P_15 Operators in Python | Assignment and Comparison Operators | Python Tutorials for Beginners
Summary
TLDRThis Python tutorial delves into the world of operators, focusing on assignment and comparison operators. The instructor explains the fundamental concept of assigning values to variables with assignment operators, highlighting the importance of using variables on the left-hand side for proper assignment. Shorthand assignment operators are introduced, allowing for concise code by combining operations with assignment. The video also covers comparison operators, which are pivotal for conditional checks, returning boolean values based on the condition. Practical examples are provided to illustrate the application of these operators, ensuring a clear understanding of their functionality in Python programming.
Takeaways
- ๐ Assignment operators are used to assign values to variables in Python, with the equal sign (`=`) being the most basic form.
- ๐ก The left-hand side of the assignment operator must be a variable (or a named memory location), while the right-hand side is the value being assigned.
- ๐ซ It's illegal to have a constant on the left-hand side of an assignment, e.g., `5 = a` will result in a syntax error.
- โ Shorthand assignment operators, like `+=`, `-=`, `*=`, and `/=`, allow for more concise code by combining an operation with assignment.
- ๐ข You can perform multiple assignments in one line, such as `a, b, c = 5, 6, 7`, which assigns `5` to `a`, `6` to `b`, and `7` to `c`.
- โ๏ธ Comparison operators, also known as relational operators, are used to compare values. They include `==` (equals), `!=` (not equals), `<` (less than), and `>` (greater than), among others.
- ๐ Comparison operations return a Boolean value (`True` or `False`) based on the condition being evaluated.
- ๐ Relational operators can be used in combination with expressions, not just simple variables, allowing for more complex comparisons.
- ๐ฅ๏ธ The script demonstrates practical examples of both assignment and comparison operators, reinforcing the concepts with hands-on practice.
- ๐ An assignment exercise and lecture notes are provided in the video description for further practice and learning.
Q & A
What is the main topic of the video script?
-The main topic of the video script is discussing assignment and comparison operators in Python programming language.
What are the two types of operators primarily discussed in the video?
-The two types of operators primarily discussed in the video are assignment operators and comparison (relational) operators.
What is an assignment operator in Python, and how is it used?
-An assignment operator in Python is used to assign a value to a variable. It is represented by the '=' symbol, and the variable name is placed on the left-hand side, while the value or expression to be assigned is on the right-hand side.
Why is it incorrect to write '5 = a' in Python?
-It is incorrect to write '5 = a' in Python because the left-hand side of the assignment operator must be a variable, not a constant. Constants like '5' cannot be assigned values.
What are shorthand assignment operators in Python, and how do they work?
-Shorthand assignment operators in Python are a convenient way to perform an operation and assignment in a single step. They include '+=', '-=', '*=', '/=', '%=', '//=', '**=', and others, allowing for operations like addition, subtraction, multiplication, division, modulo, and exponentiation, respectively.
Can you assign values to multiple variables in one line using assignment operators?
-Yes, in Python, you can assign values to multiple variables in one line using assignment operators. For example, 'a, b, c = 5, 6, 7' assigns the values 5, 6, and 7 to the variables a, b, and c, respectively.
What are comparison operators, and what do they return?
-Comparison operators, also known as relational operators, are used to compare two values and check if a certain condition is true. They include '<', '>', '<=', '>=', '==', and '!='. The result of these operators is a boolean value, either 'True' or 'False'.
What is the purpose of the '==' operator in Python?
-The '==' operator in Python is used to check if two values are equal. If the values on both sides of the operator are the same, it returns 'True'; otherwise, it returns 'False'.
Can you use expressions with comparison operators in Python?
-Yes, you can use expressions with comparison operators in Python. For example, you can use 'a + 1 != 6' to check if the result of the expression 'a + 1' is not equal to 6.
What is the next topic the video series will cover after assignment and comparison operators?
-The next topic the video series will cover after assignment and comparison operators is logical operators.
