Learn useMemo In 10 Minutes
Summary
TLDRThis video from Kyle's React hooks series dives into the use of the `useMemo` hook in React. It explains how `useMemo` can improve performance by caching the result of a function, preventing unnecessary re-computations. The video demonstrates how `useMemo` can be used to optimize a slow function that is executed on every render, showing how it can reduce the delay caused by such functions. Additionally, it covers the concept of referential equality in JavaScript and how `useMemo` can be utilized to ensure hooks like `useEffect` only rerun when there's a meaningful change in the referenced objects. The video concludes with a reminder to use `useMemo` judiciously to avoid unnecessary memory and performance overhead.
Takeaways
- ๐ **UseMemo Introduction**: The video introduces the `useMemo` hook in React, explaining its purpose and when it should be used.
- ๐ **Performance Optimization**: `useMemo` is used to optimize performance by memoizing the result of expensive function calls and only recomputing when necessary.
- โฑ๏ธ **Slow Function Problem**: The script demonstrates a slow function that is re-run on every render, which can be inefficient and cause delays in the user interface.
- ๐ก **Memoization Concept**: `useMemo` is based on the concept of memoization, caching the result of a function so it's not recalculated on every render when the inputs remain the same.
- ๐ **Dependencies List**: The hook takes a function and a list of dependencies; the function is re-executed only when one of the dependencies changes.
- ๐ **Instantaneous Updates**: By using `useMemo`, the video shows how the theme toggle can be updated instantly without waiting for a slow function to execute.
- ๐ง **Overuse Caution**: The video warns against overusing `useMemo` due to the additional performance and memory overhead it can introduce if not necessary.
- ๐ค **Referential Equality**: A second use case for `useMemo` is managing referential equality, ensuring objects or arrays are only considered changed when their actual content changes, not just their reference.
- ๐ **Effect on useEffect**: `useMemo` can be used to control when effects run by ensuring the reference to an object remains the same unless the dependency changes.
- ๐ ๏ธ **Selective Use of useMemo**: The video advises using `useMemo` selectively, only in cases where there are clear performance benefits or when dealing with referential equality issues.
- ๐ **Debugging Tips**: The script provides insights into debugging and understanding the reactivity and rendering behavior in React components.
Q & A
What is the main topic of the video?
-The main topic of the video is an explanation of the `useMemo` hook in React and its proper usage.
Why is `useMemo` important in React applications?
-`useMemo` is important because it helps improve performance by memoizing the results of expensive function calls and only recomputing them when one of the dependencies changes.
What does the video suggest as a condition for using `useMemo`?
-The video suggests using `useMemo` when there is a need for performance optimization with slow-running functions or when ensuring referential equality of objects or arrays.
Why might one not want to use `useMemo` for every piece of state in a React component?
-One might not want to use `useMemo` for every piece of state because it introduces additional memory and performance overhead by storing previous values and adding the extra function call on every render.
What is the downside of re-rendering a component with a slow function every time the state updates?
-The downside is that the slow function will be executed on every render, causing unnecessary delays and performance issues, especially when the function does not need to be re-executed for the specific state change.
How does `useMemo` help with referential equality in React?
-`useMemo` helps with referential equality by ensuring that the reference to an object or array remains the same across renders unless one of its dependencies changes, thus preventing unnecessary re-renders.
What is the purpose of the 'change theme' button in the example provided in the video?
-The 'change theme' button is used to toggle between a dark and light theme in the example application, demonstrating how state updates can trigger re-renders and the potential performance impact.
How does the video demonstrate the effectiveness of `useMemo`?
-The video demonstrates the effectiveness of `useMemo` by showing a noticeable performance improvement when toggling the theme without the delay that was present before using `useMemo` to wrap the slow function.
What is the role of dependencies in the `useMemo` hook?
-Dependencies in the `useMemo` hook determine when the memoized function should re-run. If any of the dependencies change, the function will be re-executed; otherwise, the cached result will be used.
What is the second use case for `useMemo` discussed in the video?
-The second use case for `useMemo` discussed in the video is to ensure that the reference of an object or array remains constant across component re-renders unless the actual content of the object changes.
What does the video recommend for optimizing React applications?
