Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3)
Summary
TLDRThis video introduces the fundamental concepts of classes and objects in programming. Using a concrete example of robots with varying attributes like name, color, and weight, the video explains how classes serve as blueprints for creating objects and organizing properties and functionalities. It also covers methods, instance variables, and the purpose of constructors in simplifying object creation. The video uses Java code samples to demonstrate object creation and manipulation, and it promises further content on data structures and object interactions in upcoming videos, with a focus on making these concepts accessible for learners across various programming languages.
Takeaways
- ๐ Classes and objects are fundamental concepts in data structures and algorithms, applicable in various programming languages.
- ๐ A class acts as a blueprint, defining the structure (properties) and behavior (methods) of objects.
- ๐ An object is an instance of a class, containing specific data (attributes) and functionality (methods).
- ๐ Attributes (or instance variables) represent an object's properties, such as 'name', 'color', and 'weight'.
- ๐ Methods are functions defined within an object that allow it to perform actions, such as 'introduceSelf()'.
- ๐ You can use classes to define how objects should behave, for example, creating robots with different attributes like name and color.
- ๐ The 'this' keyword in object-oriented programming refers to the current object, helping distinguish between object attributes and method arguments.
- ๐ Classes and objects help organize and manage data efficiently, allowing for easy creation and manipulation of similar objects.
- ๐ Constructors are special methods that allow objects to be created and initialized with predefined values, simplifying the object creation process.
- ๐ Java uses constructors to initialize object attributes and can use both default and custom constructors depending on the requirements.
- ๐ The video also introduces how classes and objects can interact with each other, providing a foundation for building more complex data structures like linked lists.
Q & A
What is the main topic discussed in the video?
-The video discusses the fundamentals of classes and objects in programming, along with attributes, methods, constructors, and how they relate to creating objects in Java, though the concepts are applicable to various programming languages.
How does the presenter explain the concept of classes and objects?
-The presenter uses a concrete example of robots on a website to explain classes and objects, showing how each robot (like Tom and Jerry) has properties such as name, color, and weight, and methods like introduceSelf() to demonstrate their behavior.
What is the purpose of a class in object-oriented programming?
-A class serves as a blueprint for creating objects. It defines the properties (attributes) and methods (functions) that the objects created from the class will have, but does not hold any specific data until instantiated.
What is the role of an object in object-oriented programming?
-An object is an instance of a class that contains specific data (attributes) and can perform functions (methods). For example, Tom and Jerry are objects created from the 'Robot' class with unique attributes like name, color, and weight.
What are attributes and methods in the context of objects?
-Attributes (also called instance variables) are the properties of an object, such as 'name', 'color', and 'weight'. Methods are the functions associated with an object that define its behavior, such as 'introduceSelf()' in the robot example.
What is the difference between a class and an object?
-A class is a blueprint for creating objects, specifying the attributes and methods they will have. An object is a specific instance created from that class, holding actual data for the attributes and being able to perform the methods.
What is the significance of the 'introduceSelf()' method in the robot example?
-The 'introduceSelf()' method demonstrates how an object can perform an action. In the robot example, it prints a message introducing the robot by name, with each robot object (Tom and Jerry) calling this method to show their unique name.
How does the presenter define a constructor and its purpose?
-A constructor is a special function used to initialize an object when it is created. It can be used to set default values for an object's attributes. The presenter demonstrates using both a default constructor and a custom constructor to set attributes during object creation.
What is the difference between the default constructor and a custom constructor?
-The default constructor is automatically provided by the programming language and doesn't initialize the object's attributes. A custom constructor, defined by the programmer, allows for setting specific values for an object's attributes when the object is created.
How does the 'this' keyword work in the context of the constructor?
-'This' refers to the specific object that the constructor is acting on. In the custom constructor example, 'this.name' refers to the name attribute of the object being created, while 'name' refers to the parameter passed to the constructor.
