Add a ViewModel with @EnvironmentObject in SwiftUI | Todo List #3
Summary
TLDR在这段视频中,Nick 通过构建视图模型(ViewModel),向观众展示了如何在他们的应用程序中实现 MVVM(Model-View-ViewModel)架构。他首先解释了 ViewModel 在连接视图(UI 组件)和模型(数据点)之间的中心作用,然后逐步引导观众创建用于添加、读取、更新和删除数据的 ViewModel 类。Nick 还展示了如何在 SwiftUI 中实现列表的删除和移动功能,并将这些功能逻辑移至 ViewModel,以保持视图的简洁性。此外,他还介绍了如何通过环境对象在整个应用中共享 ViewModel,以及如何使用 `@StateObject` 属性包装器来观察 ViewModel 的变化。最后,Nick 通过添加新项、检查文本长度以及切换完成状态等功能,展示了如何在 ViewModel 中封装 CRUD(创建、读取、更新、删除)操作,强调了这种实践对于保持代码清晰和高效的重要性。
Takeaways
- 📝 创建视图模型(ViewModel)是MVVM架构中的核心组件,它连接视图(View)和数据模型(Model)。
- 🔄 ViewModel负责管理视图所需的数据,并通过模型来处理数据逻辑,如创建、读取、更新和删除数据。
- 🎬 通过SwiftUI的ForEach循环,可以使用onDelete和onMove来实现列表项的删除和移动。
- 🚀 将数据逻辑移动到ViewModel中,可以使得视图层(View)更加简洁,只负责UI界面的展示。
- 📚 ViewModel使用`@Published`和`@StateObject`来确保数据变化时,视图能够自动更新。
- 📦 通过创建环境对象(EnvironmentObject),可以在App的不同视图中共享ViewModel。
- 🔗 使用`firstIndex(where:)`来查找数组中特定元素的索引,这是更新数据时常用的方法。
- 🔧 在ItemModel中实现`updateCompletion`函数,用于更新项的状态,保持了数据模型的不可变性。
- 🛠️ 遵循CRUD原则(创建、读取、更新、删除),在ViewModel中实现数据的基本操作。
- 🔄 使用`withAnimation`为视图的交互添加动画效果,提升用户体验。
- 📛 通过`Environment`和`presentationMode`来控制视图的导航和状态。
- 🧩 在视图模型中封装所有数据逻辑,使得视图只关注UI展示,遵循MVVM的设计原则。
Q & A
在Swift UI中,ViewModel的作用是什么?
-ViewModel是MVVM架构中的核心组件,它连接视图(View)和数据模型(Model)。ViewModel负责存储和管理与视图相关的数据,以及包含创建、读取、更新和删除数据的逻辑。
为什么在MVVM架构中需要分离视图和数据逻辑?
-分离视图和数据逻辑可以使得代码更加模块化,易于维护和重用。视图仅负责UI展示,而数据逻辑则由ViewModel处理,这样可以提高代码的可读性和可测试性。
如何在Swift UI中实现删除列表项的功能?
-在Swift UI中,可以通过为List中的每个Item添加一个On Delete手势,来实现删除功能。在On Delete的perform方法中,调用删除数据的函数,并传入相应的Index Set作为参数。
如何实现在Swift UI列表中移动项的功能?
-在Swift UI中,可以通过为List中的每个Item添加一个On Move手势来实现移动功能。在On Move的perform方法中,调用移动数据的函数,并传入源索引集(Index Set)和目标偏移量(Integer)作为参数。
为什么需要将数据和逻辑移动到ViewModel中?
-将数据和逻辑移动到ViewModel中是为了遵循MVVM的设计原则,使得视图(View)只包含UI相关的代码,而所有的数据和业务逻辑都封装在ViewModel中,这样可以提高应用的架构清晰度和可维护性。
如何在Swift UI中创建一个新的ViewModel?
-在Swift UI中创建一个新的ViewModel,首先需要在项目导航器中创建一个新的Swift文件,并命名为ViewModel的名称,如`ListViewModel`。然后在该文件中定义一个类,使其符合`ObservableObject`协议,以便视图可以观察到ViewModel的变化。
如何确保ViewModel在整个应用中被使用?
