First C Program - English
Summary
TLDRThis tutorial teaches beginners how to write, compile, and execute a simple C program using the Ubuntu OS and GCC compiler. It covers the basics, including opening a text editor, writing a simple program with comments, and using the `printf` function. The tutorial also emphasizes best practices, such as saving files frequently and handling common errors like missing semicolons and incorrect header file names. Through step-by-step guidance, the viewer learns how to troubleshoot errors and run their first C program successfully, with an emphasis on coding conventions and debugging techniques.
Takeaways
- π Learn how to write a simple C program using an editor like gedit.
- π Understand the purpose of the `#include <stdio.h>` directive for standard input/output functions.
- π The `main()` function marks the entry point of the program in C and must return an integer.
- π Use `printf()` to display messages on the terminal, with special characters like ` ` for new lines.
- π Every C statement must end with a semicolon `;` to signify the end of the statement.
- π Save your program frequently to avoid losing progress due to unexpected shutdowns or crashes.
- π Compile a C program using the `gcc` compiler with the `-o` flag to specify the output executable file name.
- π To execute a compiled program, use `./myoutput` to run the executable and see the output.
- π Avoid placing any code after the `return` statement in the `main()` function as it will not execute.
- π Common errors include missing semicolons or incorrect header file names (e.g., missing dot in `stdio.h`).
- π Debugging common C errors, such as missing semicolons or wrong function placement, is essential for learning.
Q & A
What is the main purpose of this tutorial?
-The main purpose of this tutorial is to teach how to write, compile, and execute a simple C program on an Ubuntu operating system using the GCC compiler. It also covers basic C programming concepts and common errors.
Why is 'stdio.h' included in a C program?
-'stdio.h' is a standard library in C that includes functions for input and output, such as `printf` and `scanf`. It must be included to allow the program to use these functions.
What does the 'main' function do in a C program?
-The 'main' function is the entry point of a C program. It is where the execution of the program begins, and it must be present in every C program. The return type 'int' indicates that it returns an integer value.
What is the significance of the 'return 0;' statement in the main function?
-'return 0;' signifies the successful completion of the program. The integer value returned by the 'main' function indicates the program's exit status, where 0 generally means successful execution.
Why is indentation important in C programming?
-Indentation improves the readability of the code, making it easier to understand the structure and flow of the program. It also helps in quickly identifying errors in the program.
What does the '\n' symbol do in the 'printf' function?
-The '\n' symbol is an escape sequence that represents a new line. It moves the cursor to the next line after printing the text in the terminal.
What happens if the 'return 0;' statement is placed after another 'printf' statement?
-Once the 'return 0;' statement is executed, the program exits and no further code is executed. Therefore, if another 'printf' statement is placed after 'return 0;', it will not be executed.
What is the role of the semicolon in a C program?
-A semicolon in C marks the end of a statement. Every C statement must end with a semicolon, except for control structures and functions.
What is the effect of missing the dot in 'stdio.h'?
-If the dot is missing in 'stdio.h' (e.g., writing 'stdioh'), the compiler will not recognize the file, resulting in an error: 'fatal error: stdioh: No such file or directory'.
What should you do if the compiler gives an error about a missing semicolon?
-If a semicolon is missing, the compiler will give an error indicating the absence of the semicolon. To fix it, simply add a semicolon at the end of the statement where it is missing.