Outlines
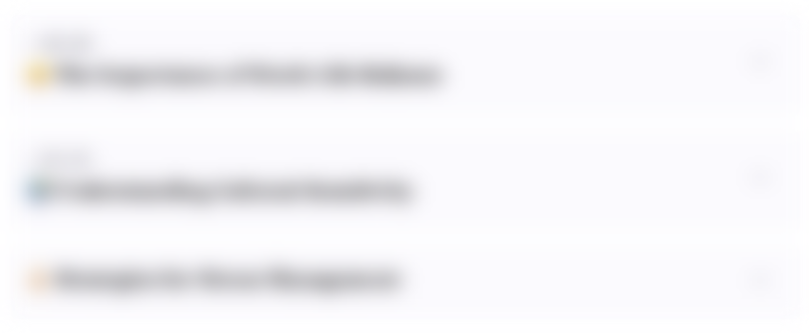
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
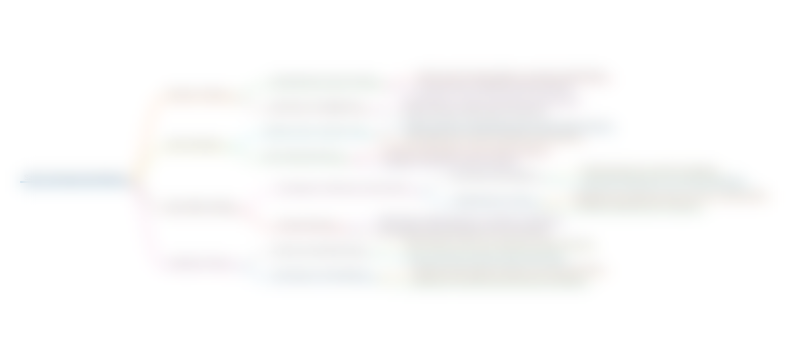
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
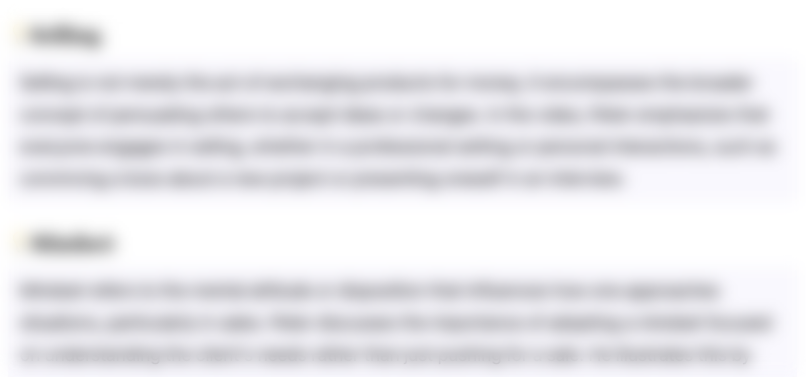
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