-为了确保ViewModel在整个应用中被使用,可以将其作为环境对象(EnvironmentObject)嵌入到App的根视图中。这样,所有继承自根视图的子视图都能访问到这个ViewModel。
如何在添加视图中实现添加新项的功能?
-在添加视图中,可以通过创建一个按钮,并为其添加一个点击事件处理函数来实现添加新项的功能。在该函数中,首先检查输入的文本是否符合要求(如长度至少为3个字符),然后通过ViewModel将新项添加到数据数组中,并返回到前一个视图。
如何实现在列表项被点击时切换其完成状态?
-在列表项的ForEach循环中,可以为每个列表项添加一个On Tap Gesture手势。在该手势的处理函数中,调用ViewModel中的更新项的函数,并传入被点击的项,ViewModel会负责切换该项的完成状态。
为什么在更新数据模型时使用不可变结构(Struct)?
-使用不可变结构(Immutable Struct)可以确保数据模型的一致性和安全性。由于所有的属性都是常量(let),它们不能在Struct外部被修改,只能通过Struct提供的函数进行更新,这样可以避免数据不一致的问题。
如何在Swift UI中实现数据的CRUD操作?
-在Swift UI中实现CRUD操作,即创建(Create)、读取(Read)、更新(Update)、删除(Delete)。