Flutter Tutorial for Beginners #5 - Scaffold & AppBar Widgets
Summary
TLDRThis script provides a step-by-step guide on setting up a basic Flutter app using the Material App as the root widget. It introduces the Scaffold widget for implementing a basic layout, including an app bar, body, and floating action button. The tutorial demonstrates how to customize the app bar with a title and center-align text within the body. It also includes adding a floating action button with a text child. The script emphasizes the ease of using built-in widgets to quickly create a functional app layout and encourages further exploration of the Scaffold widget's properties.
Takeaways
- 📝 The script is a tutorial on setting up a basic Flutter app using the Material App widget as the root widget.
- 🏠 The 'home' property is crucial as it defines what appears on the home screen when the app is launched.
- 📝 Initially, the home screen contains a simple Text widget with the message 'Hey Ninjas'.
- 🛠 The Scaffold widget is introduced as a way to implement a basic layout, including an app bar and floating action buttons.
- 🔄 The Scaffold widget is a built-in Flutter widget that can be customized with various properties.
- 📝 The AppBar widget is used to create a title bar at the top of the app, and it can be customized with properties like 'title'.
- 🎨 The 'centerTitle' property is used to center the app bar title on the screen, which is common for Android devices.
- 📝 The 'body' property of the Scaffold widget is used to define the main content area of the app, placed below the app bar.
- 🔄 The Center widget is used to centralize its child widget, which in this case is a Text widget saying 'Hello Ninjas'.
- 🔄 The 'floatingActionButton' property is used to add a floating action button to the app, which can contain a child widget like Text.
- 🔗 The script encourages further exploration of the Scaffold widget and its properties through the official Flutter documentation.
Q & A
What is the purpose of the Material App widget in Flutter?
-The Material App widget in Flutter serves as the root widget for an app, providing a basic structure and acting as a wrapper for the rest of the app.
What is the role of the 'home' property in the Material App widget?
-The 'home' property in the Material App widget specifies the initial screen or widget that is displayed when the app is launched.
What is the Scaffold widget and how does it help in app development?
-The Scaffold widget is a layout widget in Flutter that provides a basic layout for an app, including an app bar, a body, and a floating action button, making it easier to set up the structure of an app.
How can you add an AppBar to your app using the Scaffold widget?
-You can add an AppBar to your app by specifying an 'appBar' property within the Scaffold widget, and setting its value to an AppBar widget with the desired properties.
What is the significance of the 'title' property in the AppBar widget?
-The 'title' property in the AppBar widget determines the text that is displayed in the title section of the app bar.
Why is a Text widget used inside the 'title' property of the AppBar?
-A Text widget is used inside the 'title' property of the AppBar to display text, as Flutter requires the use of a widget to output text rather than using a plain string.
What does the 'centerTitle' property do in the AppBar widget?
-The 'centerTitle' property, when set to true, centers the title text within the AppBar on Android devices.
How can you add content to the body of the screen in a Flutter app?
-You can add content to the body of the screen by specifying a 'body' property within the Scaffold widget, and setting its value to the desired widget, such as a Text widget.
What is the purpose of the Center widget in Flutter?
-The Center widget in Flutter is used to centralize the content of its child widget, which is useful for aligning text or other widgets in the center of the screen.
How can you add a floating action button to your app using the Scaffold widget?
-You can add a floating action button by specifying a 'floatingActionButton' property within the Scaffold widget, and setting its value to a FloatingActionButton widget with a 'child' property containing the desired content, such as a Text widget.
What is the 'hot restart' feature in Flutter and when is it used?
-The 'hot restart' feature in Flutter allows developers to see the changes they made in the code without needing to relaunch the app. It is used to quickly refresh the app and display the updated screen after making modifications.