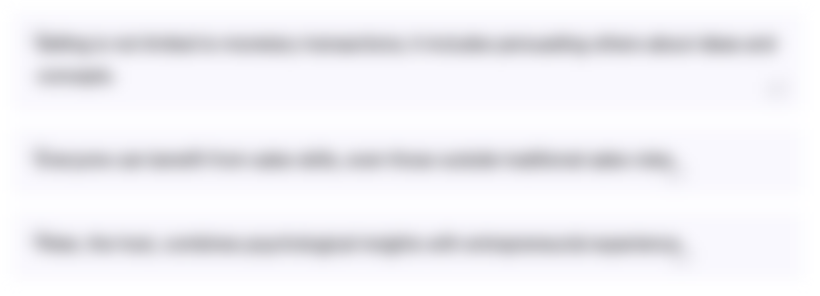
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
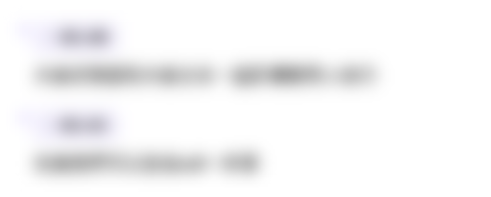
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
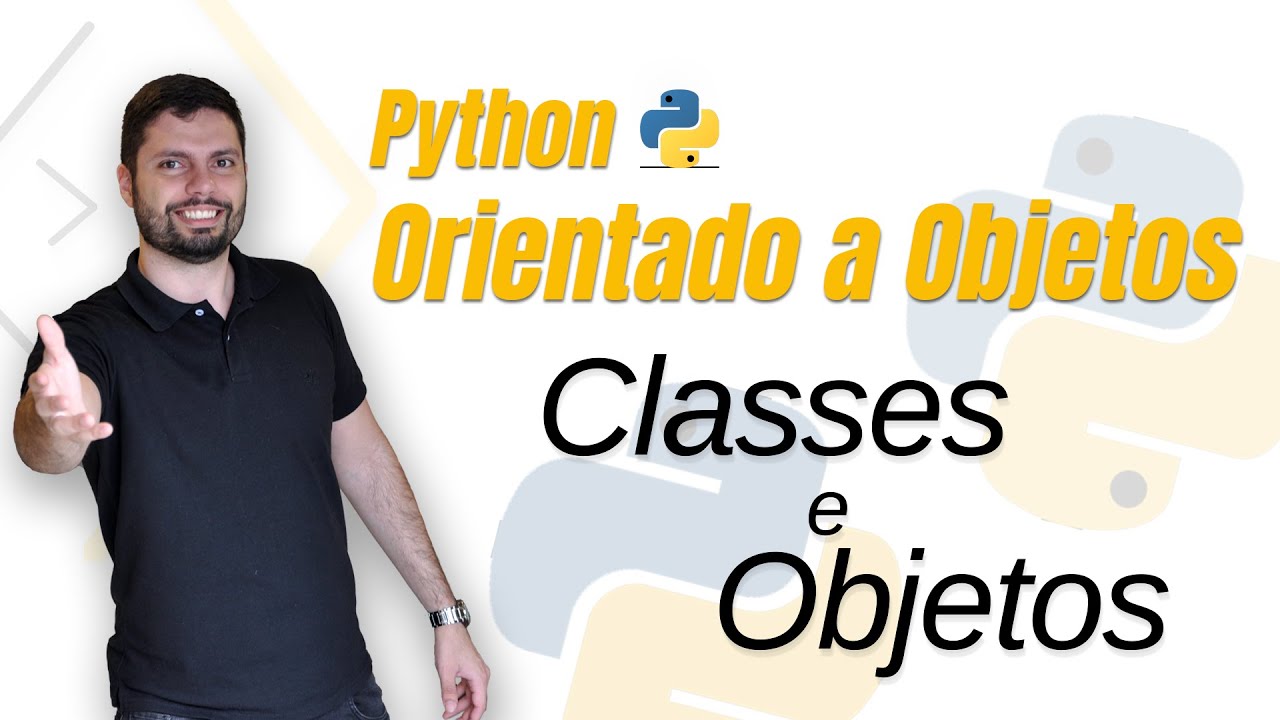
