C_81 Null Pointer in C | C Programming Tutorials
Summary
TLDRThis video script discusses null pointers in C programming, explaining their definition, usage, and importance. It differentiates null pointers from void and uninitialized pointers, emphasizing the risks of dereferencing a null pointer and the benefits of initializing pointers with null for error handling. Practical examples are provided to illustrate the concepts.
Takeaways
- 😀 A null pointer in C is a special pointer that does not point to any valid object or memory location.
- 🔍 Null pointers are typically initialized with the value `NULL` (which is zero in C), ensuring they do not point to any valid memory address.
- 🚫 It is crucial not to dereference a null pointer, as doing so will lead to undefined behavior and potentially crash the program.
- 💡 The primary use of null pointers is for error handling, especially in scenarios where dynamic memory allocation might fail.
- 📚 Null pointers are defined in standard C header files like `<stdio.h>` and `<stddef.h>`, making them a standard part of the language.
- 🔄 When a pointer is not initialized, it contains a garbage value, making it a wild pointer, which is risky to use without proper checks.
- 🔑 Initializing a pointer with `NULL` is safer than leaving it uninitialized, as it avoids undefined behavior and potential crashes.
- 🔍 Before performing operations on a pointer, it is essential to check if it is null to prevent dereferencing a null pointer.
- 🔗 Null pointers are different from void pointers, which are pointers that can point to any type of data, while null pointers specifically do not point to any valid object.
- 🔄 Comparing two null pointers will always result in equality because they both contain the value zero, indicating they do not point to any valid memory location.
Q & A
What is a null pointer?
-A null pointer is a special pointer in C that does not refer to any valid object or address. It is initialized with the value zero. In the context of pointers, null is a macro defined in the standard library headers that represents a null pointer constant.
Why is it important to initialize a pointer with null?
-Initializing a pointer with null is important to avoid undefined behavior. An uninitialized pointer can contain garbage values, which may point to any random memory location, potentially leading to crashes or incorrect program behavior. Using null ensures that the pointer does not accidentally dereference an invalid memory address.
What happens if you dereference a null pointer?
-Dereferencing a null pointer will result in a program crash because a null pointer does not point to any valid memory location. Attempting to access the memory location it points to (which is zero) will lead to undefined behavior, typically causing the program to terminate.
How is a null pointer different from an uninitialized pointer?
-A null pointer is explicitly initialized to zero and is a valid pointer constant that does not point to any object. An uninitialized pointer, on the other hand, has not been assigned any value and may contain a garbage value, which is unpredictable and could point to any random memory location.
What is the purpose of using a null pointer in programming?
-The primary purpose of using a null pointer is for error handling and to indicate that a pointer does not point to a valid memory location. It is commonly used in scenarios where memory allocation might fail, or when a function needs to indicate that no valid address is being passed.
Can you compare two null pointers for equality?
-Yes, you can compare two null pointers for equality. Since both null pointers are initialized to zero, comparing them will always result in true, indicating that they are equal.
What is the difference between a null pointer and a void pointer?
-A null pointer is a pointer that does not point to any valid object and is initialized to zero. A void pointer, on the other hand, is a generic pointer type that can point to any type of data. The null pointer is a specific value that indicates no valid address, whereas a void pointer is a type that can be used to point to any data type.
How can you check if a pointer is null before dereferencing it?
-You can check if a pointer is null by using an if statement to compare the pointer to null. For example, `if (ptr == NULL) { /* pointer is null, handle accordingly */ }`. This check helps prevent dereferencing a null pointer, which would lead to a program crash.
Why is it risky to use an uninitialized pointer?
-Using an uninitialized pointer is risky because it may contain a garbage value that points to an arbitrary memory location. Dereferencing such a pointer can lead to undefined behavior, including program crashes or corruption of data, as the program might access or modify memory that it should not.
How does a null pointer affect dynamic memory allocation?
