Learn Redux Toolkit in under 1 hour
Summary
TLDRIn this informative video, the presenter Hitesh introduces viewers to the Redux toolkit, a state management library that simplifies data handling in React applications. Hitesh explains the fundamentals of Redux, emphasizing its non-React centric design and how it can be integrated with React for state management. He demonstrates the creation of a Redux store and reducers, and how to use the Redux Dev tools for inspection. The tutorial includes building a to-do application to showcase the power of Redux for managing state efficiently, walking through each step from adding and deleting to-dos, to querying the state with useSelector. Hitesh's approach is practical and straightforward, aiming to clarify common misconceptions and make Redux more accessible for developers.
Takeaways
- π Introduction to Redux Toolkit: A beginner-friendly guide to understanding and using Redux for state management in React applications.
- π¦ Redux is a state management library that is not exclusively tied to React, but can be used alongside React and other libraries through plugins.
- π§ The problem of prop drilling: Redux helps to eliminate the issue of having to pass props through multiple layers of components by offering a centralized state management system.
- π’ Core concepts of Redux: Store (centralized state container), Reducers (pure functions that specify how the state can be updated), and Actions (objects that describe what should happen to the state).
- π Redux data flow: Data should flow in one direction from the store to the UI, with updates made through reducers in response to actions.
- π οΈ Use of hooks in React for Redux: 'useSelector' to read data from the store and 'useDispatch' to dispatch actions to update the store.
- π Project structure: Organizing Redux logic into features or slices, with each slice containing its own reducer and initial state.
- π Debugging with Redux DevTools: Using the Redux DevTools extension to inspect the state, actions, and changes in the store during development.
- π Best practices: Avoiding direct state mutation and using descriptive action and reducer names for better readability and maintainability.
- π Handling undo/redo patterns: Redux makes it easier to implement complex patterns like undo/redo by keeping track of all state changes.
- π Importance of learning Redux: Despite its initial complexity, mastering Redux can greatly simplify state management and improve the scalability and maintainability of large applications.
Q & A
What is Redux and why is it used in state management?
-Redux is a state management library that is not specifically centered towards React or Next.js, but can be combined with them using plugins. It is used to manage the state of an application in a more structured and predictable manner, making it easier to handle complex state logic and maintain consistency across different parts of the application.
What does the Redux Toolkit provide that makes working with Redux simpler?
-The Redux Toolkit provides a set of utilities and conventions that simplify the creation of a Redux store, the management of reducers, and the interaction with the store through actions and selectors. It reduces boilerplate code and helps in following best practices, making the overall process of using Redux more straightforward and less error-prone.
How does Redux help in solving the issue of prop drilling in React applications?
-Redux helps in solving prop drilling by providing a centralized store where components can directly access and update the state, eliminating the need to pass props down through multiple levels of the component hierarchy. This allows for a more efficient and clean way of managing state across the application.
What are the main components of a Redux state management system?
-The main components of a Redux state management system include the store, reducers, actions, and selectors. The store holds the application state, reducers define how the state can be updated, actions are used to describe changes to the state, and selectors are functions that help in selecting and extracting data from the store.
How does the Redux Toolkit's 'createSlice' method simplify the process of creating reducers?
-The 'createSlice' method in the Redux Toolkit simplifies the process of creating reducers by allowing developers to define the initial state, reducers, and actions in a single object. It automatically generates action creators and action types, making it easier to manage and maintain the state logic within the application.
What is the role of 'useDispatch' and 'useSelector' hooks in a React application using Redux?
-The 'useDispatch' hook is used to dispatch actions to the Redux store, allowing components to trigger state changes. The 'useSelector' hook is used to access and select data from the Redux store, enabling components to display the current state or parts of it. These hooks facilitate the connection between React components and the Redux state management system.
How does the Redux state management system ensure a unidirectional data flow?
-Redux ensures a unidirectional data flow by mandating that state can only be updated through reducers in response to actions. This means that all changes to the state must go through a clear and predictable path: actions describe what happens, reducers define how the state changes, and the store holds the current state.
What is the significance of using unique IDs for each to-do item in the Redux store?
-Using unique IDs for each to-do item in the Redux store allows for easier management and manipulation of individual items. It enables specific items to be targeted for actions such as updates or deletions without affecting other items in the list, leading to a more efficient and organized state management process.
