React Interview Questions | A Preparation Guide
Summary
TLDRThis video provides essential React interview preparation tips, focusing on key topics like lifecycle methods, conditional rendering, and the importance of using keys when rendering lists. It also covers React hooks such as `useState`, `useEffect`, and `useContext`, and touches on performance optimization techniques with tools like `React.memo` and `useCallback`. Additionally, it dives into Redux, explaining its core concepts like actions, reducers, and the store, while comparing it to the Context API. The video concludes with advice on coding rounds and using React with common packages and tools in the ecosystem, preparing viewers for both conceptual and practical challenges.
Takeaways
- π HarperDB is a distributed database that simplifies database management with a REST API, supporting NoSQL and SQL, and can be used without coding.
- π Be prepared to answer 'What do you like and dislike about React?' to assess your understanding of React's features like unidirectional data flow and JSX.
- π Conditional rendering and list rendering in React are common interview topics; know the significance of using keys when rendering lists and the issues with using indexes as keys.
- π Understanding class components' lifecycle methods (mounting, updating, unmounting) is still crucial for maintaining legacy code, even with hooks being popular.
- π The Context API helps resolve prop drilling by managing state more efficiently across components, a key topic to prepare for.
- π React hooks like `useState`, `useEffect`, and `useContext` are essential; know how `useEffect` relates to component lifecycle methods.
- π Optimization topics such as `React.memo`, `useMemo`, and `useCallback` are critical for preventing unnecessary re-renders and improving app performance.
- π To share logic across components, be familiar with techniques like Higher Order Components (HOCs), Render Props, and custom hooks.
- π Familiarity with the React ecosystem, including styling, routing, form handling, and state management libraries, is important for interviews.
- π Redux is a key state management library in React, and interview questions often cover its core concepts: store, actions, reducers, and control flow.
- π In coding rounds, be prepared to build tricky layouts or popular components like modals and toast notifications, combining React with CSS knowledge.
Q & A
What is HarperDB, and how does it help developers?
-HarperDB is a distributed database that simplifies the database management process for developers of all skill levels. It offers an easy-to-use REST API, supports both SQL and NoSQL databases, and includes tools like a management studio to handle databases without needing to write code. Developers can also use example code, SDKs, and npm drivers, such as a React hook, to integrate HarperDB into their applications.
Why is it important to prepare for the question 'What do you like and dislike about React' in an interview?
-This question serves as an icebreaker and helps interviewers assess your understanding of React. It also gives you the opportunity to discuss specific aspects of React that you enjoy, such as its unidirectional data flow, while acknowledging potential challenges, like the initial difficulty with JSX.
What is JSX, and why might it be challenging for new React developers?
-JSX is a syntax extension for JavaScript that allows developers to write HTML-like code within JavaScript. It can be challenging for new developers because it combines JavaScript and HTML, which can initially feel confusing, especially when it comes to concepts like expressions and nesting.
What is the significance of using keys when rendering a list in React?
-In React, keys are used to uniquely identify elements in a list to help React efficiently compare and re-render only the changed elements. Using an index as a key can introduce bugs because it doesn't guarantee unique identifiers, leading to incorrect rendering or performance issues.
Why are class components and lifecycle methods still relevant in interviews, even with the rise of React Hooks?
-Class components and lifecycle methods are still relevant in interviews because many legacy codebases continue to use them. Understanding how lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount work is essential for maintaining and updating such code.
What is prop drilling, and how does the Context API solve this problem?
-Prop drilling occurs when you pass data through multiple layers of components, even if intermediate components don't need it. The Context API solves this by allowing you to share state directly between components at different levels of the component tree, eliminating the need to pass props down manually at each level.
What are React Hooks, and why were they introduced?
-React Hooks were introduced to allow developers to manage state and side effects in functional components, without needing to use class components. Key hooks like useState, useEffect, and useContext simplify component logic and enhance code readability by replacing lifecycle methods.