Outlines
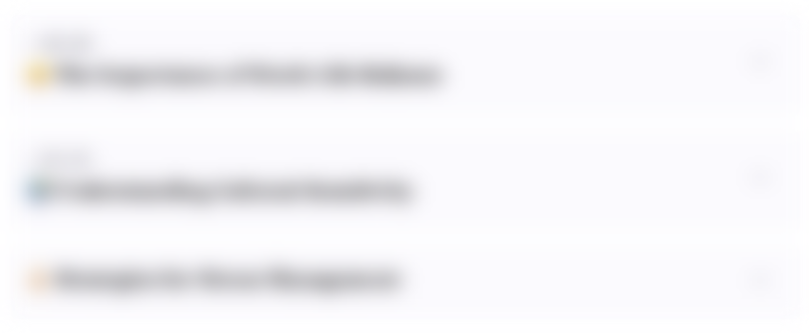
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
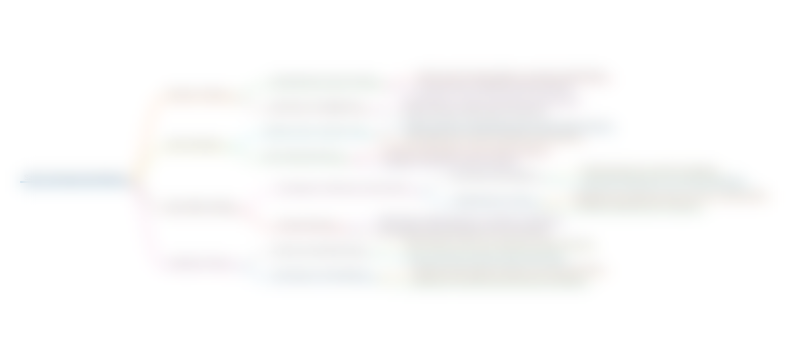
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
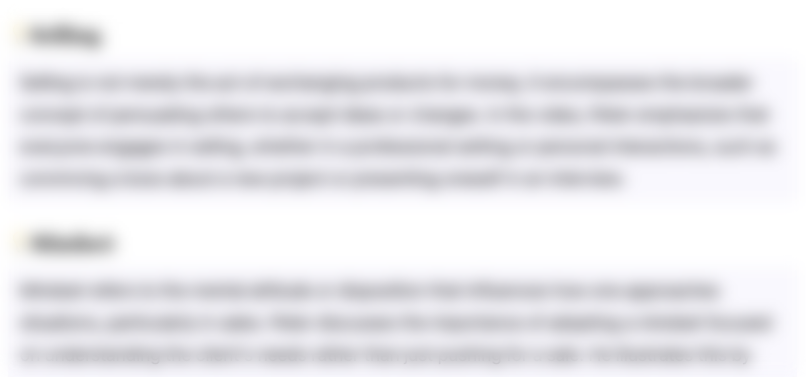