What is the role of the 'Provider' component in a Redux-enabled React application?
-The 'Provider' component from React-Redux is used to make the Redux store available to all components in the application. It wraps the root component of the application, ensuring that any component can access and interact with the Redux store through the use of hooks like 'useDispatch' and 'useSelector'.
How does the Redux Toolkit handle state immutability?
-The Redux Toolkit automatically handles state immutability, ensuring that the state is not directly mutated. Instead, it requires the use of specific methods and patterns, such as the 'createSlice' method and the 'immer' library, which allows for the creation of draft state copies that can be modified without directly altering the original state.
Outlines
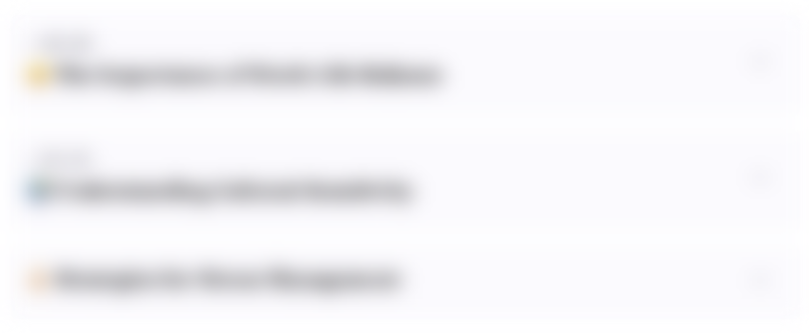
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