How does useEffect work in React, and how does it relate to class component lifecycle methods?
-The useEffect hook manages side effects in functional components. It runs after the component renders, making it similar to componentDidMount, componentDidUpdate, and componentWillUnmount in class components. You can control when useEffect runs by specifying dependencies, making it versatile for various lifecycle needs.
What are some performance optimization techniques in React?
-To optimize React performance, you can use PureComponent and React.memo to prevent unnecessary re-renders. Additionally, hooks like useMemo and useCallback help by memoizing values and functions, reducing the need to recompute or recreate them on every render.
What are higher-order components (HOCs) and render props, and how do they help with code reuse in React?
-Higher-order components (HOCs) and render props are patterns that allow logic to be shared between components. HOCs are functions that take a component and return a new component with additional functionality. Render props involve passing a function as a prop to a component, enabling dynamic behavior and reusability.
Outlines
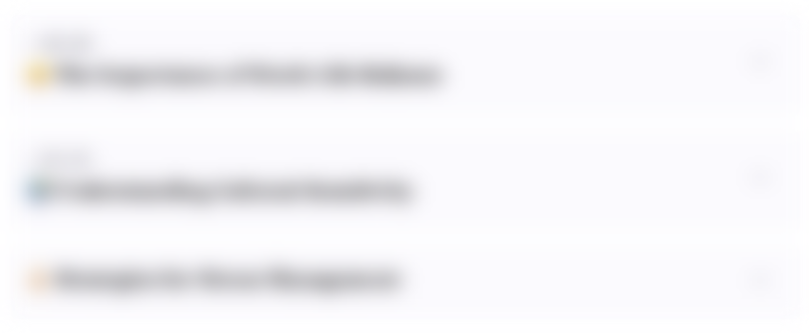
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
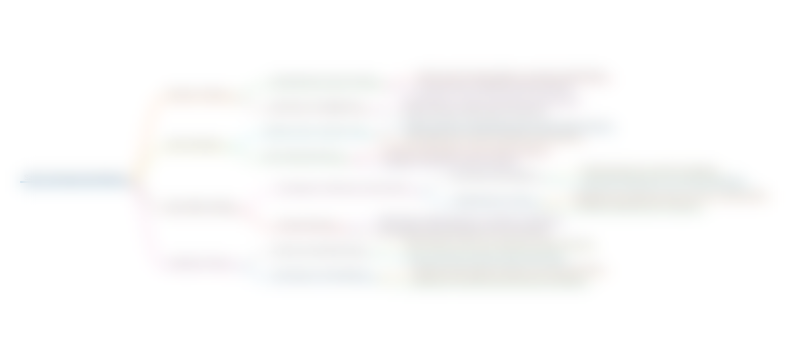
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
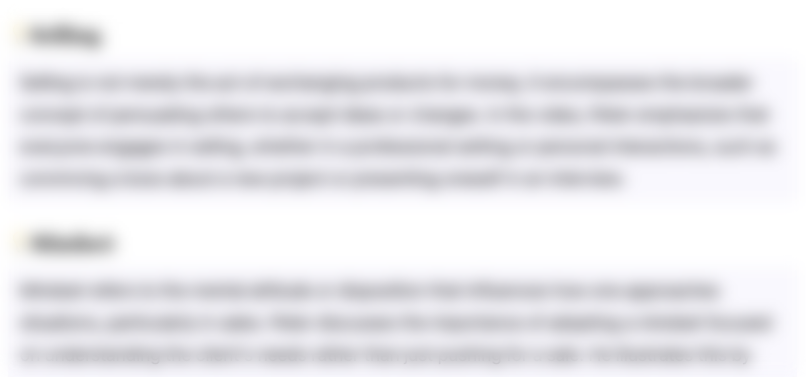
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
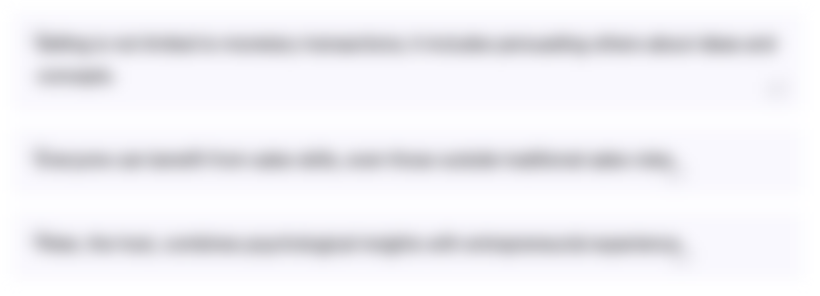
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
