1.1 Liskov Substitution Principle (LSP) with Solution in Java - SOLID Principles of Low Level Design
Summary
TLDRIn this insightful video, Shreyansh explains the Liskov Substitution Principle (LSP), a fundamental concept in object-oriented programming that ensures subclasses can replace parent classes without disrupting code functionality. Using a practical example of a `Vehicle` class and its child classes, including `Motorcycle`, `Car`, and `Bicycle`, he highlights potential issues when a subclass undermines the parent class's capabilities. Shreyansh offers a solution by introducing a separate `EngineVehicle` class to maintain functionality while avoiding null references, emphasizing the importance of proper inheritance design to ensure code safety and stability.
Takeaways
- 😀 The Liskov Substitution Principle (LSP) states that subclasses should be interchangeable with their parent classes without breaking the functionality of the program.
- 🚗 A basic `Vehicle` class can have different subclasses like `Motorcycle`, `Car`, and `Bicycle`, each representing different types of vehicles.
- 🔄 When substituting objects, the program should continue to function correctly regardless of which subclass is used.
- ⚠️ Violating the LSP can lead to runtime errors, such as null pointer exceptions, when a subclass does not adhere to the expected behavior of the parent class.
- 🔧 In the example, adding `Bicycle`, which has overridden `hasEngine()` to return null, breaks the code when checking if it has an engine.
- 📏 To avoid LSP violations, separate vehicle classes into those that have engines and those that do not, using an `EngineVehicle` subclass.
- 👨💻 By creating specific subclasses for vehicles with engines, the base `Vehicle` class remains generic and applicable to all types of vehicles.
- 🛠️ Client code should only access methods defined in the parent class to ensure safety and maintainability of the code.
- 📚 Proper inheritance and method overriding are essential to uphold the Liskov Substitution Principle.
- ✅ Following LSP allows for a more robust and error-free code structure, enabling easier maintenance and scalability.
Q & A
What is the Liskov Substitution Principle (LSP)?
-The Liskov Substitution Principle states that objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program.
How does the Liskov Substitution Principle relate to object-oriented programming?
-LSP is a fundamental principle of object-oriented programming that ensures derived classes can stand in for their base classes without causing issues in the code's functionality.
What example is used to illustrate the LSP in the transcript?
-The example involves a parent class `Vehicle` and child classes `Motorcycle`, `Car`, and `Bicycle` to demonstrate how substituting these objects can lead to errors if the principles of LSP are violated.
What problem arises when adding the `Bicycle` subclass to the list of vehicles?
-The `Bicycle` subclass violates the LSP because it overrides the `hasEngine()` method to return null, leading to a NullPointerException when the client code tries to call methods on it.
How can one avoid violating the Liskov Substitution Principle?
-To avoid violating LSP, define generic methods in the parent class and use composition for specific features in separate classes, ensuring that all subclasses adhere to the same interface.
What changes were suggested to the class structure to adhere to LSP?
-The suggested changes include creating an `EngineVehicle` class that inherits from `Vehicle` for vehicles with engines, while `Bicycle` should only inherit from `Vehicle` to prevent breaking the code.
What is the role of the `Vehicle` class in this example?
-The `Vehicle` class serves as the parent class containing common methods applicable to all vehicle types, such as `getNumberOfWheels()`.
What happens when you attempt to add a `Bicycle` to an `EngineVehicle` list?
-Attempting to add a `Bicycle` to an `EngineVehicle` list results in a compile-time error because `Bicycle` does not inherit from `EngineVehicle`.
Why is it important for the client code to be able to substitute different vehicle objects?
-It's important because it allows for flexibility and code reusability, enabling developers to work with various vehicle types without modifying the core logic of the program.
What is the consequence of not following the Liskov Substitution Principle in a codebase?
-Not following LSP can lead to fragile code that breaks when subclasses are used interchangeably, resulting in errors that can be difficult to trace and fix.
