#3: Data Types in C Programming | C Programming for Beginners
Summary
TLDRIn this video, we dive into the basics of data types in C programming, covering the essential types like `int`, `float`, `double`, and `char`. The tutorial explains how to declare and print variables of each type using the correct format specifiers. We explore the difference between `float` and `double`, and how to manage precision in floating-point numbers. Additionally, we introduce the `sizeof` operator to check the memory size of variables. With practical examples and tips, this video is perfect for beginners who want to understand the role of data types in C and how to use them effectively.
Takeaways
- 😀 Data types in C define the type and size of data that can be stored in a variable.
- 😀 The `int` data type is used for storing whole numbers (both positive and negative) and typically takes up 4 bytes.
- 😀 To print an `int` variable, you use the `printf` function with the `%d` format specifier.
- 😀 `float` and `double` are used for storing decimal and exponential values, with `double` offering higher precision (8 bytes vs. 4 bytes for `float`).
- 😀 When printing a `double` value, use the `%lf` format specifier. You can control decimal precision with a number after `%lf` (e.g., `%.2lf`).
- 😀 The `float` data type can be used similarly to `double` for decimals but uses less memory (4 bytes). Use the `F` suffix to indicate a float value.
- 😀 You can store and print exponential values using `float` or `double`, e.g., `5.5e6` is equivalent to `5.5 * 10^6`.
- 😀 The `char` data type is used for storing individual characters and is printed with the `%c` format specifier. It is internally stored as an integer.
- 😀 If you print a `char` variable as an integer (using `%d`), it shows the ASCII value of the character (e.g., `'J'` is 122).
- 😀 The `sizeof` operator can be used to find the size of data types like `int`, `double`, `float`, and `char`, helping to understand memory usage in C.
Q & A
What is the purpose of data types in C programming?
-In C programming, data types specify the type of data that can be stored in a variable. They determine both the type and the size of the variable, ensuring that the correct data is stored and manipulated in the program.
What does the 'int' data type store in C programming?
-The 'int' data type is used to store integer values, which can be positive, negative, or zero. It typically occupies 4 bytes of memory and can represent a range of values determined by the system's architecture.
What is the difference between 'float' and 'double' data types in C?
-'float' and 'double' are both used for storing floating-point numbers (decimal values). The main difference is that 'float' takes 4 bytes of memory, while 'double' takes 8 bytes, providing more precision for larger numbers.
Why should you use 'double' instead of 'float' in C programming?
-It is recommended to use 'double' because it provides greater precision for decimal numbers, as it uses 8 bytes of memory compared to 4 bytes for 'float', making it suitable for larger or more precise calculations.
How do you print a 'double' value in C?
-To print a 'double' value in C, you use the 'printf' function with the '%lf' format specifier. For example, 'printf("%lf", number);' would print the value stored in the 'number' variable of type 'double'.
What does the '%f' format specifier do in C programming?
-The '%f' format specifier is used to print floating-point numbers stored in variables of type 'float'. For better output formatting, you can specify the number of decimal places by using '%.2f', for example.
How do exponential numbers work with 'float' and 'double' data types in C?
-'float' and 'double' can both store exponential numbers using the 'E' notation. For example, '5.5E6' represents '5.5 * 10^6'. 'double' is typically preferred for storing larger exponential numbers due to its greater precision.
How does the 'char' data type work in C?
-The 'char' data type is used to store single characters, such as 'a', 'b', or any other alphabetic or symbolic character. Internally, 'char' values are stored as integers corresponding to the character's ASCII value.
How do you print a 'char' variable in C?
-To print a 'char' variable in C, you use the 'printf' function with the '%c' format specifier. For example, 'printf("%c", character);' would print the character stored in the 'character' variable.
What is the purpose of the 'sizeof' operator in C?
-The 'sizeof' operator in C is used to determine the size (in bytes) of a data type or variable. It helps in understanding memory allocation and optimizing data storage, especially when dealing with different data types like 'int', 'float', and 'char'.