-In the context of dynamic memory allocation, a null pointer is used to indicate that memory allocation has failed. If a function like `malloc` or `calloc` cannot allocate the requested memory, it returns a null pointer. Checking for a null pointer after a memory allocation attempt is crucial to handle such failures gracefully.
Outlines
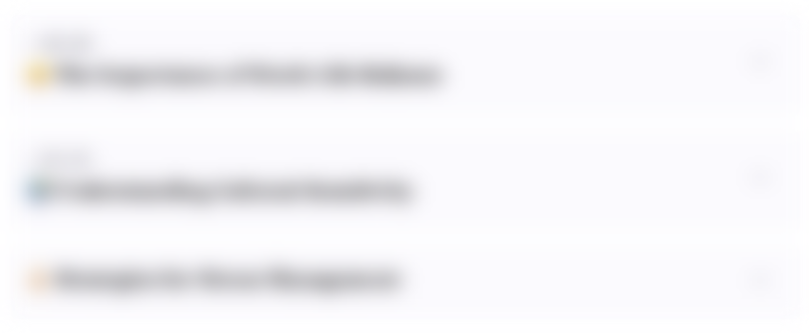
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
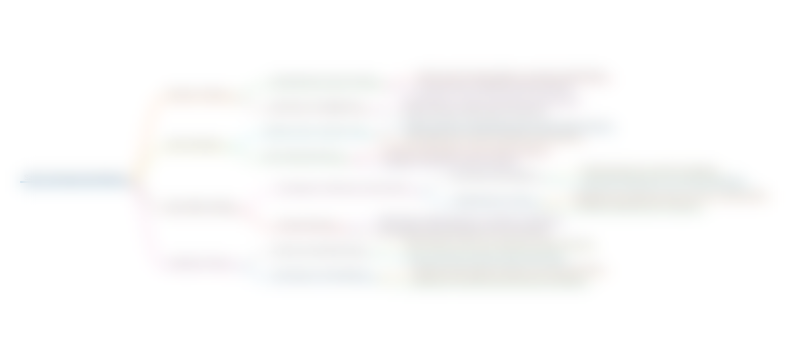
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