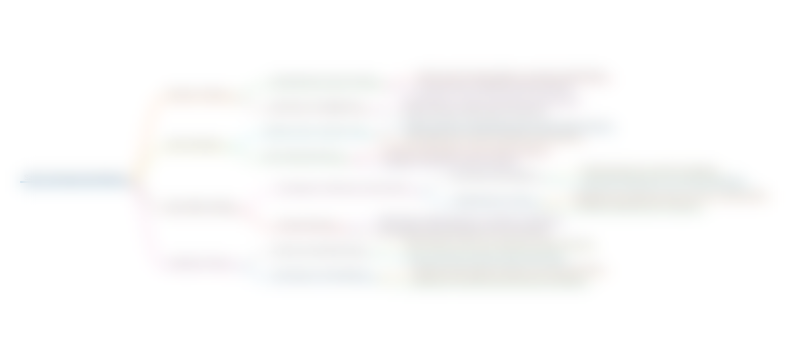
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
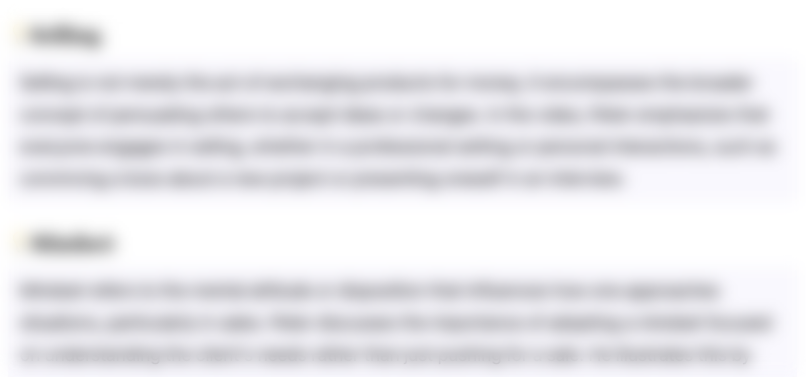
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
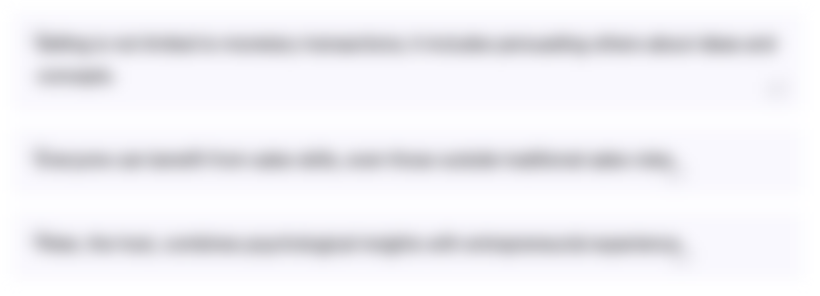
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
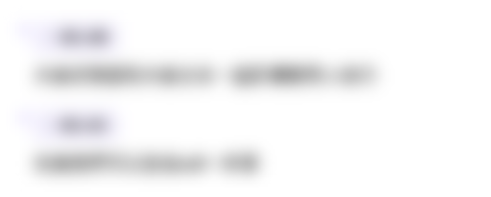
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
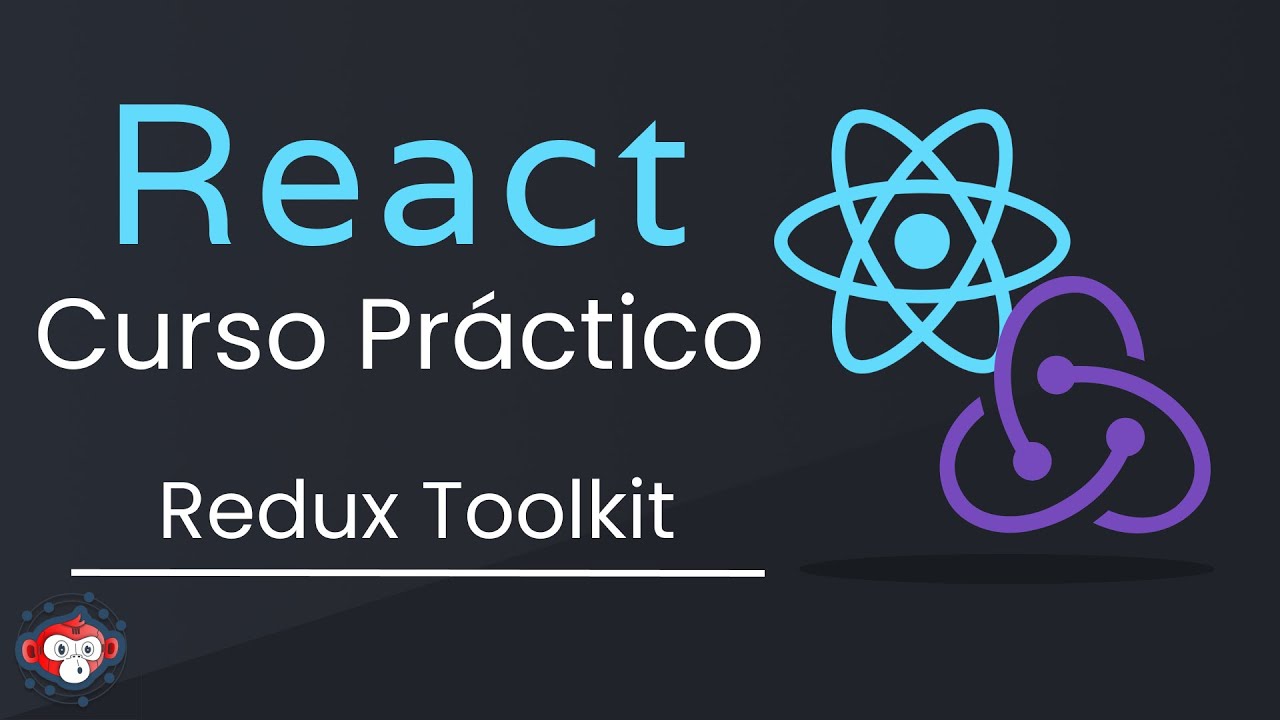
