C_07 Variables in C Language | C Programming Tutorials
Summary
TLDRIn this educational video, the concept of variables in the C programming language is introduced as the counterpart to constants. The video explains that variables can change their values during program execution and are symbolic names used to access memory addresses. It outlines the rules for naming variables, emphasizing starting with a letter or underscore, avoiding special characters except for underscores, and not exceeding 31 characters. The importance of case sensitivity and the prohibition of using reserved keywords are highlighted. The video concludes with a simple C program example that demonstrates declaring variables, performing addition, and using the printf function to display the result.
Takeaways
- 📚 Variables in programming are symbolic names associated with memory addresses, allowing programmers to access and manipulate data without using memory addresses directly.
- 🔄 Variables are the opposite of constants; their values can change during program execution, unlike constants, which remain fixed.
- ✍️ Variable names in C must start with a letter (uppercase or lowercase) or an underscore, and may contain letters, digits, or underscores.
- 🚫 Special characters (other than underscores) and spaces are not allowed in variable names, and variable names cannot start with digits.
- 🚫 Keywords and reserved words in C (like 'if', 'else', 'while', etc.) cannot be used as variable names, as they have predefined meanings in the language.
- 🔠 C is a case-sensitive language, meaning variables like 'sum' and 'SUM' are treated as two different variables.
- 📏 Variable names must not exceed 31 characters, and for some C compilers, it's recommended to keep names under 8 characters for compatibility.
- 📝 Variables must be declared with a specific data type (e.g., 'int' for integers) before use in a program.
- ➕ Example C program: A simple addition of two integer variables (a and b) and storing the result in a third variable (c), followed by printing the result.
- 🖨️ To print integer values in C, the format specifier '%d' is used within the printf function.
Q & A
What is a variable in C language?
-A variable in C language is a symbolic name associated with a memory address, which allows the programmer to access and manipulate data stored at that address. The value of a variable can change during the execution of a program.
How are variables different from constants in C language?
-Constants are values that remain unchanged during program execution, while variables are capable of holding values that can change as the program runs.
What are the rules for naming a variable in C?
-Variable names in C must start with an alphabet or an underscore, can contain alphanumeric characters and underscores, cannot contain spaces or special symbols except underscores, must not start with a digit, and should avoid using keywords or reserved words.
Can a variable name in C language start with a digit?
-No, a variable name in C cannot start with a digit. It must begin with an alphabet or an underscore.
What special character is allowed in a variable name?
-The only special character allowed in a variable name is the underscore ('_').
Why is it recommended to use variable names with fewer than eight characters?
-Using fewer than eight characters for a variable name is a good practice because some older C compilers do not accept names longer than eight characters. Additionally, shorter names are easier to write and read, making the code more convenient to work with.
What does it mean that C is a 'case-sensitive' language?
-C is case-sensitive, meaning that it treats uppercase and lowercase letters as distinct. For example, the variable names 'sum' and 'SUM' would be considered two different variables.
How do you declare a variable in C language?
-To declare a variable in C, you must specify its data type followed by the variable name. For example, 'int sum;' declares a variable named 'sum' of type integer.
What is a format specifier in C, and why is it used?
-A format specifier in C is a way to indicate how a specific data type should be printed. For example, '%d' is used to print an integer, '%c' is used for a character, and '%f' is used for a floating-point number.