这通常通过ViewModel来完成,其中会包含相应的函数来处理数据的增加、查询、修改和删除逻辑。
Outlines
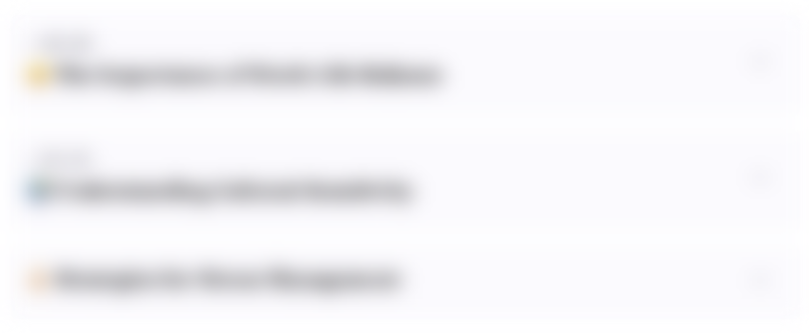
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
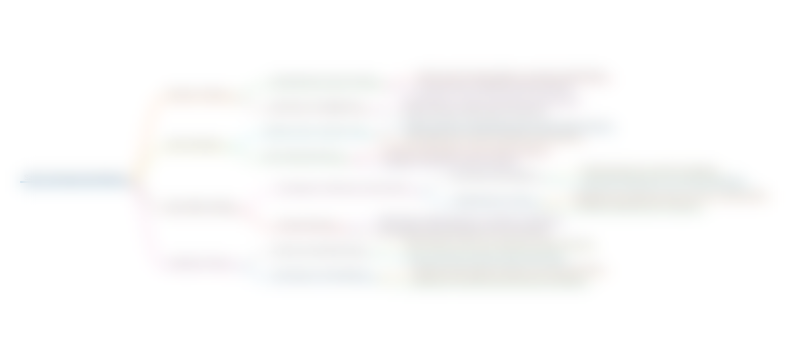
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
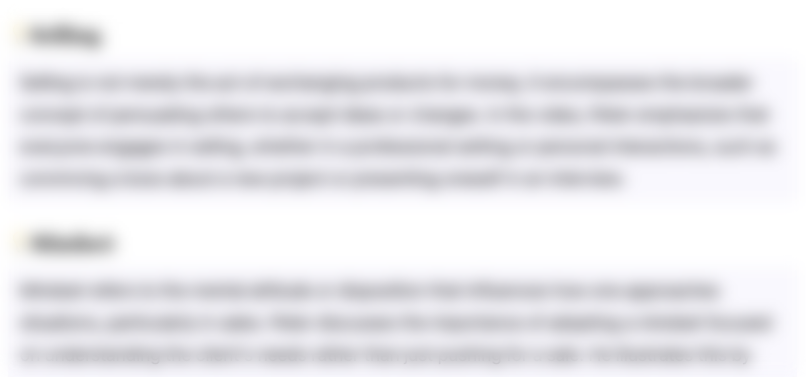
