Linguagem C - Aula 3.2 - Aprenda a realizar cálculos em C (2022)
Summary
TLDRIn this programming lesson, the focus is on understanding variables, arithmetic operations, and assignment operators in C. The instructor explains key concepts like variable declaration, types of data (integers, floats, doubles, and text), and how arithmetic operators such as addition, subtraction, multiplication, and division work. Emphasis is placed on operator precedence and the importance of parentheses. The video also covers the use of assignment operators, including increment and decrement, and demonstrates practical examples to reinforce these concepts. The lesson concludes with an encouragement to master these fundamental concepts before progressing to more advanced topics like flow control and conditionals.
Takeaways
- 😀 Variables in C are used to store data, allowing for manipulation and calculation within a program.
- 😀 Data types in C include: 'int' for integers, 'float' for decimals, and 'double' for higher precision floating-point numbers.
- 😀 Text data in C is handled using 'char[]', where the number in brackets indicates the maximum length of the string.
- 😀 Arithmetic operators in C include: addition (+), subtraction (-), multiplication (*), division (/), and modulus (%).
- 😀 Operator precedence in C follows mathematical rules: multiplication and division have higher precedence than addition and subtraction.
- 😀 Parentheses in C force operations within them to be evaluated first, regardless of operator precedence.
- 😀 Assignment operators in C can combine arithmetic operations with assignment, such as +=, -=, *=, and /=.
- 😀 The increment (++) and decrement (--) operators increase or decrease a variable's value by 1, respectively.
- 😀 Using assignment operators like '+=', '+=3' will increase a variable’s value by 3, while '-=' will decrease it by a specific value.
- 😀 In the example program, user input is read, arithmetic operations are performed, and results are printed on the screen, demonstrating how variables interact dynamically.
- 😀 Future lessons will cover conditional statements (if), and it's essential to fully grasp variables and arithmetic operations before moving forward.
Q & A
What are the main types of variables covered in this script?
-The script covers three main types of variables: `int` for integers, `float` for decimal numbers, and `double` for double precision floating-point numbers. It also briefly mentions `char[]` for text, noting that the size needs to be specified, like `char name[50]`.
What is the importance of knowing variable types when programming?
-Knowing variable types is crucial because it determines the kind of data a variable can store and how much memory it will occupy. This affects how we manipulate data, perform operations, and ensure the program runs efficiently.
What does the script say about the complexity of working with text (string) variables?
-The script mentions that working with text variables in C can be more complicated than handling numeric types due to the need to define the size of the array (e.g., `char name[50]`), making text handling more tedious compared to higher-level languages.
What is operator precedence, and why is it important in arithmetic expressions?
-Operator precedence determines the order in which operations are performed. For example, multiplication and division have higher precedence than addition and subtraction. This is important because it affects the outcome of complex expressions. Operations inside parentheses are always performed first.
What is the purpose of the modulo operator (%) in C?
-The modulo operator (%) is used to find the remainder of a division between two integers. For example, `5 % 2` would return `1`, as 5 divided by 2 gives a remainder of 1.
Can we use the word 'soma' as a variable name in C? Why or why not?
-Yes, 'soma' can be used as a variable name in C because it is not a reserved keyword in the language. C allows using most words, except for reserved words like `int`, `float`, `if`, etc.
What are some examples of compound assignment operators, and how do they work?
-Examples of compound assignment operators include `+=`, `-=`, `*=`, and `/=`. These operators combine an arithmetic operation with assignment. For example, `a += 5` means `a = a + 5`. Similarly, `a *= 3` means `a = a * 3`.
How does the script demonstrate the use of increment and decrement operators?
-The script demonstrates increment (`++`) and decrement (`--`) operators by first initializing a variable and then using `++` to increase its value by 1, and `--` to decrease it by 1. These operators modify the value of the variable in place.
What is the difference between `a++` and `++a` in C?
-Both `a++` and `++a` increment the value of `a` by 1, but the difference lies in when the increment occurs. In `a++`, the value of `a` is used first (post-increment), then incremented. In `++a`, the value is incremented first (pre-increment) before being used.