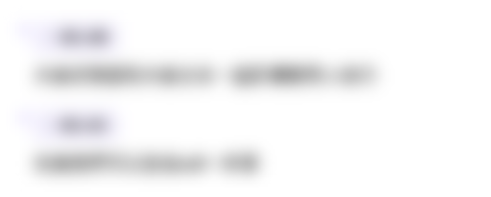
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
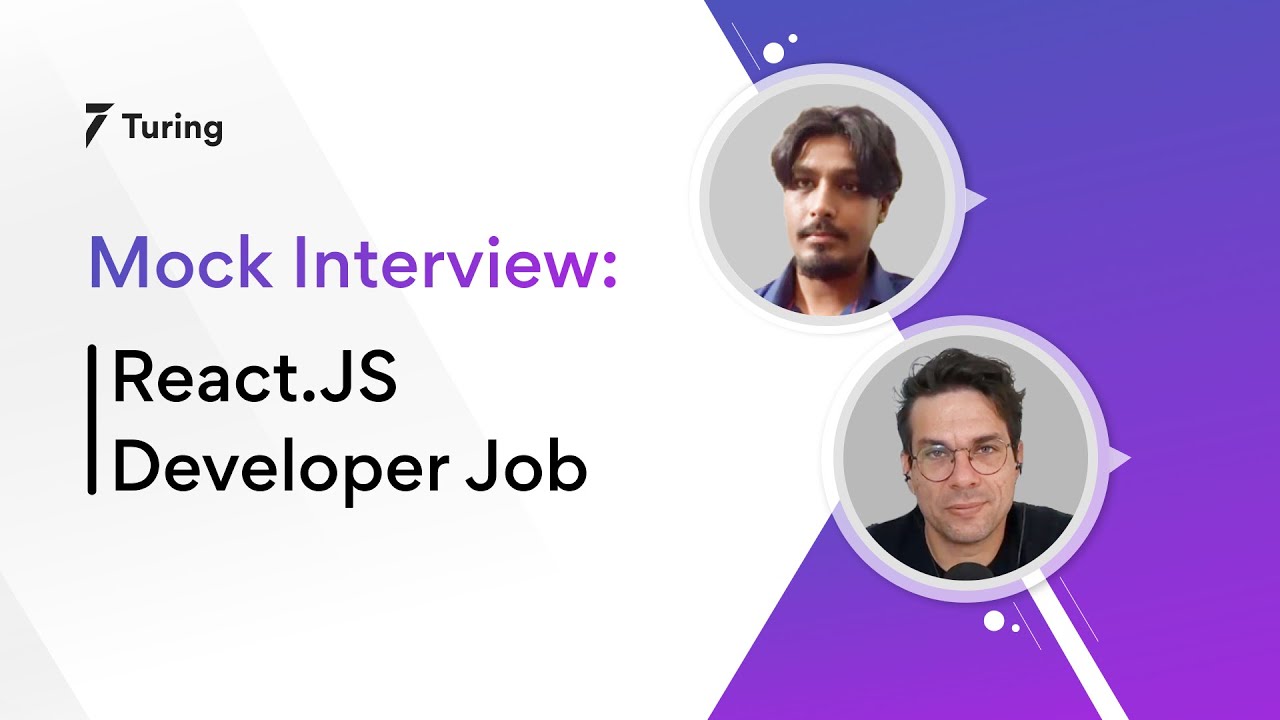
React.JS Mock Interview | Interview Questions for Senior React.JS Developers
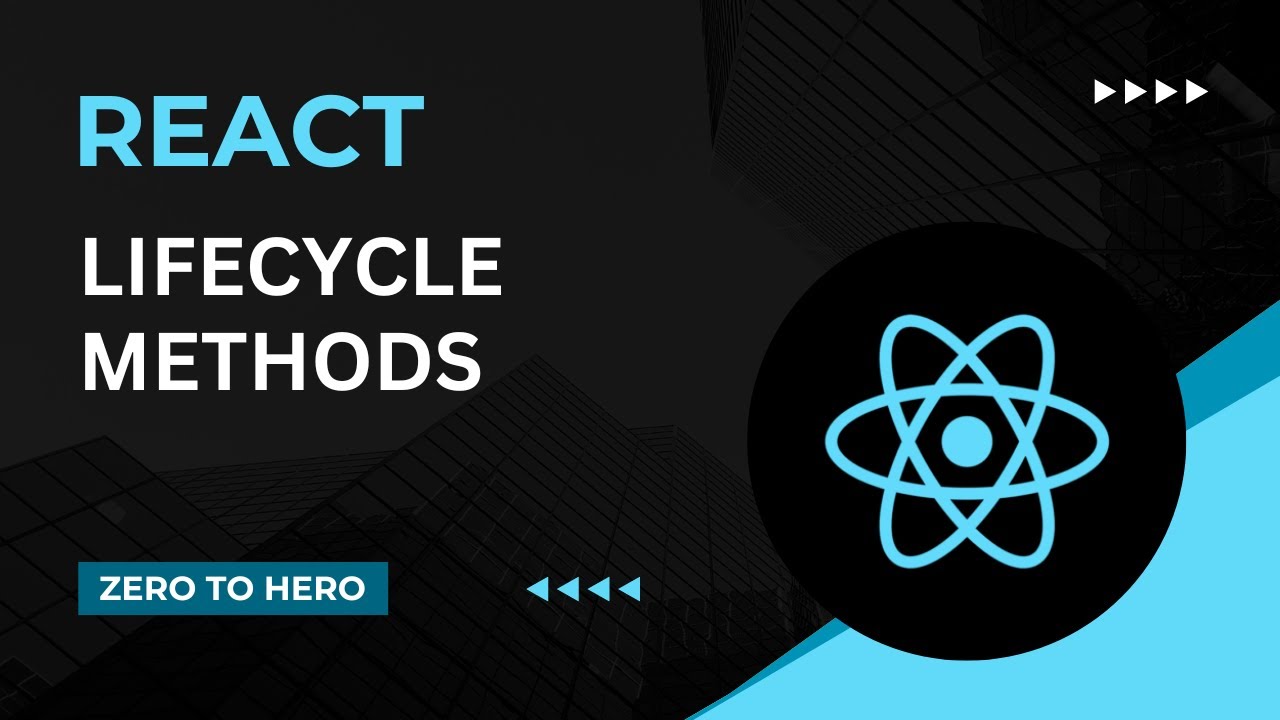
Life Cycle Methods | Mastering React: An In-Depth Zero to Hero Video Series
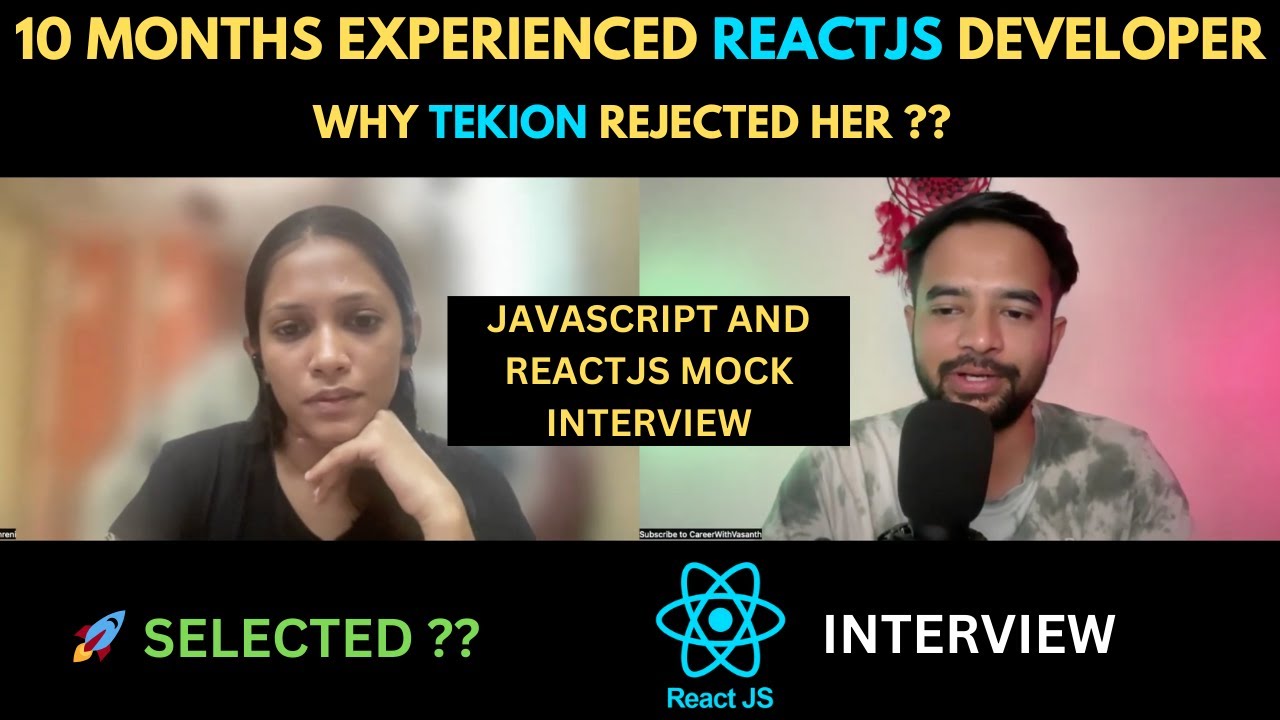
