Arrays vs Linked Lists - Data Structures and Algorithms
Summary
TLDRIn this video, the differences between arrays and linked lists are explained, focusing on their structures, memory handling, and performance. Arrays are great for storing fixed-size data but come with limitations in resizing, whereas dynamic arrays automatically grow as needed. Linked lists, on the other hand, allow for efficient insertion and deletion but require sequential access to elements, making them slower for indexed retrieval. The speaker discusses practical use cases, including hash tables where linked lists are used for collision handling. The video is ideal for developers and students looking to understand the advantages and trade-offs of these data structures.
Takeaways
- π Static arrays store data in contiguous memory, and their size is fixed when created. They are best used when you know the exact number of elements upfront.
- π Dynamic arrays can resize automatically when more elements are added than the array's current capacity. This gives flexibility when the size of the data set is unknown.
- π A key advantage of dynamic arrays is that they handle memory resizing automatically, eliminating the need for developers to manage memory manually.
- π Static arrays are prone to errors if elements exceed their size limit. Developers must keep track of array length manually in languages like C.
- π Dynamic arrays use a 'logical length' (how many elements are currently used) and a 'physical length' (how much memory is allocated), which can differ.
- π Linked lists store data in nodes, where each node contains both the data and a pointer to the next node. This allows for flexible memory allocation, unlike arrays.
- π Linked lists are particularly useful when frequent insertions and deletions are needed, as these operations don't require shifting elements like in arrays.
- π The downside of linked lists is that accessing elements by index is slower than in arrays, because you must traverse the list sequentially from the head.
- π Linked lists are not stored sequentially in memory. Instead, each node can be scattered across memory, with pointers connecting them.
- π Dynamic arrays are more commonly used in modern programming due to their ease of use and automatic resizing, but linked lists are still valuable in specific scenarios, like in hash tables for handling collisions.
- π When working with hash maps, linked lists can be used to resolve collisions by storing multiple elements at the same index in a linked list structure.
- π In programming languages like C++, Java, and Python, dynamic arrays (e.g., vectors, ArrayLists, and lists) are the default collection types due to their flexibility and performance.
Q & A
What is the primary reason to use an array or linked list?
-The primary reason to use an array or linked list is to store multiple pieces of data efficiently, especially when managing large sets of data. Both structures allow grouping of data, making it easier to access and manipulate multiple values compared to using separate variables.
What is the difference between a static array and a dynamic array?
-A static array has a fixed size determined at the time of declaration and cannot be changed. In contrast, a dynamic array can resize itself when more space is needed, allowing for flexibility as data grows.
How does a static array store data in memory?
-A static array stores its data in contiguous memory locations, meaning all elements are placed next to each other in the computerβs RAM. Once the size of the array is set, it cannot grow or shrink.
What is a major disadvantage of using static arrays?
-The major disadvantage of static arrays is that they require you to manage the array's size manually. If you try to add more data than the array can hold, it will throw an error or cause unexpected behavior.
What is the benefit of using dynamic arrays over static arrays?
-Dynamic arrays automatically resize themselves when more space is needed. This feature makes them more flexible and easier to work with, as you do not have to manage the size manually.
How do dynamic arrays manage their memory when resizing?
-When the logical length of a dynamic array exceeds its physical length, the array automatically doubles its allocated memory size. The data is then copied into a new, larger memory block.
Why do some programming languages provide built-in dynamic arrays like Java's ArrayList or Python's list?
-These languages provide built-in dynamic arrays because they make memory management simpler for developers. They automatically handle resizing and maintaining the array's length, reducing the potential for errors and improving code efficiency.
What is a linked list, and how does it differ from an array?
-A linked list is a data structure where each element (node) contains data and a pointer to the next node in the list. Unlike arrays, which store elements in contiguous memory locations, linked lists can store data anywhere in memory and are more flexible in terms of adding or removing elements.
What are the key advantages of using a linked list?
-Linked lists are flexible and efficient for insertions and deletions, especially when adding or removing elements from the middle of the list. They don't require contiguous memory, which makes them more adaptable when data sizes change.
What are the performance drawbacks of linked lists compared to arrays?
-Linked lists have slower access times compared to arrays because you cannot directly access an element by its index. To find a specific element, you must start at the head of the list and traverse through each node sequentially.
In what situations might you prefer a linked list over a dynamic array?
-You might prefer a linked list when your program requires frequent insertions or deletions, especially in the middle of the data structure. Linked lists are also useful when memory is fragmented and a contiguous block of memory is not available.