Outlines
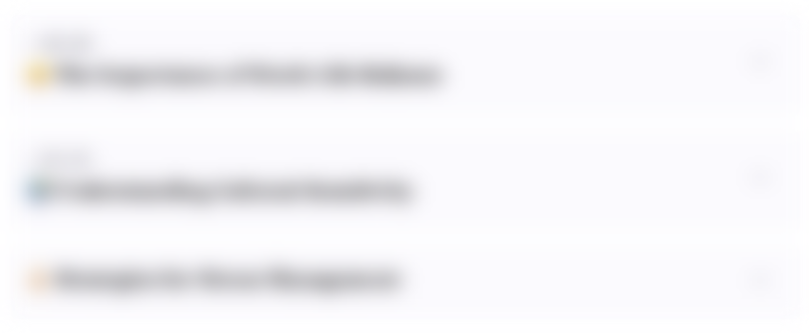
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
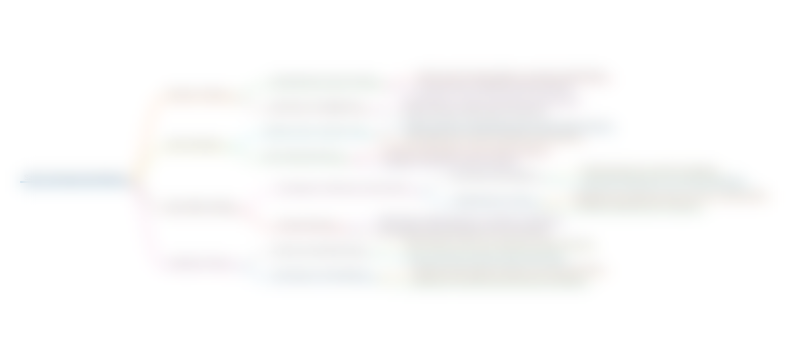
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
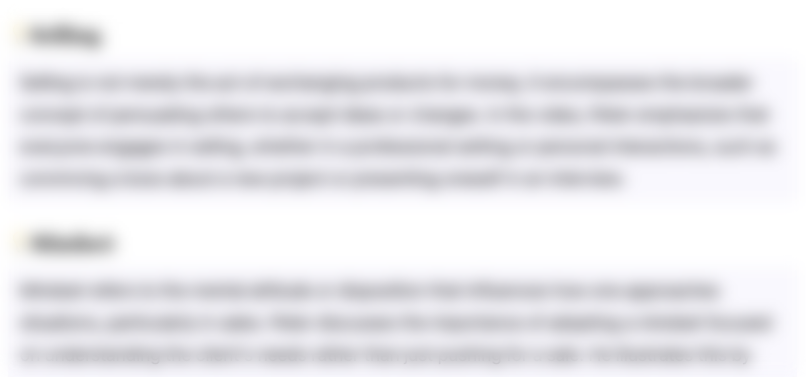
