Some New Features in Python 3.11
Summary
TLDRThis video discusses new features in Python 3.11, including enhanced tracebacks for better error debugging, improvements in handling asynchronous tasks with task groups, and the introduction of exception groups for managing multiple exceptions simultaneously. The presenter demonstrates these updates with code examples, highlighting how Python 3.11 makes debugging and handling asynchronous processes more efficient. Additional features like performance improvements and updates to Python's typing module are briefly mentioned. Viewers are encouraged to explore these updates and share their thoughts on the usefulness of these new additions.
Takeaways
- 😀 Python 3.11 introduces improved tracebacks, offering more precise error location details compared to Python 3.10.
- 🔍 With Python 3.11, you can easily spot where a ZeroDivisionError occurs in a complex expression, saving debugging time.
- 🚀 The new Task Groups in async programming allow asynchronous tasks to be grouped and executed immediately, unlike the old gather method which waits for task completion.
- 🛠️ Task Groups don't require gathering results explicitly, making them ideal for cases where the result doesn't need to be returned.
- 📊 If results from tasks are needed, using asyncio.gather is more straightforward, but Task Groups offer a simpler syntax for non-result-driven tasks.
- 🔗 Python 3.11 introduces exception groups, allowing multiple exceptions to be raised and handled together, which is especially useful for asynchronous programming.
- ⚡ With the new `accept*` syntax, multiple exceptions within a group can be handled, unlike the traditional method that stops after the first match.
- 📝 Exception notes are a new feature that lets you attach additional information to exceptions, providing more context during debugging.
- 💡 The script demonstrates how to use exception groups and notes by showing how to manage multiple failed URL requests with informative error handling.
- ⏩ Python 3.11 includes performance improvements, making it faster globally without requiring changes to existing code, along with enhanced type hints.
Q & A
What is the main focus of the video?
-The video covers new features introduced in Python 3.11, focusing on improved tracebacks, task groups, exception groups, and performance improvements.
How has the traceback feature improved in Python 3.11?
-In Python 3.11, the traceback provides more precise information about where an error occurs, highlighting the exact part of the expression causing the issue, such as showing which variable is causing a zero division error.
What are task groups in Python 3.11, and how do they differ from using 'asyncio.gather'?
-Task groups are introduced in Python 3.11 as a more efficient way to manage asynchronous tasks. Unlike 'asyncio.gather', task groups start tasks immediately without needing to create a list of tasks, and the 'async with' block does not return until all tasks are completed.
Can you get the results of tasks in a task group? How?
-Yes, you can get the results of tasks in a task group by appending each task to a results list and later accessing the results using a list comprehension. This approach gives you similar functionality to 'asyncio.gather'.
What are exception groups, and when would you use them?
-Exception groups allow multiple exceptions to be raised and handled at the same time, which is particularly useful in asynchronous programming where several tasks can fail for different reasons. They enable you to manage multiple exceptions in a single block.
How do 'accept *' blocks work in Python 3.11?
-'Accept *' blocks in Python 3.11 allow multiple exception handling blocks to run for different types of errors within the same try-except statement. Each applicable exception is handled separately without exiting after the first match, unlike traditional except blocks.
What is the purpose of the 'add_note' method in Python 3.11?
-The 'add_note' method allows developers to attach additional information to exceptions without needing to create custom exceptions. For example, you can add extra details like URLs or context-specific notes to the exception for debugging purposes.
What other features are included in Python 3.11 besides task groups and exception groups?
-In addition to task groups and exception groups, Python 3.11 brings speed improvements, enhancements to the typing module, and other performance optimizations that benefit developers without requiring code changes.
Are there performance improvements in Python 3.11, and do they require code changes?
-Yes, Python 3.11 includes speed improvements, but these enhancements do not require code changes. Developers can continue writing code as usual and benefit from the performance boost automatically.
Why might a developer choose to use task groups instead of 'asyncio.gather'?
-A developer might choose task groups over 'asyncio.gather' because task groups start tasks immediately, simplifying code by removing the need for creating and appending tasks to a list. They are particularly useful when task results are not needed.
