Programming Terms: First-Class Functions
Summary
TLDRIn this video, the concept of first-class functions is explored through examples in Python and JavaScript. The video explains how functions can be treated like any other variable: they can be assigned to variables, passed as arguments, and returned from other functions. Practical examples demonstrate the use of functions in higher-order functions like `map`, as well as closures. By the end of the video, viewers will understand the importance of first-class functions in programming and how they enable more powerful and flexible coding techniques.
Takeaways
- 😀 First-class functions are functions that can be treated like any other object or variable in programming.
- 😀 In programming languages with first-class functions, functions can be passed as arguments, returned as results, and assigned to variables.
- 😀 Understanding first-class functions helps with understanding other programming concepts such as higher-order functions, currying, and closures.
- 😀 A function can be assigned to a variable without executing it, which allows the variable to act as a function.
- 😀 In both Python and JavaScript, functions can be assigned to variables (without parentheses) and then called like normal functions.
- 😀 Higher-order functions are functions that accept other functions as arguments or return functions as results.
- 😀 The `map` function in both Python and JavaScript is an example of a higher-order function that applies a given function to each element in an array.
- 😀 Functions can be passed as arguments in custom-built functions, as seen with the example of a custom `map` function that accepts other functions like `square` or `cube`.
- 😀 Returning a function from another function is a key characteristic of first-class functions, which allows for the creation of dynamic behavior.
- 😀 The concept of closures arises when a function remembers the environment in which it was created, even after it has been returned and called outside its original scope.
- 😀 Practical use cases for returning functions include HTML tag generation and logging, where functions can be returned and executed with specific parameters later.
Q & A
What are first-class functions in programming?
-First-class functions are functions that are treated as first-class citizens in a programming language. This means they can be assigned to variables, passed as arguments to other functions, and returned as values from functions.
How do first-class functions relate to other programming concepts like higher-order functions and closures?
-Understanding first-class functions is crucial for grasping more complex concepts such as higher-order functions (functions that take other functions as arguments or return them as results) and closures (functions that remember the environment in which they were created).
What is the key difference between assigning the result of a function to a variable and assigning the function itself?
-When assigning the result of a function to a variable, you execute the function. However, when you assign the function itself (without parentheses), you are referencing the function rather than calling it, allowing you to use it as a first-class object.
Can you give an example of assigning a function to a variable?
-In the provided script, a function named 'Square' is assigned to the variable 'F' without parentheses. This means 'F' is now a reference to the 'Square' function, which can later be invoked by calling 'F()'.
What happens when we pass functions as arguments to other functions?
-Passing a function as an argument allows you to apply that function to data inside the receiving function. An example in the script is the custom 'map' function, which takes another function (like 'square') and applies it to each item in an array.
What is a higher-order function?
-A higher-order function is a function that either takes one or more functions as arguments or returns a function as its result. The 'map' function is an example, as it accepts a function and an array and applies the function to each item in the array.
Why is it important to avoid parentheses when passing functions as arguments?
-Parentheses are used to execute a function. To pass a function as an argument, you need to pass a reference to the function, not the result of executing it. This is why you should omit parentheses when passing functions as arguments.
What is the purpose of returning a function from another function, as shown with the 'logger' function?
-Returning a function from another function allows for dynamic behavior. The 'logger' function returns a 'logMessage' function, which remembers the message it was originally given, demonstrating how functions can carry state with them when returned.
What does the closure in the 'logger' function example demonstrate?
-The closure in the 'logger' function shows that the inner function ('logMessage') remembers the environment in which it was created, including the 'message' argument passed to the outer function ('logger'). This behavior is foundational for understanding closures.
What practical scenarios might use returning functions, like in the 'HTML tag' example?
-Returning functions is useful in many practical scenarios such as creating reusable templates, decorators, or event handlers. In the 'HTML tag' example, the returned 'wrapText' function allows for dynamic generation of HTML elements with custom messages, showing how function returns can be used to customize behavior.