-The video recommends using `useMemo` judiciously, only in cases where there are significant performance benefits, such as with slow functions or when dealing with referential equality issues.
Outlines
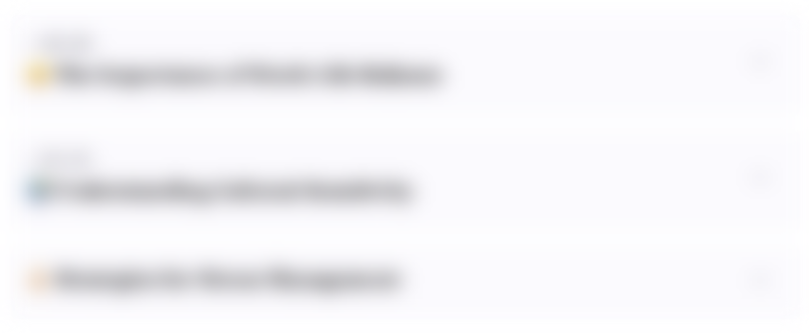
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
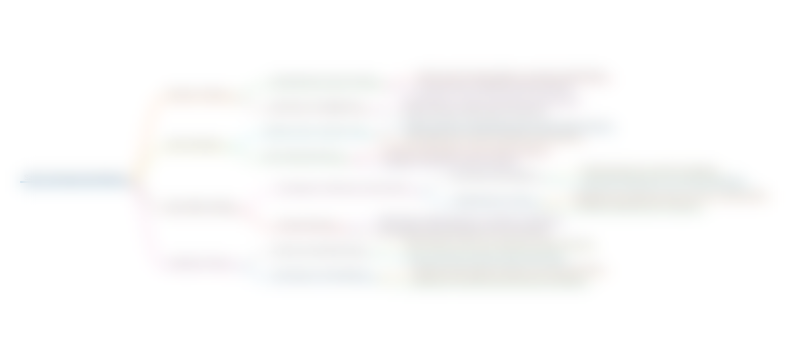
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
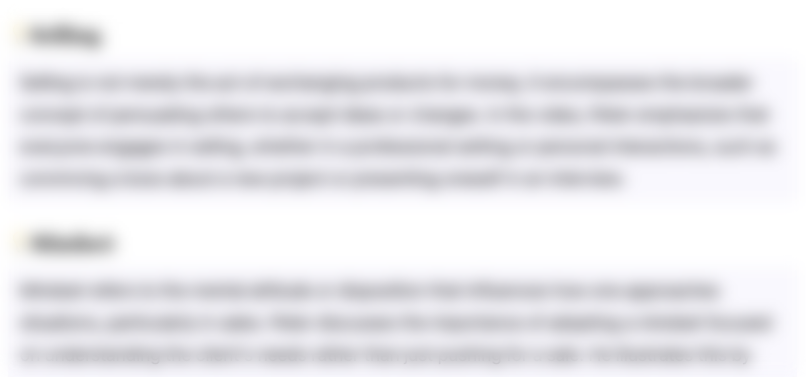
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
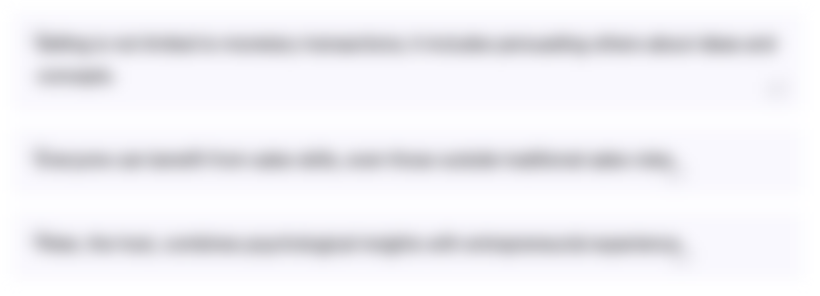
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
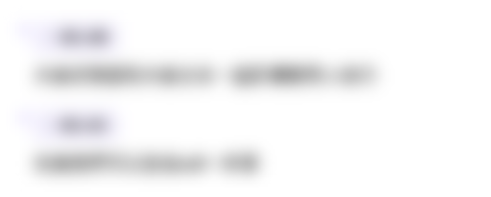
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

NEW React 19 Changes Are Amazing!