Como criar Classes e Objetos - Curso Python Orientado a Objetos [Passo a Passo]
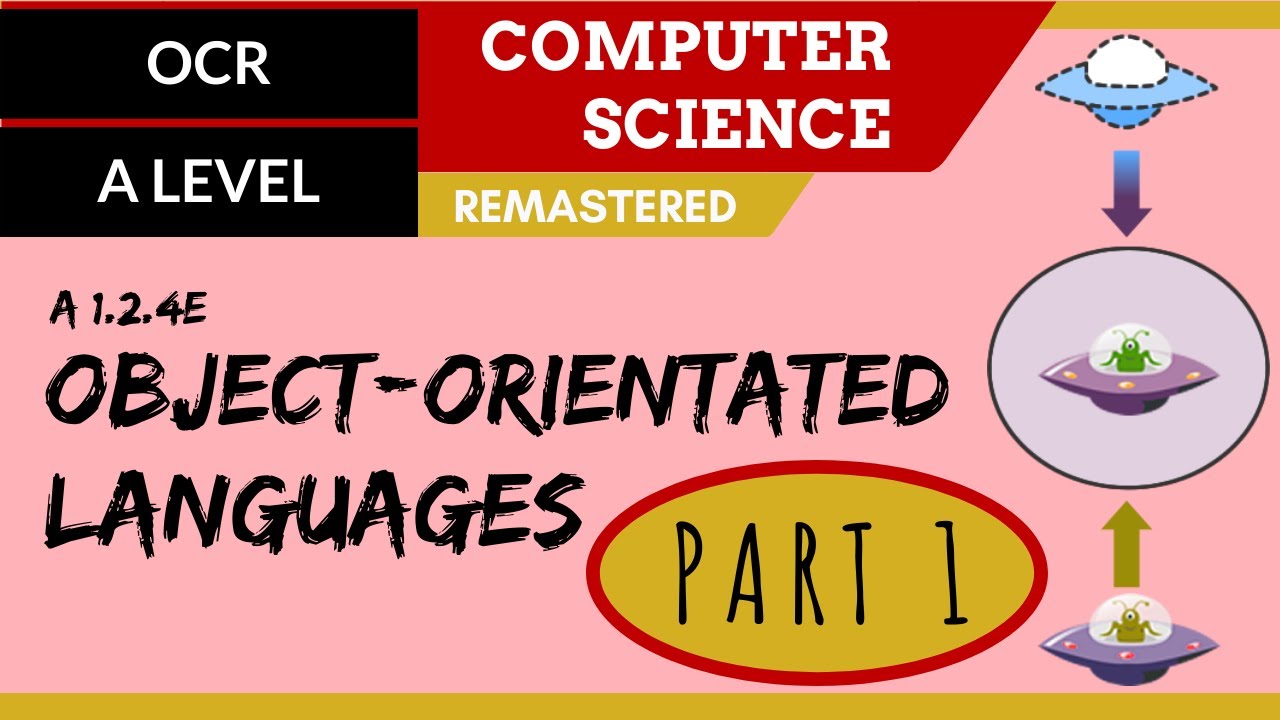
36. OCR A Level (H446) SLR7 - 1.2 Object-oriented languages part 1

Classes and Objects in Python | OOP in Python | Python for Beginners #lec85
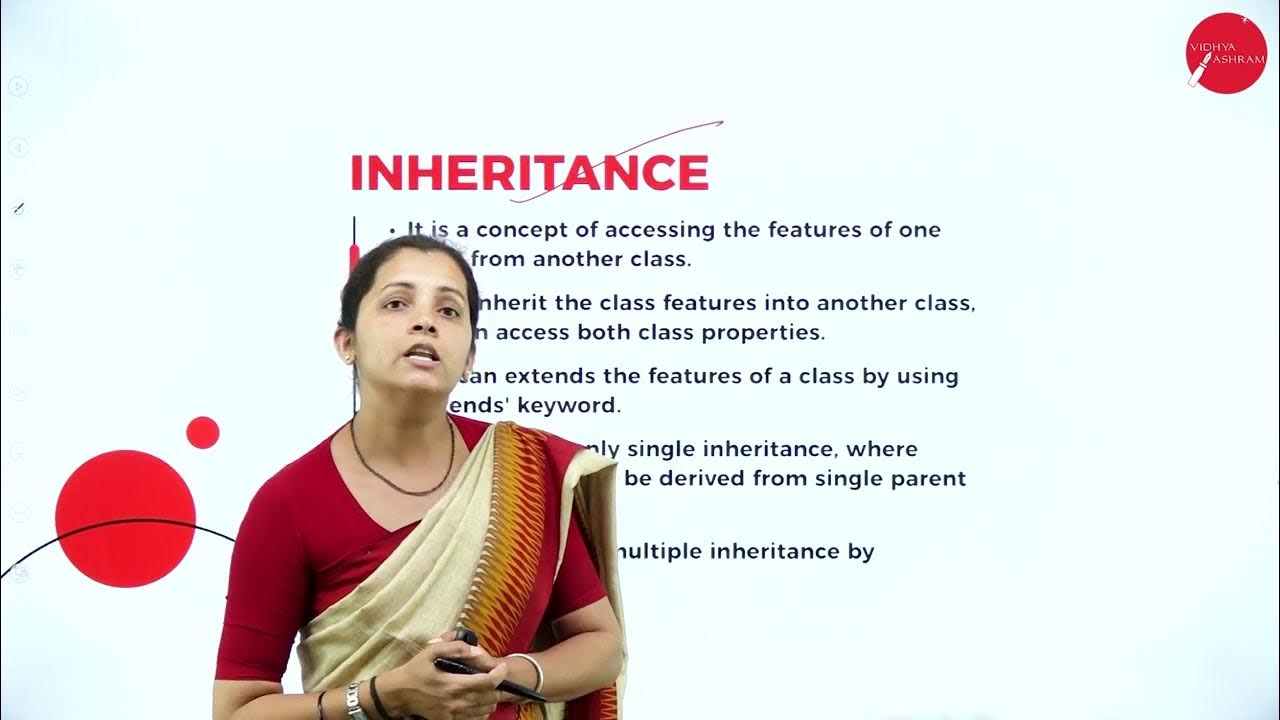