Outlines
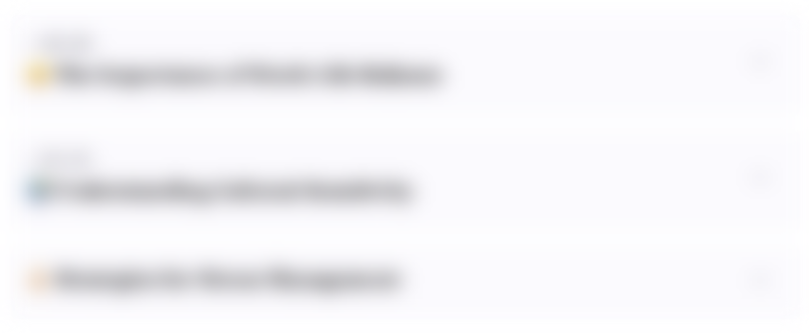
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
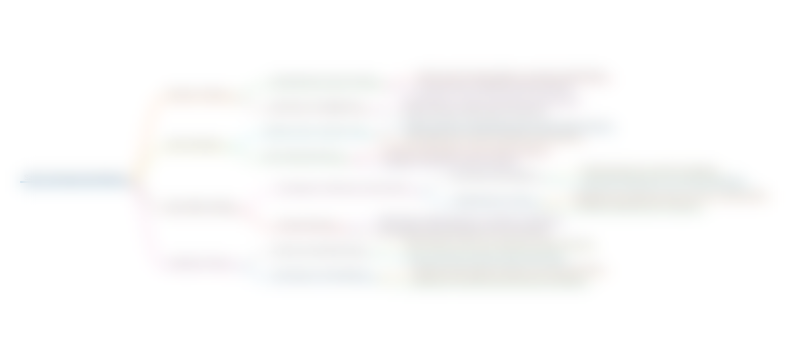
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
