C# Tutorial 1: Variables
Summary
TLDRThis video lesson, presented by Michael using a text-to-speech program, provides an introduction to variables in C#. It covers the concept of variables, expressions, and how they work in programming. Key topics include declaring and initializing variables, naming rules, data types, and the use of explicitly and implicitly typed variables using the 'var' keyword. Examples illustrate how variables store values, how they can change, and how they are used in expressions. The video emphasizes that C# is a strongly typed language and explains common errors when working with variables.
Takeaways
- 💻 Variables in C# store values of specific data types such as integers, strings, or chars.
- ➕ Expressions in programming are legal combinations of symbols that represent values, like '10 + 20' resulting in 30.
- 🧮 Variables can hold different values depending on their assignments, for example, 'x + 20' changes based on the value of x.
- ⚙️ Declaring a variable in C# requires specifying the data type, variable name, and assigning a value.
- 🔠 C# variable names must be unique, start with a letter, and can contain letters, digits, or underscores.
- 🖋 C# is strongly typed, meaning you cannot assign a value of one type to a variable of another type, like assigning a string to an integer.
- 🛠 Variables can be declared and initialized in one or multiple statements, and their values can be reassigned later.
- 🔄 Variables can participate in expressions, and the result of an expression can be assigned to a variable.
- ✨ In C# 3.0, the 'var' keyword was introduced to declare variables without specifying the data type explicitly, with the compiler inferring it.
- 🚫 Implicitly typed variables using 'var' must be initialized at the time of declaration, or the compiler will give an error.
Q & A
What is a variable in C#?
-A variable in C# is a storage location that can hold a value. Each variable has a particular data type that determines what type of value it can store, such as numeric, char, string, or other types.
How are variables similar between C# and mathematics?
-In both C# and mathematics, variables represent values that can change. For example, if x equals 5, the expression x + 20 will result in 25. Similarly, in C#, a variable's value can be updated and used in expressions.
What are the steps to declare and initialize a variable in C#?
-In C#, to declare and initialize a variable, you must specify the data type, provide the variable name, and optionally assign a value. For example: `int x = 5;`, where `int` is the data type, `x` is the variable name, and 5 is the value assigned.
What are the rules for naming variables in C#?
-The rules for naming variables in C# include: variable names must be unique, can contain letters, digits, and underscores, must start with a letter, are case-sensitive, cannot contain reserved keywords, and cannot contain spaces.
What does it mean that C# is a strongly-typed language?
-C# is a strongly-typed language, meaning you must assign values to variables that match the variable’s specified data type. For example, you cannot assign a string to an integer variable.
Can a variable's value in C# be reassigned after initialization?
-Yes, in C#, you can reassign a variable’s value after initialization. For example, if `x` is initialized to 100, you can later change it to 200. The variable will hold the last value assigned.
How can you declare multiple variables of the same data type in C#?
-You can declare multiple variables of the same data type in a single statement by separating them with commas. For example: `int a = 1, b = 2, c = 3;`.
What is the difference between explicitly and implicitly typed variables in C#?
-Explicitly typed variables require you to declare the data type (e.g., `int x = 5;`). Implicitly typed variables, introduced in C# 3.0 using the `var` keyword, allow the compiler to infer the data type based on the assigned value (e.g., `var number = 100;`).
What are some restrictions when using the 'var' keyword in C#?
-When using the `var` keyword, the variable must be initialized at the time of declaration, and you cannot declare multiple `var` variables in a single statement.
What happens if a variable is declared but not initialized in C#?
-In C#, if a variable is declared but not initialized, you cannot use it until it has been assigned a value. Attempting to use an uninitialized variable will result in a compile-time error.