React Query - Complete Tutorial
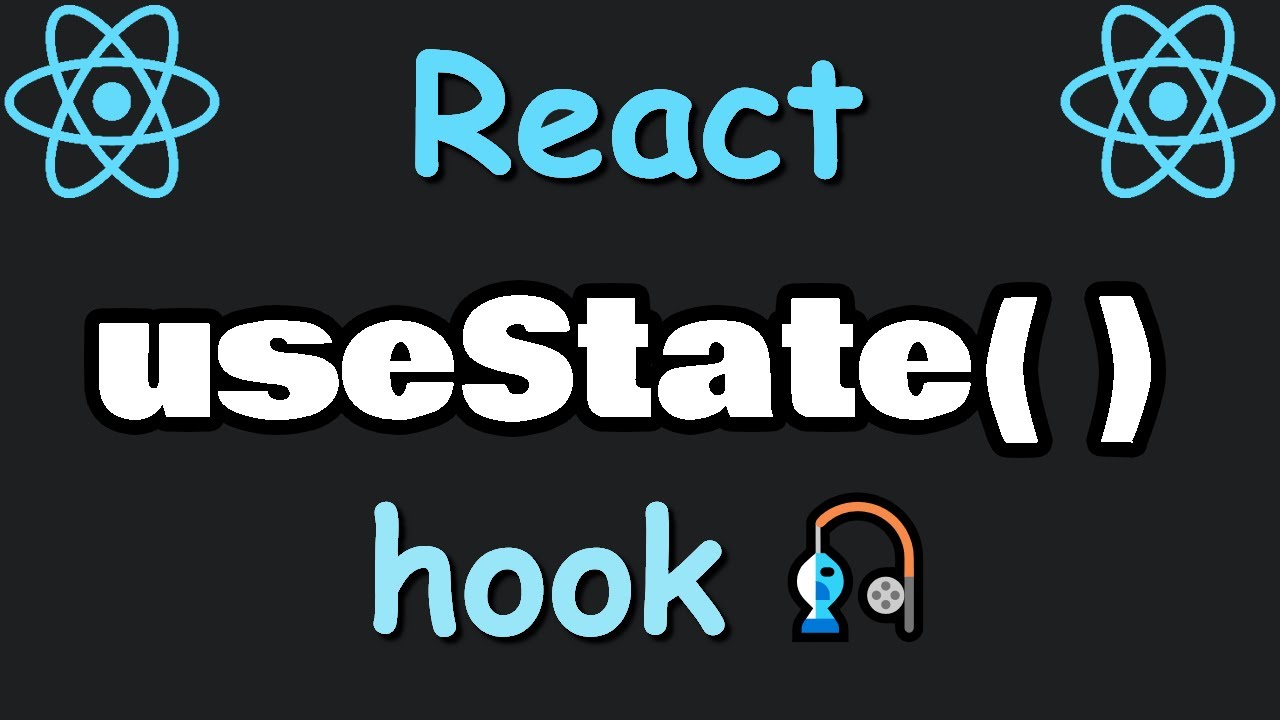
React useState() hook introduction ๐ฃ
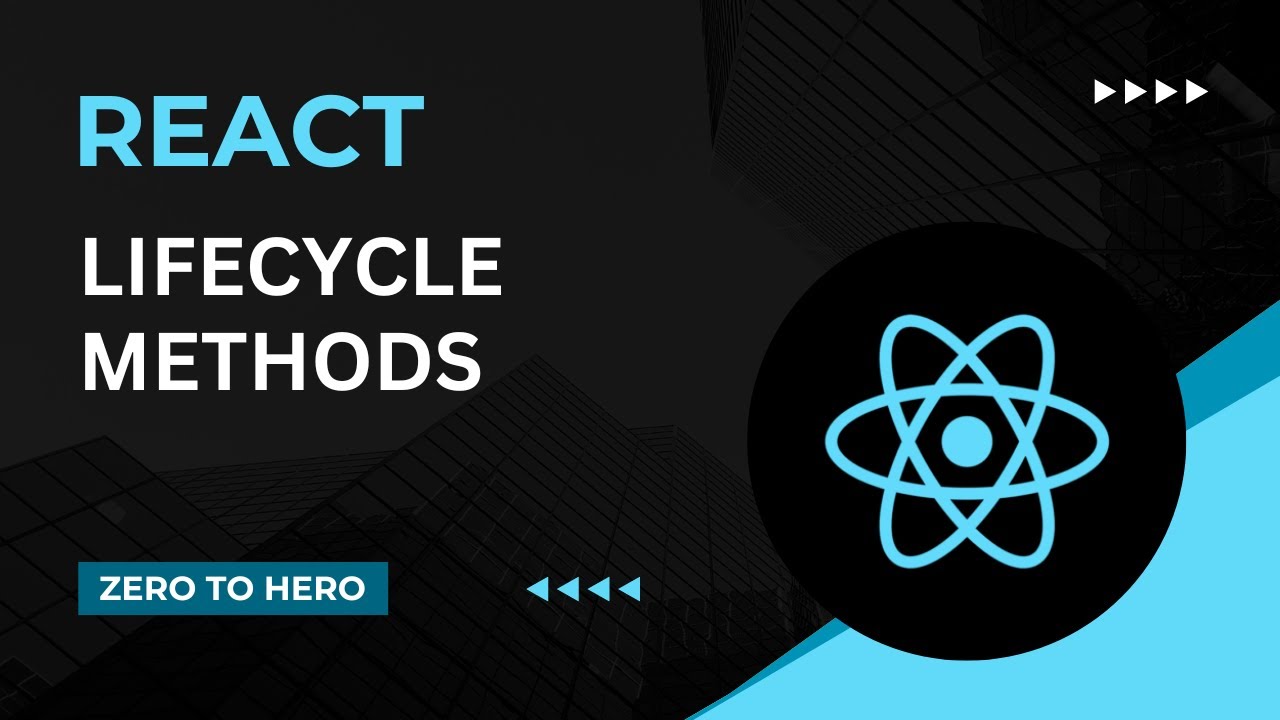