Outlines
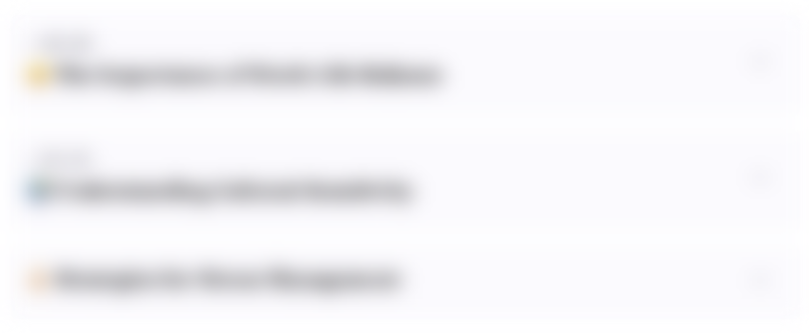
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
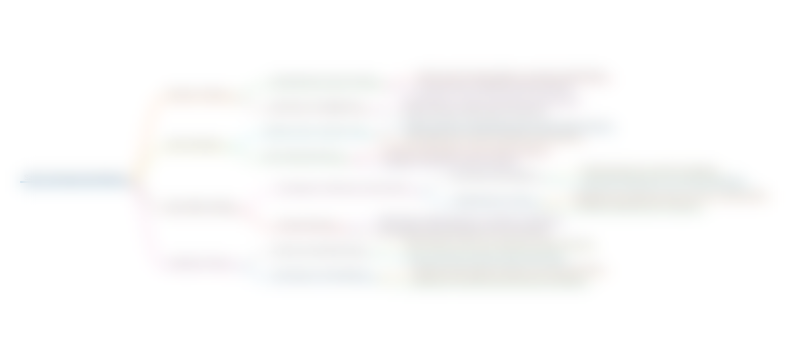
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
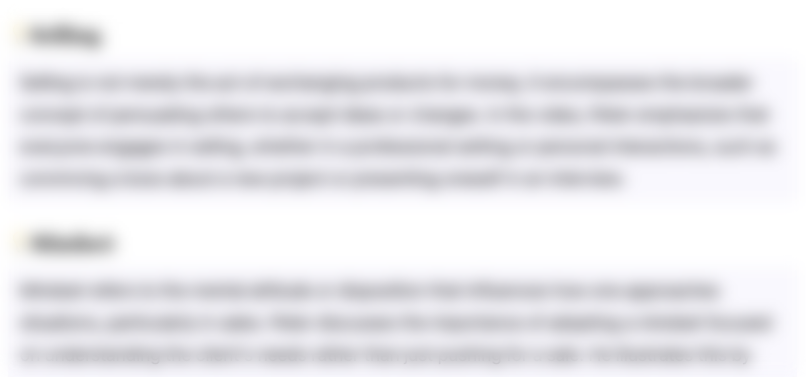