What will be the output of the following code snippet? ```c int a = 5, b = 7, c; c = a + b; printf("Addition of %d and %d is %d", a, b, c); ```
-The output of this code will be: ``` Addition of 5 and 7 is 12 ```
Outlines
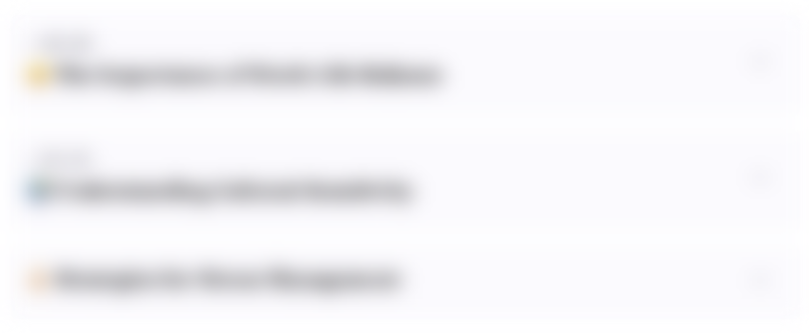
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
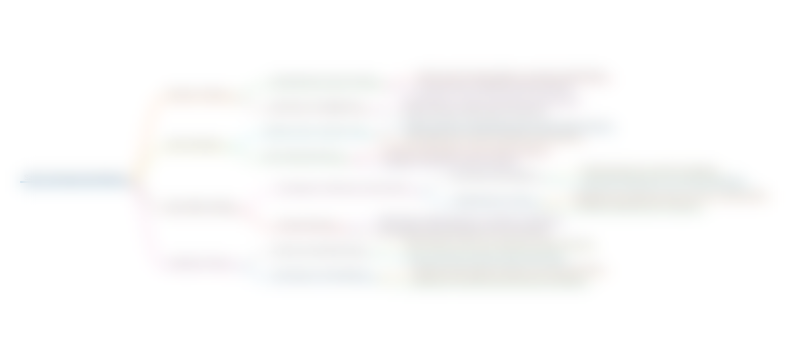
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
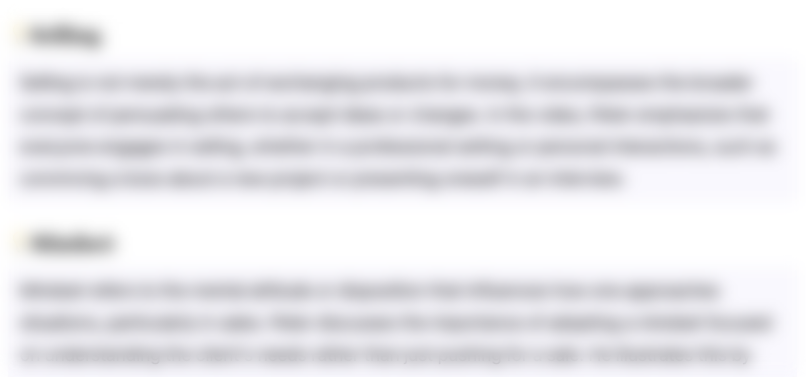
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
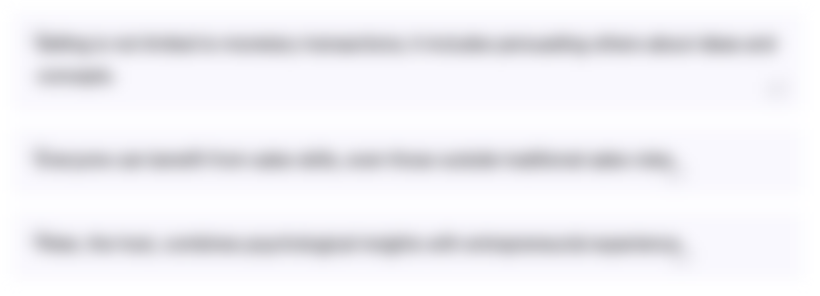