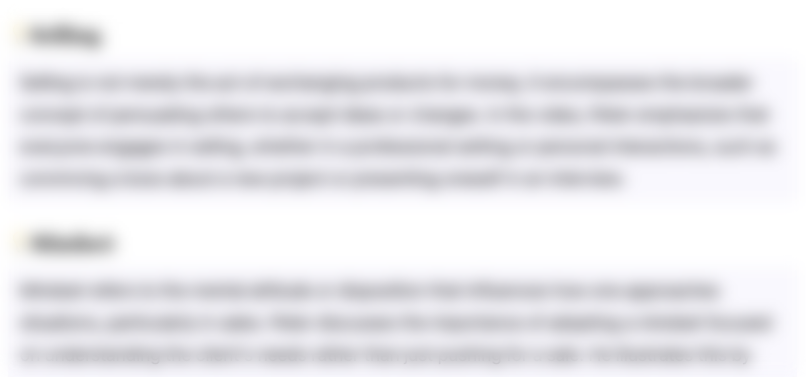
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
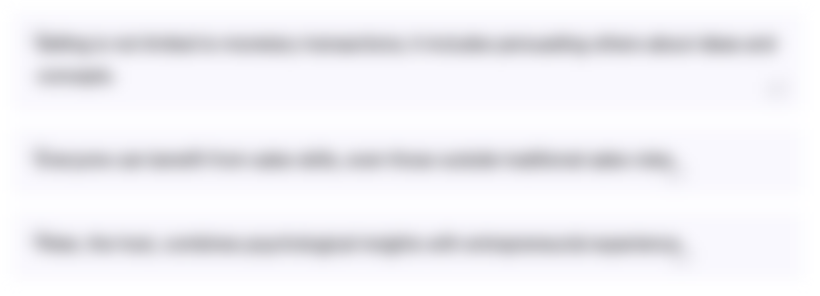
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
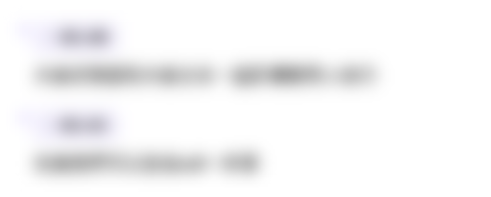
