Javascript Interview Questions ( Functions ) - Hoisting, Scope, Callback, Arrow Functions etc
Summary
TLDRThis video script is an educational guide focused on JavaScript functions, aiming to prepare viewers for technical interviews. It covers function declarations and expressions, first-class functions, IIFEs, closures, and scopes. The script also delves into hoisting, the difference between params and arguments, and explores ES6 features like arrow functions. Practical examples and interview questions are provided to solidify understanding, making it a comprehensive resource for JavaScript developers looking to enhance their knowledge and interview skills.
Takeaways
- 😀 A function declaration in JavaScript is the process of defining a function using the `function` keyword followed by the function name and parameters.
- 🔑 A function expression in JavaScript involves assigning an anonymous function to a variable, which can be used later in the code.
- 🌟 First-class functions refer to the ability of functions in JavaScript to be treated like any other variable, meaning they can be passed as arguments, returned from other functions, and assigned to variables.
- 🎯 Immediately Invoked Function Expressions (IIFE) are functions that are defined and called immediately within the same statement, often used to create a local scope.
- 🔍 Closures are created when a function remembers the environment in which it was created, allowing it to access variables from its outer scope even after that scope has finished executing.
- 📚 Understanding function scope is crucial as it determines where a function looks for variables, with local variables taking precedence over global ones unless shadowed.
- 🚀 Hoisting in JavaScript refers to the behavior where variable and function declarations are moved to the top of their containing scope during the compilation phase, but functions are hoisted entirely, including their body.
- 🛠 The difference between 'params' and 'arguments' is that 'params' are the formal parameters defined by a function, while 'arguments' are the actual values passed to the function when it's called.
- 🔄 Spread and rest operators in JavaScript allow for the expansion of iterable objects such as arrays into individual elements, and the collection of multiple parameters into a single array, respectively.
- ➡️ Callback functions are functions passed into other functions as arguments, to be executed later, which is a common pattern in asynchronous programming and higher-order functions like `map`, `filter`, and `reduce`.
- 🏹 Arrow functions, introduced in ES6, provide a concise syntax for writing functions and do not bind their own `this`, `arguments`, `super`, or `new.target`, which can lead to different behavior compared to regular functions.
Q & A
What is a function declaration in JavaScript?
-A function declaration is a way to define a function in JavaScript using the 'function' keyword followed by the function name and its body enclosed in curly braces. It can also be referred to as a function definition or function statement.
How is a function expression different from a function declaration?
-A function expression in JavaScript is a function that is assigned to a variable. It can be anonymous, meaning it does not have a name, or named. Function expressions are typically used when the function is created at runtime or when the function is passed as an argument to another function.
What is an anonymous function and how is it used?
-An anonymous function is a function that does not have a name. It is often used as a callback or assigned to a variable. Anonymous functions are commonly used in event listeners, timeouts, or as arguments to higher-order functions like `map`, `filter`, and `reduce`.
Can you explain what first-class functions are?
-First-class functions are functions that can be treated like any other variable in JavaScript. They can be passed as arguments to other functions, returned from functions, and assigned to variables. This makes functions as flexible and powerful as any other data type in the language.
What is an Immediately Invoked Function Expression (IIFE) and why is it used?
-An Immediately Invoked Function Expression (IIFE) is a function that is defined and called immediately in JavaScript. It is often used to create a local scope, prevent variable conflicts, or to execute code that runs only once at the time of definition.
How does the scope of a function work in JavaScript?
-In JavaScript, the scope of a function determines the visibility of variables and functions within that function. Variables defined inside a function are local to that function and are not accessible from outside it. However, functions can access variables from their outer (parent) scope due to closures.
What is hoisting in JavaScript and how does it affect function declarations?
-Hoisting in JavaScript is a behavior where variable and function declarations are moved to the top of their containing scope during the compilation phase. For function declarations, this means the entire function is hoisted, allowing it to be called before it is declared in the code.