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
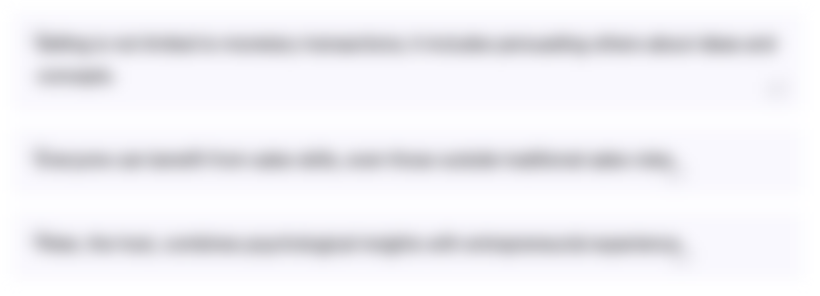
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
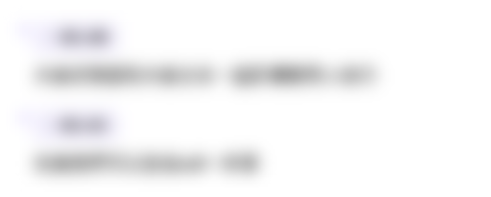
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
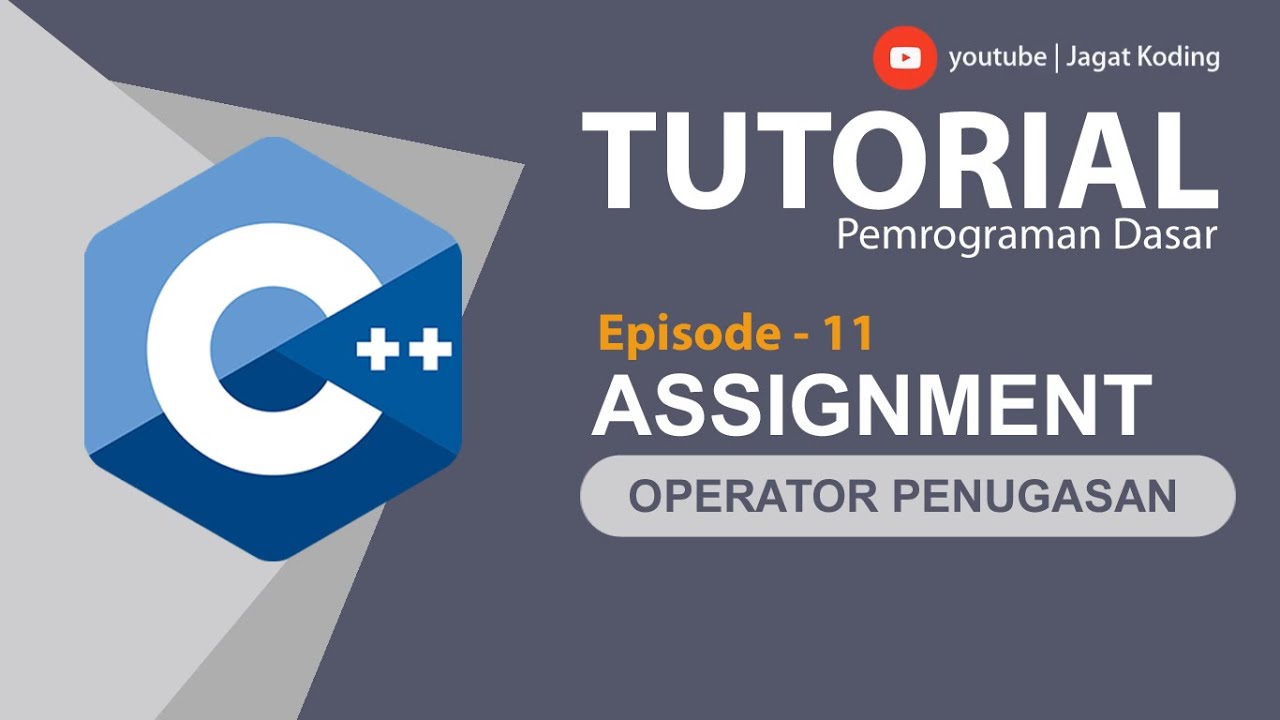
