OOP in Python | Object Oriented Programming | Python for Beginners #lec84
Summary
TLDRThis video introduces the concept of Object-Oriented Programming (OOP) in Python, explaining why it is necessary over the traditional procedure-oriented programming approach. The speaker highlights the limitations of using global variables and functions in large projects and the need for data security, which OOP provides. The video explains the basic concepts of OOP, such as classes and objects, using relatable examples. Additionally, the speaker touches on the difference between attributes and methods, providing an overview of the benefits of using OOP for managing complex projects. Future videos will explore OOP concepts in greater depth.
Takeaways
- 📚 The video introduces the concept of object-oriented programming (OOP) in Python.
- 🔍 The purpose of the video is to explain why OOP is necessary after using procedure-oriented programming (POP).
- ☕ The previous project (coffee machine project) used POP, and now the focus shifts to OOP.
- ❗ In POP, data security is a concern as global variables are freely accessible by multiple functions, making it hard to manage large-scale projects.
- 🔐 OOP addresses data security by focusing on objects and encapsulating data, preventing unauthorized access.
- 🏗️ OOP helps in managing complex relationships in large projects by modeling real-world objects and their interactions.
- 👨🏫 The video uses the example of a YouTube channel, where different roles like instructor, editor, and social media marketer represent objects in OOP.
- 💡 A class is a blueprint for creating objects, and objects are instances of classes in OOP.
- 🔄 OOP supports concepts like inheritance, polymorphism, and data hiding, which are absent in POP.
- 📝 The video promises future discussions on topics like inheritance, polymorphism, abstraction, and more details about OOP with programming examples.
Q & A
What is the main focus of this video?
-The main focus of this video is to explain why we need Object-Oriented Programming (OOP) in Python, especially after using the procedural programming approach in earlier projects.
Why is Object-Oriented Programming (OOP) introduced after using procedural programming?
-OOP is introduced because procedural programming (POP) is not efficient for handling larger, more complex projects. POP lacks features like data security, inheritance, and modularity, making it difficult to manage large codebases with many functions and global variables.
What is the key difference between procedural programming and object-oriented programming?
-The key difference is that procedural programming focuses on procedures or functions, while object-oriented programming focuses on objects that combine data and functionality, providing better data security and modularity.
What is one major drawback of procedural programming mentioned in the video?
-One major drawback is the lack of data security. In procedural programming, global data can be accessed and modified by any function, which can lead to unexpected behavior and errors in larger projects.
Why is data security important in programming?
-Data security is important because, in real-world projects, not all data should be accessible by all functions or users. For example, some personal information should be kept private and only accessible by authorized entities.
What are some features of OOP that procedural programming lacks?
-OOP includes features like inheritance, polymorphism, data hiding, and encapsulation, which procedural programming lacks. These features help manage large projects by making code more modular, reusable, and secure.
What is the role of classes and objects in OOP?
-In OOP, a class is a blueprint or template for creating objects, while objects are instances of that class. Objects have attributes (data) and methods (functions) associated with them, encapsulating both data and functionality.
Why is everything considered an object in Python?
-Everything in Python is considered an object because Python uses classes to define data types. Variables, strings, functions, and even integers are instances of predefined classes in Python.
What is meant by the term 'attributes' in OOP?
-In OOP, 'attributes' refer to the data or properties associated with an object. These are the variables defined within a class that hold information about the object.
How does OOP help in managing complex real-world projects?
-OOP helps manage complex projects by encapsulating data and functionality within objects, providing better modularity, data security, and scalability. It also allows for easier maintenance and debugging by organizing code around objects and their interactions.
What is a simple analogy used in the video to explain OOP concepts?
-The video uses the analogy of running a YouTube channel. Initially, a single person manages everything, but as the channel grows, specialists (instructors, editors, marketers) are hired for specific tasks. Similarly, in OOP, different objects (instructors, editors) handle different tasks, making the project more manageable.