Outlines
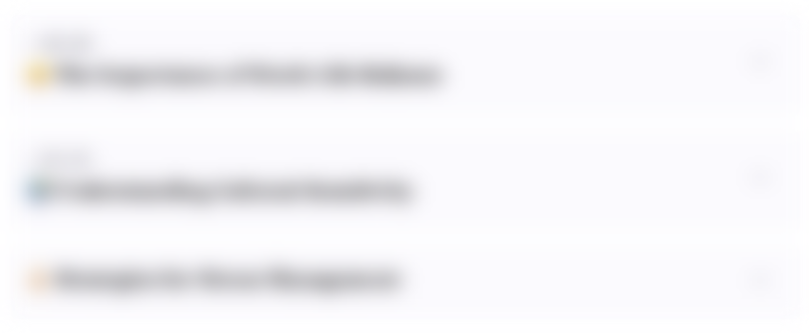
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
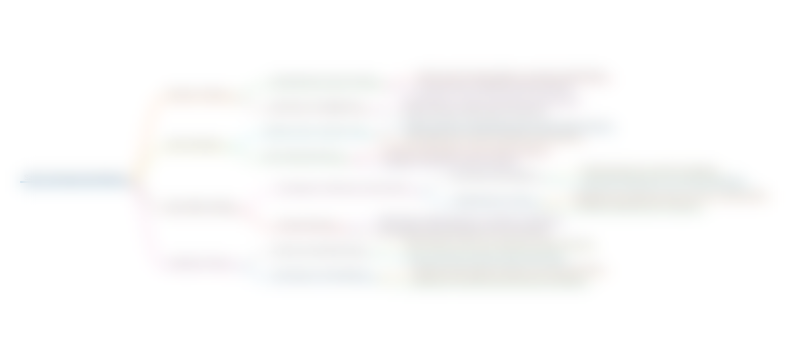
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
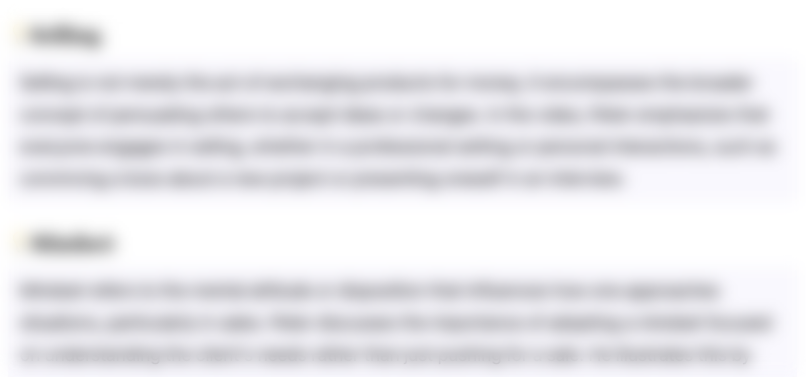
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
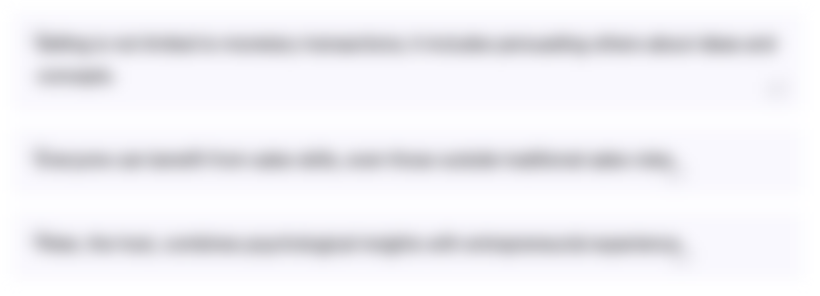
