Every React 19 Feature Explained in 8 Minutes
Summary
TLDRReact 19 introduces a game-changing update with the React compiler, which optimizes code and reduces the need for manual performance tools like memoization. It simplifies passing refs to child components, and the new 'use' hook replaces 'useEffect' and 'useContext' for cleaner data fetching and context consumption. Directives, actions, and form-related hooks enhance client and server interactions, while 'useOptimistic' provides real-time app improvements. This update streamlines React development, making it faster and more efficient.
Takeaways
- π React 19 introduces significant updates that focus on reducing the amount of code developers need to write and improving app performance.
- π οΈ The React compiler is a core feature of React 19, converting React code into regular JavaScript to enhance performance without manual intervention.
- π§ React 19 removes the need for performance hooks like `useCallback`, `useMemo`, and `React.memo` due to the compiler's automatic optimizations.
- π The `forwardRef` function is no longer necessary; refs can now be passed directly as props and accessed similarly to other props.
- π The new `use` hook can asynchronously load resources, effectively replacing `useEffect` for data fetching and `useContext` for context data.
- π Directives are a simple addition to React, allowing developers to specify where components should run, either on the client with `use client` or server with `use server`.
- π Actions in React 19 simplify form handling, executing functions on form submission that can now run on either the server or client.
- π The `useFormStatus` hook helps manage form submissions by providing information on the submission status, useful for disabling submit buttons during processing.
- π The `useFormState` hook is a stateful action that uses action functions to set new state values, enhancing form interaction and state management.
- π For e-commerce applications, `useFormState` can be used to implement features like add-to-cart buttons, providing immediate feedback to the user.
- π The `useOptimistic` hook allows for optimistic updates, improving user experience by immediately reflecting user actions in the UI before server confirmation.
Q & A
What is the main focus of React 19 update?
-React 19 focuses on reducing the amount of code developers need to write, with the introduction of a compiler that optimizes React code automatically, thus removing the need for manual performance hooks.
What does the React compiler do in version 19?
-The React compiler converts React code into regular JavaScript, improving overall app performance and reducing the need for manual performance considerations.
Why are manual memoization tools like useCallback, useMemo, and memo no longer necessary in React 19?
-The new compiler in React 19 optimizes code automatically, which means developers can remove these performance hooks as the compiler handles the optimization.
What change has been made regarding the use of the forwardRef function in React 19?
-In React 19, the need for the forwardRef function has been eliminated, allowing developers to pass refs as props and use them directly without additional functions.
What is the new 'use' hook introduced in React 19 and how does it work?
-The new 'use' hook in React 19 is a multi-purpose hook that can load different resources asynchronously, effectively replacing 'useEffect' for data fetching and 'useContext' for reading context data.
How does the 'use' hook simplify fetching data from an API compared to 'useEffect'?
-With the 'use' hook, developers can resolve promises directly, show a fallback UI with React Suspense during data fetching, and display the fetched data in the UI once the promise is resolved, making the process cleaner and easier than with 'useEffect'.
What are directives in React and how do they differ from previous versions?
-Directives are strings added to the top of component files that tell React where to run a component, either on the client with 'use client' or on the server with 'use server'. This is a new feature in React 19 that simplifies component placement.
What is the purpose of the 'actions' feature in React 19 for forms?
-The 'actions' feature in React 19 simplifies form handling by allowing functions to be called when a form is submitted. These functions can now be executed on the server or client, improving form interactivity.
How does the 'useFormStatus' hook help with form submissions in React 19?
-The 'useFormStatus' hook provides information about the status of a form submission, such as when it is pending. This helps in managing UI elements like disabling the submit button during submission to prevent multiple submissions.
What is the 'useFormState' hook in React 19 and how does it differ from 'useState'?
-The 'useFormState' hook is similar to 'useState' but uses an action function to set the new state. It is designed to work with form submissions, allowing developers to access both the previous state and the submitted form data within the action function.
How does the 'useOptimistic' hook improve user experience in real-time applications?
-The 'useOptimistic' hook performs an optimistic update, immediately reflecting user actions in the UI before the server confirms the action. This provides a faster response to user inputs, improving the perceived performance of real-time applications.