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
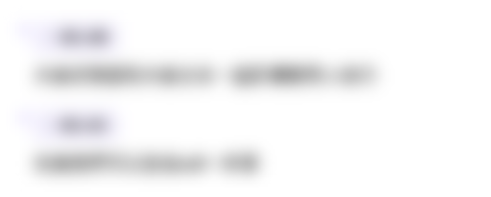
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
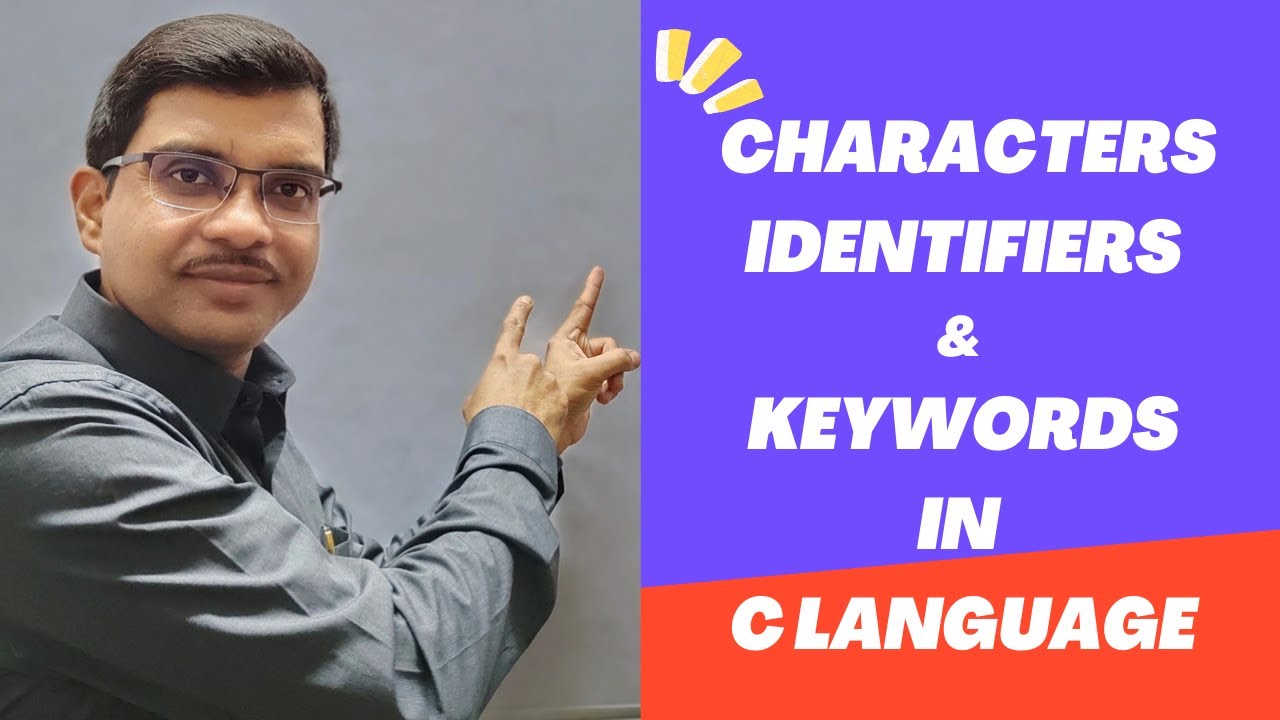
C_08 Characters | Identifiers & Keywords in C Language | C Programming Tutorials