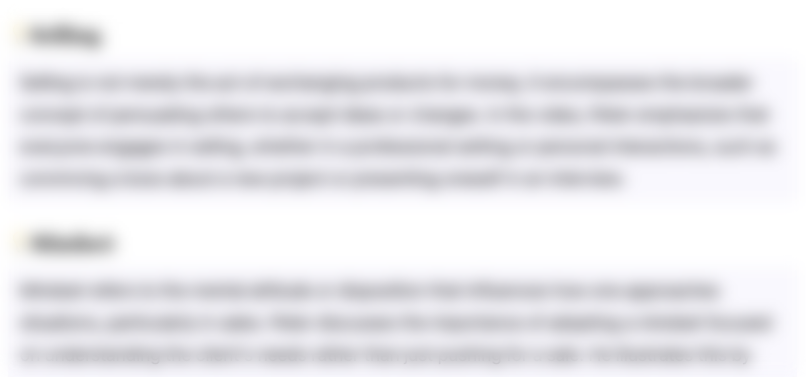
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
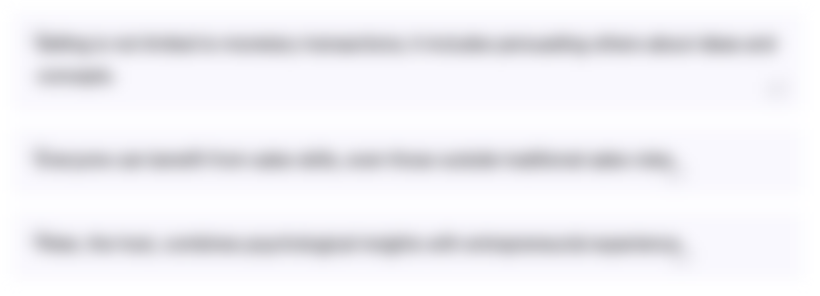
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
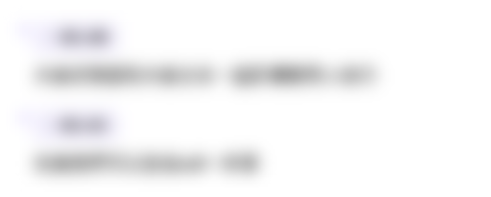
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