Outlines
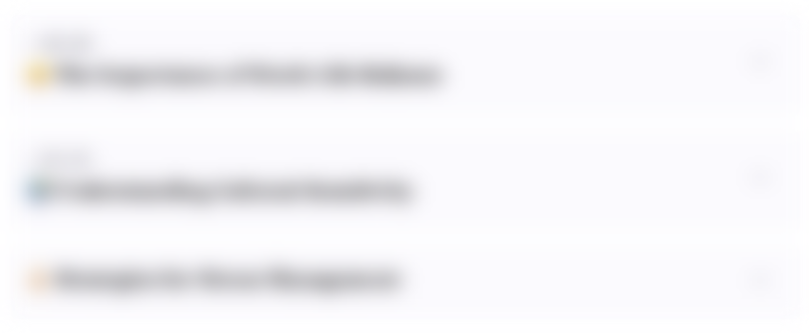
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
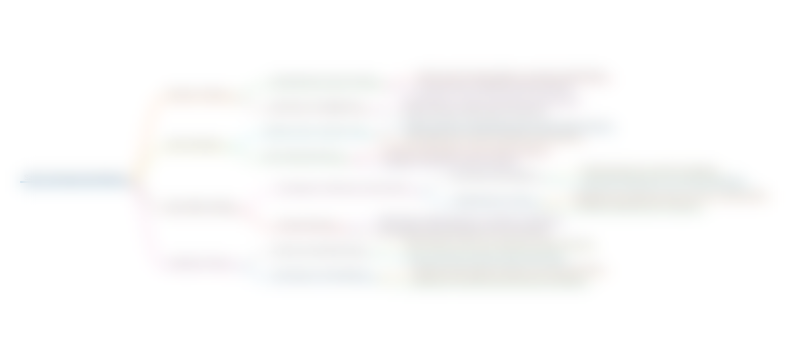
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
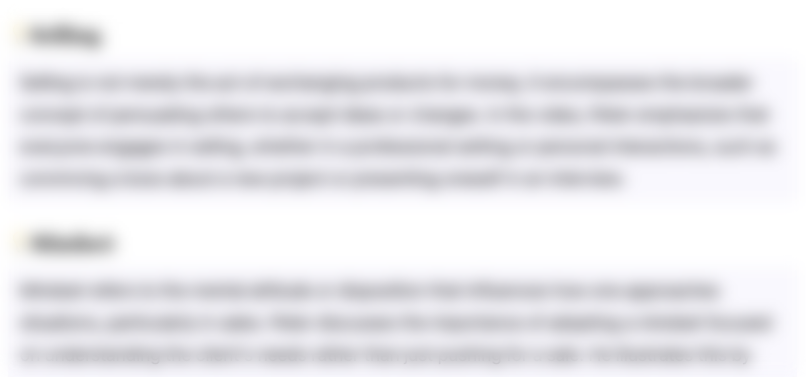
