Solving My Google Phone Interview Question
Summary
TLDRIn this video, the creator walks through the Google phone interview process, specifically focusing on a coding problem involving trees and depth-first search. The video explains how candidates are required to write code in a Google Doc without IDE support, encouraging proficiency in the coding language. The example problem involves printing a tree structure from a list of parent-child relationships. The solution utilizes a HashMap for depth-first traversal and discusses the time and space complexity of the approach. The video also provides tips for tackling coding interviews, and encourages viewers to practice in similar environments.
Takeaways
- ๐ฑ The video discusses the Google phone interview process and coding challenges.
- ๐ A link to the full Google interview experience and Amazon phone interview is provided in the description.
- ๐ During the Google phone interview, candidates code in a Google Doc without code completion or syntax error checking.
- ๐ก It is recommended to practice writing code in Google Docs before the interview to get familiar with coding without an IDE.
- ๐ณ The coding prompt provided involves writing a Java function to print a tree based on a list of parent-child relationships.
- ๐ The solution involves using a depth-first search (DFS) approach to navigate the tree structure.
- ๐ An adjacency list (represented using a HashMap) is used to store the tree relationships, where each parent points to its children.
- ๐ง To find the root of the tree, the candidate can create a set of all children and loop through the keys to identify the root node.
- ๐งฎ The time complexity of the solution is O(n), where n is the number of nodes in the tree, and the space complexity is also O(n).
- โ The provided solution passed the interview, allowing the candidate to advance to the on-site interview round.
Q & A
What is the primary topic discussed in the video?
-The primary topic discussed is the Google phone interview, including how to prepare for it and solve a specific coding problem given during the interview.
