The mystery of React key: how to write performant lists
Summary
TLDRIn this video, the importance of the 'key' attribute in React is explored, emphasizing how it helps React efficiently identify and manage list components during re-renders. The script explains the impact of using improper keys, like random values or array indexes, and their consequences on performance and state. Best practices are discussed, including using unique, stable keys (such as IDs) and leveraging techniques like React.memo for optimization. Additionally, the video covers using keys to reset component state in specific use cases, offering a comprehensive guide to improving React list rendering and state management.
Takeaways
- 😀 React uses the `key` attribute to identify components uniquely in a list, ensuring proper re-rendering and updating of the UI.
- 😀 Without a `key`, React relies on the order of components in an array to differentiate them, which is less efficient and can lead to unnecessary re-renders.
- 😀 A `key` attribute helps React determine which component has changed, been added, or removed from the list, optimizing performance.
- 😀 If a component's `key` changes, React treats it as a completely new component, unmounting the old one and remounting the new one, which can be inefficient.
- 😀 Using random values for `key` leads to performance issues because React will re-render the entire list every time the keys change.
- 😀 To avoid unnecessary re-renders, the `key` attribute should be unique and stable across re-renders, ideally based on unique identifiers like an ID.
- 😀 When using array indexes as `key`, it's safe only if the array is static (no additions, removals, or reordering) or if there are no local state or memoization concerns.
- 😀 React's `key` does not help prevent re-renders on its own. To optimize, you should combine it with techniques like `React.memo` for component memoization.
- 😀 Changing an array item's position or removing an item can cause problems with array index keys, as it might lead to incorrect updates to component state.
- 😀 The `key` attribute can also be used on any component to reset its state, particularly useful for uncontrolled components like forms, but this can have performance trade-offs.
- 😀 Using `key` for state reset forces the component to unmount and remount, triggering any necessary side effects like data fetching, which may negatively impact performance.
Q & A
What is the primary purpose of the key attribute in React?
-The key attribute in React helps identify components in a list, allowing React to track changes, additions, and removals efficiently. It enables React to optimize re-renders by determining which component has changed, which is new, and which should be removed.
What happens if you don't use a key or use an unstable key in React lists?
-If you don't use a key or use an unstable key (e.g., a random value), React may re-render the entire list unnecessarily. This can cause performance issues, including slower mounts and loss of component state.
Why is using the array index as a key considered a bad practice?
-Using array indexes as keys is generally considered a bad practice because it can lead to issues when items are added, removed, or rearranged. This is because React might confuse the updated list with unchanged components, causing unnecessary re-renders or loss of component state.
When is it safe to use the array index as a key in React?
-It's safe to use the array index as a key if the list is static, meaning items are not added, removed, or rearranged. It is also acceptable if the components in the list do not maintain local state or memoization is not required.
What is the role of the key attribute when adding or removing items from a list?
-When items are added or removed from a list, React uses the key attribute to identify which component needs to be mounted, unmounted, or updated. It ensures that only the affected components are re-rendered, while the others remain unchanged.
What happens when you change the key of an existing component in a list without changing its data?
-Changing the key of an existing component without altering its data causes React to treat the component as a new one. It will unmount the old component and mount a new one, resulting in unnecessary re-renders and potentially losing any component state.
How can you prevent unnecessary re-renders in a list of components in React?
-To prevent unnecessary re-renders in a list, you can use React.memo to memoize the components. This ensures that components are only re-rendered when their props change, not when the parent list re-renders.
How does React.memo work, and when should you use it?
-React.memo is a higher-order component that prevents a component from re-rendering unless its props change. It should be used when you have static components in a list or when the props passed to a component rarely change, helping improve performance by avoiding unnecessary renders.
What are the drawbacks of using React.memo incorrectly in a list of components?
-The most common issue with React.memo is failing to properly memoize non-primitive props (e.g., objects or functions), which can lead to unnecessary re-renders. Additionally, if the key attribute is not stable, React.memo will not prevent re-renders.
Can the key attribute be used to reset a component's state in React, and how does it work?
-Yes, the key attribute can be used to reset a component's state. By changing the key, React unmounts the old component and mounts a new one, effectively resetting the component's state. This technique is often used with uncontrolled components but can be performance-heavy if overused.