What is the difference between 'params' and 'arguments' in the context of functions?
-In JavaScript, 'params' refer to the formal parameters of a function, which are the variables listed in the function's definition. 'Arguments', on the other hand, are the actual values passed to the function when it is called. Params are local to the function, while arguments are the values received by the function.
Can you describe the spread operator and the rest operator in JavaScript?
-The spread operator (...) in JavaScript is used to expand elements of an iterable into individual arguments or parameters. It is often used with arrays and function calls. The rest operator is used to collect the remaining elements of an iterable into an array, and it must be the last element in a function's parameter list.
What is a callback function and how is it used in JavaScript?
-A callback function is a function that is passed into another function as an argument and is then invoked inside the outer function. Callbacks are used for asynchronous programming, event handling, and as a way to pass behavior to a function, allowing for more flexible and dynamic code.
How do arrow functions differ from traditional function declarations in JavaScript?
-Arrow functions in JavaScript, introduced in ES6, provide a shorter syntax for writing functions and do not have their own `this`, `arguments`, `super`, or `new.target`. They also cannot be used as constructors and do not have a `prototype` property. Arrow functions are often used for callbacks and to create concise, anonymous functions.
Outlines
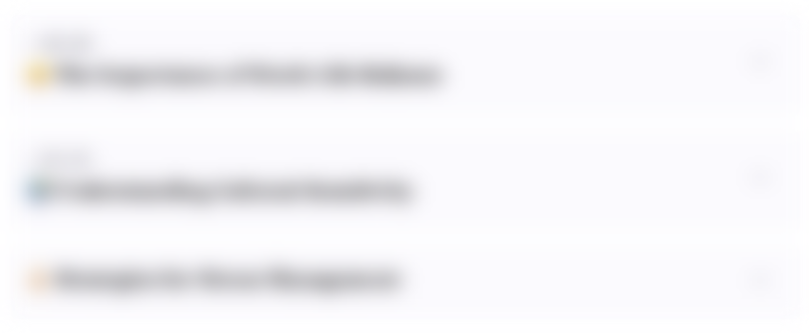
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
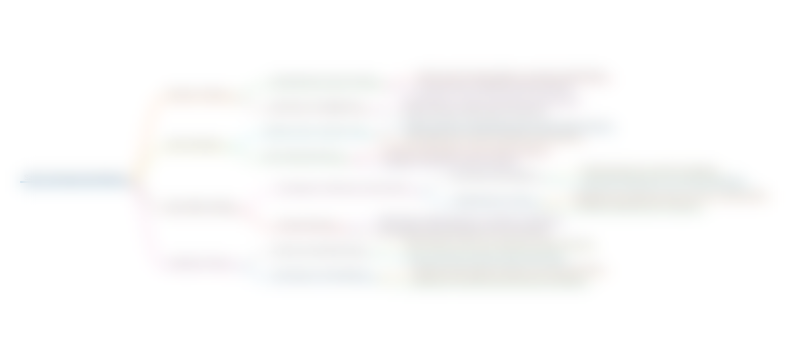
