Object Oriented Programming - The Four Pillars of OOP
Summary
TLDRIn this video, the presenter delves into the four pillars of object-oriented programming (OOP): abstraction, inheritance, polymorphism, and encapsulation. Using examples like a computer monitor and a video game enemy, the video demonstrates how OOP allows for code reusability, simplifying complex systems. The presenter explains how abstraction hides unnecessary details, inheritance promotes code reuse, polymorphism allows for flexible method usage at runtime, and encapsulation ensures data safety. Through practical code examples, viewers gain an in-depth understanding of these essential concepts, with a focus on real-world application in software development.
Takeaways
- 😀 Object-Oriented Programming (OOP) is a programming paradigm focused on objects that contain both data and methods.
- 😀 The four pillars of OOP are Abstraction, Polymorphism, Inheritance, and Encapsulation, which are key principles for effective object-oriented design.
- 😀 Abstraction allows users to interact with an object without needing to know its internal details, promoting simplicity and separation of concerns.
- 😀 Inheritance enables code reuse by allowing new classes to inherit attributes and methods from existing classes.
- 😀 Polymorphism enables objects to use methods in different ways, with the correct method being executed based on the object type at runtime.
- 😀 Encapsulation involves hiding internal object details and providing controlled access to properties and methods through getters and setters.
- 😀 An example of abstraction was demonstrated with a computer monitor object, where users only interact with high-level functions like turning the monitor on/off.
- 😀 Inheritance was explained using an 'enemy' class and a 'vampire' subclass that inherited behaviors from the parent class without needing to rewrite code.
- 😀 Polymorphism was illustrated by having different subclasses (vampire and werewolf) override the 'talk' method, which was dynamically called based on the object type at runtime.
- 😀 Encapsulation was demonstrated by making object properties private and using getters and setters to control access to these properties, promoting better safety and structure in the code.
Q & A
What is the main concept behind object-oriented programming (OOP)?
-Object-oriented programming (OOP) is a programming paradigm based on the concept of objects, which contain data in the form of attributes or properties and actions in the form of methods or functions.
What does the acronym 'PIE' stand for in OOP, and how is it related to its pillars?
-The acronym 'PIE' stands for Abstraction, Polymorphism, Inheritance, and Encapsulation. These are the four pillars of object-oriented programming, and they work together to define how objects and their behaviors are structured in OOP.
What is the purpose of abstraction in OOP?
-Abstraction in OOP refers to the idea of exposing only the necessary details of an object to the user, hiding the inner workings and implementation. This simplifies user interaction and decouples the user from the complex underlying logic.
How does inheritance help in object-oriented programming?
-Inheritance allows code reusability in OOP by enabling a new class (child or subclass) to inherit attributes and methods from an existing class (parent or superclass), allowing for extension and modification without rewriting the original code.
Can you explain how a subclass inherits a method from its superclass in OOP?
-A subclass inherits the methods of its superclass automatically, but it can override those methods if needed. For example, a subclass like 'Vampire' inherits the 'talk' method from its 'Enemy' superclass, but can provide its own implementation of the method.
What is polymorphism in OOP and why is it important?
-Polymorphism in OOP allows different objects to be treated as instances of the same class through a shared interface, but their specific behaviors are determined at runtime. This enables flexibility and simplifies the code by allowing one interface to work with different data types or objects.
How does polymorphism work when calling methods on objects of different classes?
-Polymorphism allows the same method name to behave differently depending on the object that invokes it. For example, calling the 'talk' method on an 'Enemy' pointer that points to a 'Vampire' object will execute the 'Vampire's' version of the method, even though the pointer type is 'Enemy'.
What is the purpose of encapsulation in OOP?
-Encapsulation in OOP focuses on hiding an object's internal state and restricting access to it. It ensures that an object's attributes are protected from unintended modification, and that interaction with the object is done through well-defined methods.
What are getter and setter methods, and why are they used in OOP?
-Getter and setter methods provide controlled access to private attributes of an object. A getter retrieves the value of an attribute, while a setter allows modifying it. These methods ensure that attributes are not directly altered, maintaining the integrity and safety of the object.
Why is OOP an important concept for software engineers to grasp?
-OOP is an essential concept in software engineering because it promotes code reuse, maintainability, and scalability. It’s used in real-world applications, and many interview processes revolve around understanding OOP principles and design, making it a fundamental skill for developers.