What is the first tip provided for preparing for the Google phone interview?
-The first tip is to practice writing code in a Google Doc without code completion or syntax error checking, as the interview environment does not provide these features.
What is the coding problem presented in the Google interview example?
-The coding problem involves writing a Java function called `printTree` that prints a given tree to standard output based on a list of parent-child relationships.
What data structure is recommended for solving the problem in the video?
-The video suggests using an adjacency list, which is represented by a HashMap, where each parent node is a key and the corresponding value is a list of its children.
What approach is used to traverse the tree in the given solution?
-The video uses a Depth-First Search (DFS) approach to traverse the tree, printing each node along the path and keeping track of the level to format the output correctly.
How is the root of the tree determined in the solution?
-The root of the tree is determined by looping through all the parent nodes and checking which one is not a child in the relations. This node is the root since it has no parent.
What challenge did the presenter face when implementing the solution?
-The presenter mentions that the biggest challenge was determining the root of the tree, as an extra set was required to store all the child nodes.
What is the time complexity of the solution provided?
-The time complexity is O(n), where n is the number of nodes in the tree. The algorithm loops through the nodes multiple times, but constants are dropped in Big-O notation.
What is the space complexity of the solution provided?
-The space complexity is also O(n) since the algorithm uses extra space for the adjacency list and the set of child nodes, which both can store up to n elements.
What advice is given for candidates who donโt understand the problem during the interview?
-The video advises candidates to ask questions during the interview to ensure they fully understand the problem before trying to solve it.
Outlines
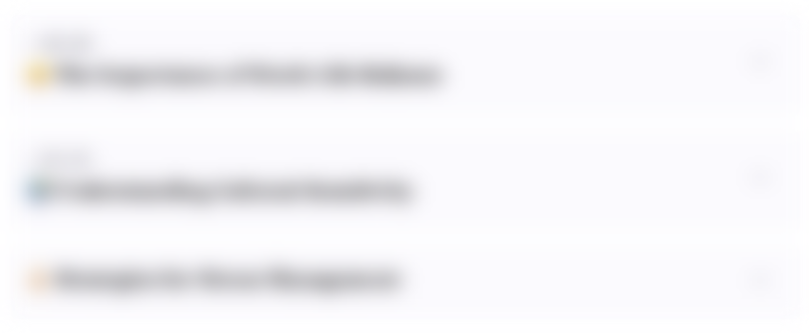
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