Outlines
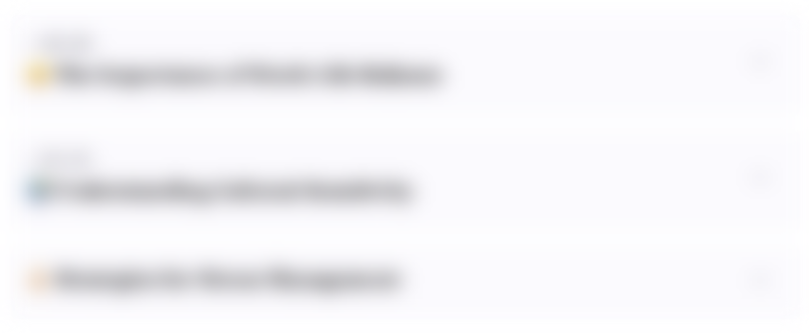
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
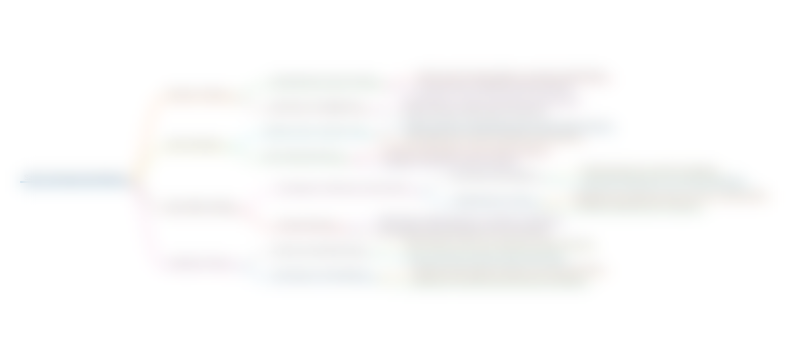
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
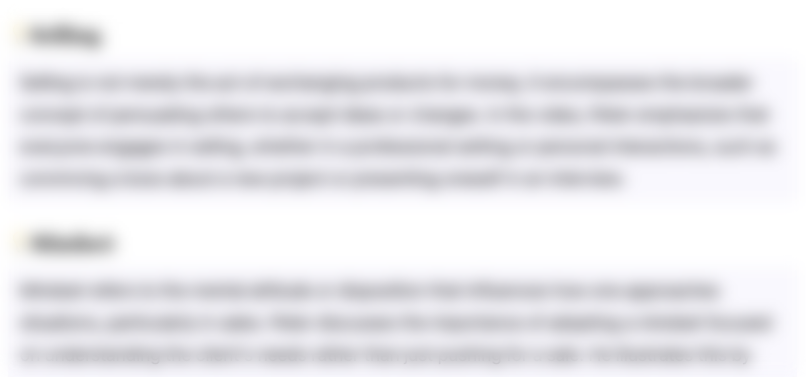
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
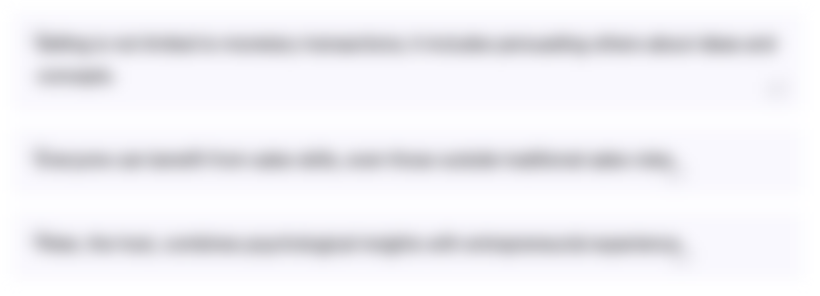
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
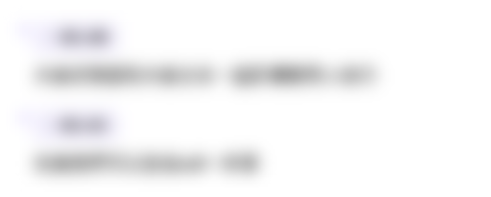
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)