Why is it important to understand operators like `+=` and `*=` before moving on to more advanced topics like loops?
-Understanding these operators is important because they simplify code and are frequently used in loops and conditional expressions. If you do not master these basic operations, you may struggle with more complex concepts like loops, which rely on manipulating variables efficiently.
Outlines
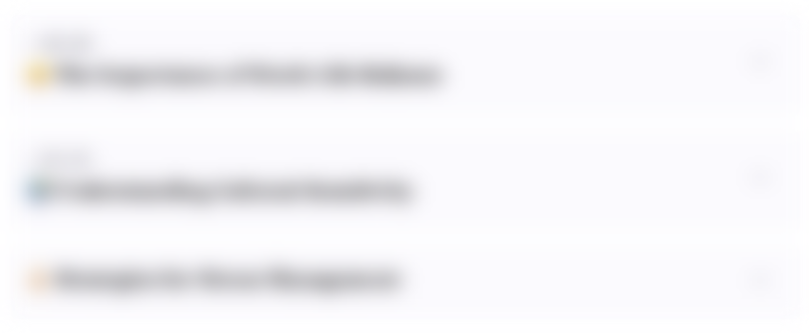
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
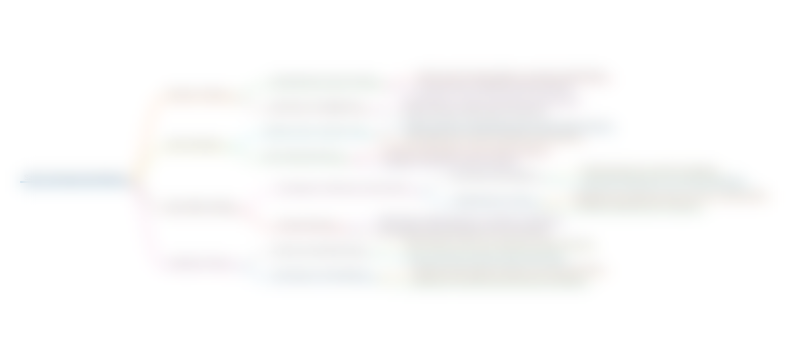
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