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
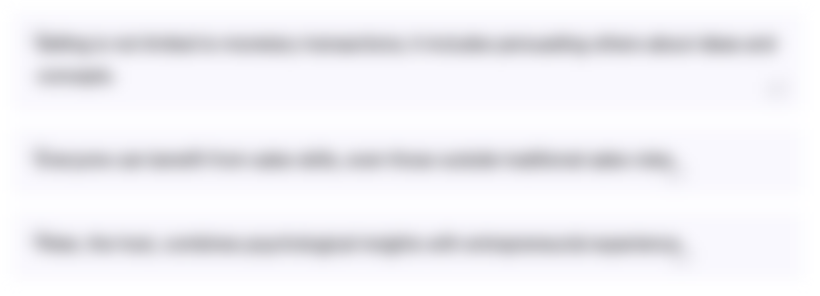
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
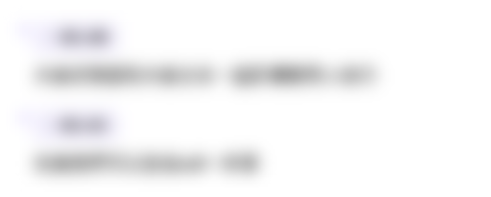
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
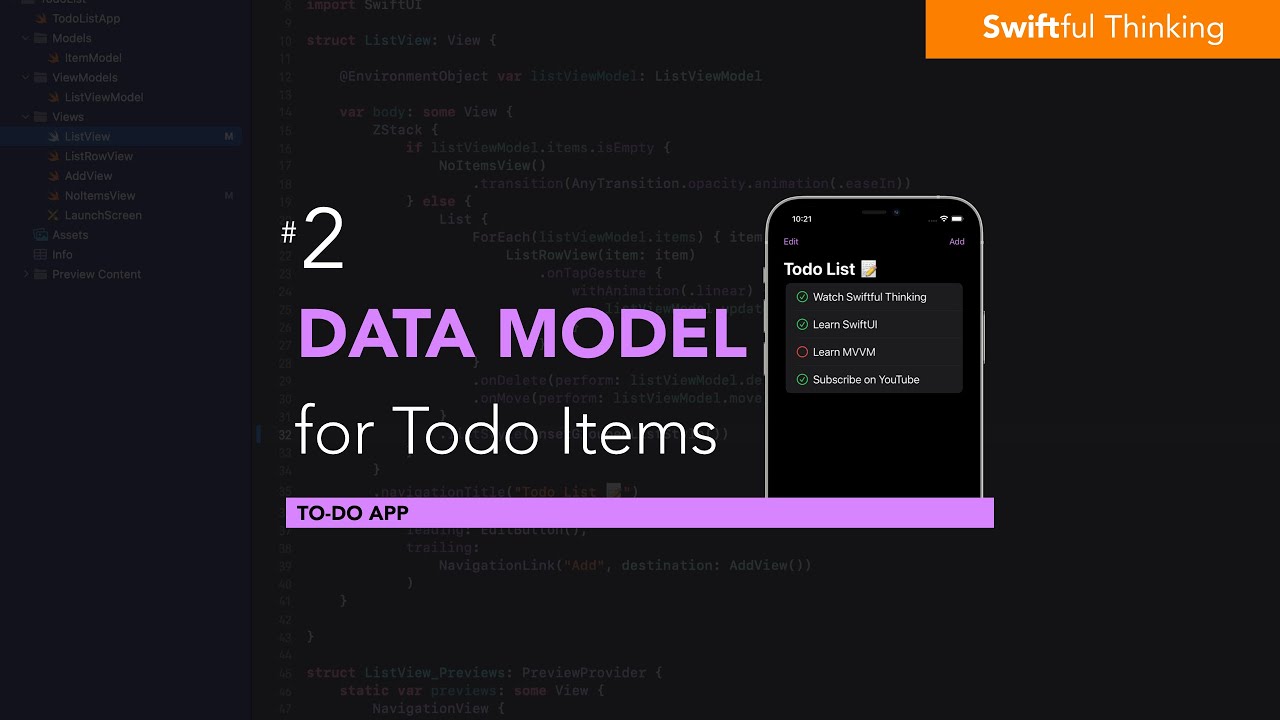