Life Cycle Methods | Mastering React: An In-Depth Zero to Hero Video Series
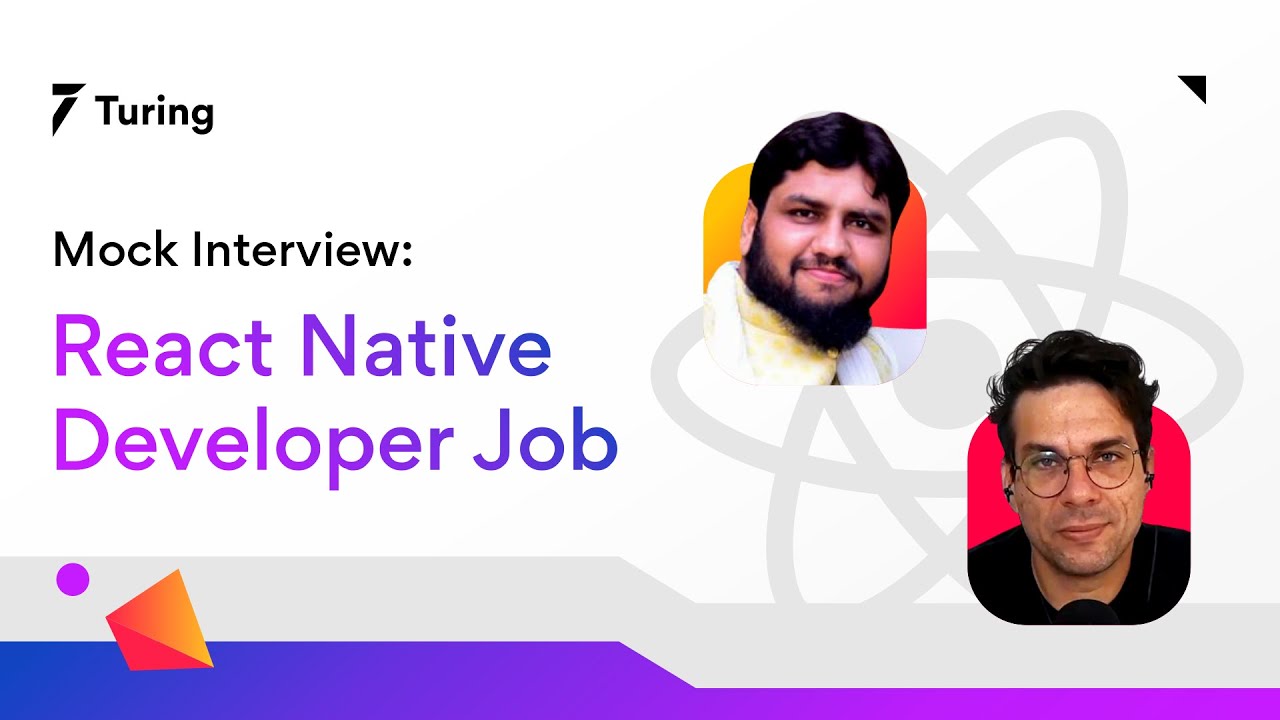
React Native Mock Interview | Interview