Outlines
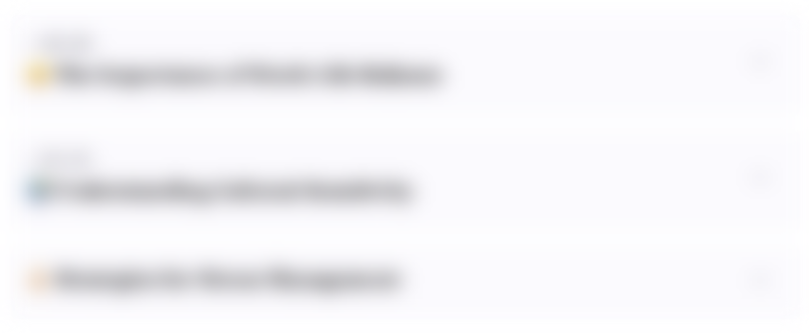
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
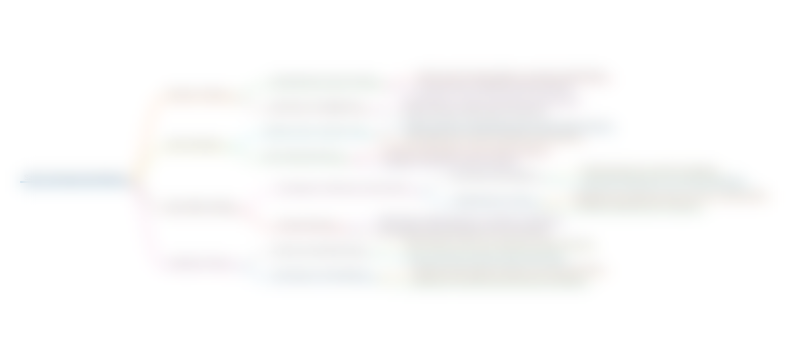
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
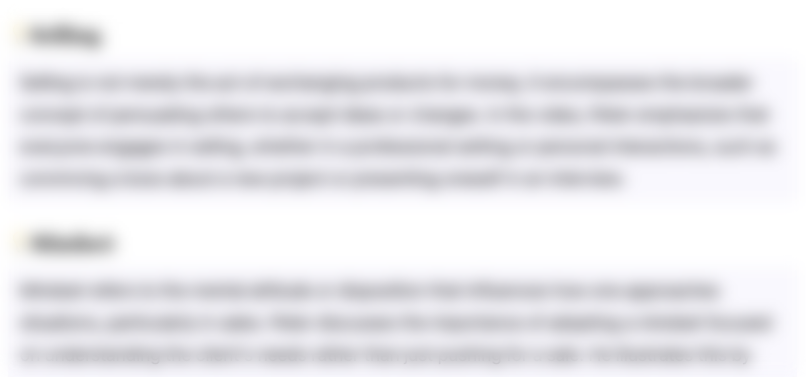
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
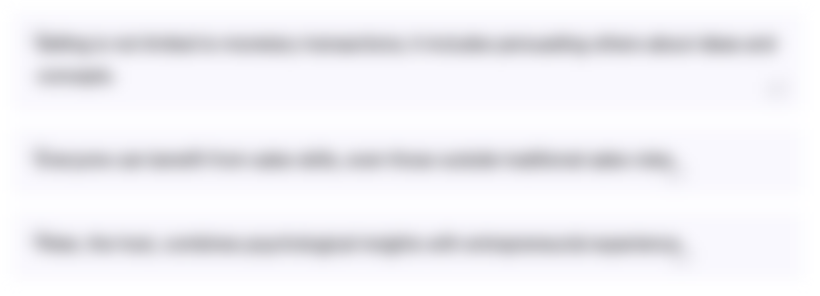
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
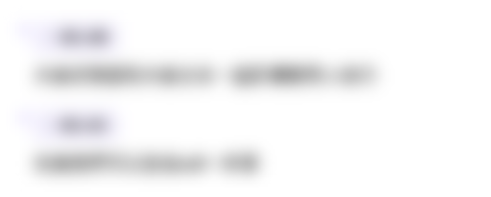
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)