Create a custom data model for Todo items in SwiftUI | Todo List #2
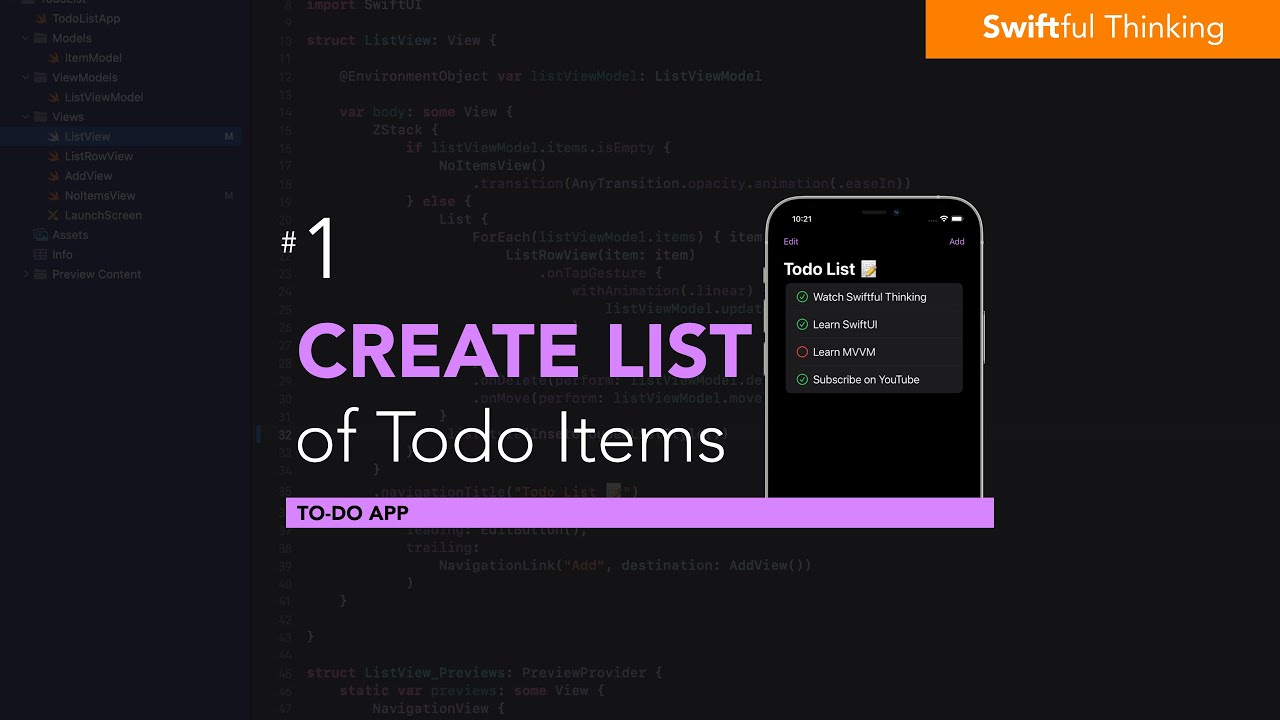
Create a List of Todo items in SwiftUI | Todo List #1
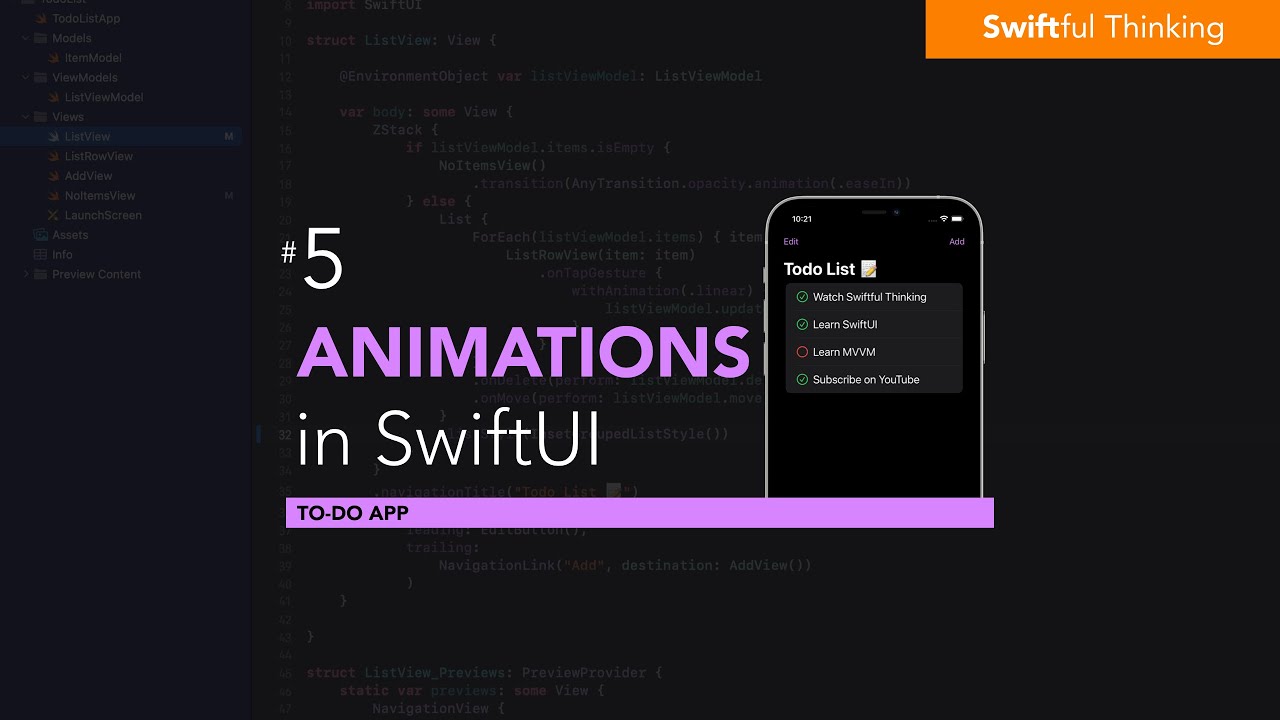
User Experience and Animations in SwiftUI app | Todo List #5
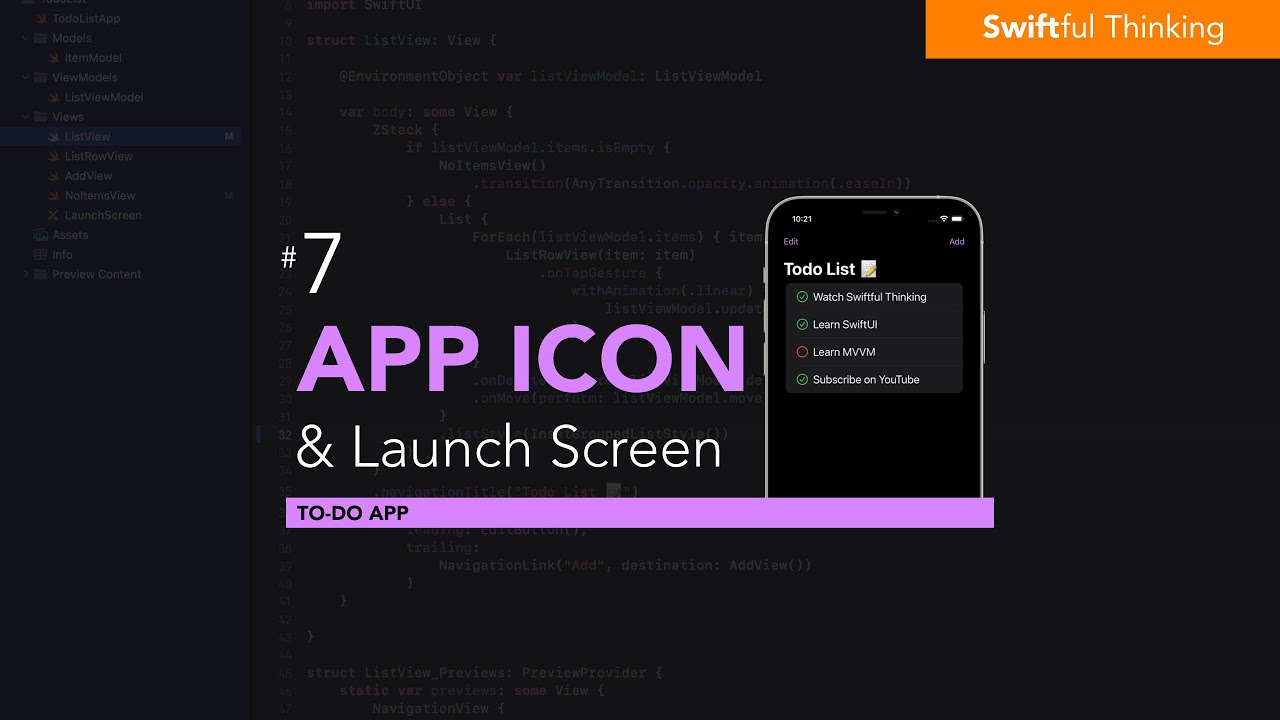