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
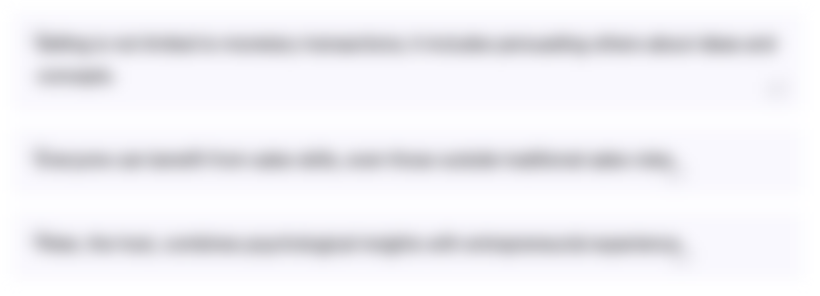
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
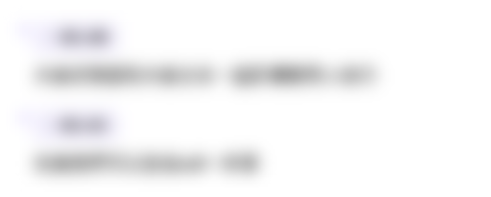
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео

PBO 2 - JAVA - Liskov Substitution
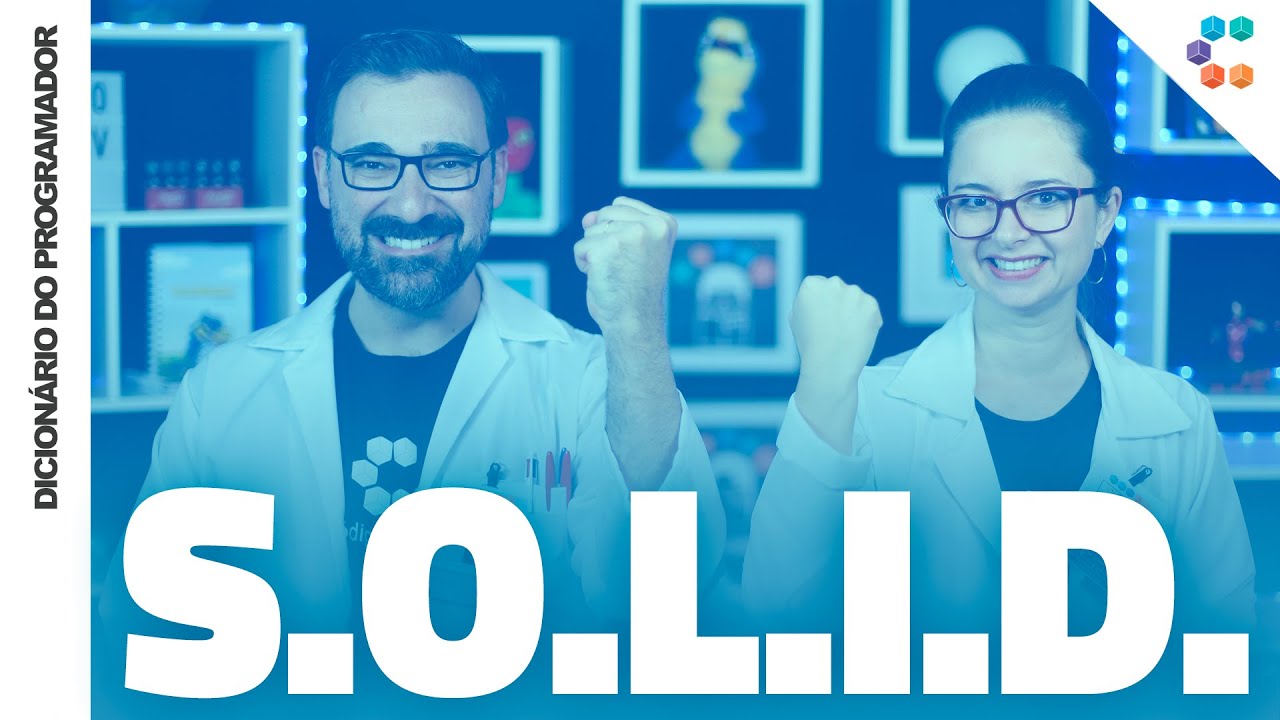
SOLID (O básico para você programar melhor) // Dicionário do Programador
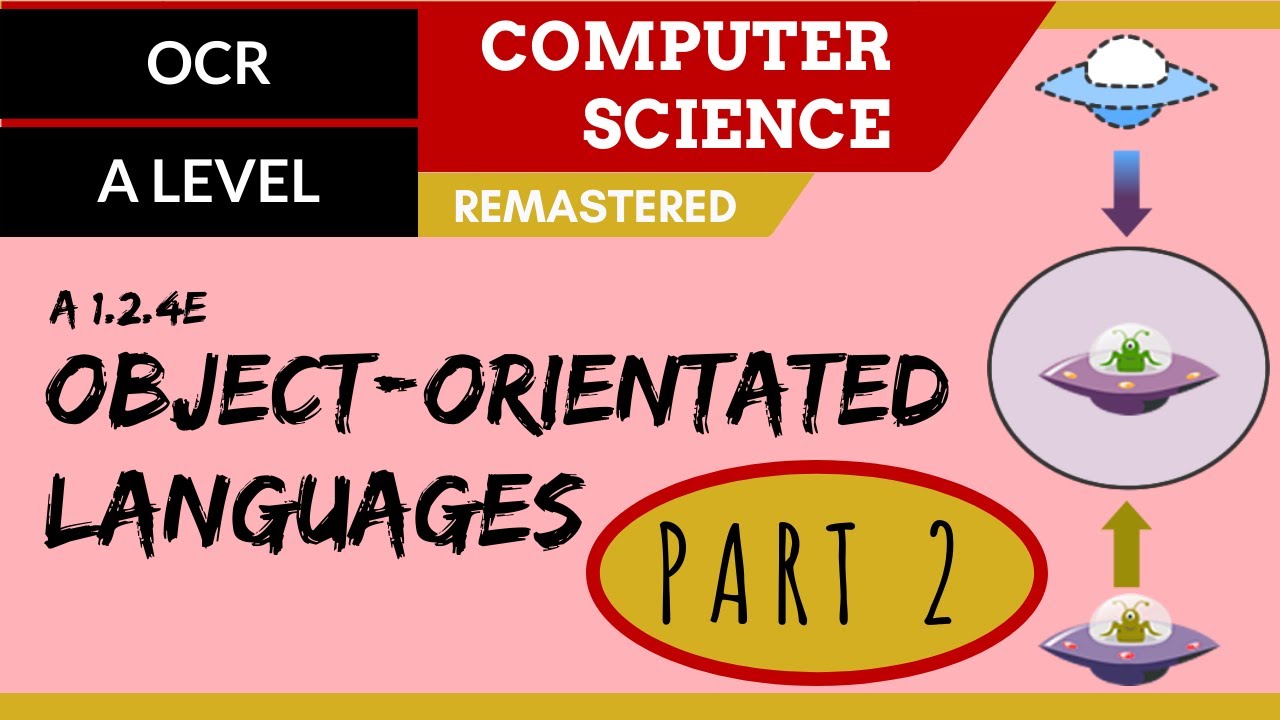
37. OCR A Level (H446) SLR7 - 1.2 Object-oriented languages part 2