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
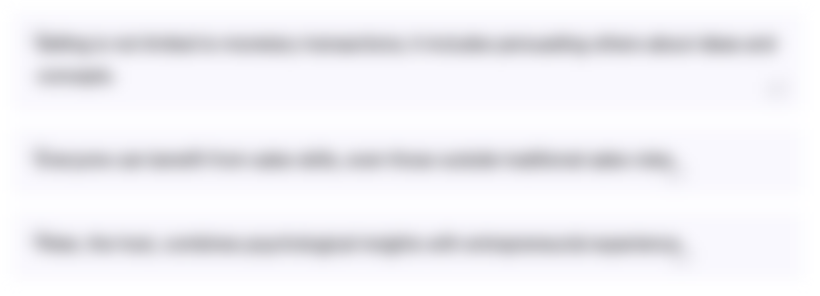
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
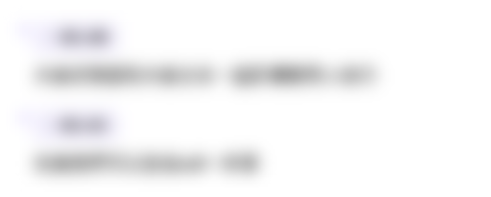
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
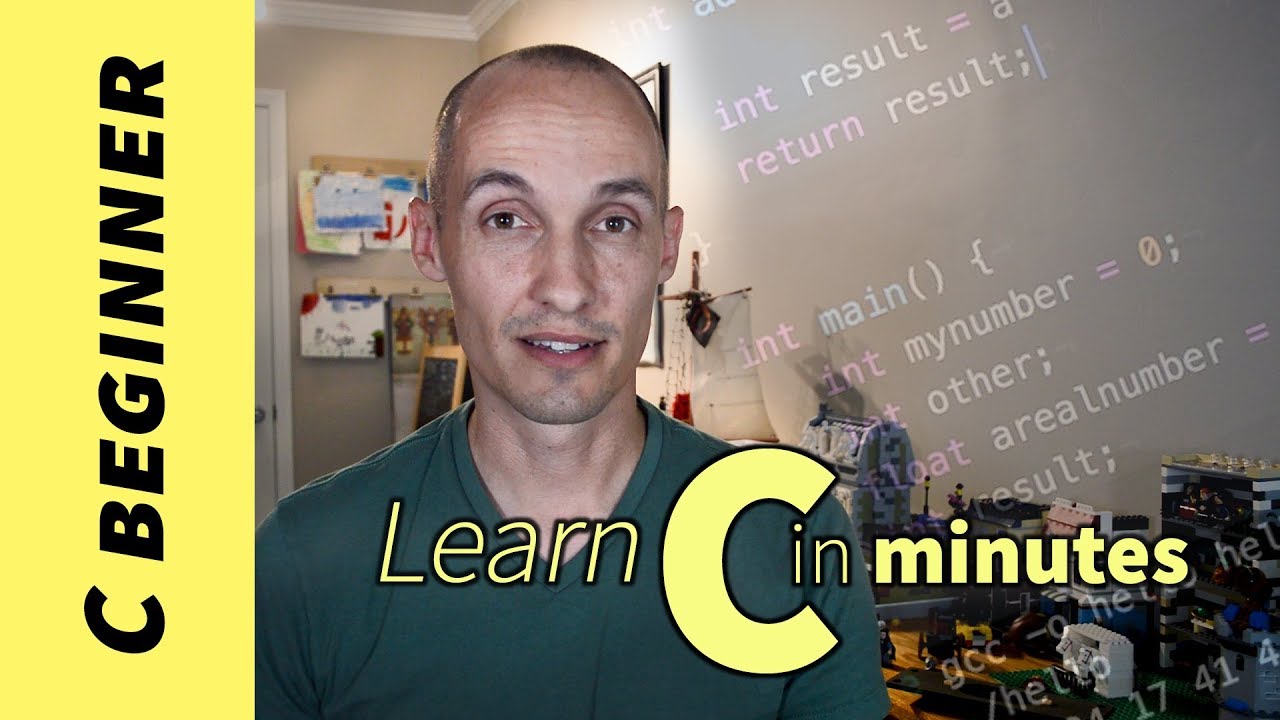
