Data Structures: Arrays vs Linked Lists
Summary
TLDRThis lesson compares arrays and linked lists based on their performance and use cases. Arrays provide constant time access but require fixed memory allocation, which can lead to wasted space or fragmentation issues. Linked lists, on the other hand, offer dynamic memory allocation and faster insertions/removals at the cost of slower access times. The choice between them depends on the specific requirements of the task, such as frequent operations and data size.
Takeaways
- π There's no universally superior data structure; the choice between an array and a linked list depends on specific requirements such as operation frequency and data size.
- π Arrays provide constant time complexity (O(1)) for element access due to their contiguous memory storage, unlike linked lists which have a time complexity of O(n).
- πΎ Arrays require fixed memory allocation upfront, potentially leading to unused memory, while linked lists allocate memory dynamically without reserved space but use extra memory for pointer variables.
- π Linked lists can be advantageous when dealing with large data elements, as they consume less memory compared to arrays with significant unused space.
- π§© Memory fragmentation is less of an issue with linked lists since they can utilize small blocks of memory, unlike arrays that might struggle to find a single large block.
- π The cost of inserting or deleting elements in arrays is O(n) for operations at the beginning or middle, while linked lists offer O(1) for insertions at the beginning and O(n) for ends or middles.
- π For dynamic arrays, inserting at the end is O(1) if there's space, but requires O(n) time for array resizing and copying if the array is full.
- π Implementing linked lists can be more complex and error-prone compared to arrays, with potential issues like segmentation faults and memory leaks.
- π The choice between arrays and linked lists should consider factors like ease of use, memory efficiency, and the specific operations that need to be optimized for the application.
- π¬ The next lesson will involve practical implementation of linked lists in C or C++, providing hands-on experience with the concepts discussed.
Q & A
What is the primary difference between arrays and linked lists?
-Arrays store elements in a contiguous block of memory, allowing constant time access to any element, while linked lists store elements in separate blocks, connected by pointers, which results in linear time complexity for accessing elements.
Why might a linked list be preferred over an array in terms of memory usage?
-Linked lists are more memory efficient when dealing with large data types because they do not reserve unused memory, unlike arrays which allocate a fixed size and may have unused space.
What is the time complexity for accessing an element in an array?
-Accessing an element in an array is O(1), meaning it takes constant time regardless of the array's size.
How does the time complexity for accessing an element in a linked list compare to an array?
-Accessing an element in a linked list is O(n), where n is the number of elements in the list, because you may need to traverse the entire list to reach a specific element.
What is the memory requirement difference between an array and a linked list when storing integers?
-An array of 7 integers requires 28 bytes (assuming 4 bytes per integer), while a linked list of 3 integer nodes requires 24 bytes (8 bytes per node, with 4 bytes for the integer and 4 bytes for the pointer).
Why might an array be difficult to use when the size of the data elements is large?
-When the data elements are large, the memory requirement for an array increases significantly due to the fixed size allocation, which can lead to memory wastage and potential fragmentation issues.
What is the time complexity for inserting an element at the beginning of a list in an array?
-Inserting an element at the beginning of an array is O(n) because all existing elements must be shifted to make room for the new element.
How does the time complexity for inserting an element at the beginning of a linked list compare to an array?
-Inserting at the beginning of a linked list is O(1) because it only requires the creation of a new node and adjustment of the head pointer and the new node's link.
What are the three scenarios for inserting an element in an array, and what is the time complexity for each?
-The three scenarios are: inserting at the beginning (O(n)), inserting at the end if there is space (O(1)), and inserting at the end if the array is full and requires resizing (O(n)).
Which data structure is generally easier to use and implement, and why?
-Arrays are generally easier to use and implement because they have a straightforward structure and are less prone to errors like segmentation faults and memory leaks, which can occur with linked lists.