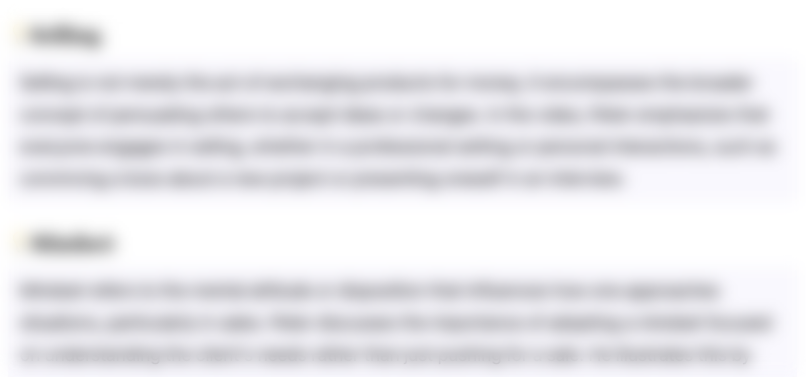
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
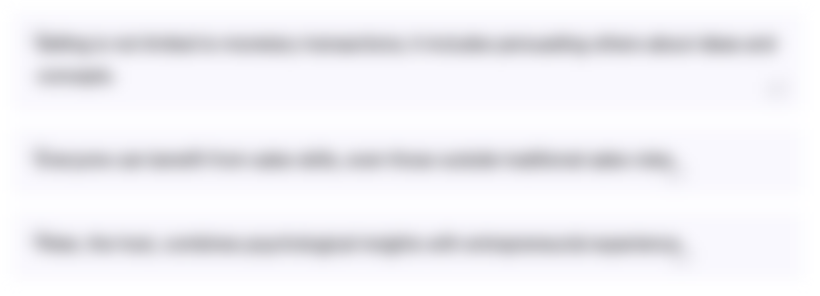
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
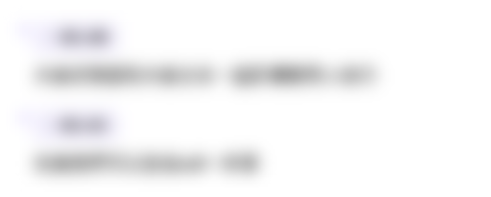
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