Outlines
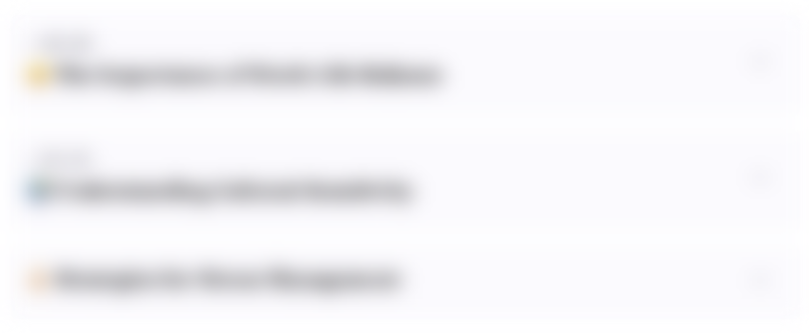
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
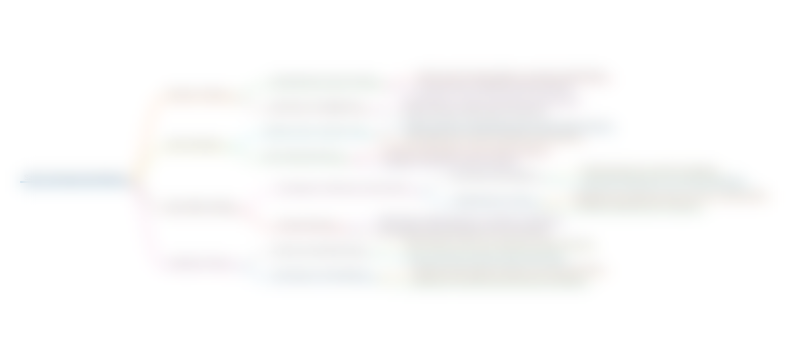
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
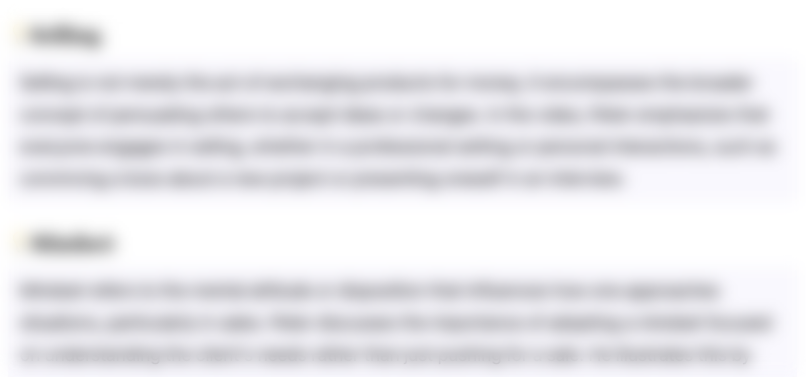