Learn C in minutes (lesson 0)

How to Run first C++ program on TurboC/C++ (Updated 2021)
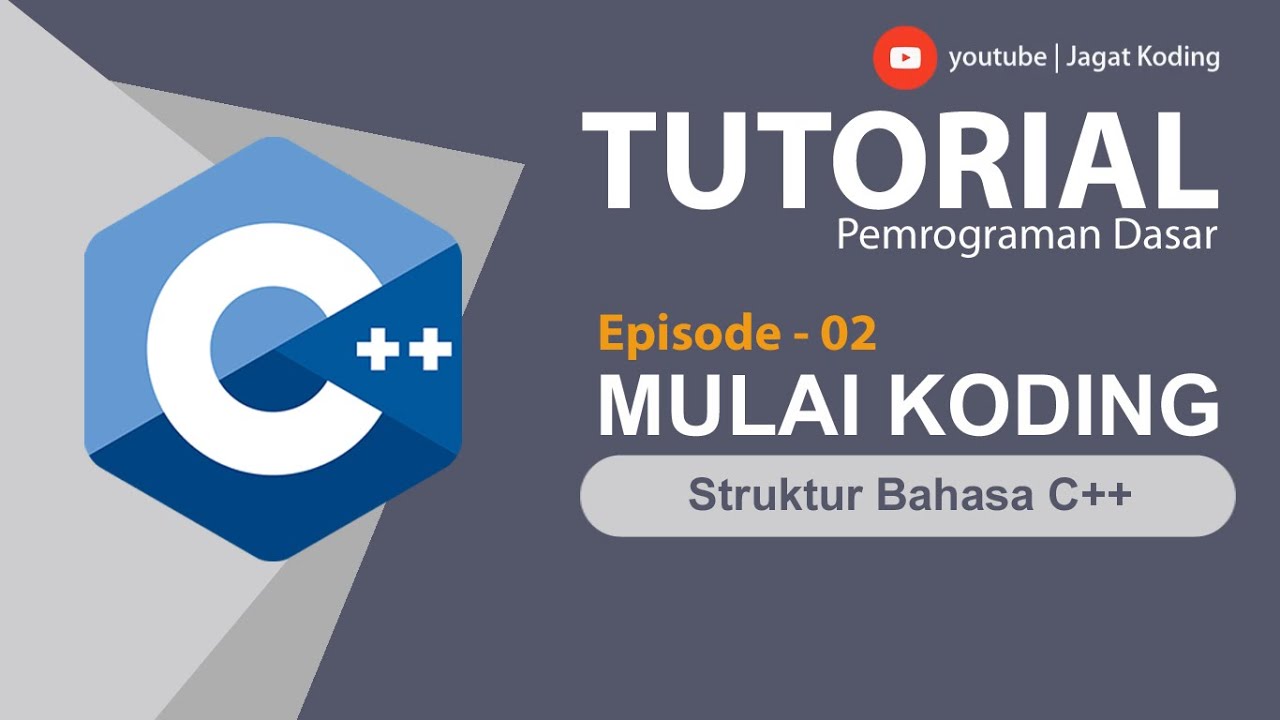
C++ 02 | Belajar Struktur Bahasa Pemrograman C++ | Tutorial Dev C++ Indonesia
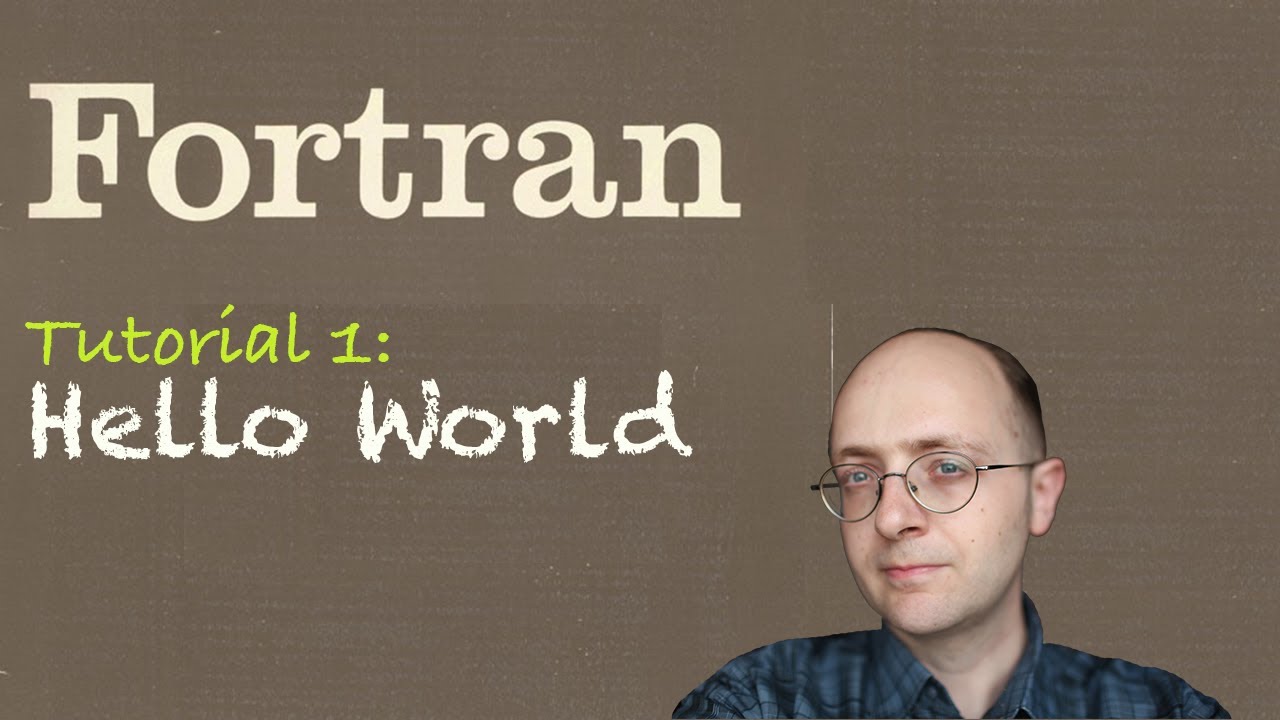
[Fortran Tuto 1] Hello World !