C++ 11 | Operator Assignment | Tutorial Pemrograman C++
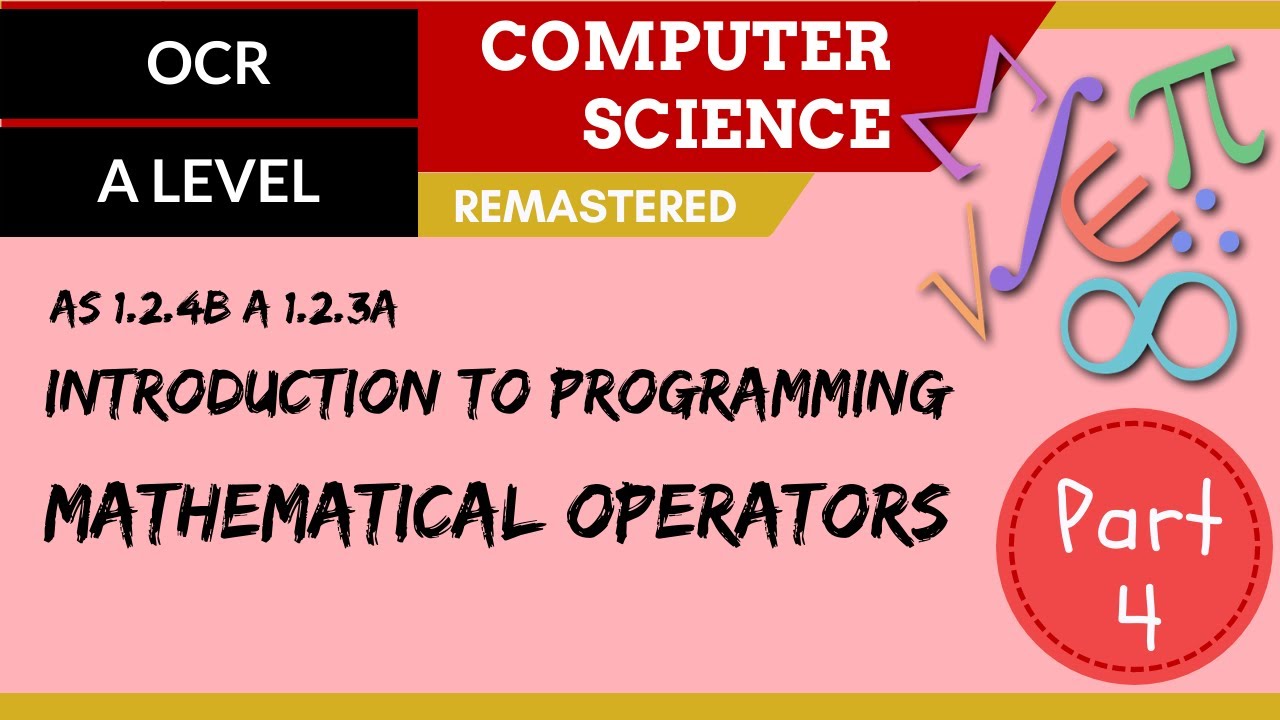
43. OCR A Level (H046-H446) SLR8 - 1.2 Introduction to programming part 4 mathematical operators

#11 Python Tutorial for Beginners | Operators in Python