React & Redux Toolkit - Bases y proyecto prΓ‘ctico
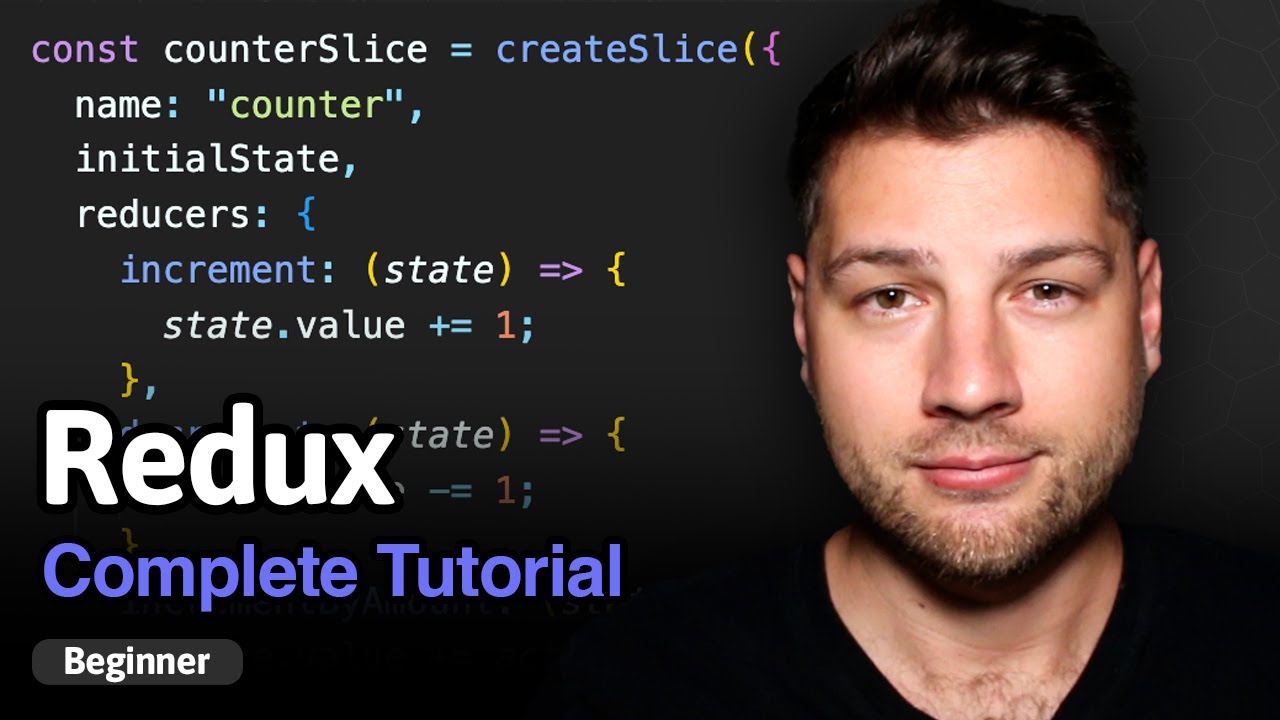
Redux - Complete Tutorial (with Redux Toolkit)

Introduction to Redux | Lecture 257 | React.JS π₯

Vuex State Management Introduction & Create Store | #35 | Vuex State Management Vue js 3 in Hindi
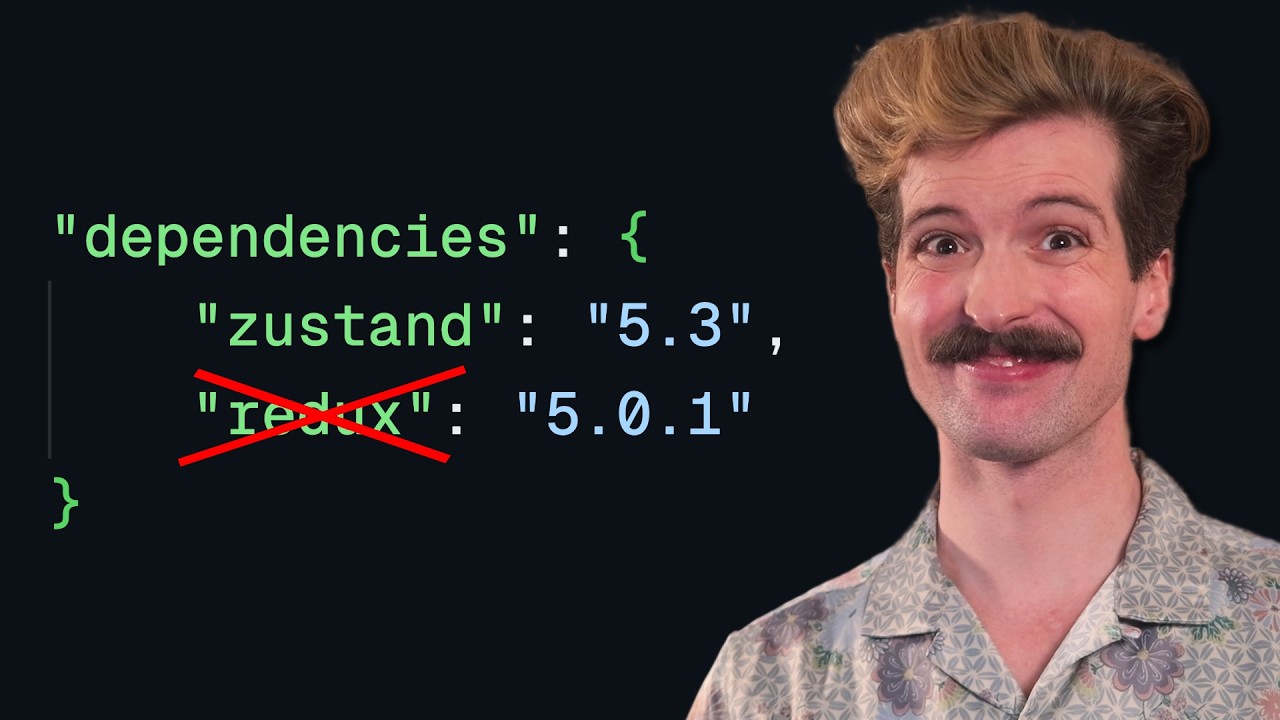
Why Everyone Loves Zustand
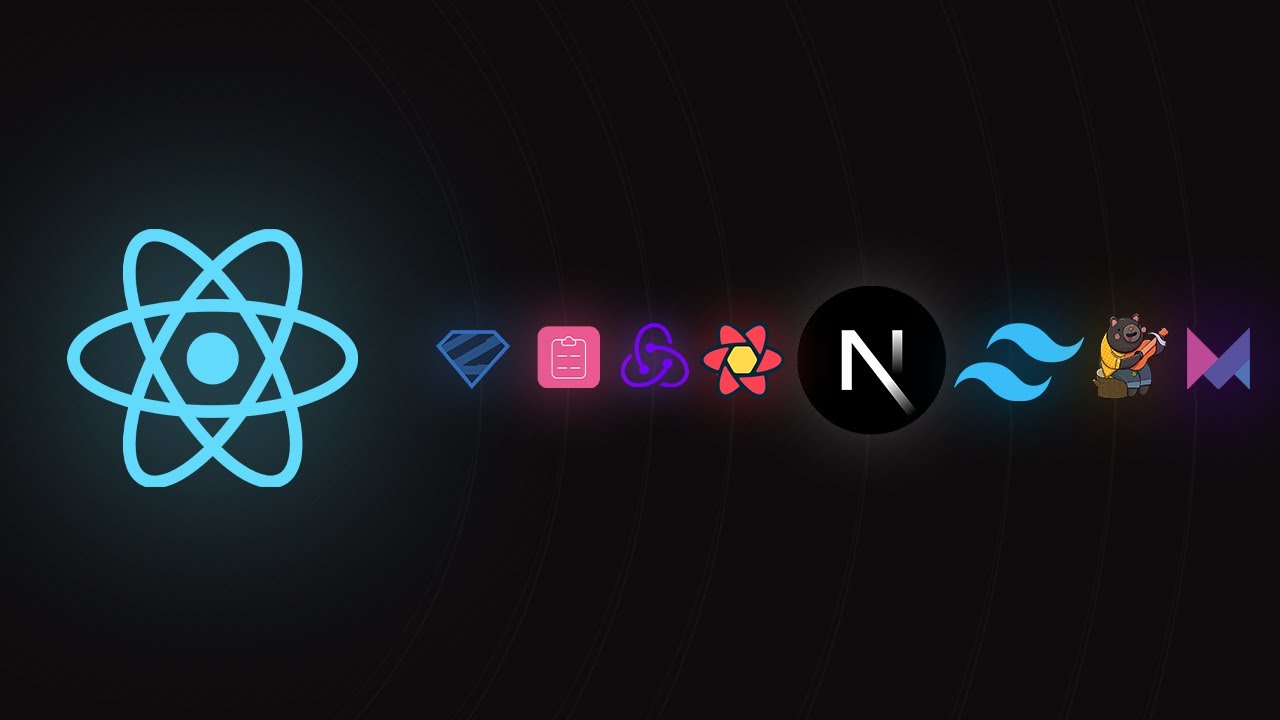
Master the React ecosystem in 2024
5.0 / 5 (0 votes)