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
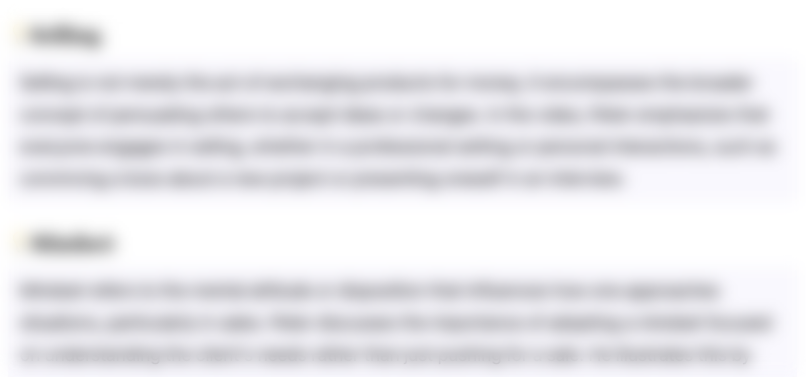
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
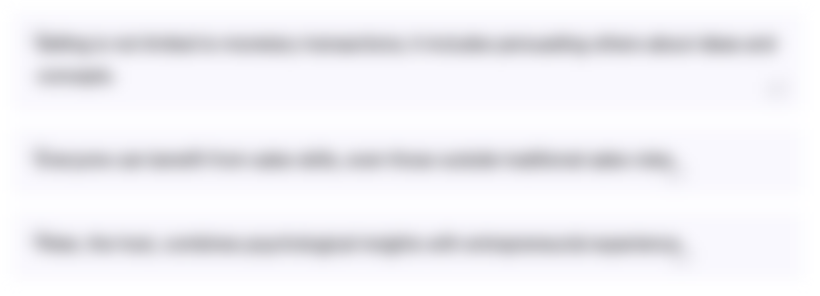
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
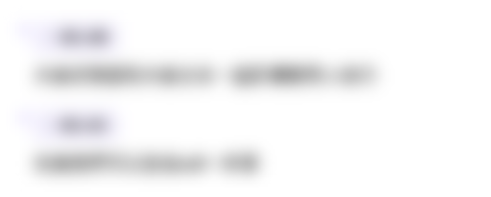
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Crack Your Java Interview With Most-Asked Questions | Java Fundamentals

A react interview question on counter
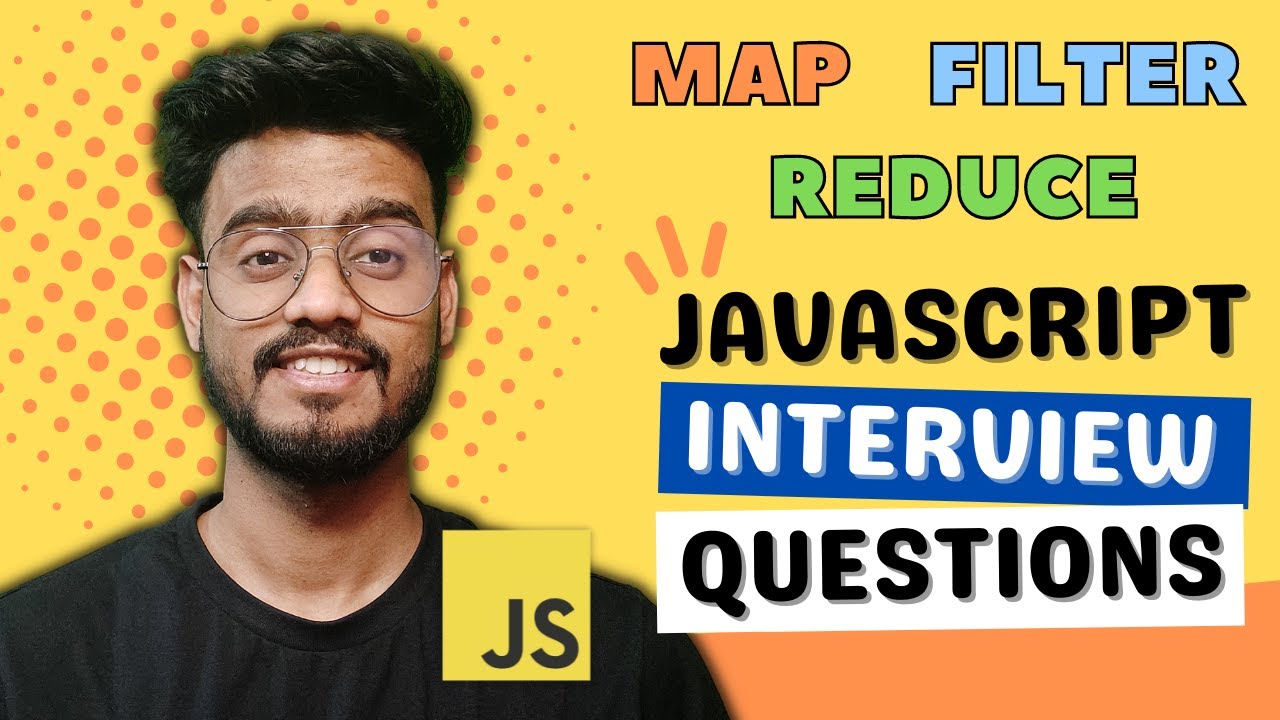
Javascript Interview Questions ( map, filter and reduce ) - Polyfills and Output Based Questions
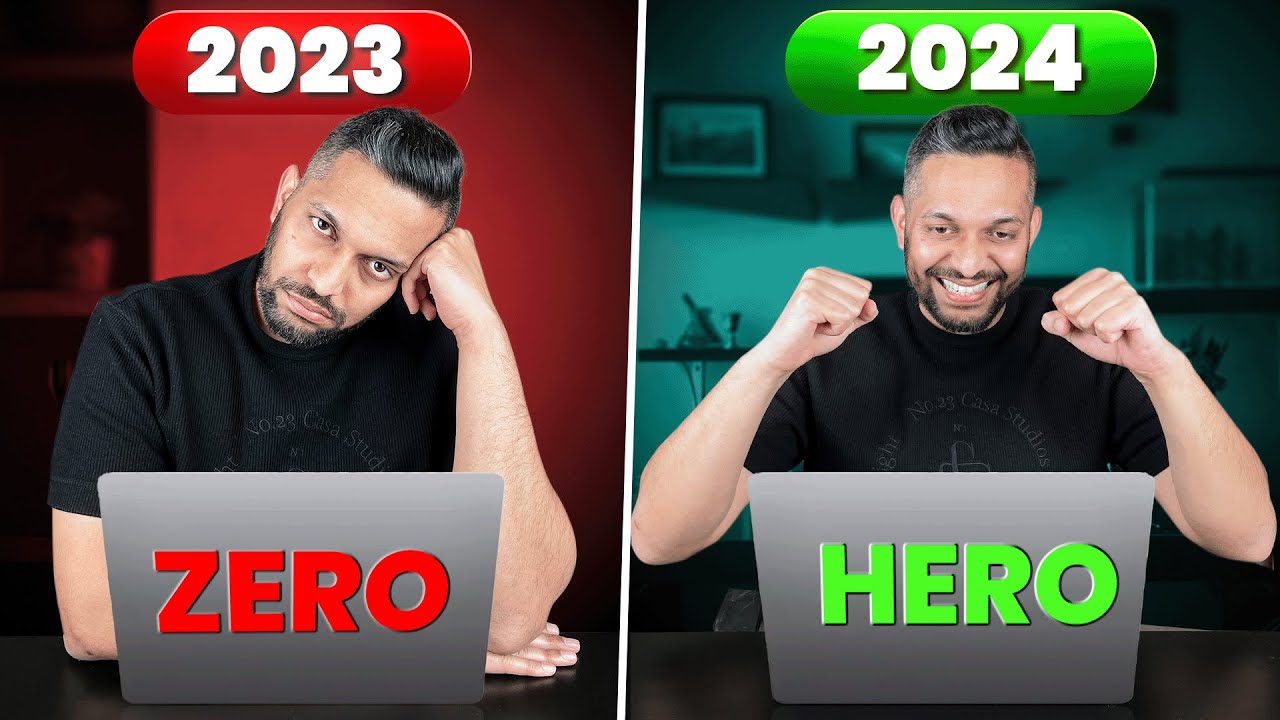
Fastest way to become a Software Engineer in 2024

How JavaScript Works 🔥& Execution Context | Namaste JavaScript Ep.1

All You Need To Know About Behavioral Interviews (for software engineers)
5.0 / 5 (0 votes)