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
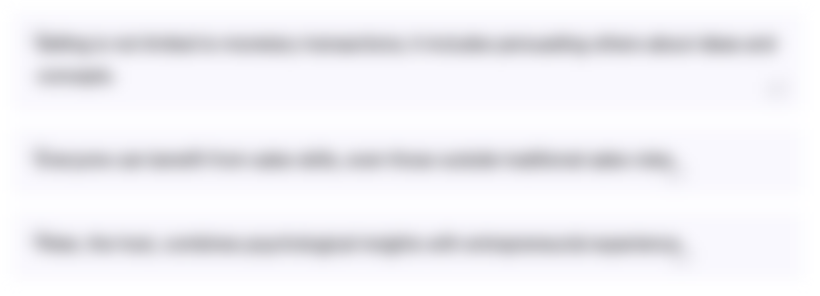
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
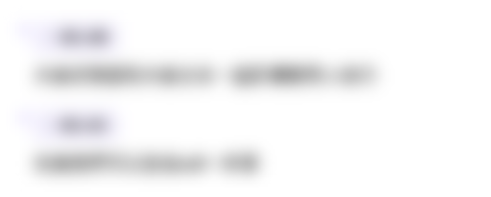
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
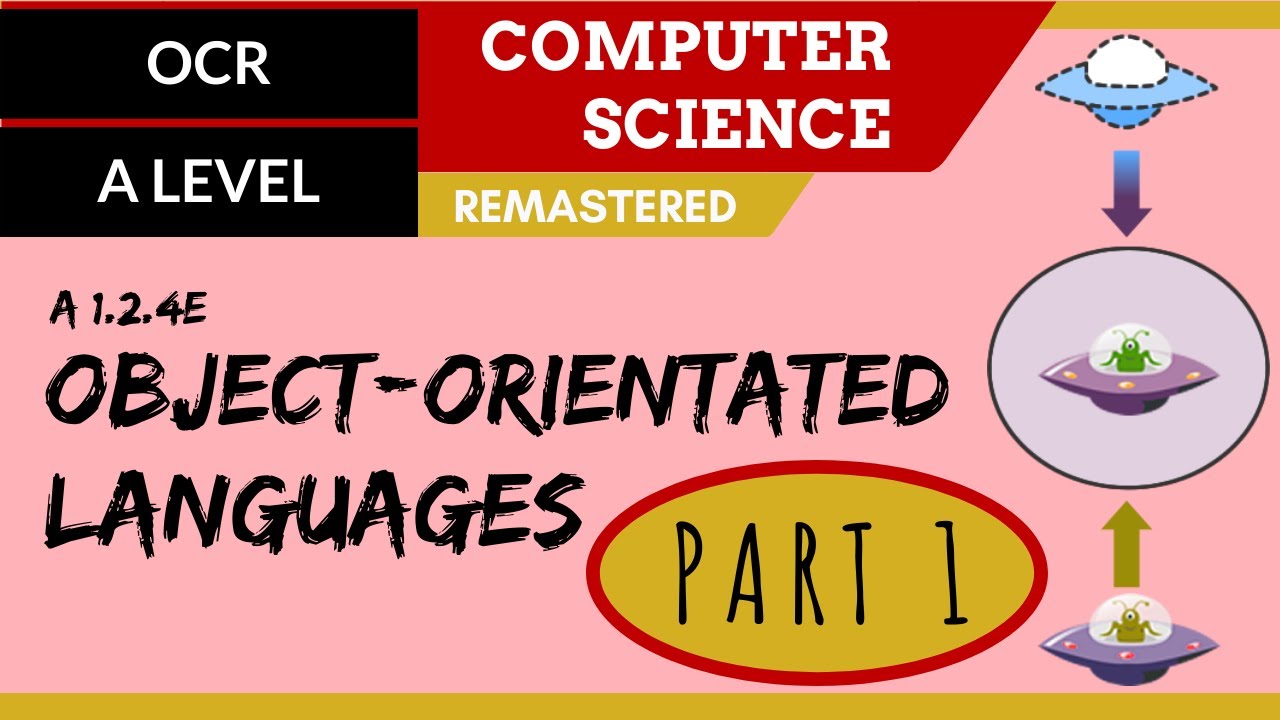
36. OCR A Level (H446) SLR7 - 1.2 Object-oriented languages part 1

Classes and Objects in Python | OOP in Python | Python for Beginners #lec85
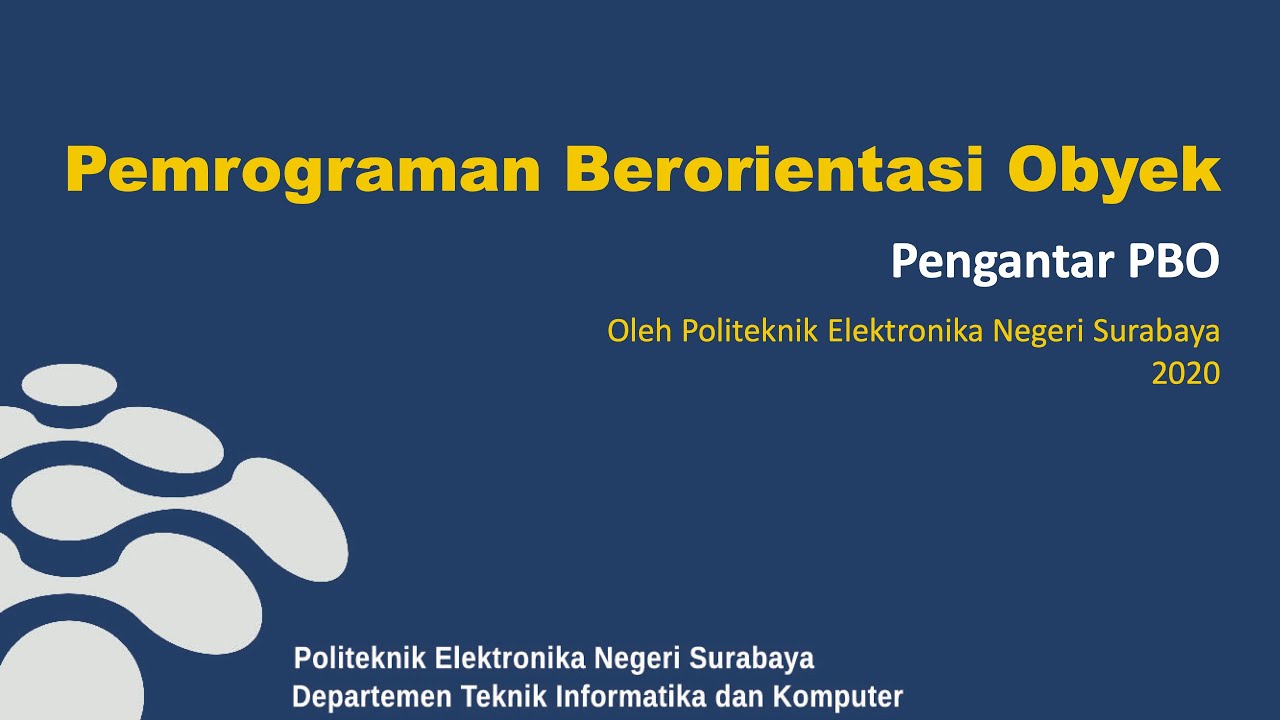
Materi 1 Introduction