10-Months Experienced @React Engineer's Mock Interview β Why she Rejected in Tekion Interview
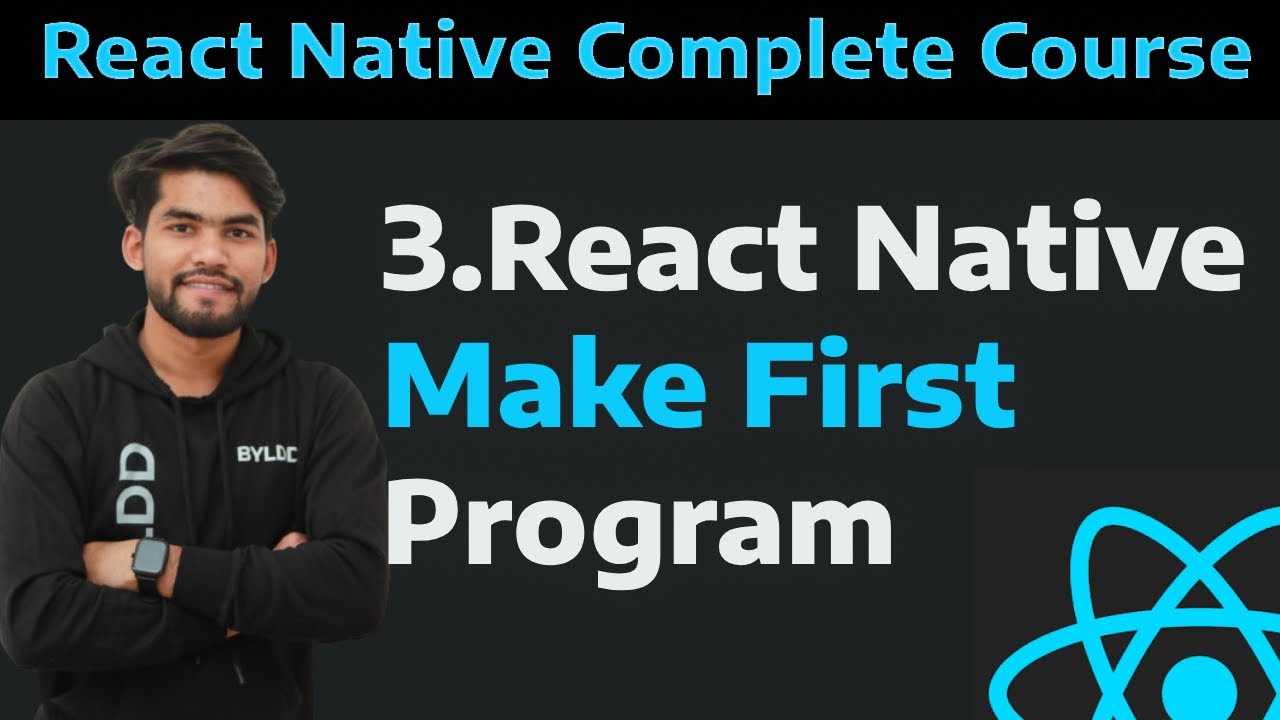
[3] React Native make your first program| Build your first program in React Native tutorials
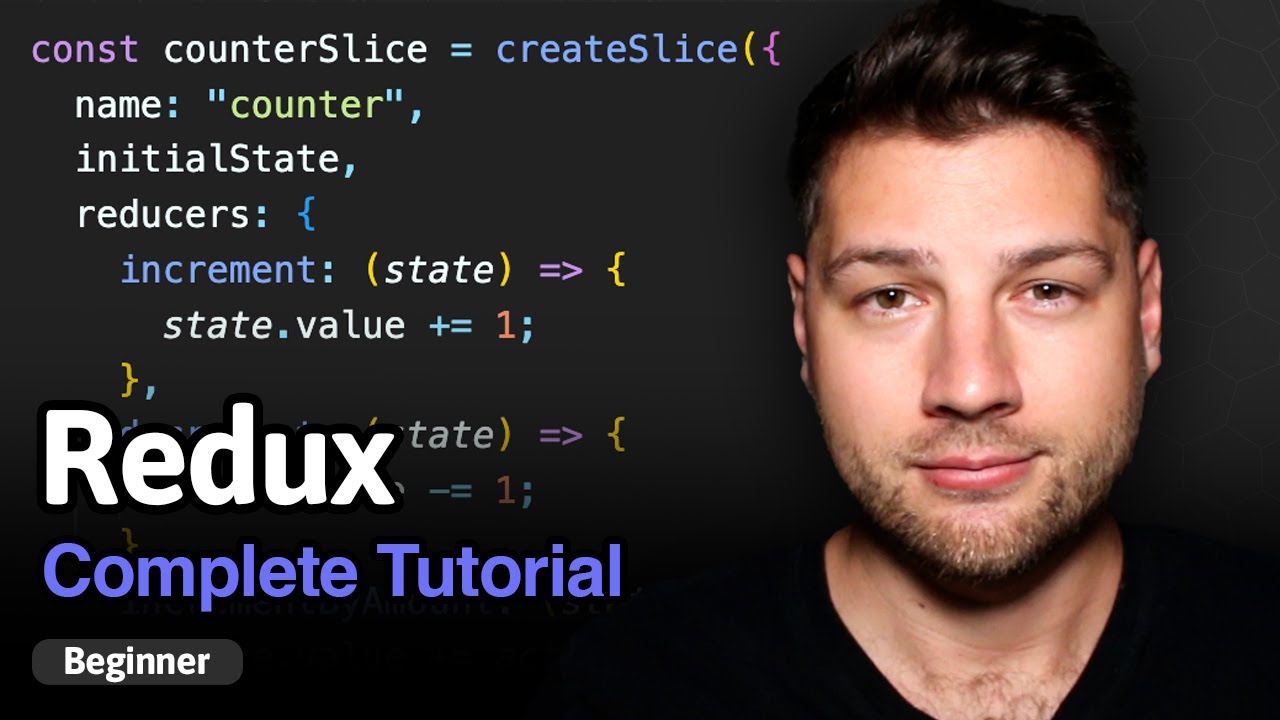
Redux - Complete Tutorial (with Redux Toolkit)
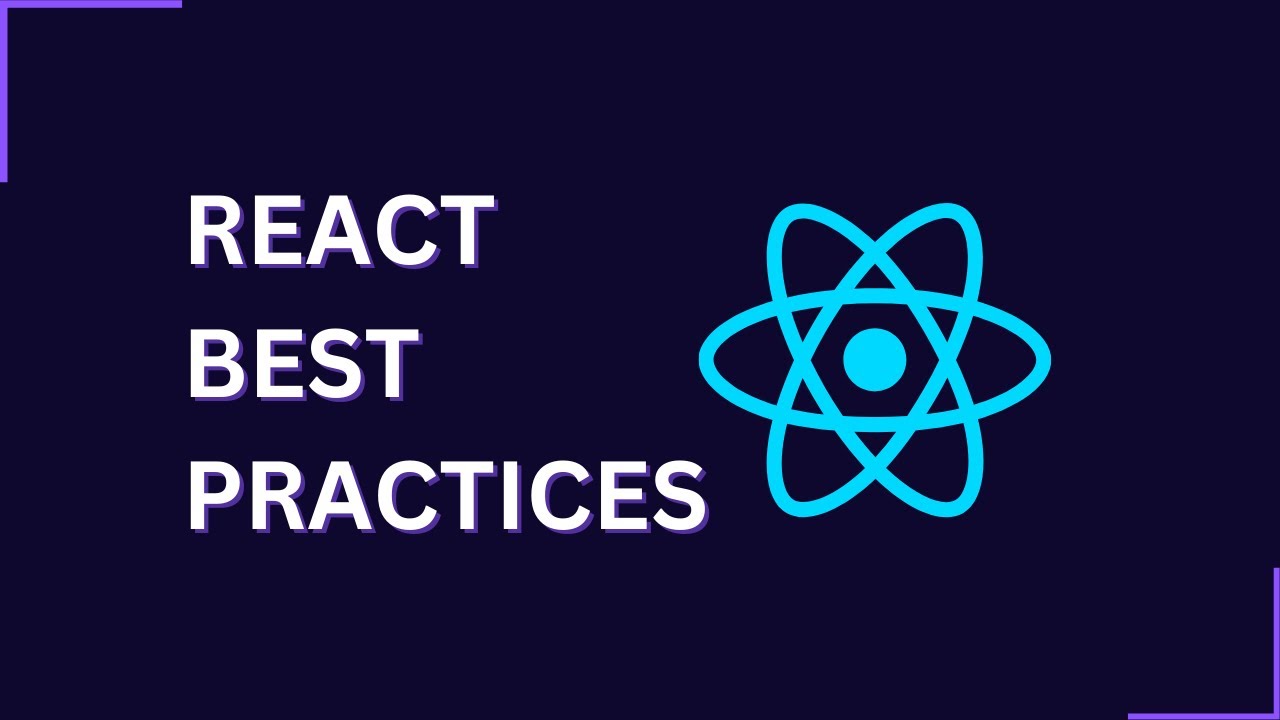
React Like a Pro: React Best Practices
5.0 / 5 (0 votes)