DAY 07 | PHP AND MYSQL | VI SEM | B.CA | CLASS AND OBJECTS IN PHP | L1
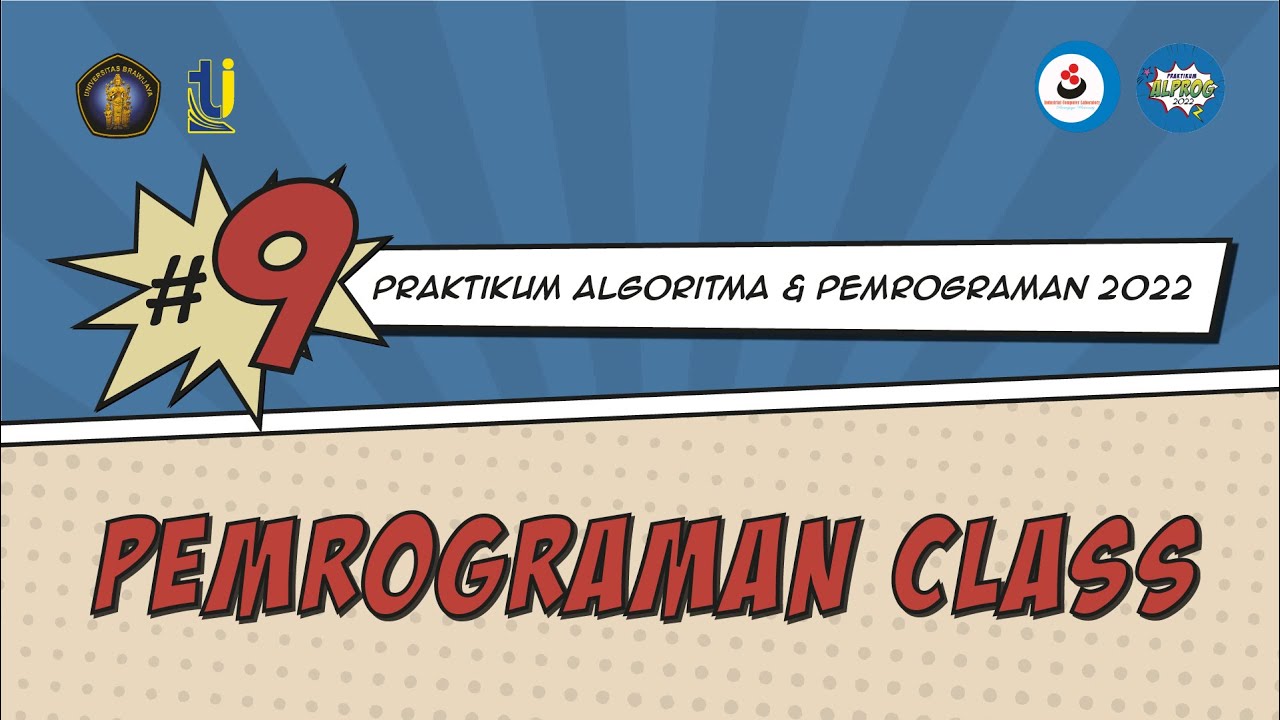
9. Pemrograman Class - Praktikum Algoritma dan Pemrograman 2022

Introduction to OOPs in Python | Python Tutorial - Day #56
5.0 / 5 (0 votes)