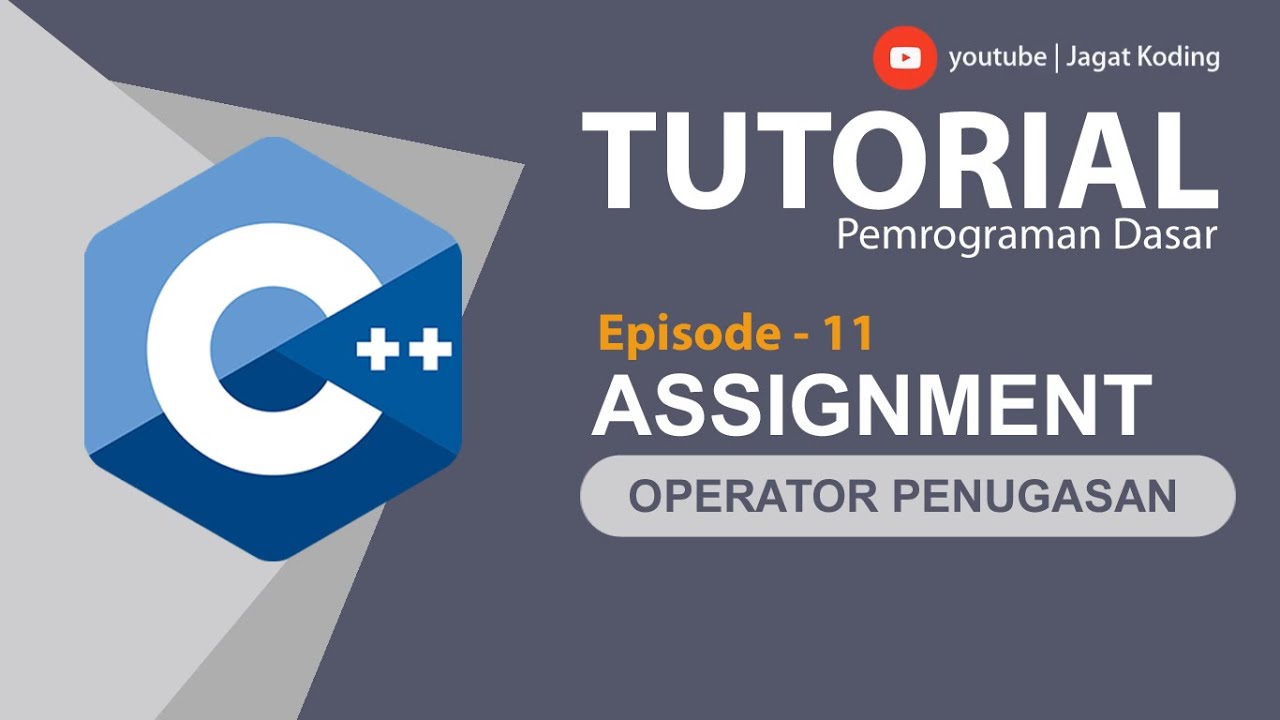
C++ 11 | Operator Assignment | Tutorial Pemrograman C++
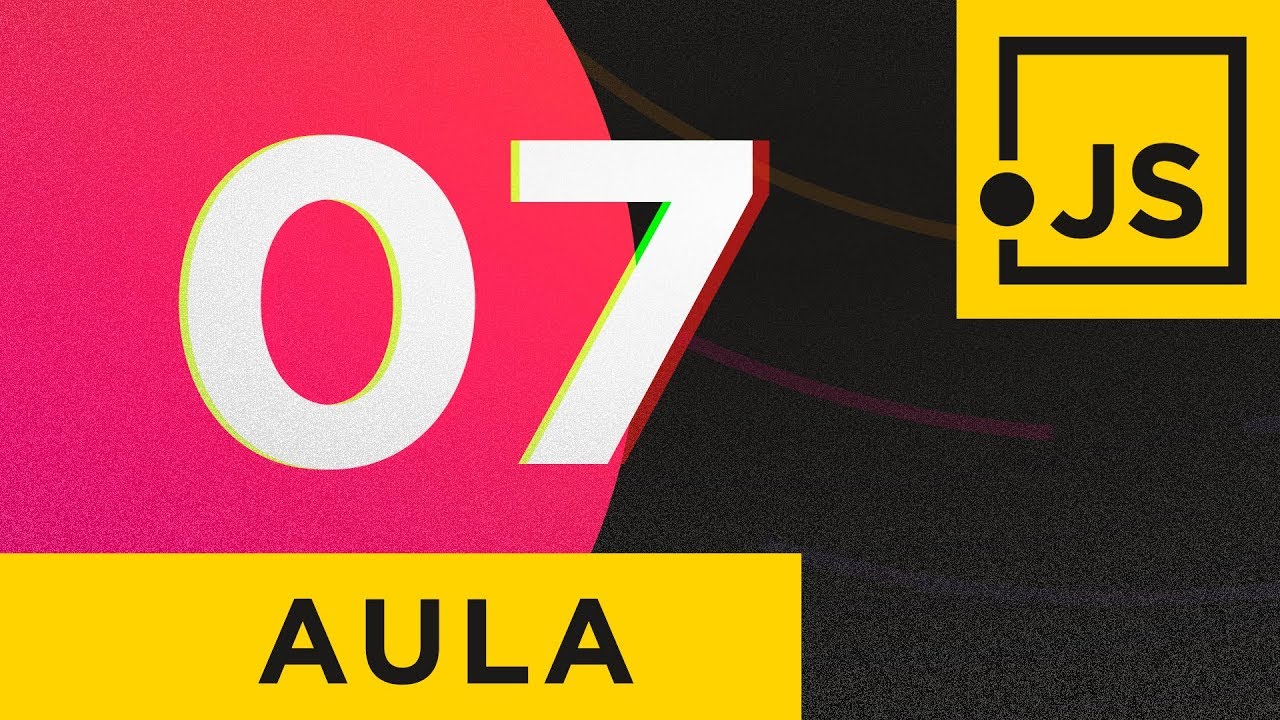
Operators (Part1) - JavaScript Course #07
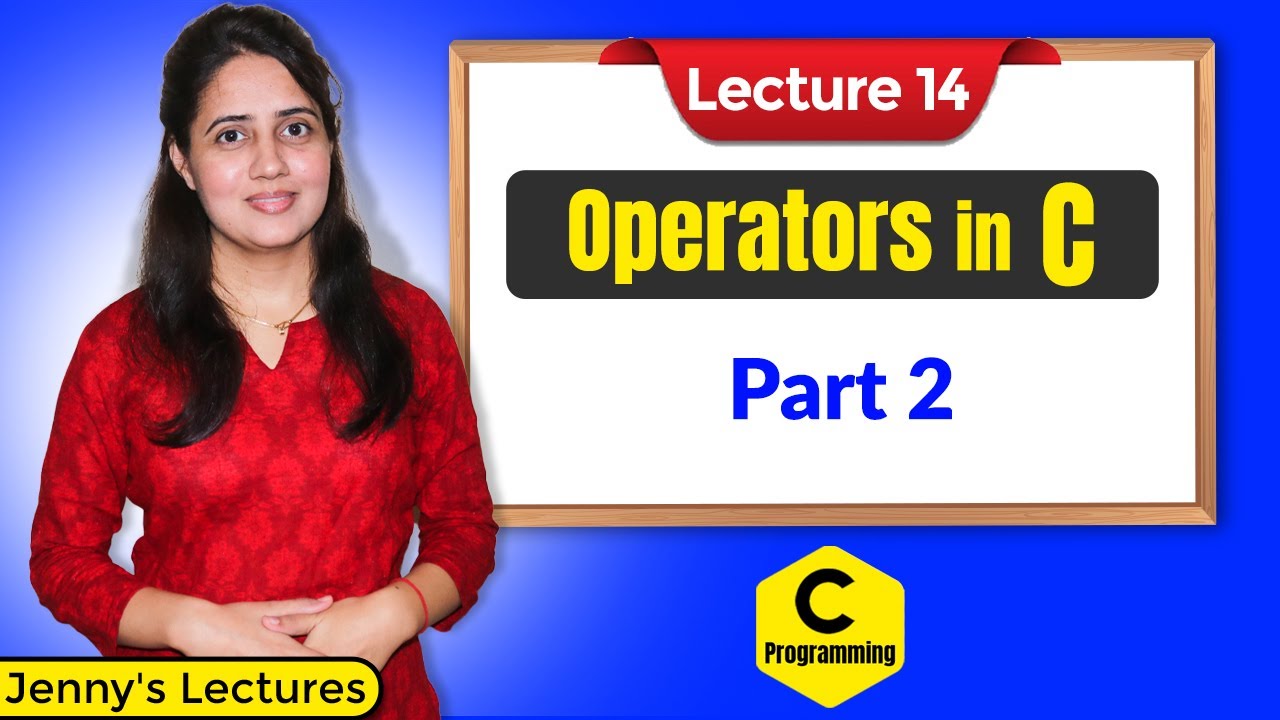
C_14 Operators in C - Part 2 | Arithmetic & Assignment Operators | C Programming Tutorials