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
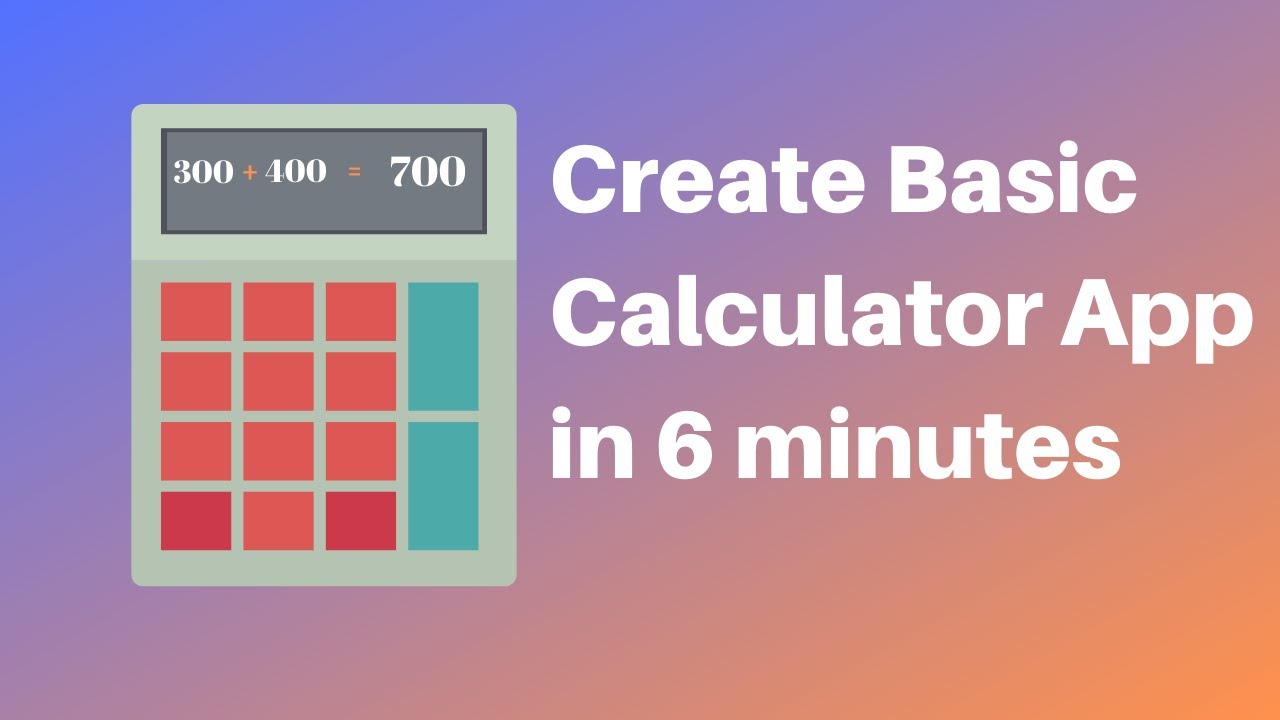
How to make a calculator in MIT App Inventor 2
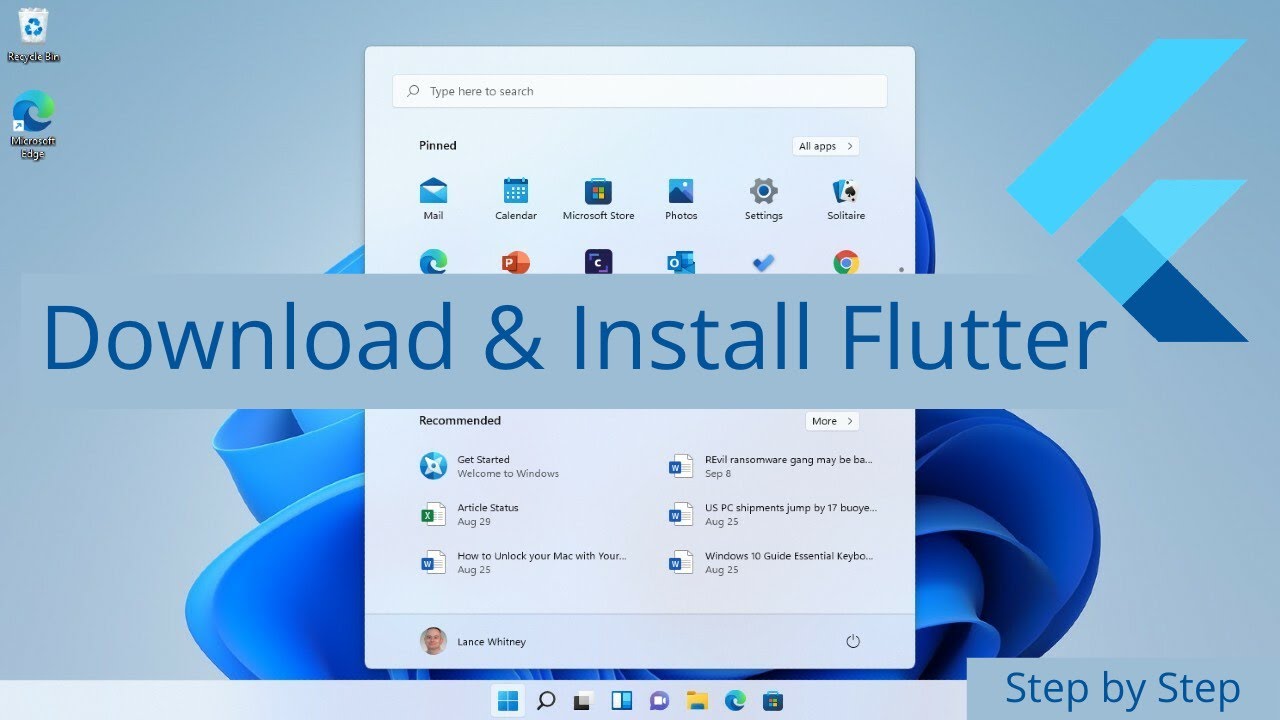