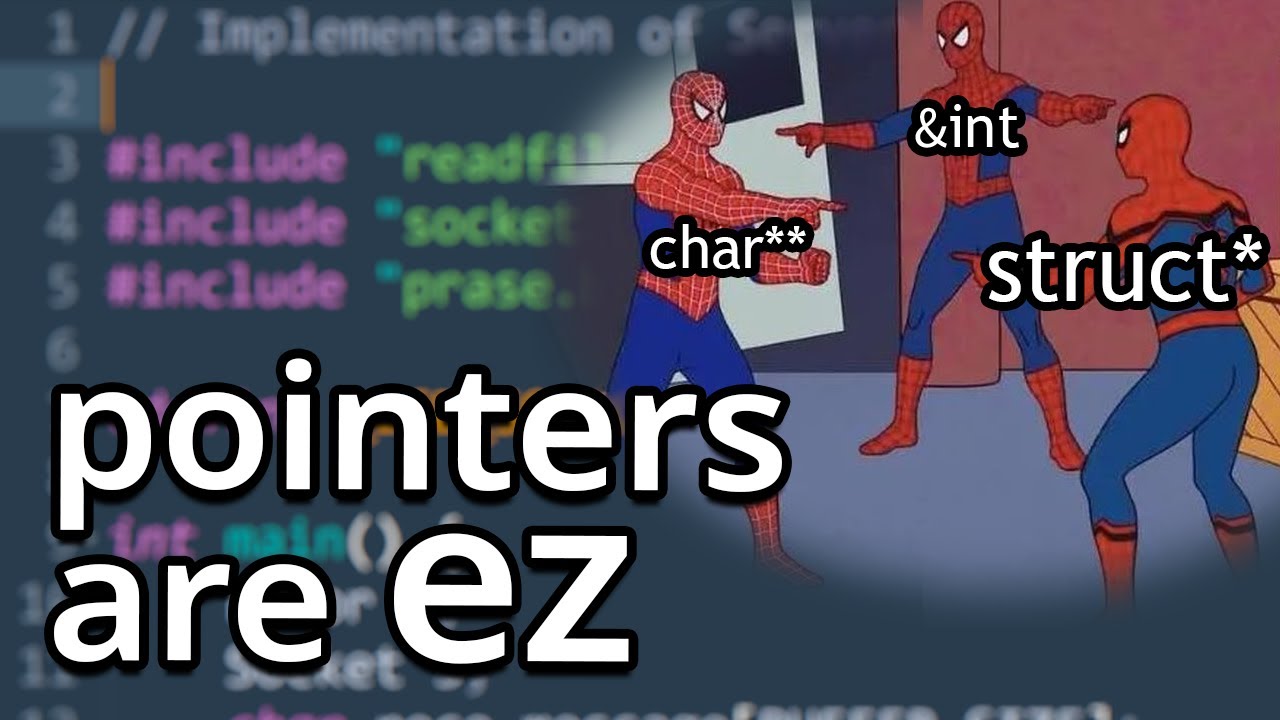
you will never ask about pointers again after watching this video
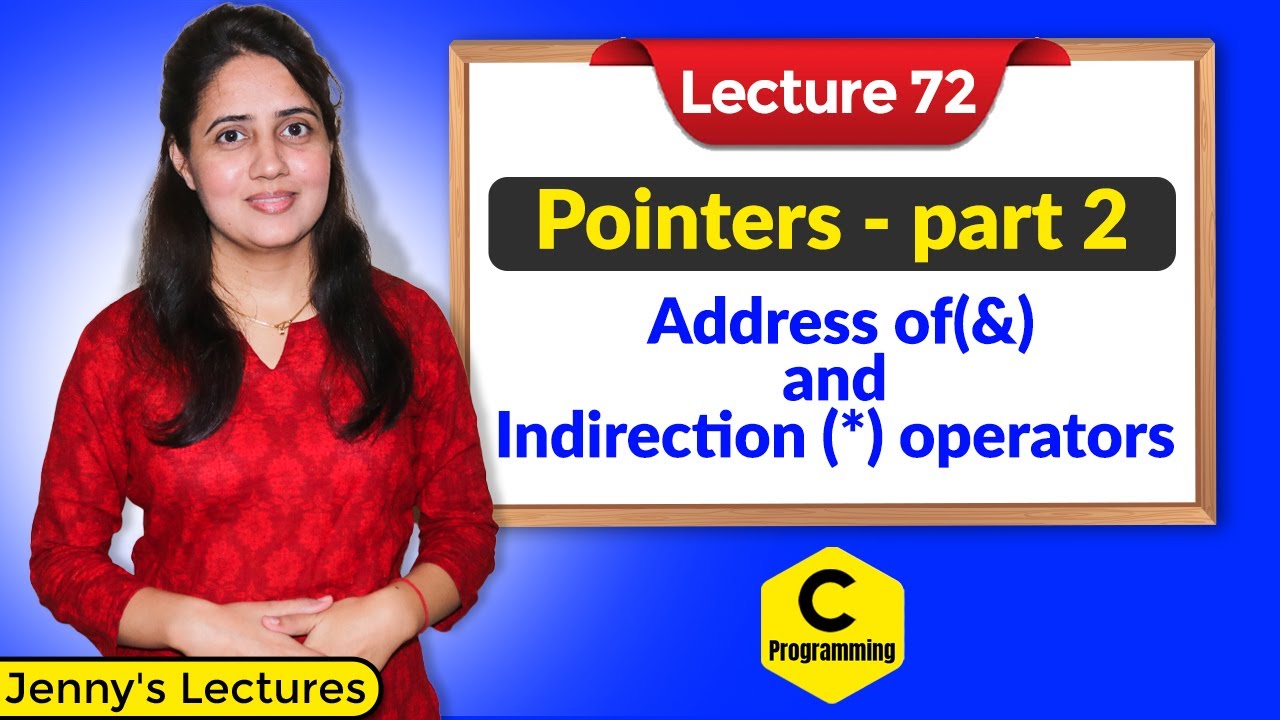
C_72 Pointers in C- part 2 |Address of(&) and Indirection (*) operator in Pointers I C Programming
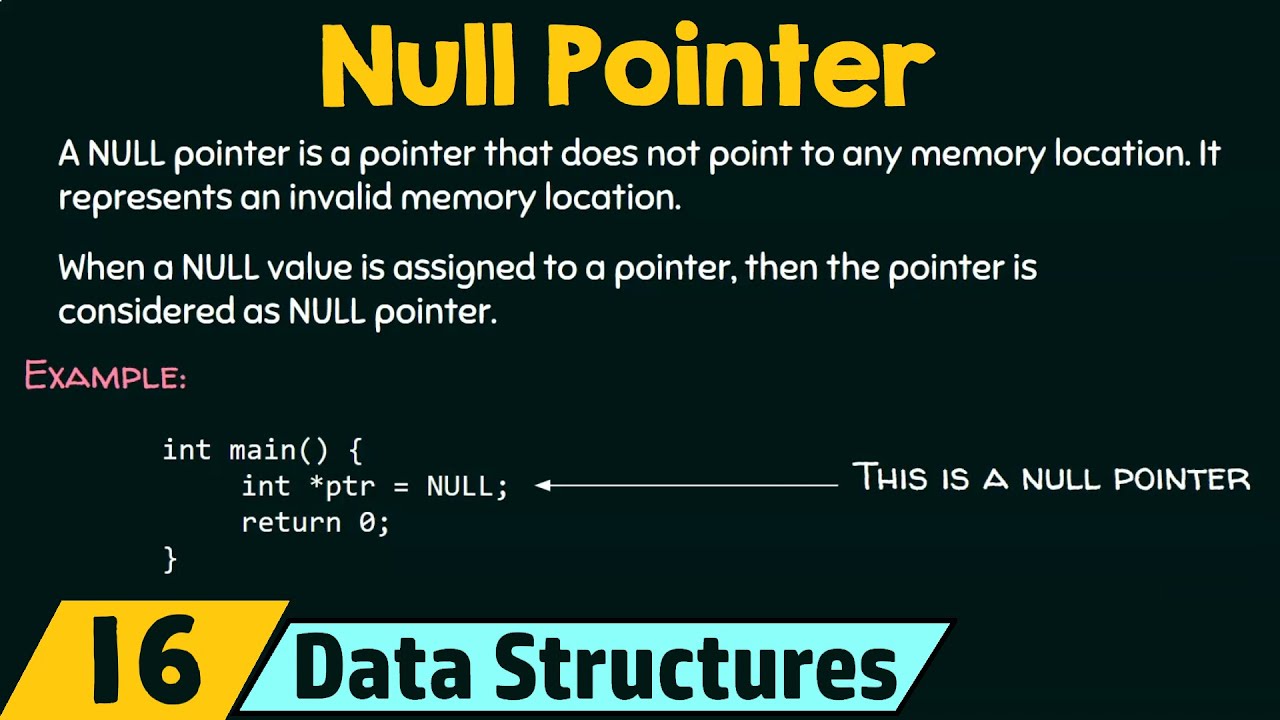