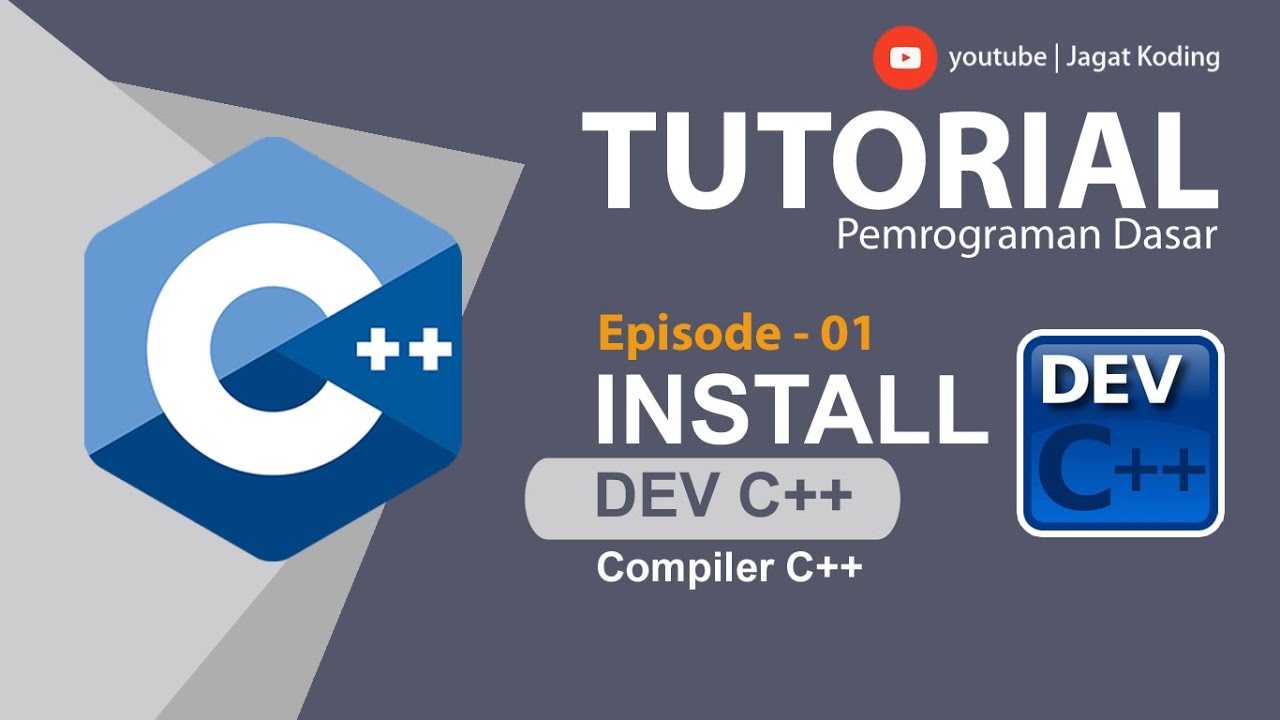
C++ 01 | Cara Instalasi Dev C++ | Tutorial Dev C++ Indonesia
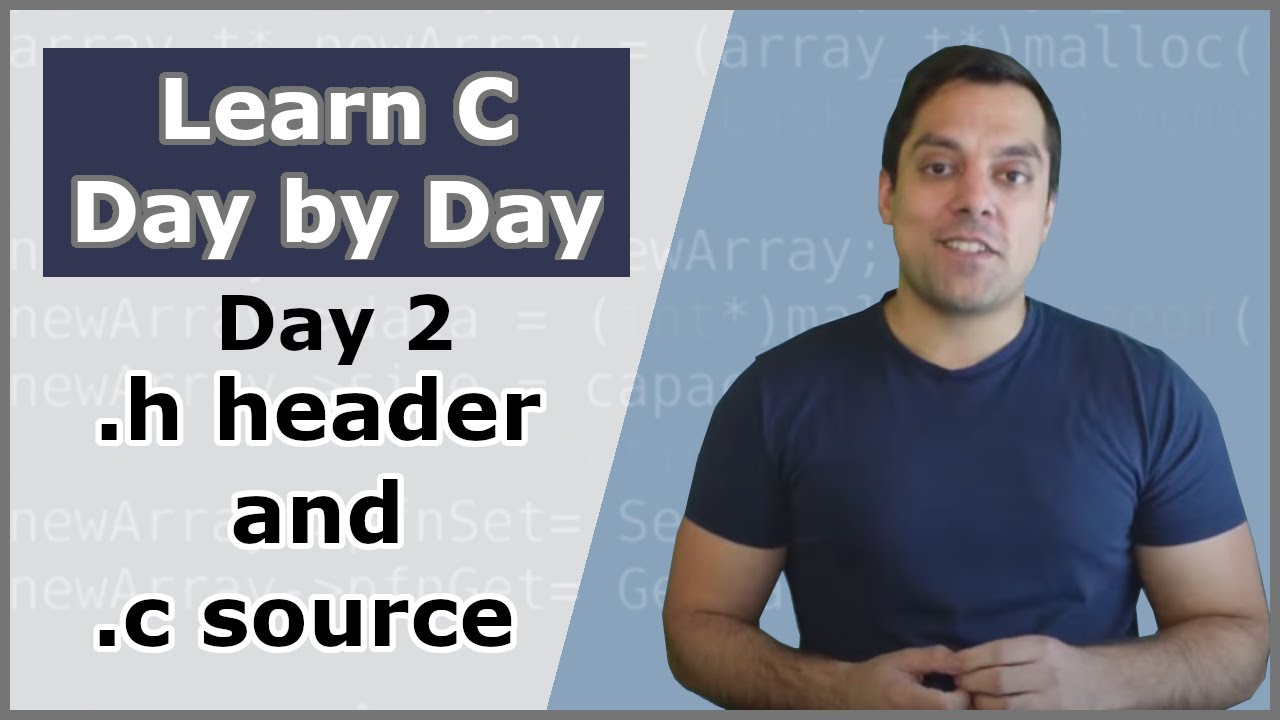
Your Second Day in C (Understand .h header and .c source files) - Crash Course in C Programming
5.0 / 5 (0 votes)