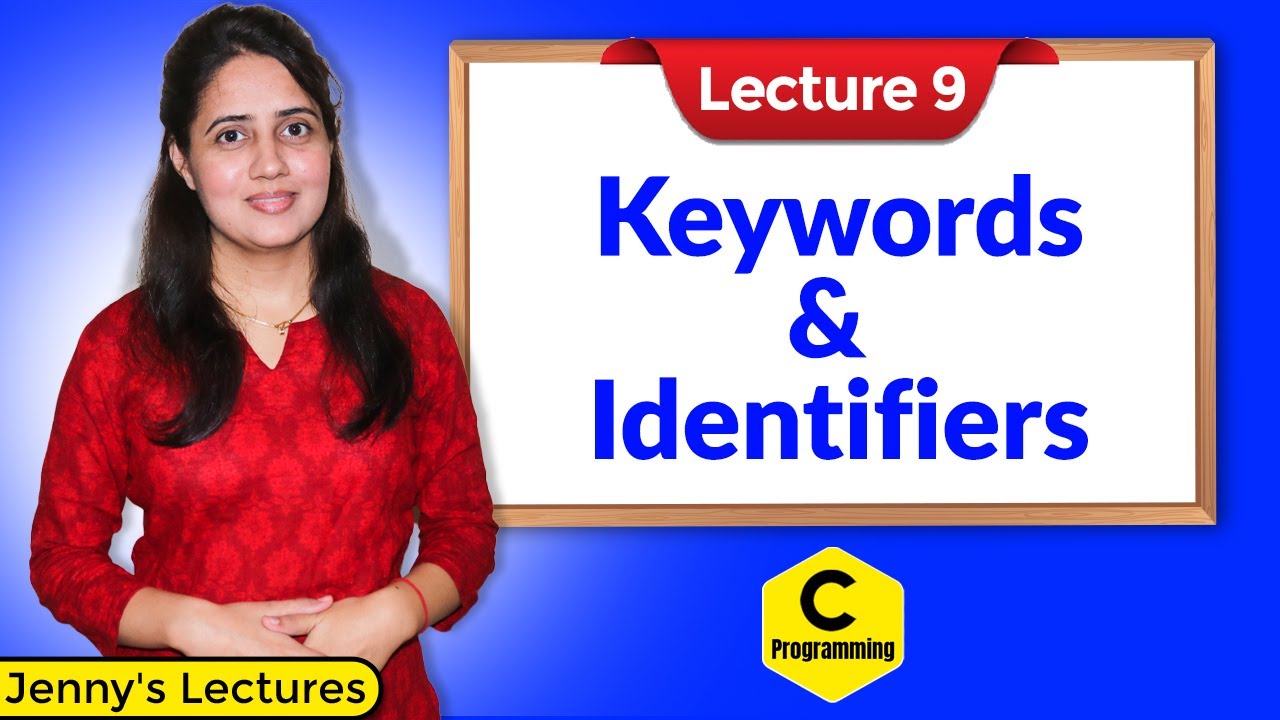
C_09 Keywords and Identifiers | Programming in C
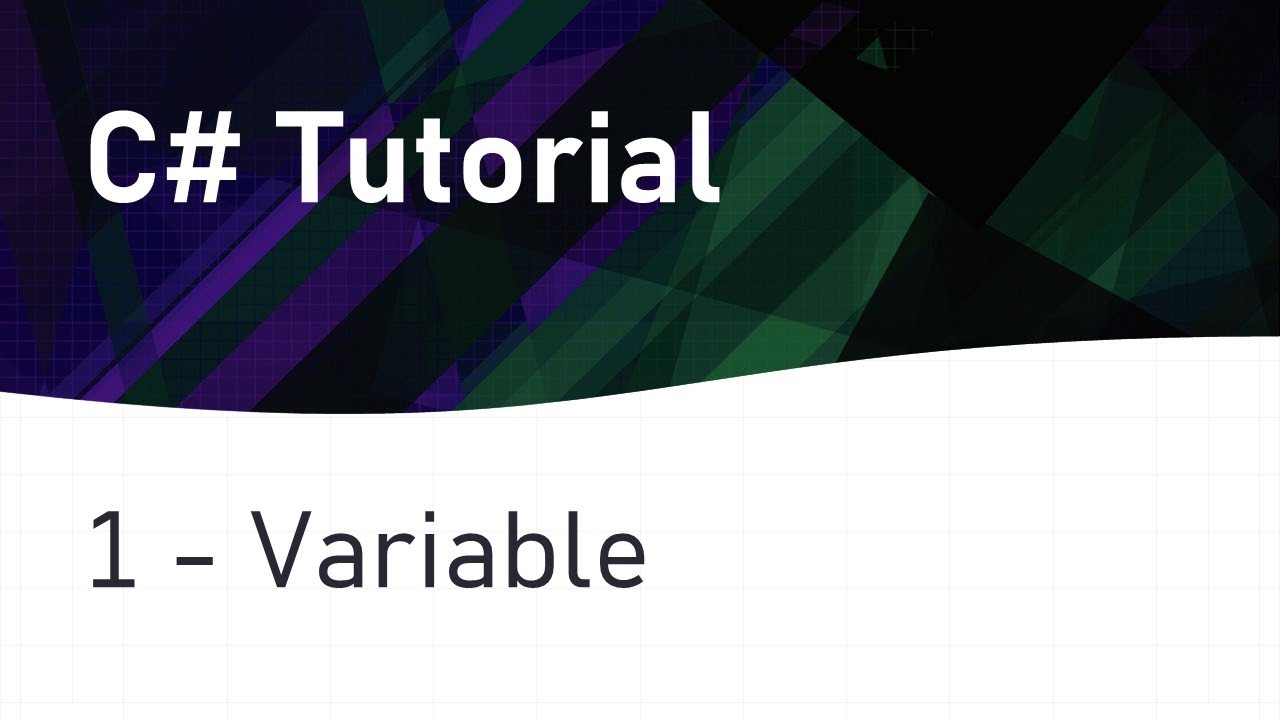
C# Tutorial 1: Variables
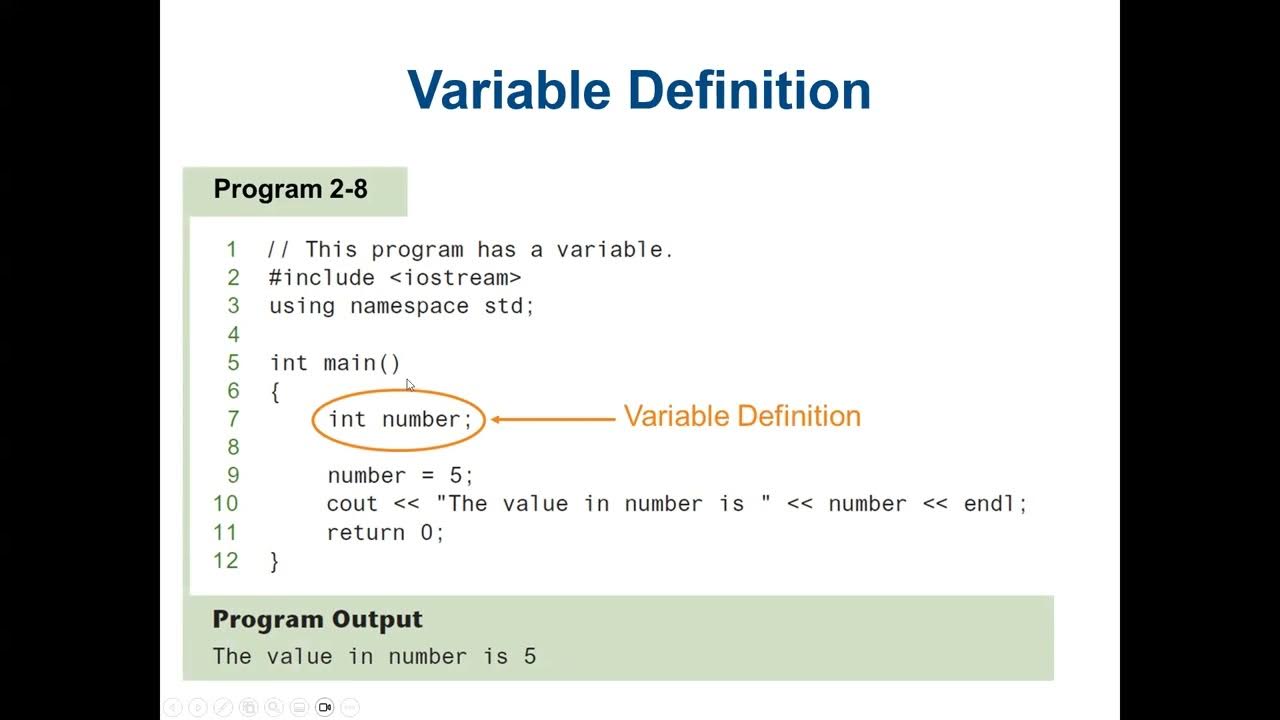
Introduction to C++, The Parts of a C++ Program, Identifier