Outlines
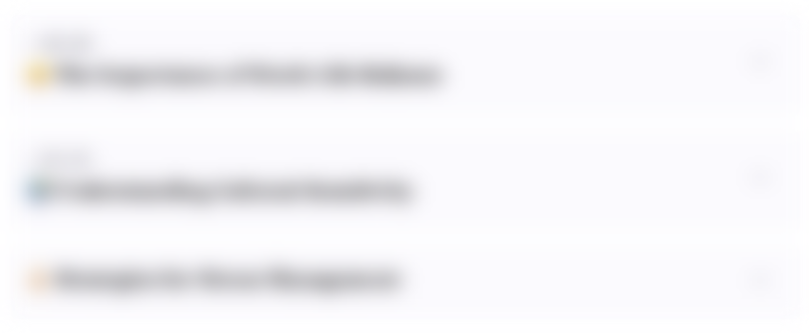
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
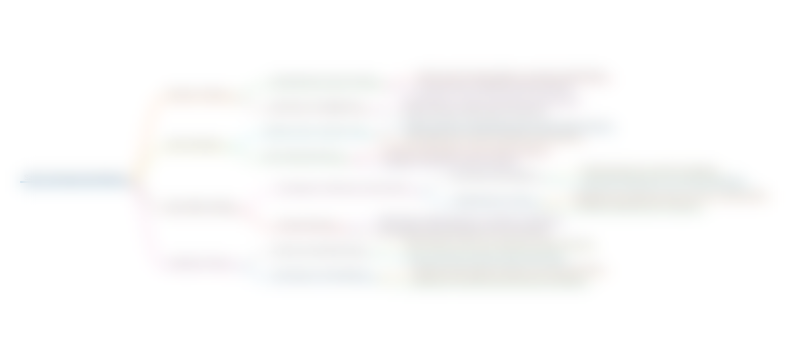
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
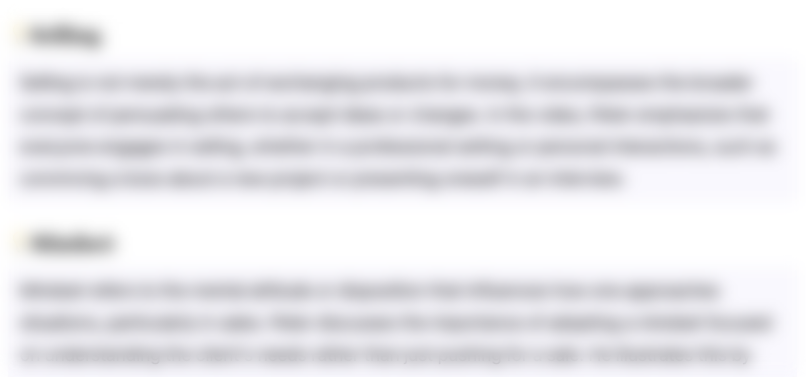
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
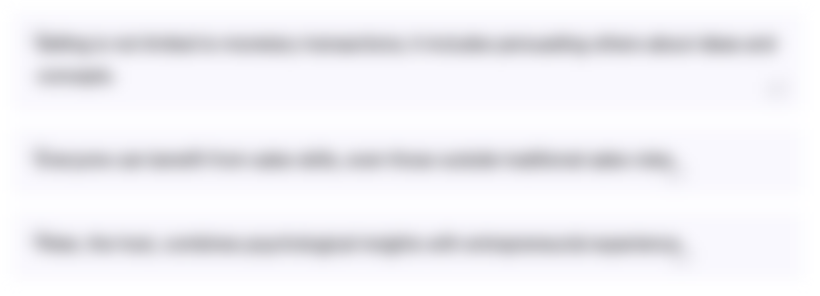
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
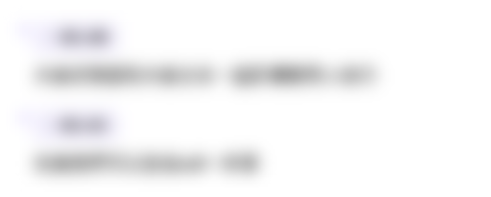
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)