Outlines
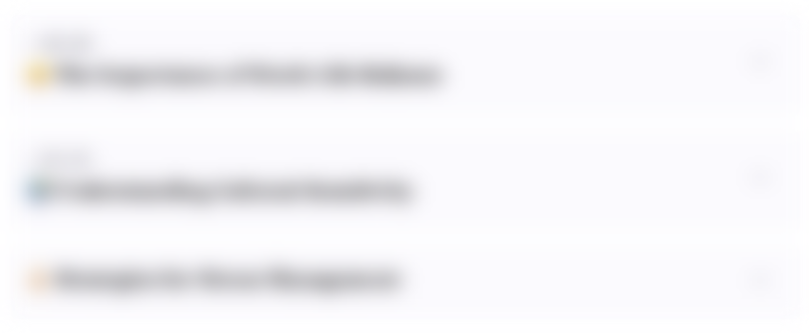
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
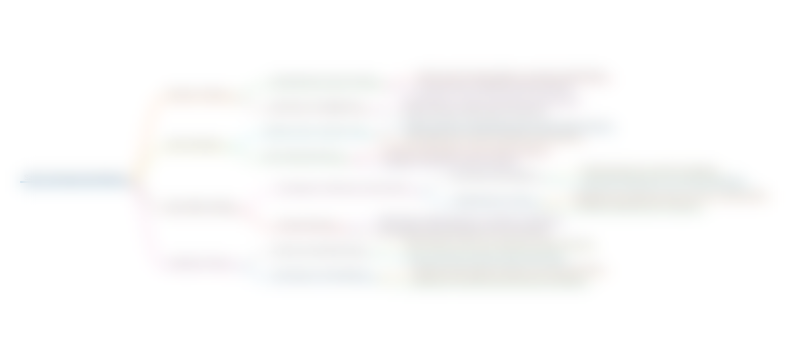
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
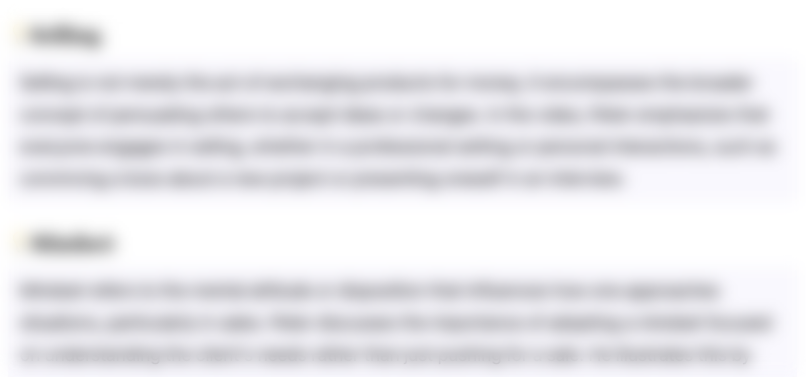
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
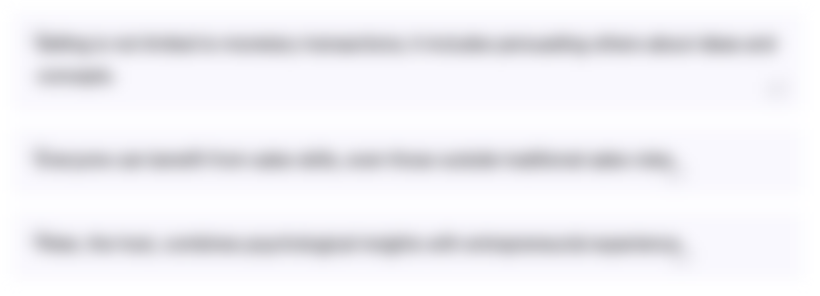