Adding an App Icon and Launch Screen to SwiftUI | Todo List #7
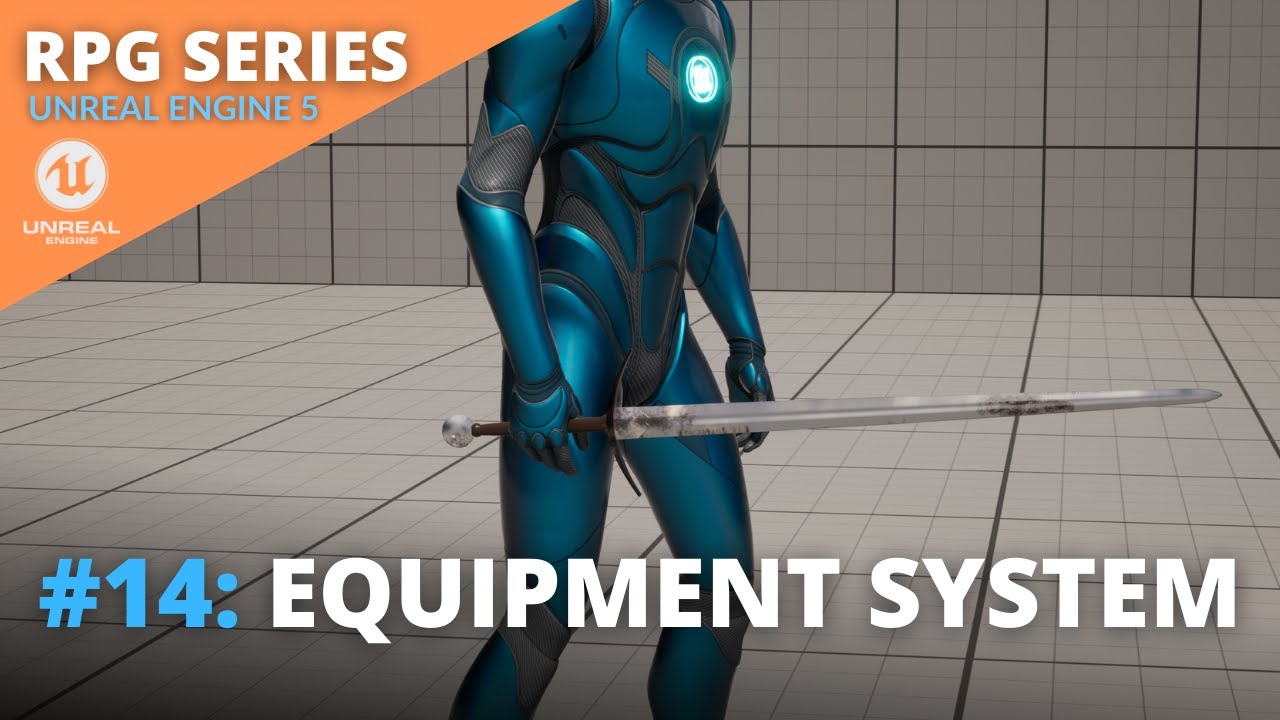
Unreal Engine 5 RPG Tutorial Series - #14: Equipment System
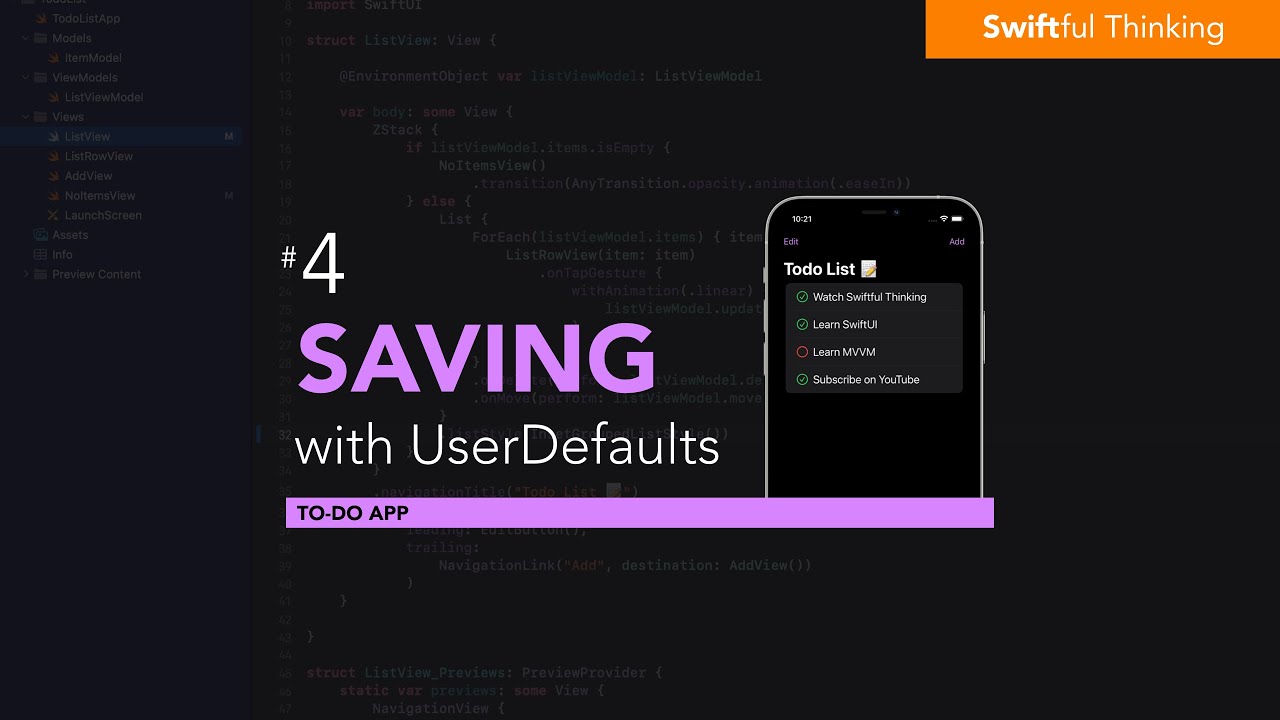
Save and persist data with UserDefaults | Todo List #4
5.0 / 5 (0 votes)