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
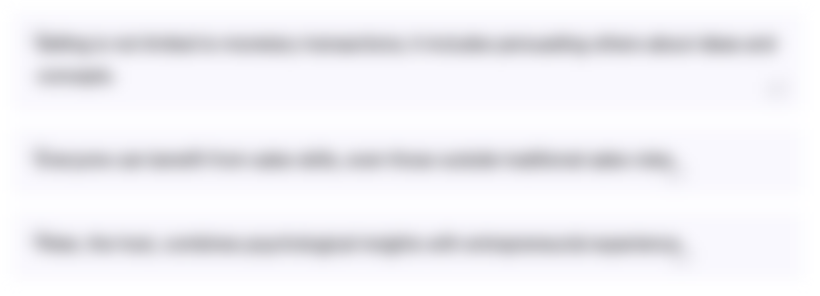
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
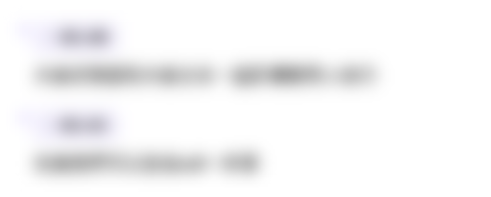
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео

First-Class and Higher-Order Functions | JavaScript 🔥 | Lecture 121
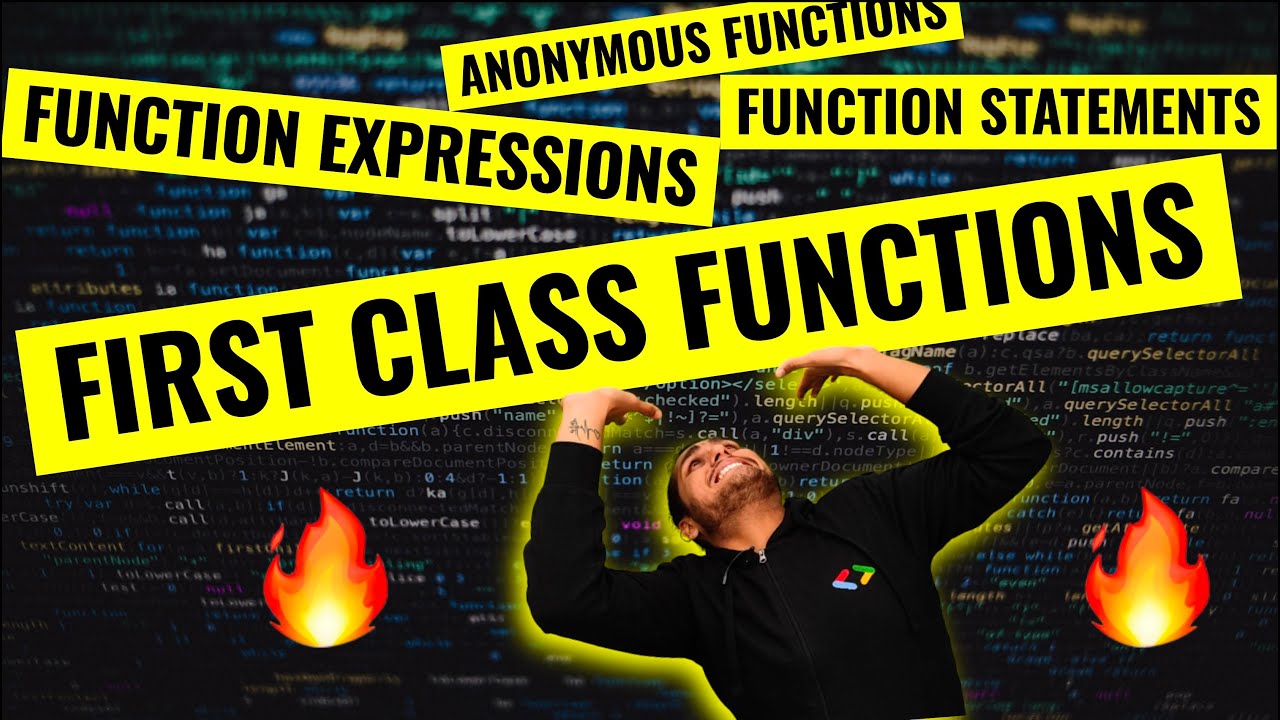
FIRST CLASS FUNCTIONS 🔥ft. Anonymous Functions | Namaste JavaScript Ep. 13

Learn Closures In 7 Minutes
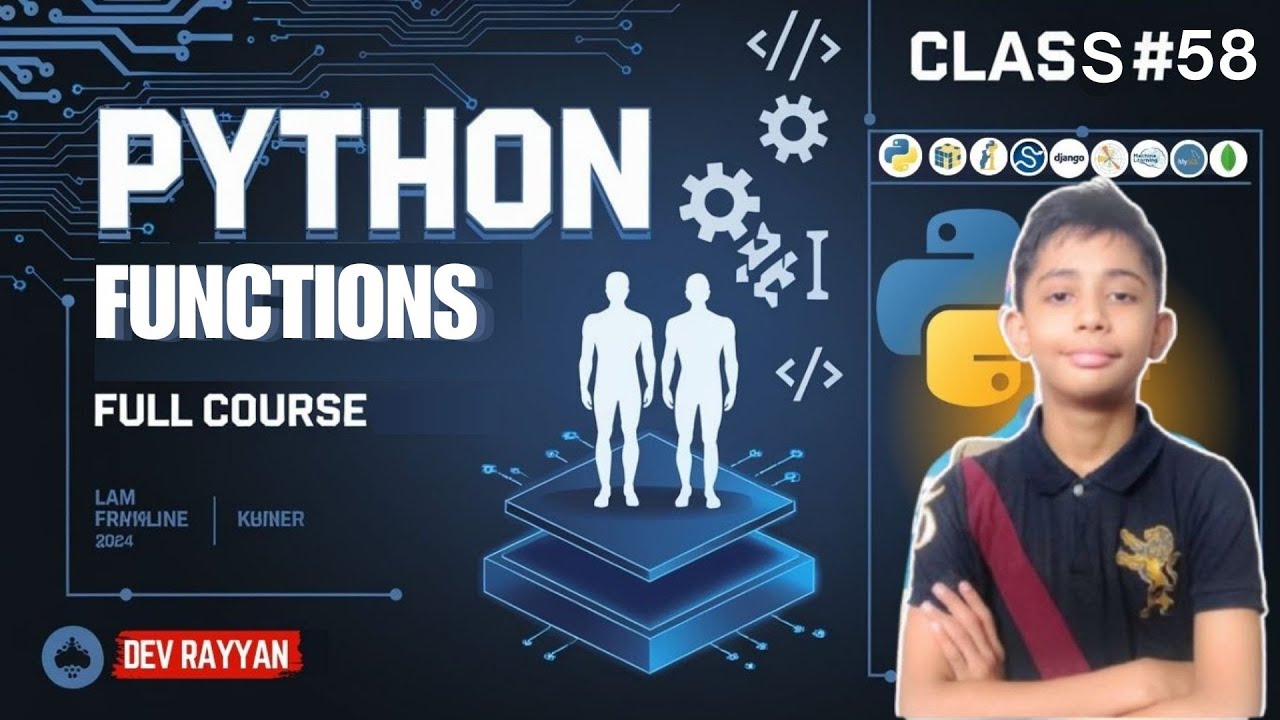