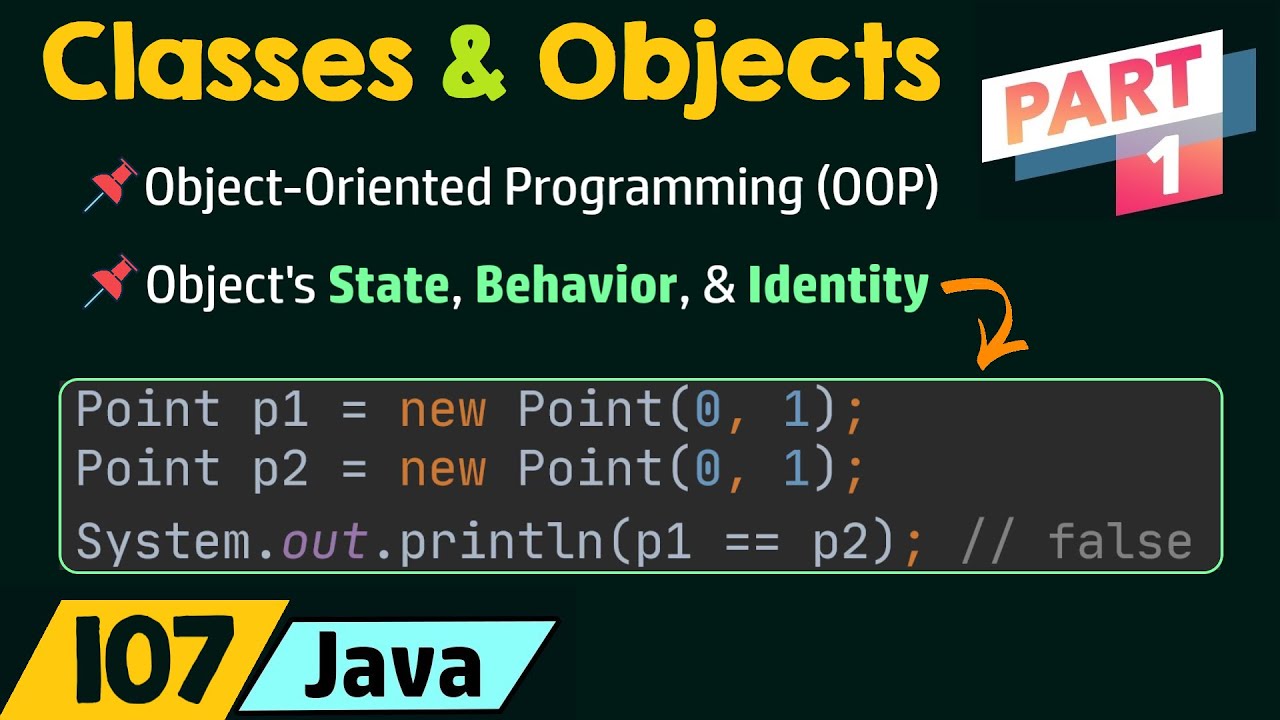
Introduction to Classes and Objects (Part 1)

Introduction to OOPs in Python | Python Tutorial - Day #56
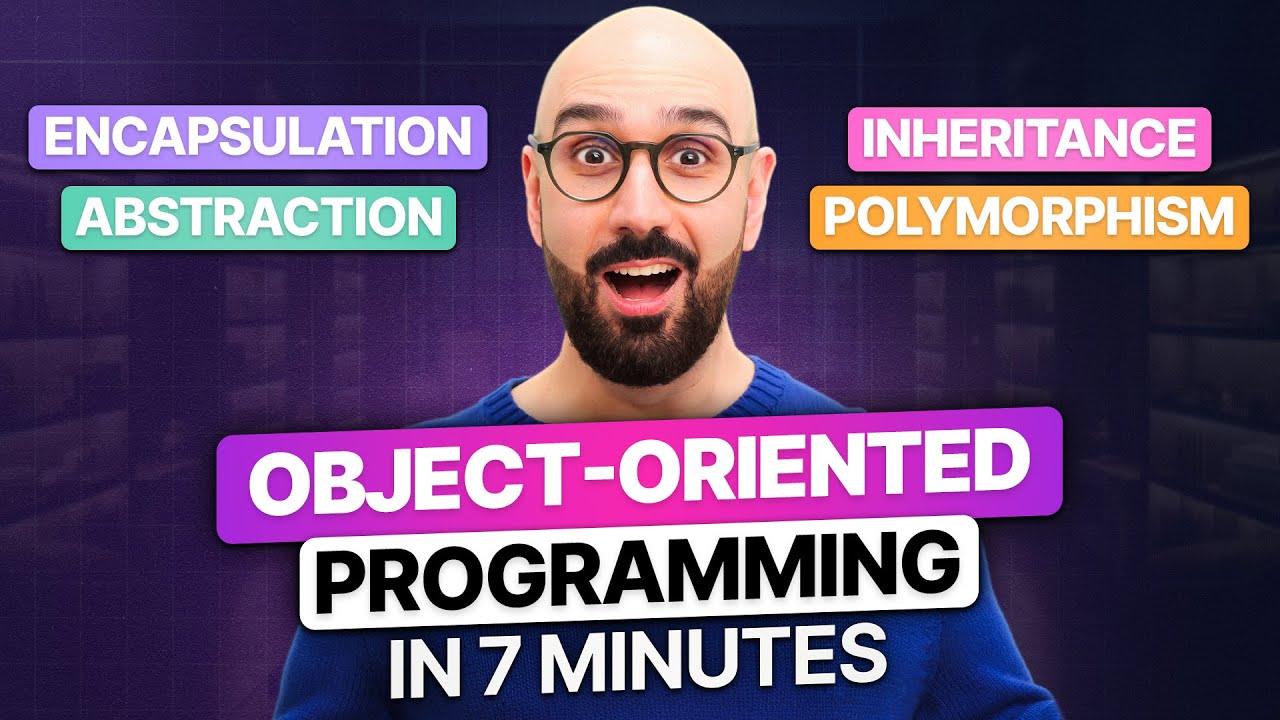
Object-oriented Programming in 7 minutes | Mosh
5.0 / 5 (0 votes)