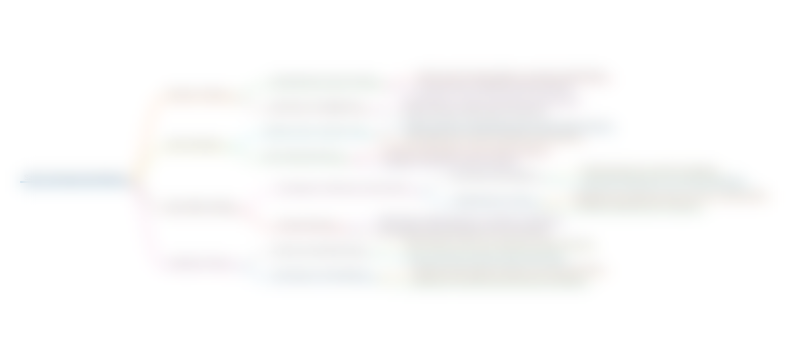
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
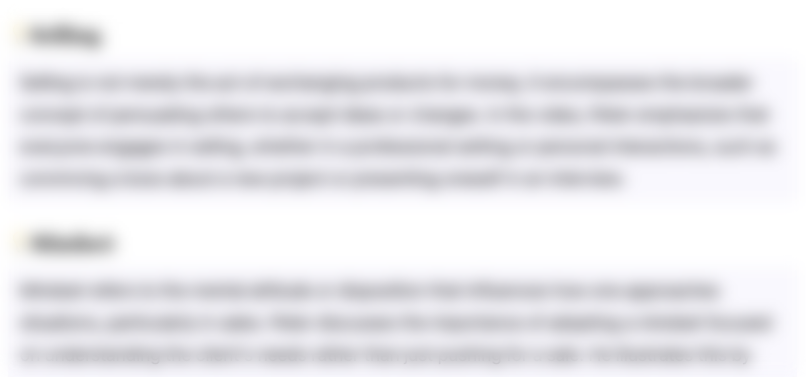
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
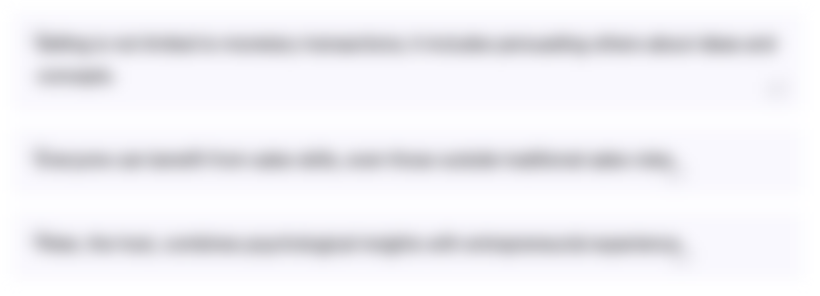
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
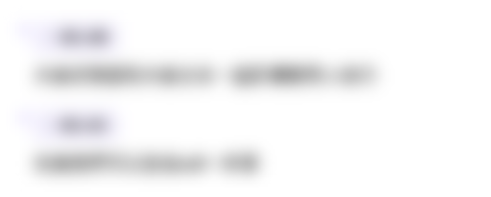
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)