Naming Rules, output statements
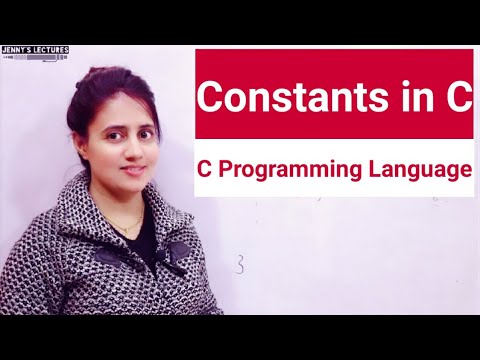
C_07 Constants in C | Types of Constants | Programming in C
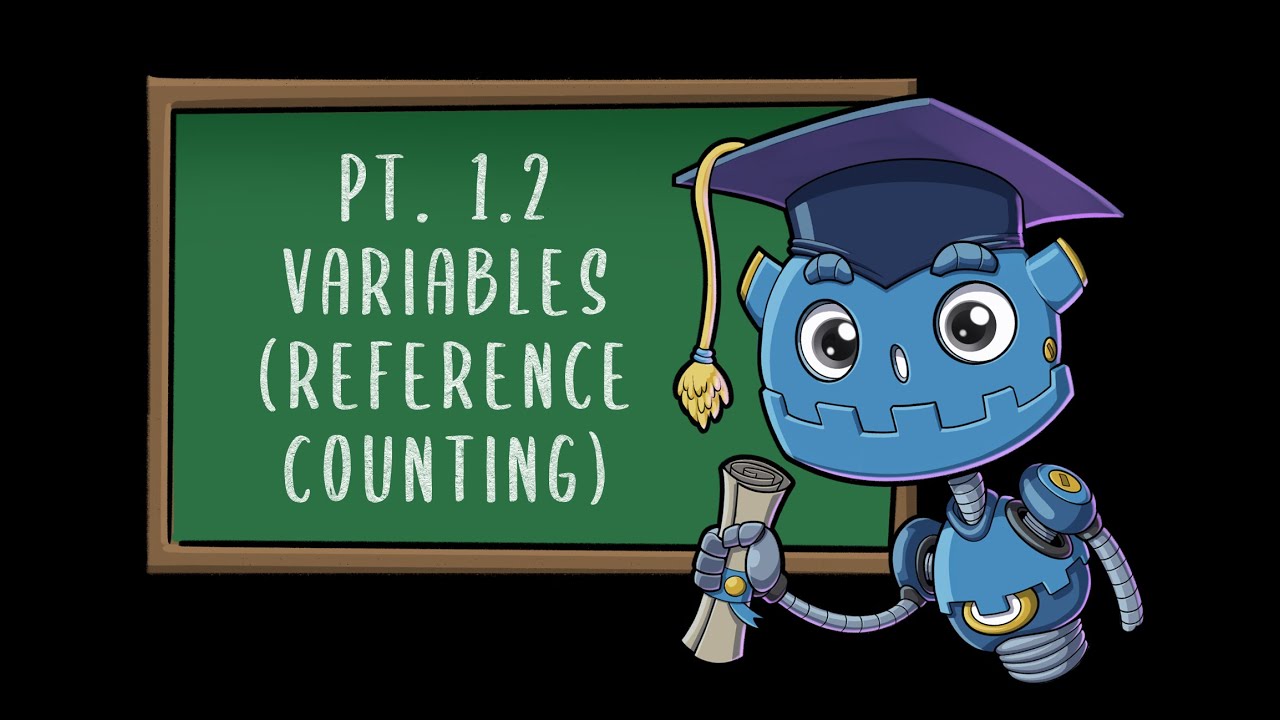
Life Cycle & Reference Counting | Godot GDScript Tutorial | Ep 1.2
5.0 / 5 (0 votes)