How does a linked list work in the context of hash maps?
-In hash maps, a linked list is used to handle hash collisions. When two keys hash to the same index, the linked list stores the multiple key-value pairs at that index, allowing efficient retrieval despite the collision.
Outlines
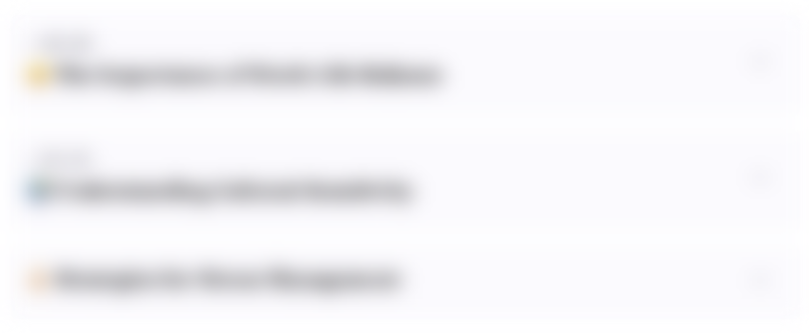
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
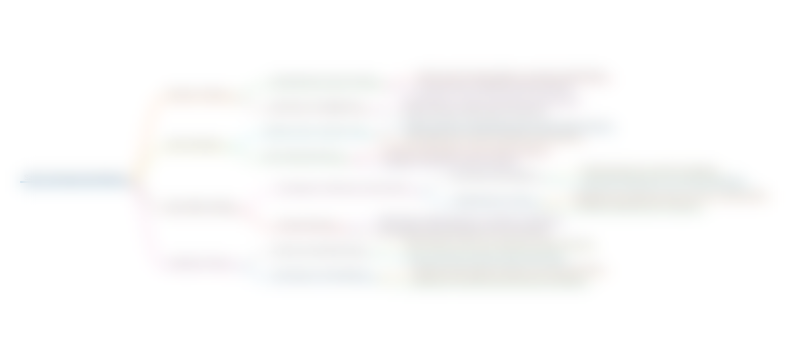
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
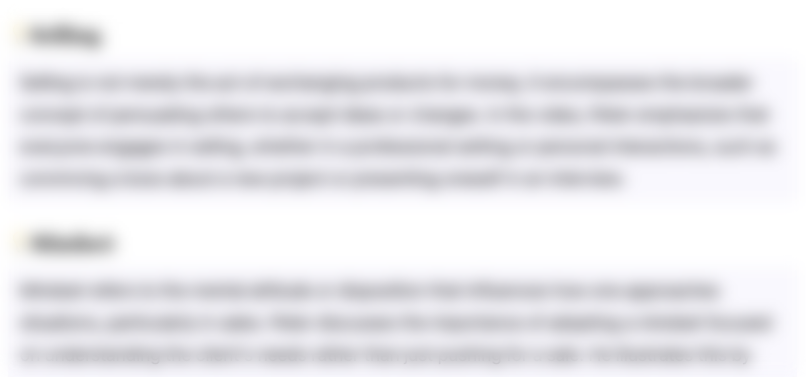
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
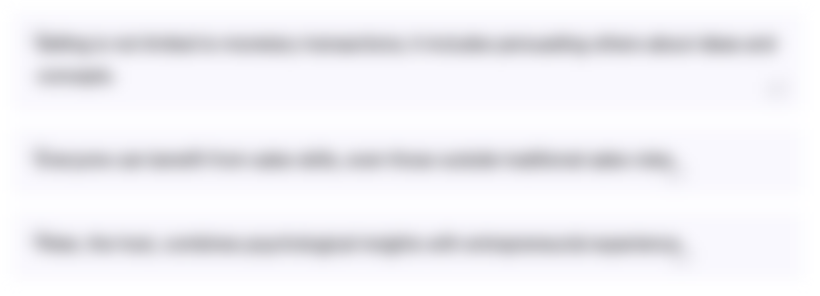
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
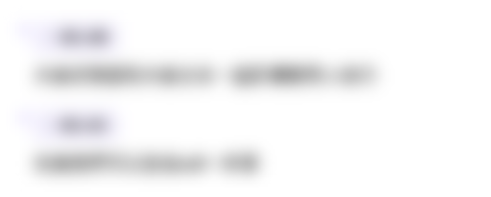
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)