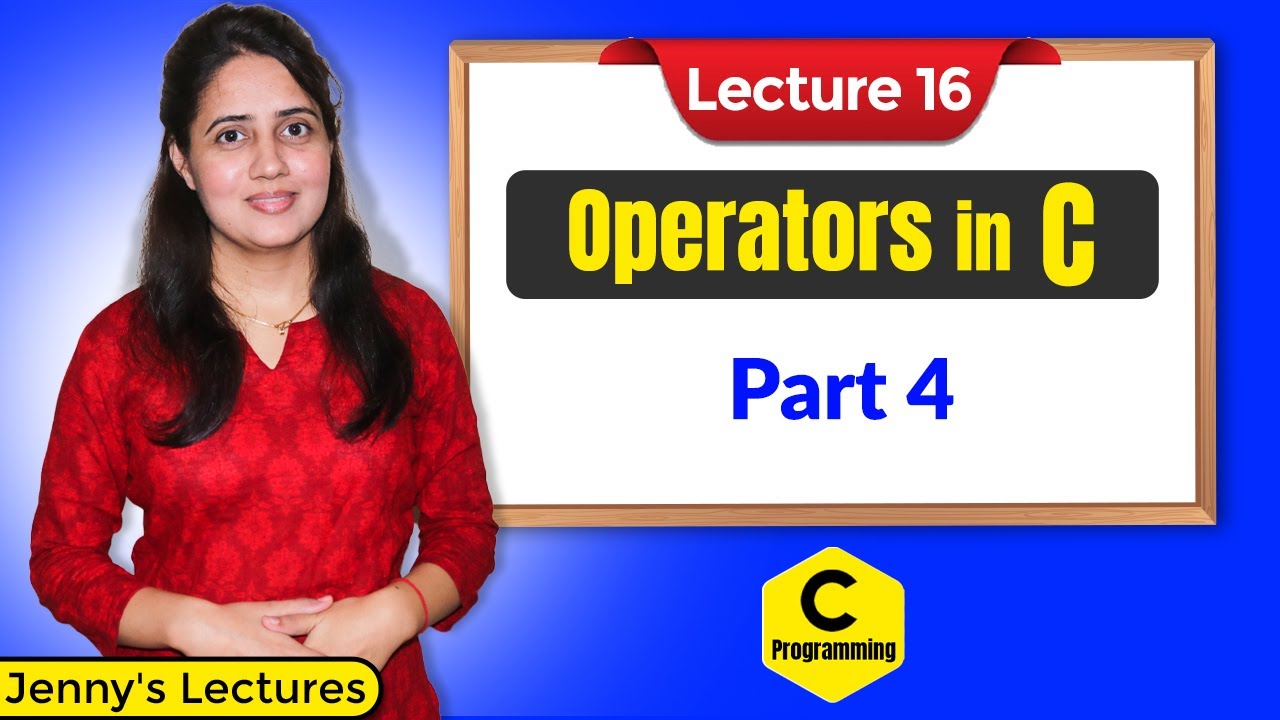
C_16 Operators in C - Part 4 | C Programming Tutorials

Operators & Operands | Godot GDScript Tutorial | Ep 02
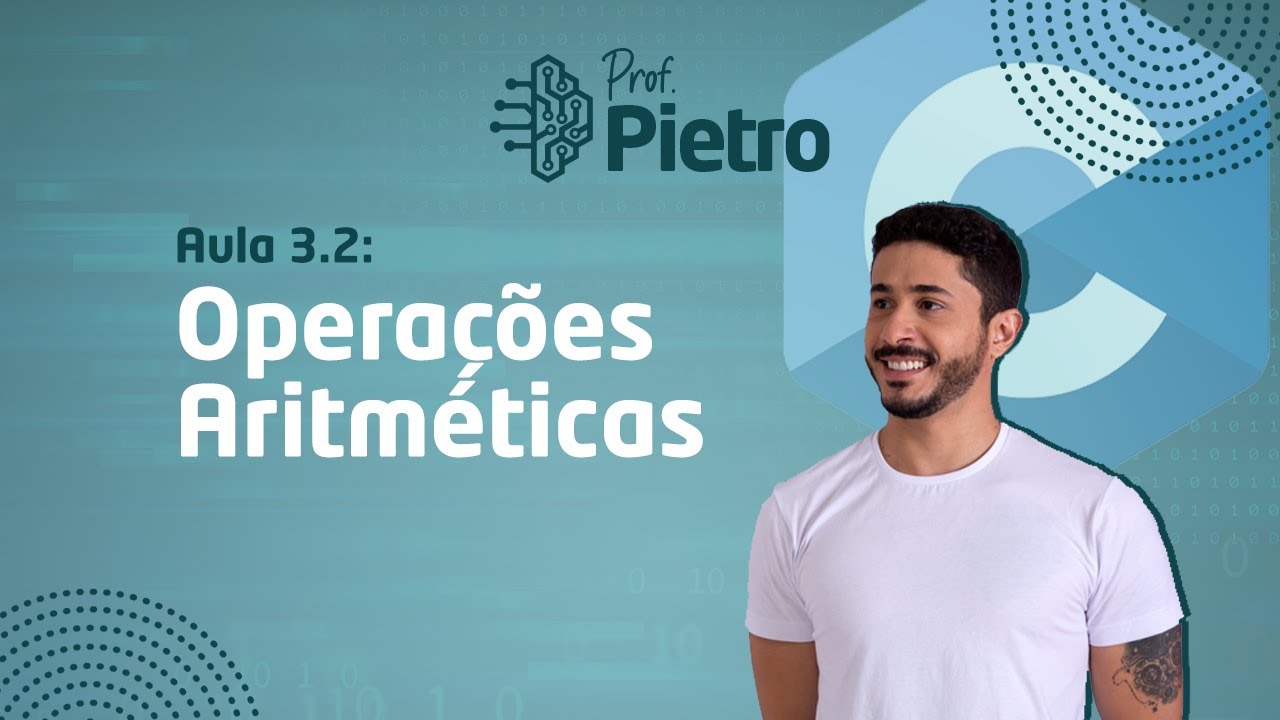
Linguagem C - Aula 3.2 - Aprenda a realizar cรกlculos em C (2022)
5.0 / 5 (0 votes)