How to Install Flutter in Windows 11 - Flutter Windows Installation Step by Step Setup
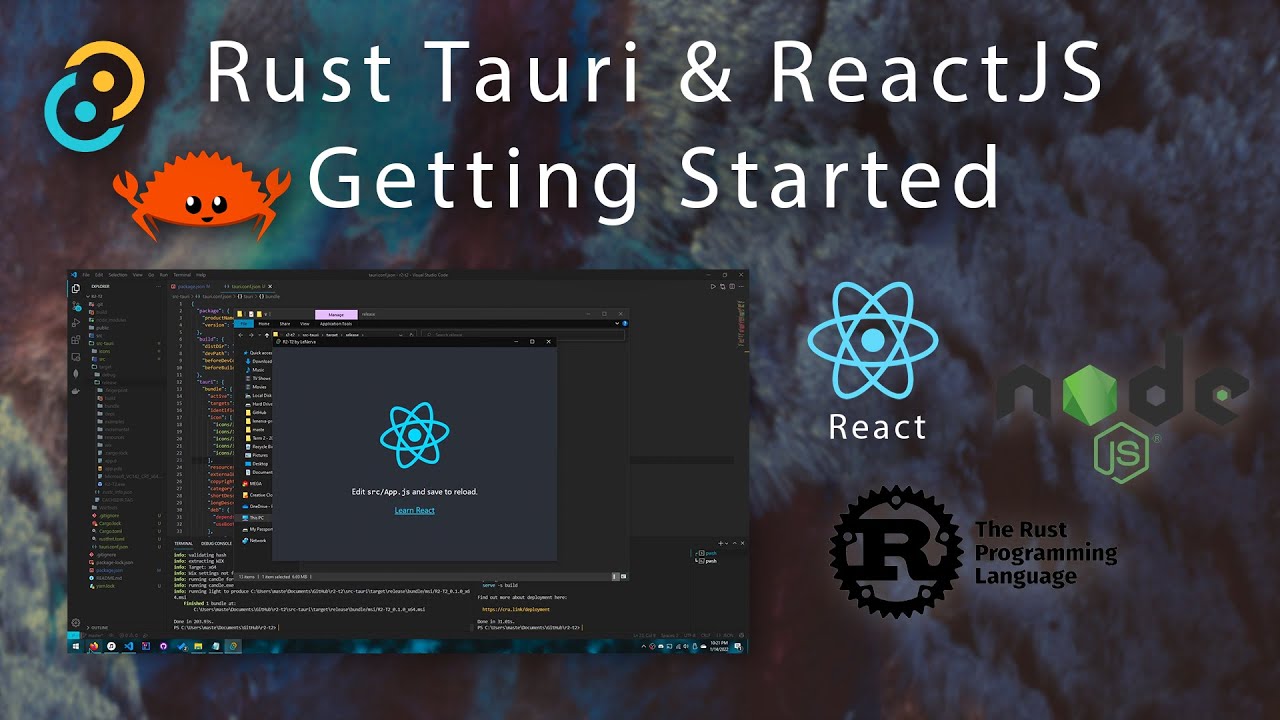
Tauri & ReactJS - Creating Modern Desktop Apps (read desc.)
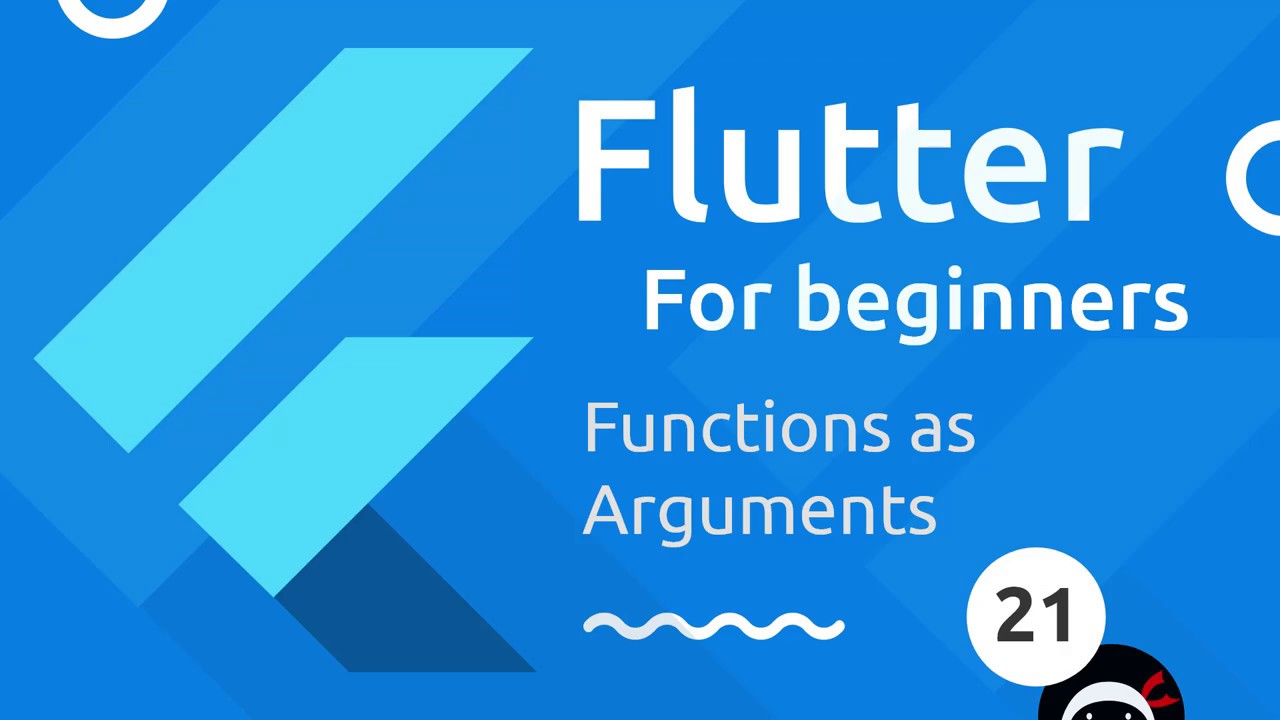