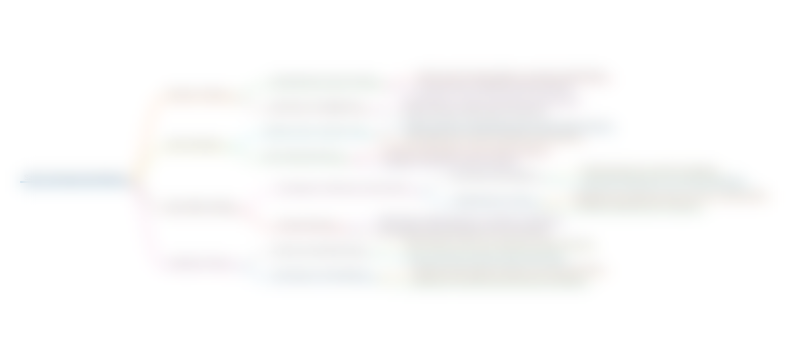
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
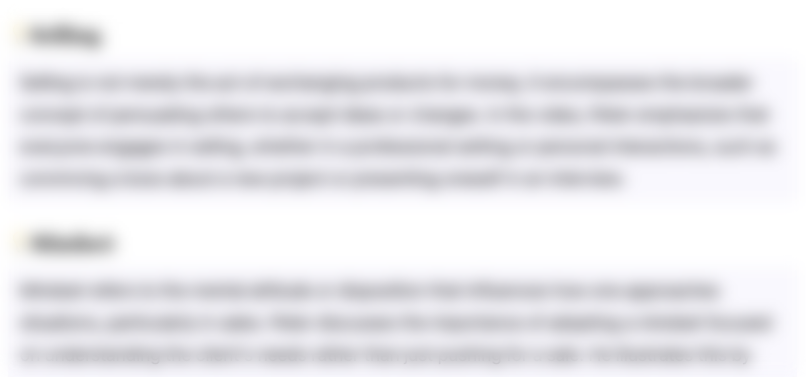
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
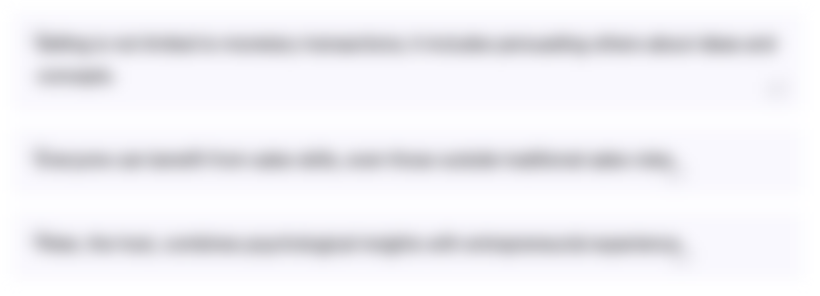
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
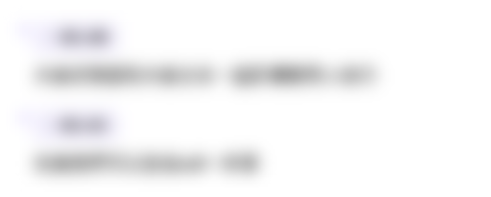
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
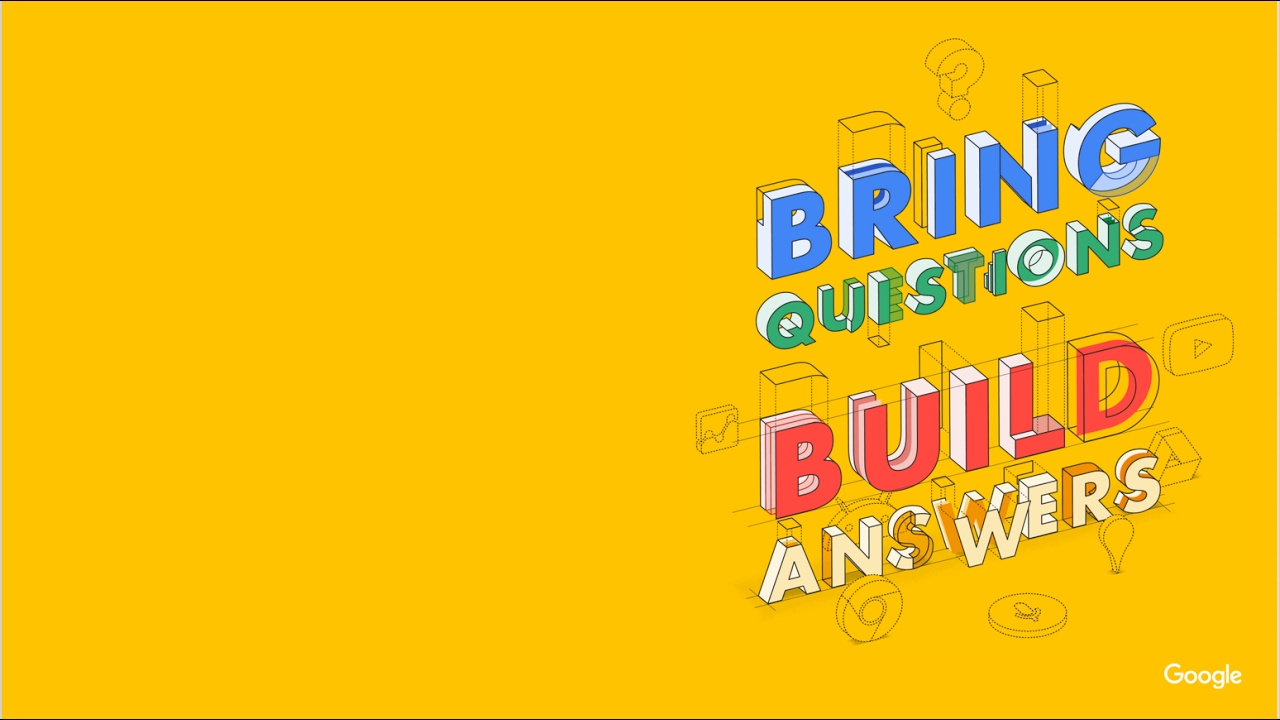
Work at Google: How to Communicate in Technical Interviews & What to do When You're Stumped

Prepare for your Google Interview: Tips and Example General Cognitive Ability Question
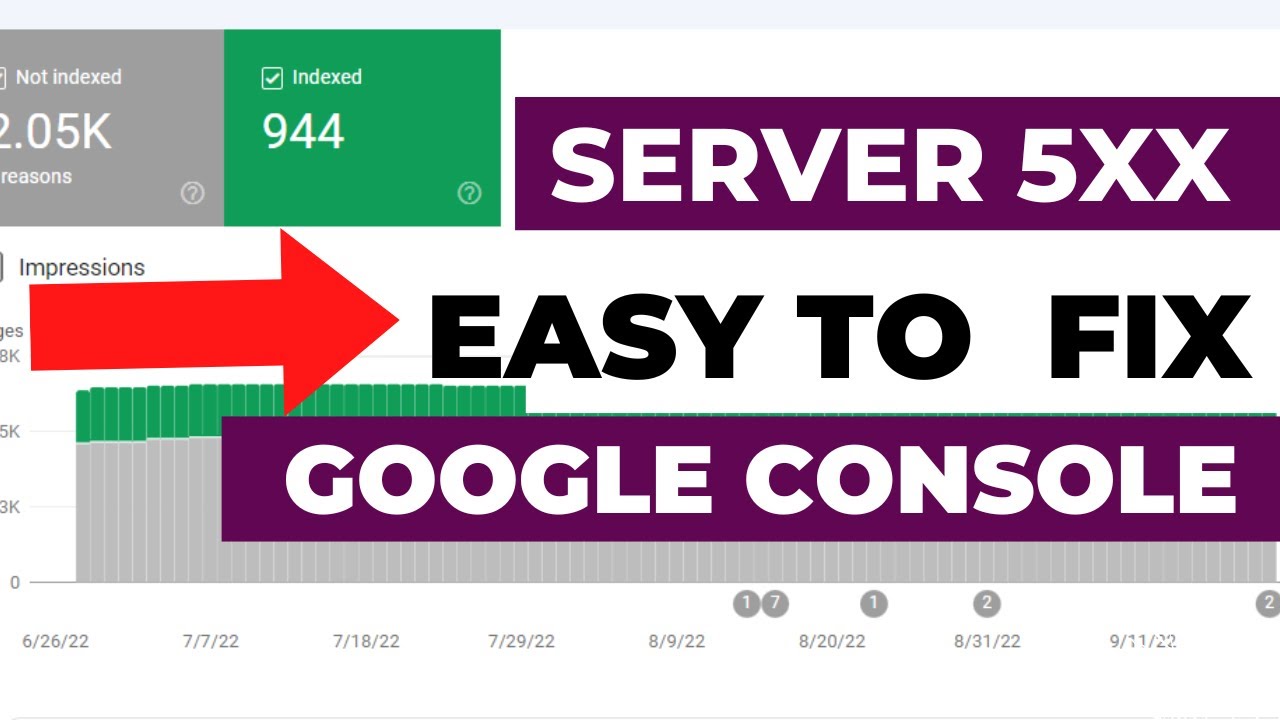
How To Fix " Server error (5xx) " into Your Google Search Console
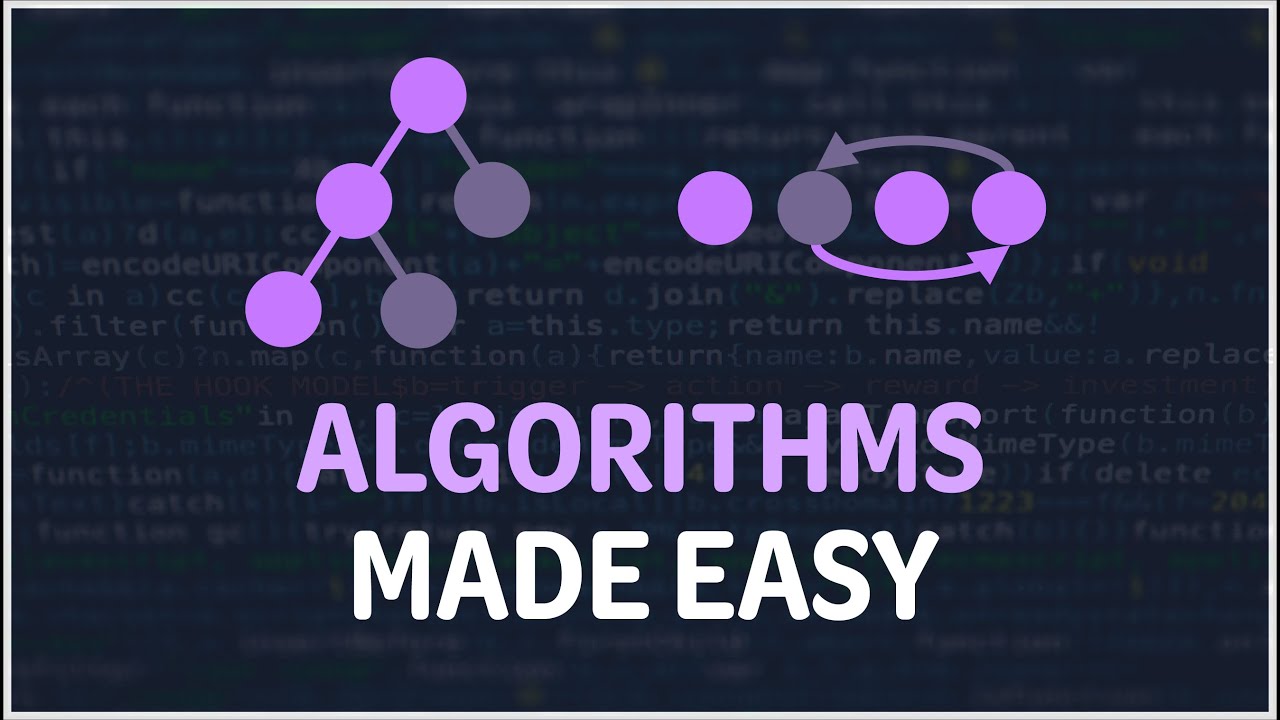
Top 7 Algorithms for Coding Interviews Explained SIMPLY
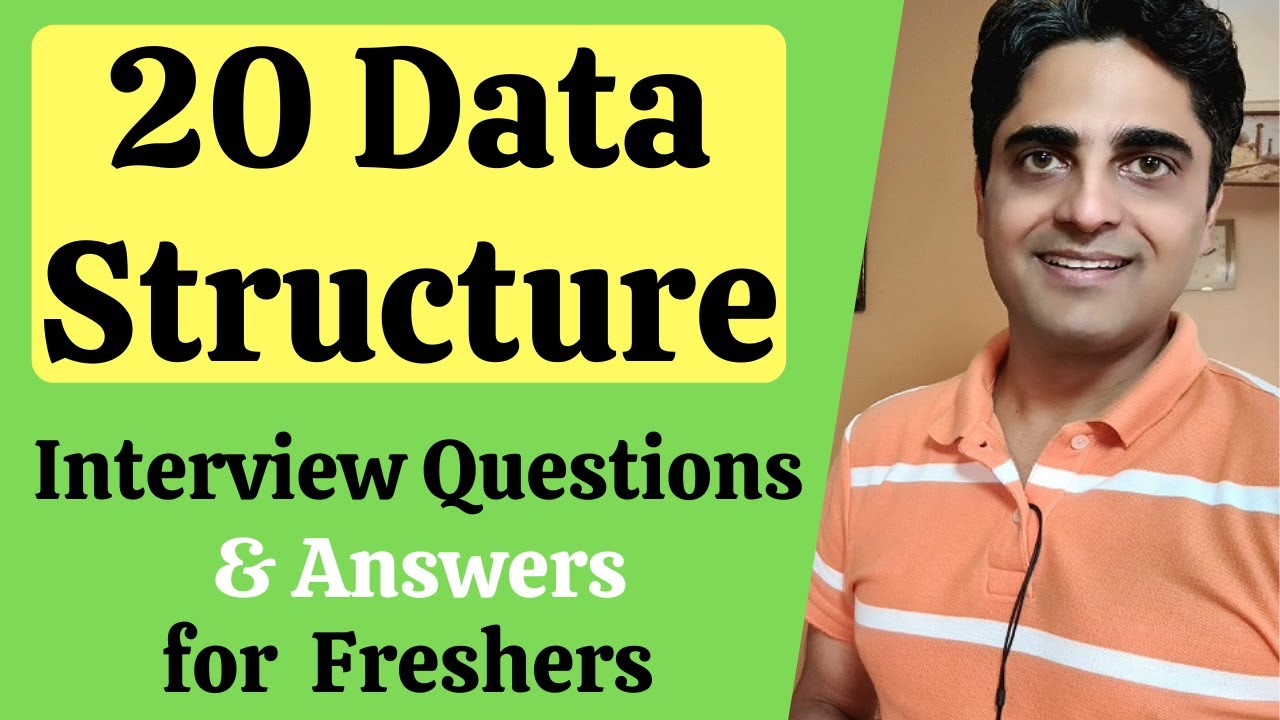
20 Data Structure Interview Questions for Freshers - TCS, Accenture, Infosys, Wipro,Cognizant,Amazon
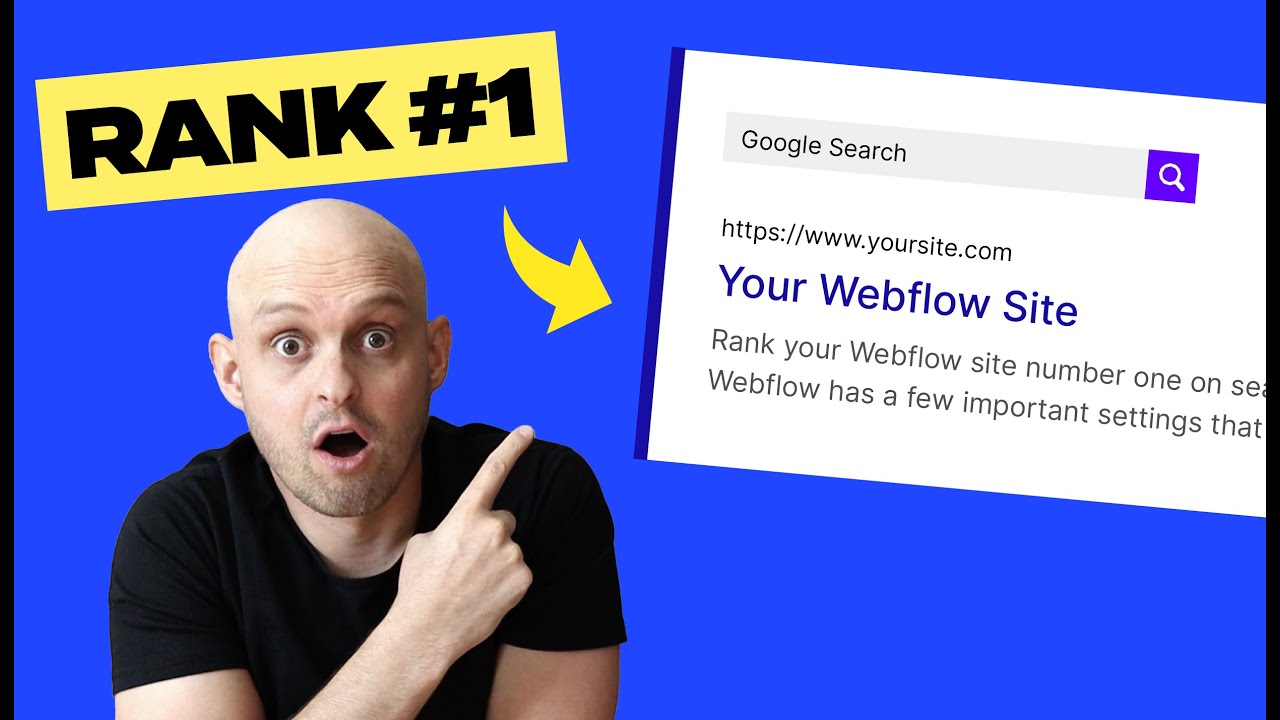
Webflow SEO Full Step-By-Step Guide to Rank #1
5.0 / 5 (0 votes)