Understanding the Null Pointers

Variables & Data Types In C: C Tutorial In Hindi #6
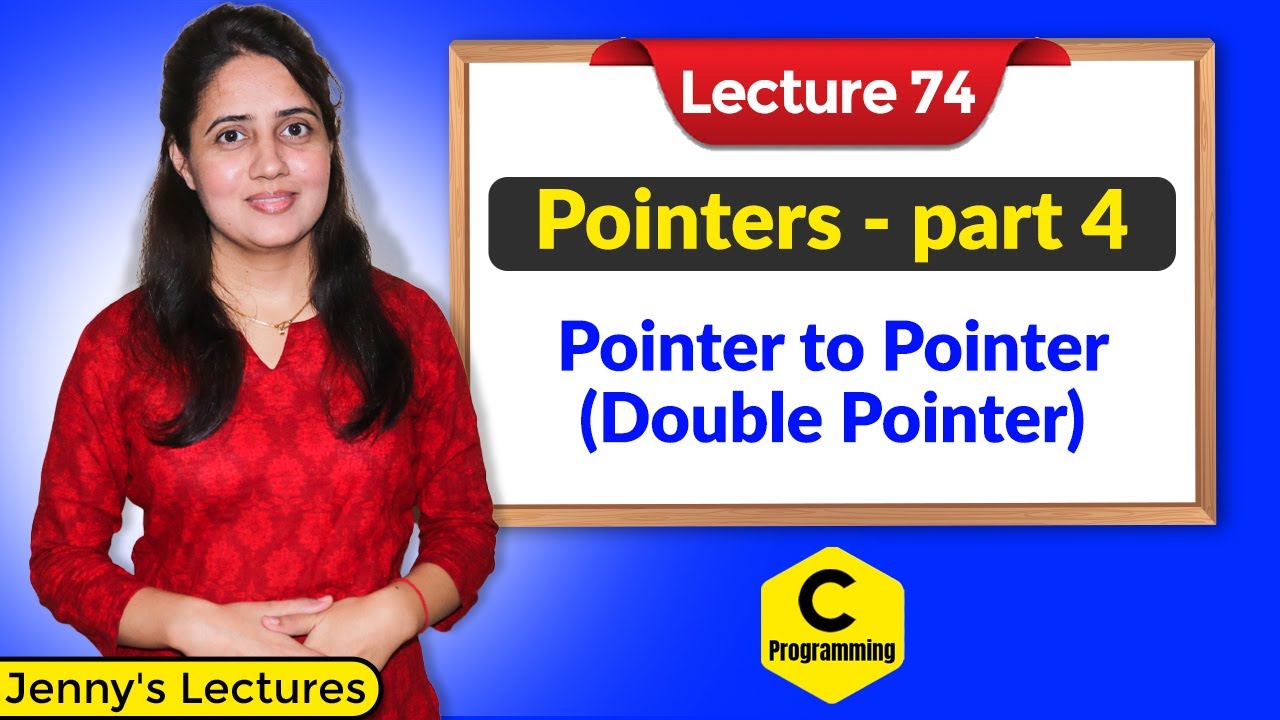
C_74 Pointers in C- part 4 | Pointer to Pointer (Double Pointer)
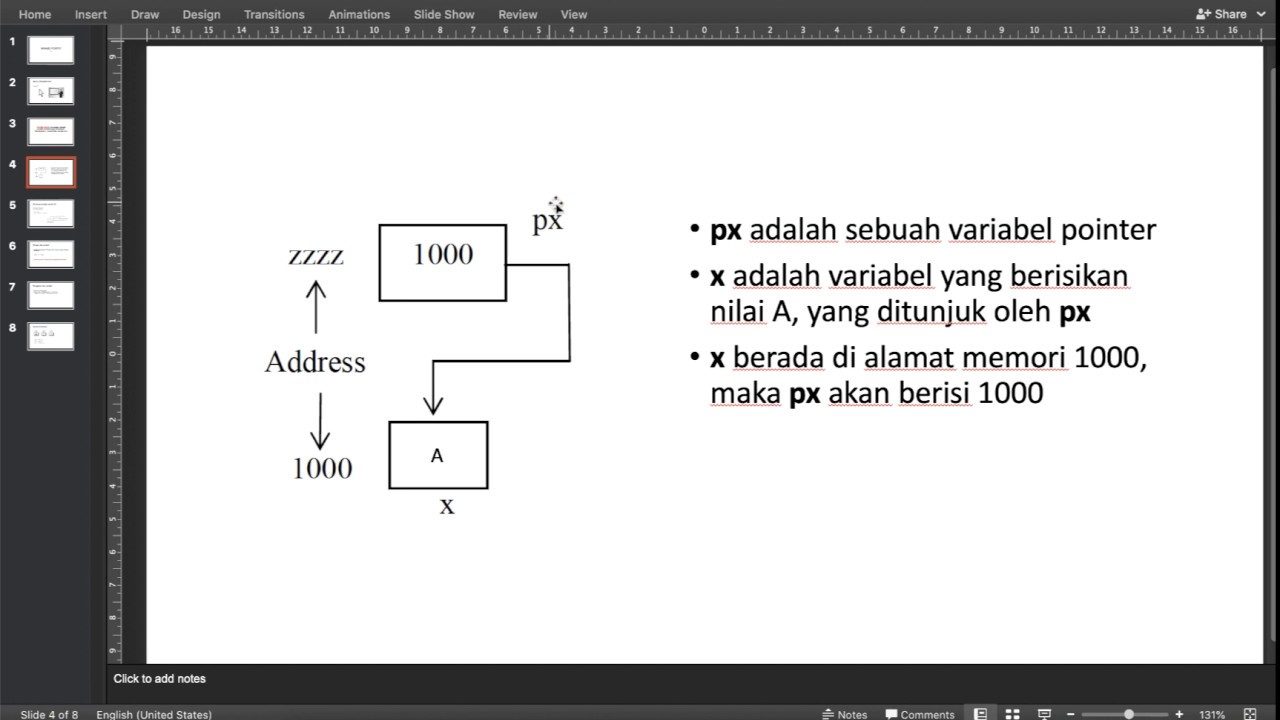
Variabel Pointer
5.0 / 5 (0 votes)