Flutter Tutorial for Beginners #21 - Functions as Parameters

Cara menghidupkan lampu rumah pakai INTERNET | Bisa seluruh dunia
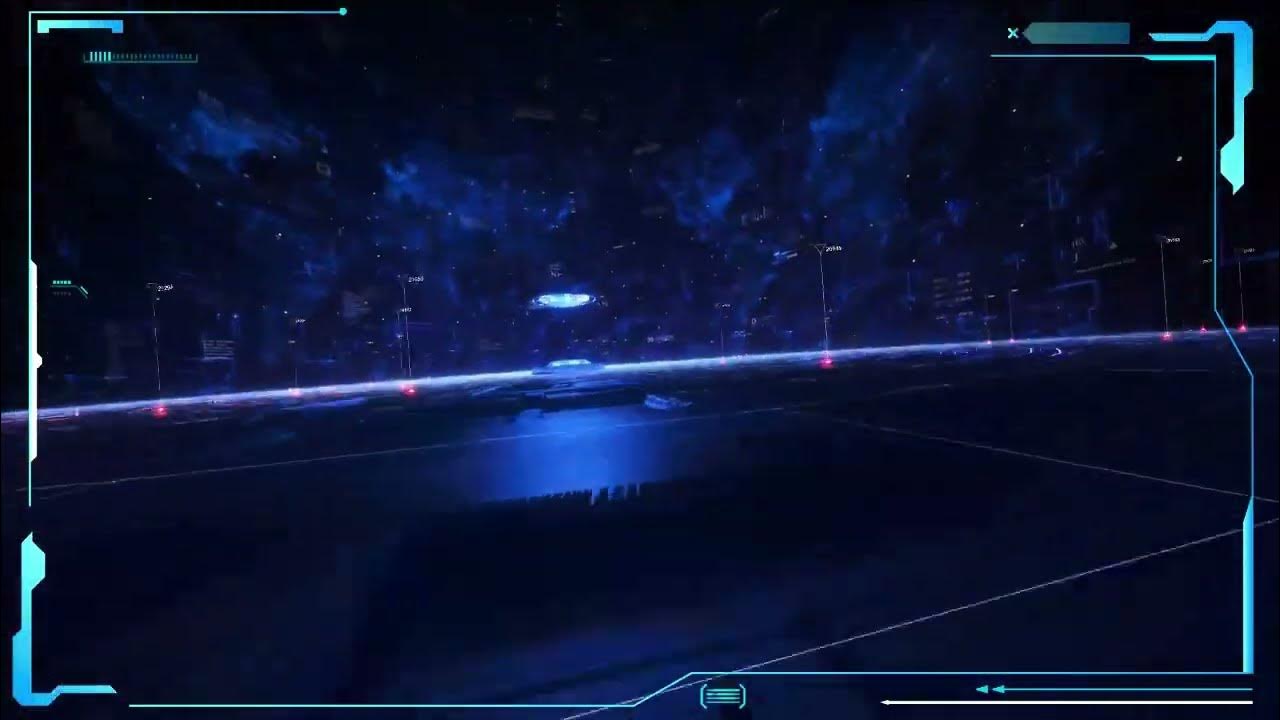
Media Ajarku: Instalasi Debian 12 CLI
5.0 / 5 (0 votes)