Questions for Senior React Native Developers
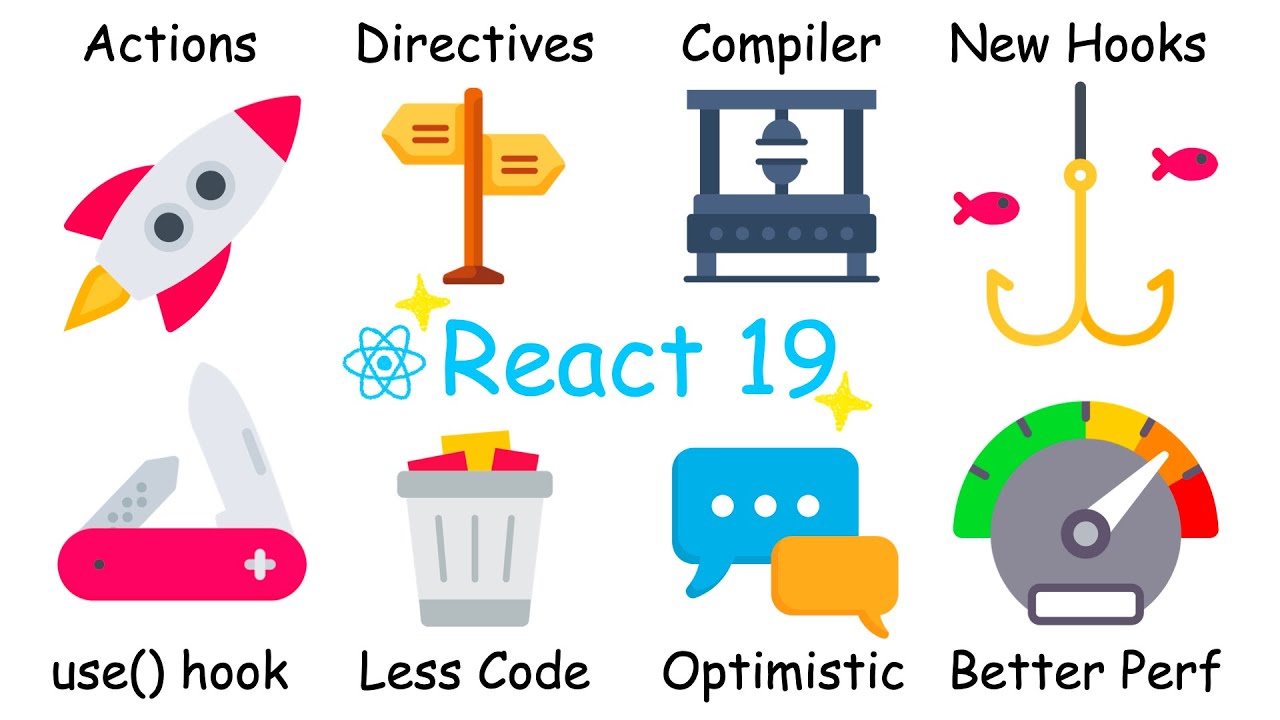
Every React 19 Feature Explained in 8 Minutes
5.0 / 5 (0 votes)