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
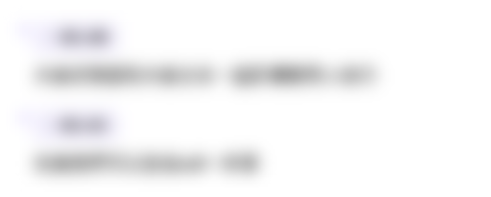
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
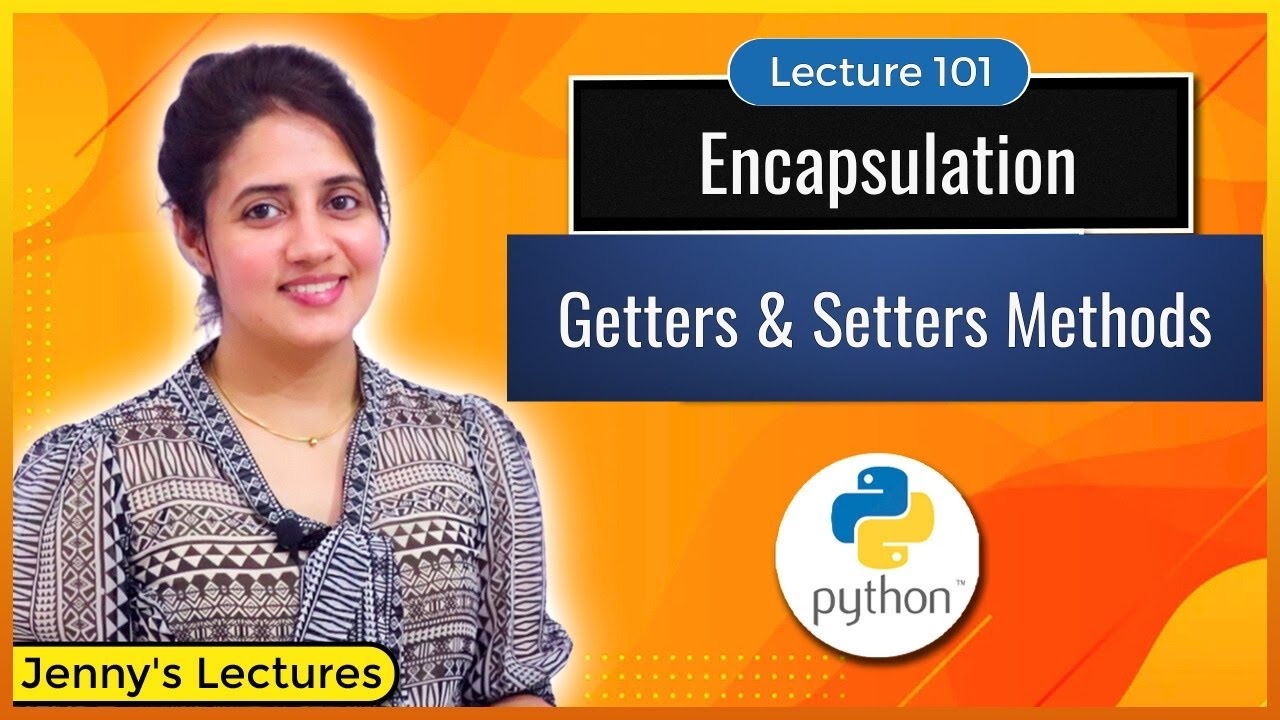
Encapsulation in Python | Getters & Setters methods | Python Tutorials for Beginners #lec101

#02 - Apa itu Enkapsulasi [Java OOP]