PYTHON FUNCTIONS | Python Tutorial - Lesson #58
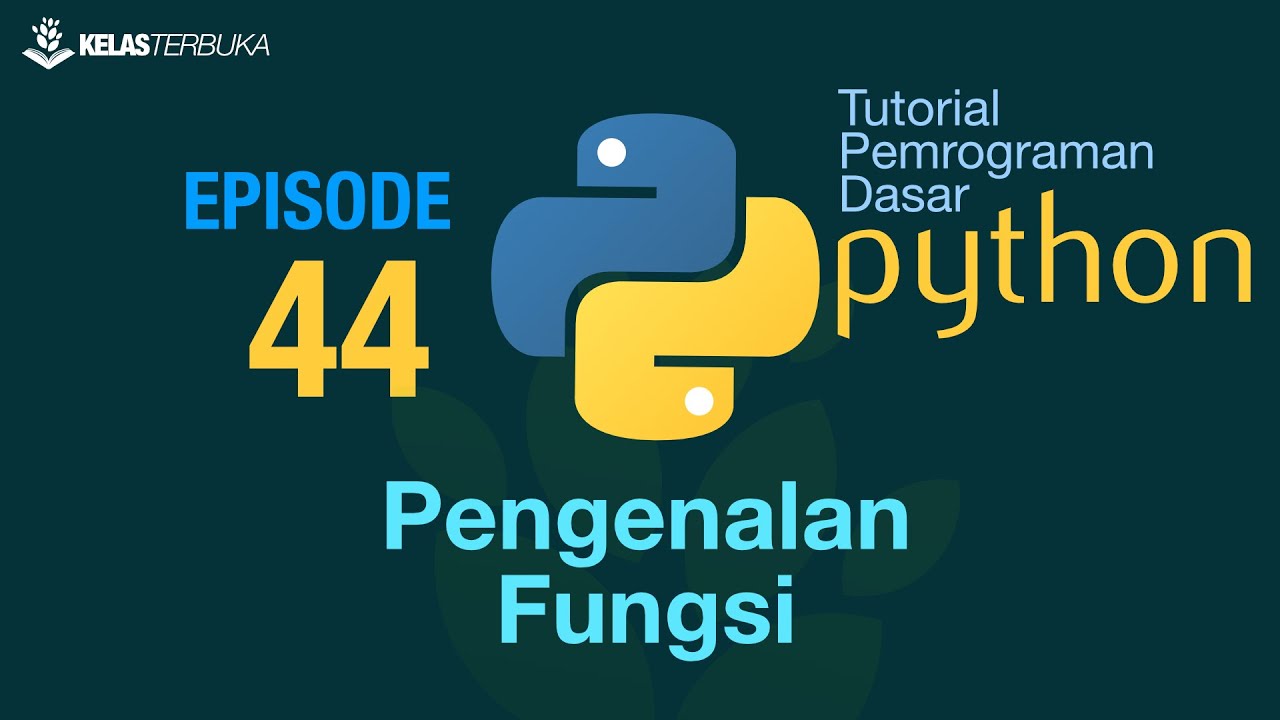
Belajar Python [Dasar] - 44 - Pengenalan Fungsi
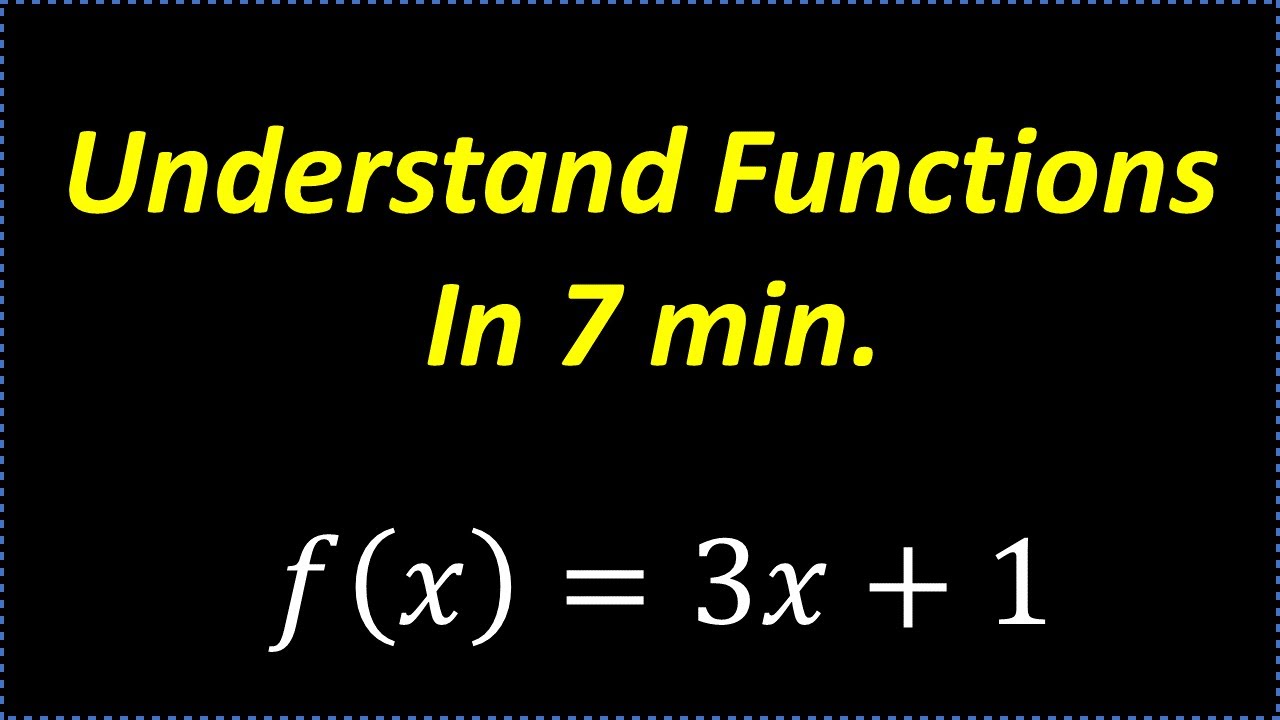
Learn Functions – Understand In 7 Minutes
5.0 / 5 (0 votes)