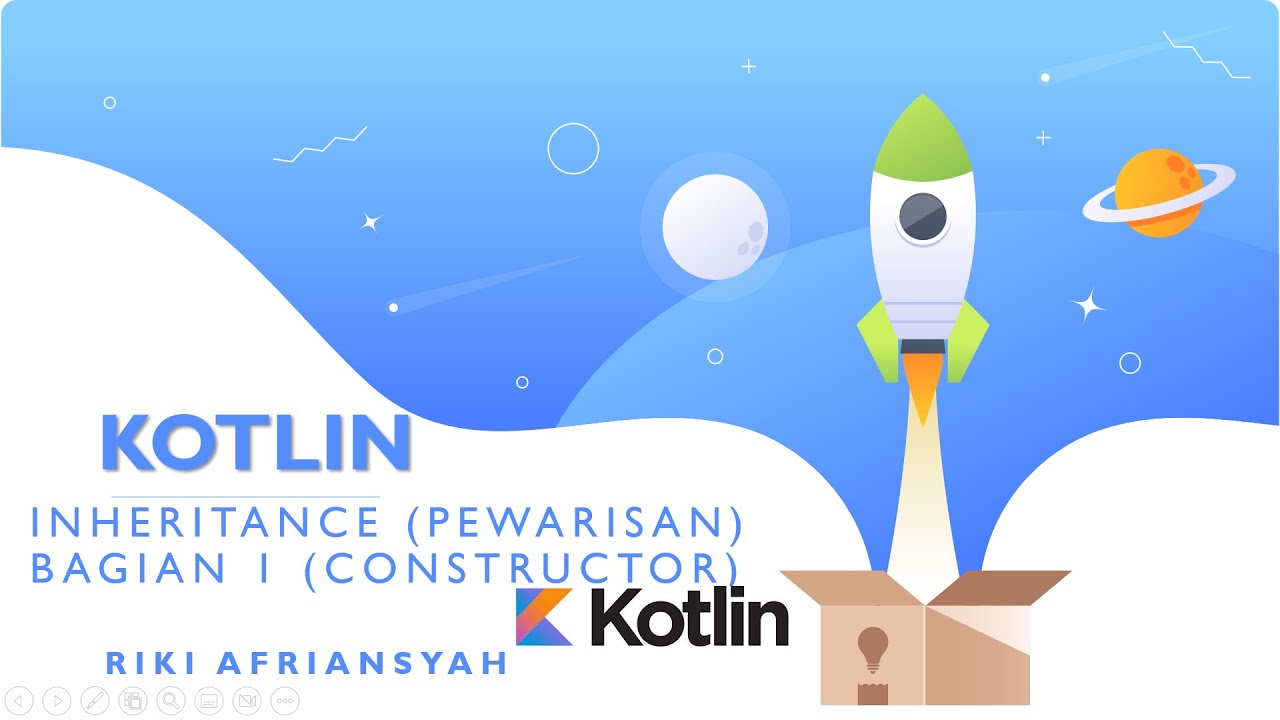
Inheritance (Pewarisan), Konsep Constructor inheritance Pada Kotlin

Java Polymorphism Fully Explained In 7 Minutes
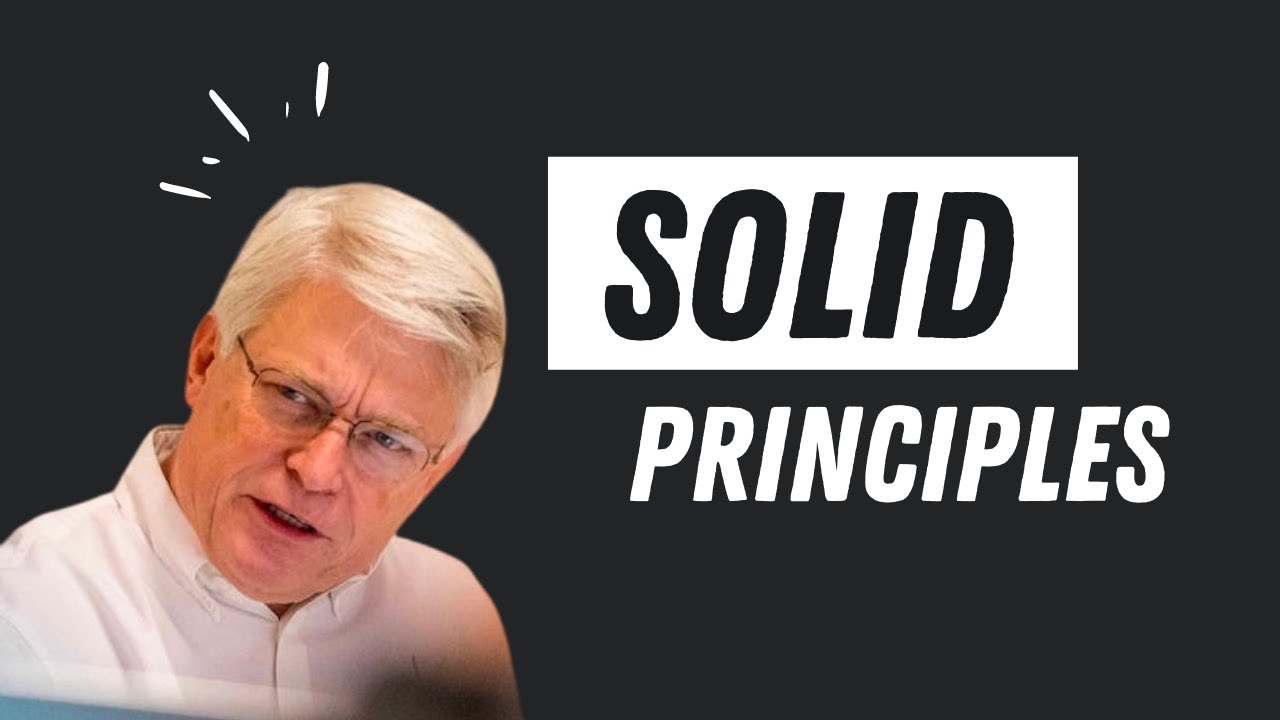
Learn SOLID Principles with CLEAN CODE Examples
5.0 / 5 (0 votes)