Fundamental Concepts of Object Oriented Programming
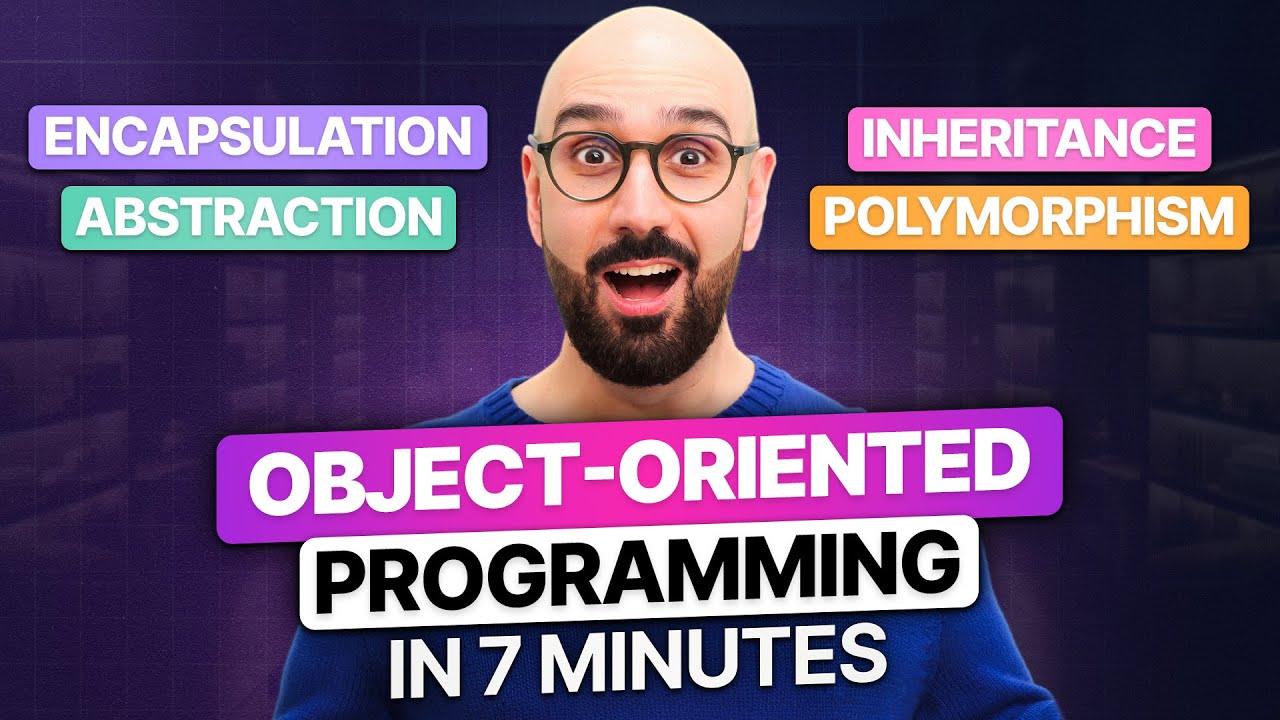
Object-oriented Programming in 7 minutes | Mosh
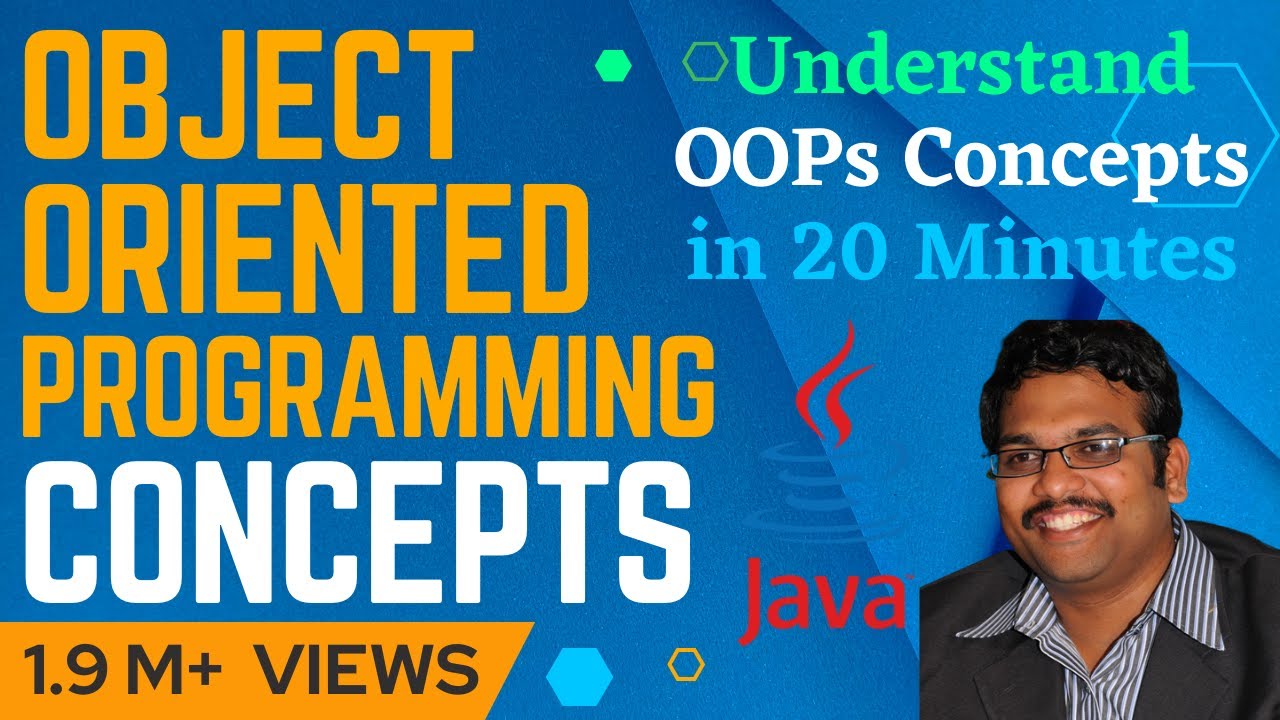
OOPS CONCEPTS - JAVA PROGRAMMING

Overview of Object-oriented programming in C# | C# object-oriented programming | C# oops
5.0 / 5 (0 votes)