Outlines
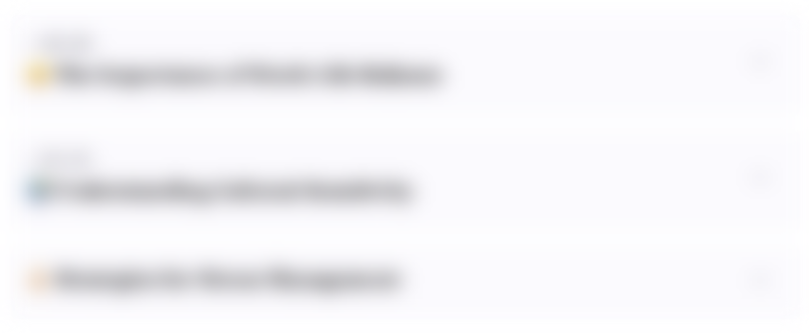
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
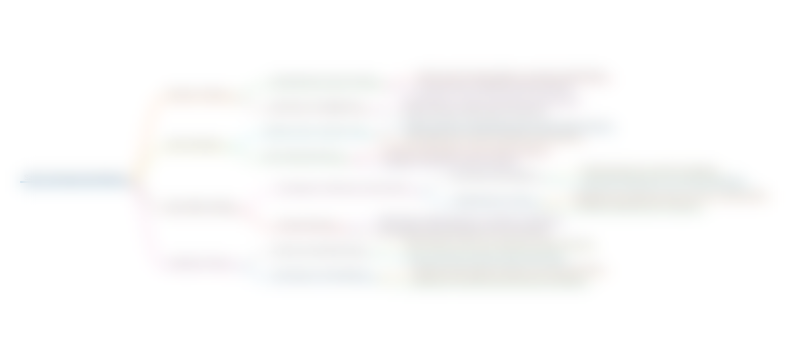
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
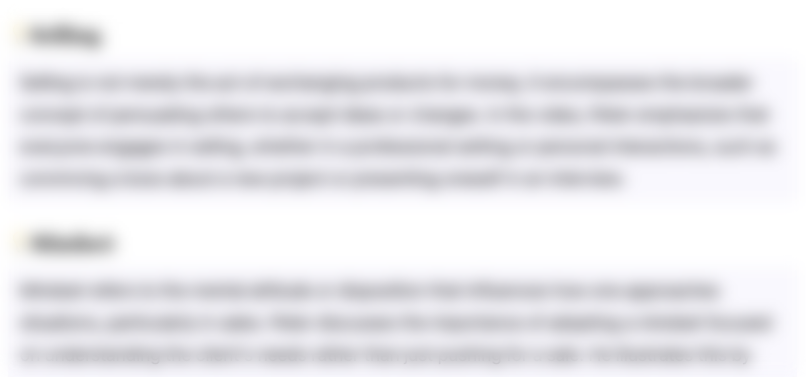
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
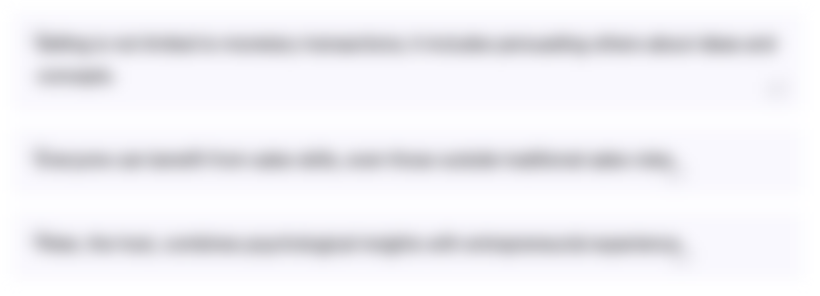
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
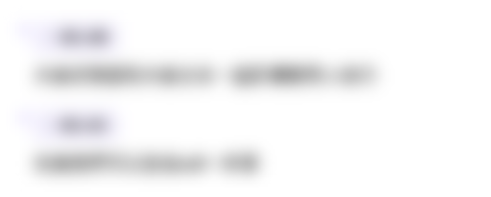
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)