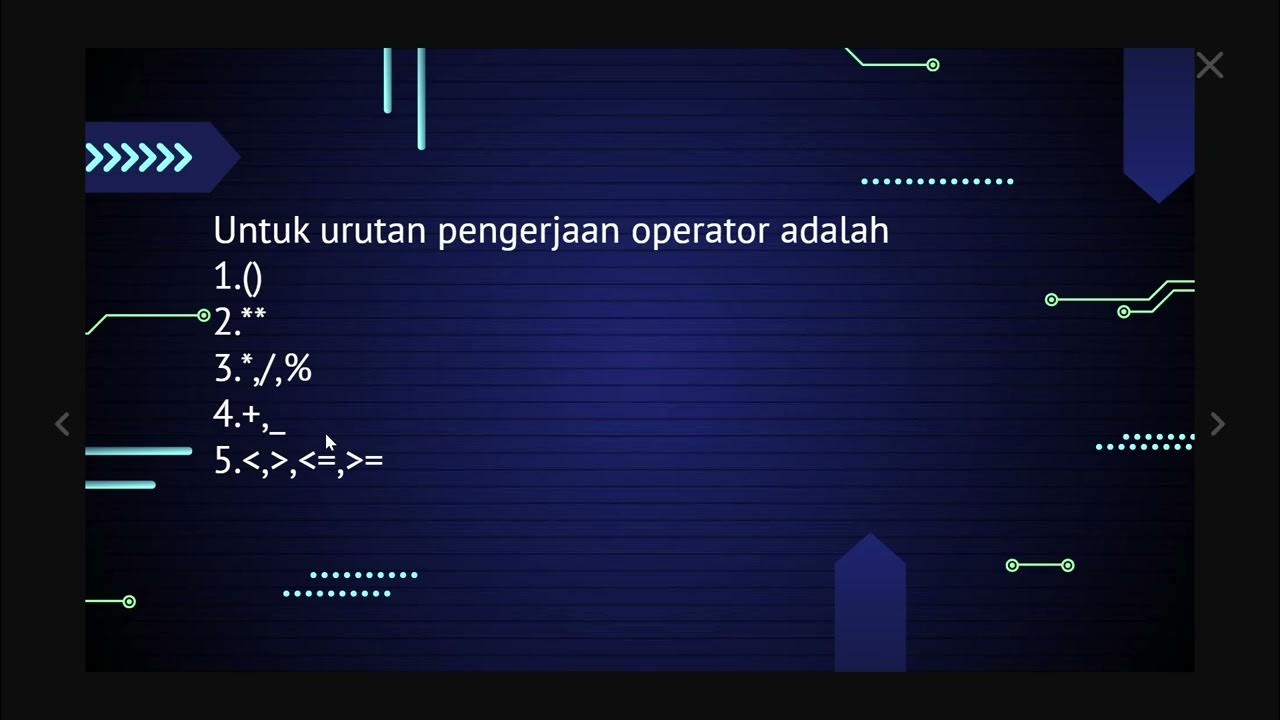
ALGORITMA dan PEMROGRAMAN || OPERATOR

#11 Python Tutorial for Beginners | Operators in Python
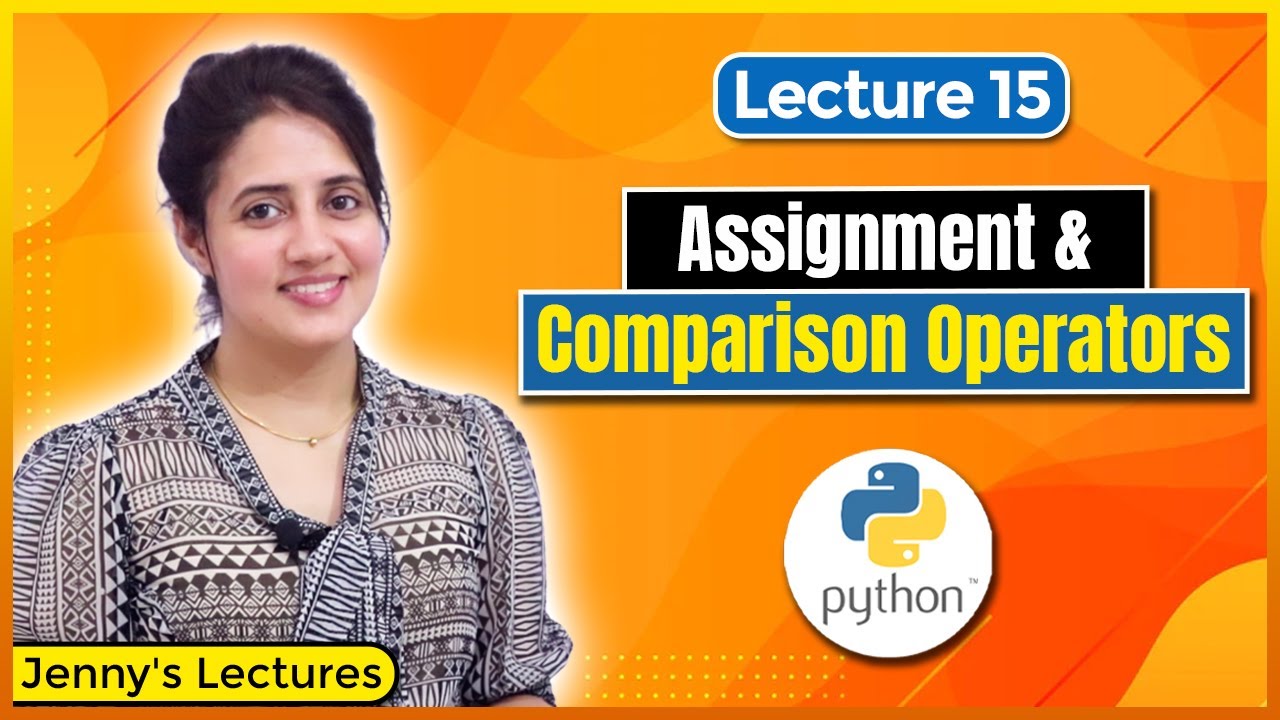
P_15 Operators in Python | Assignment and Comparison Operators | Python Tutorials for Beginners
5.0 / 5 (0 votes)