Outlines
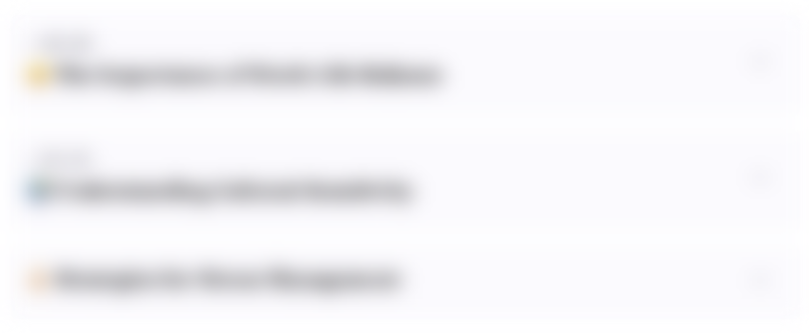
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
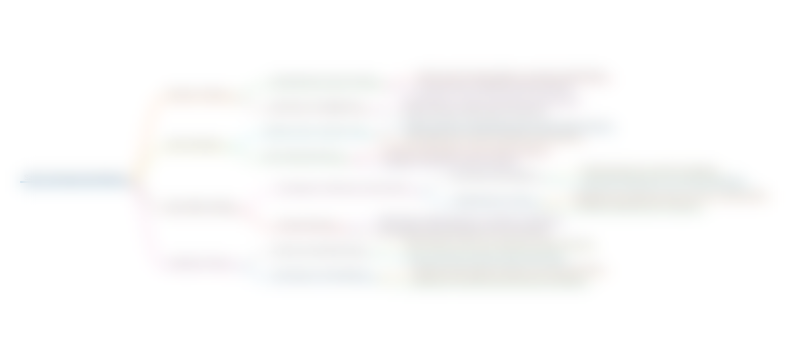
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
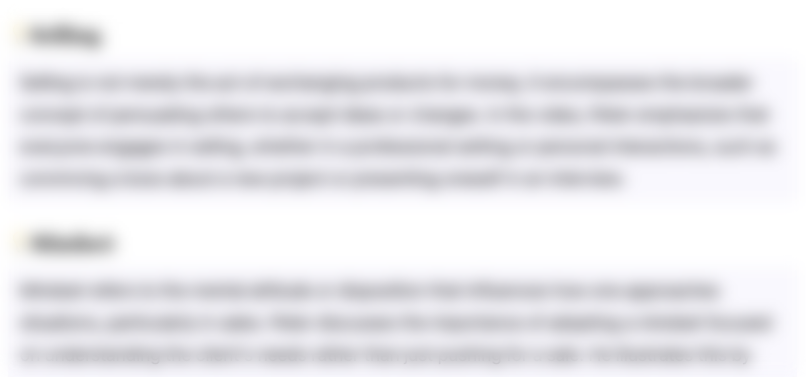
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
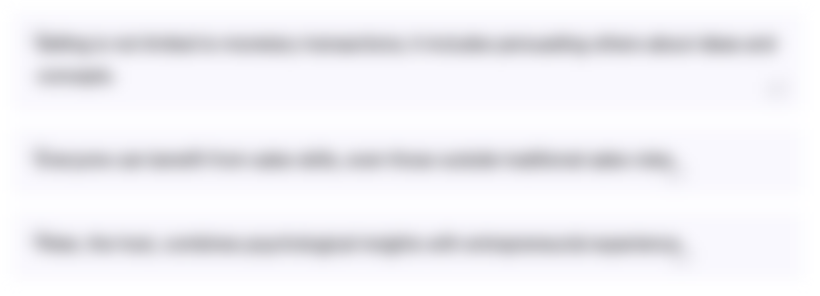
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
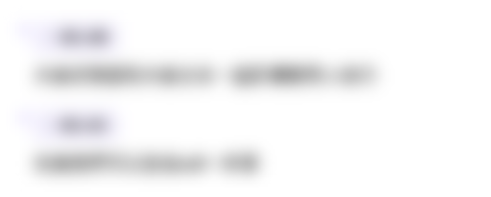
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)