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
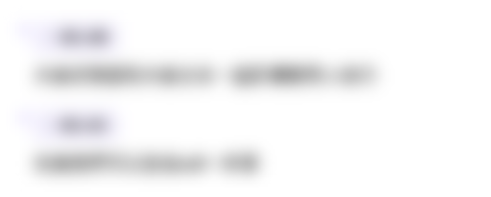
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
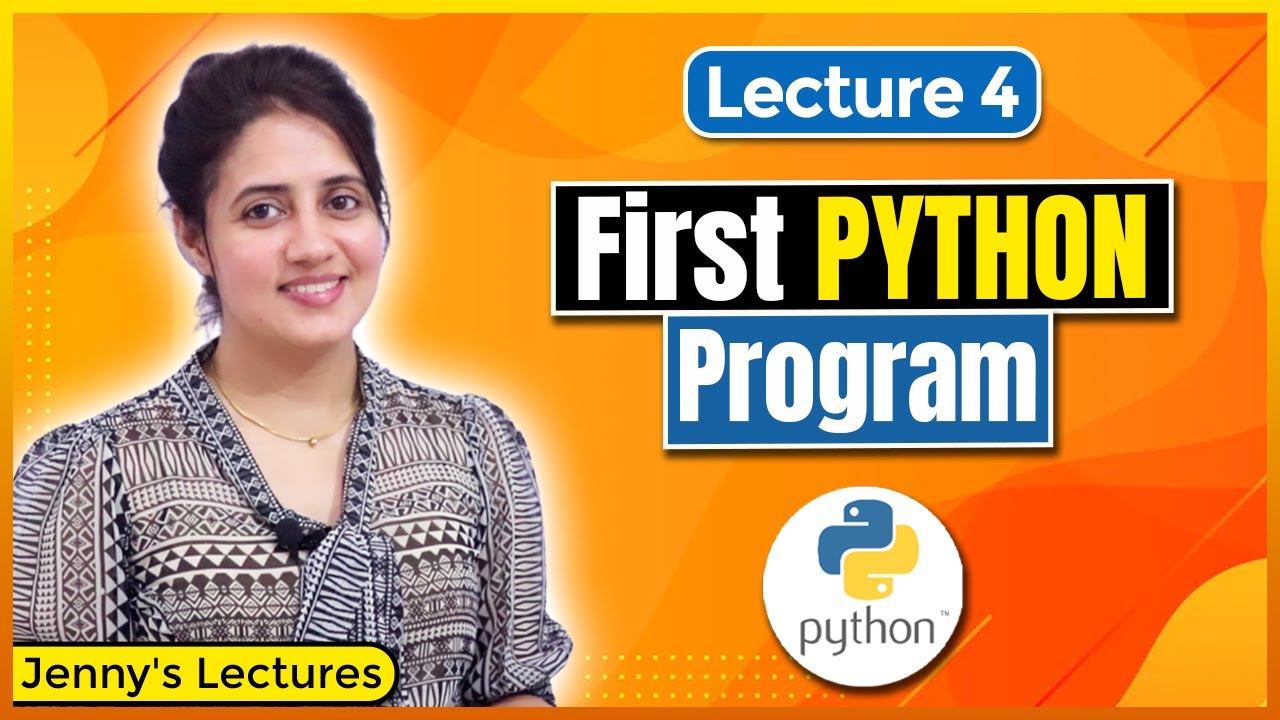
Writing First Python Program | Printing to Console in Python | Python Tutorials for Beginners #lec4
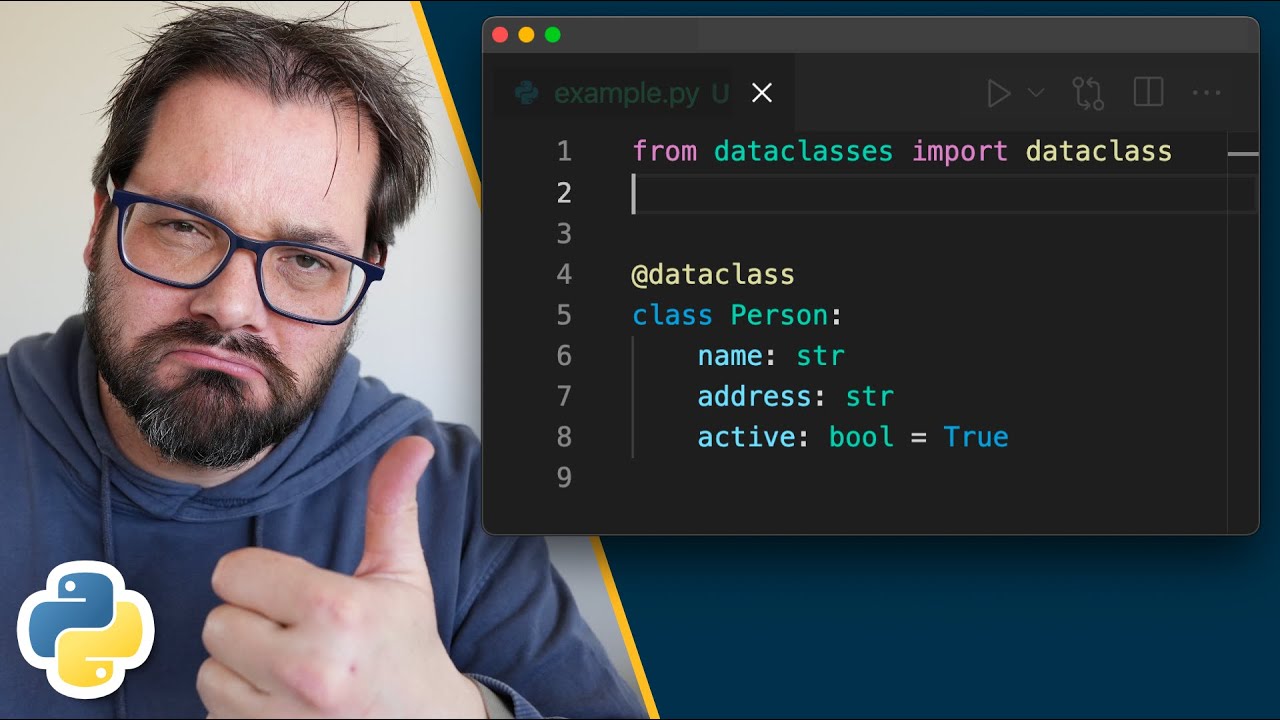
This Is Why Python Data Classes Are Awesome
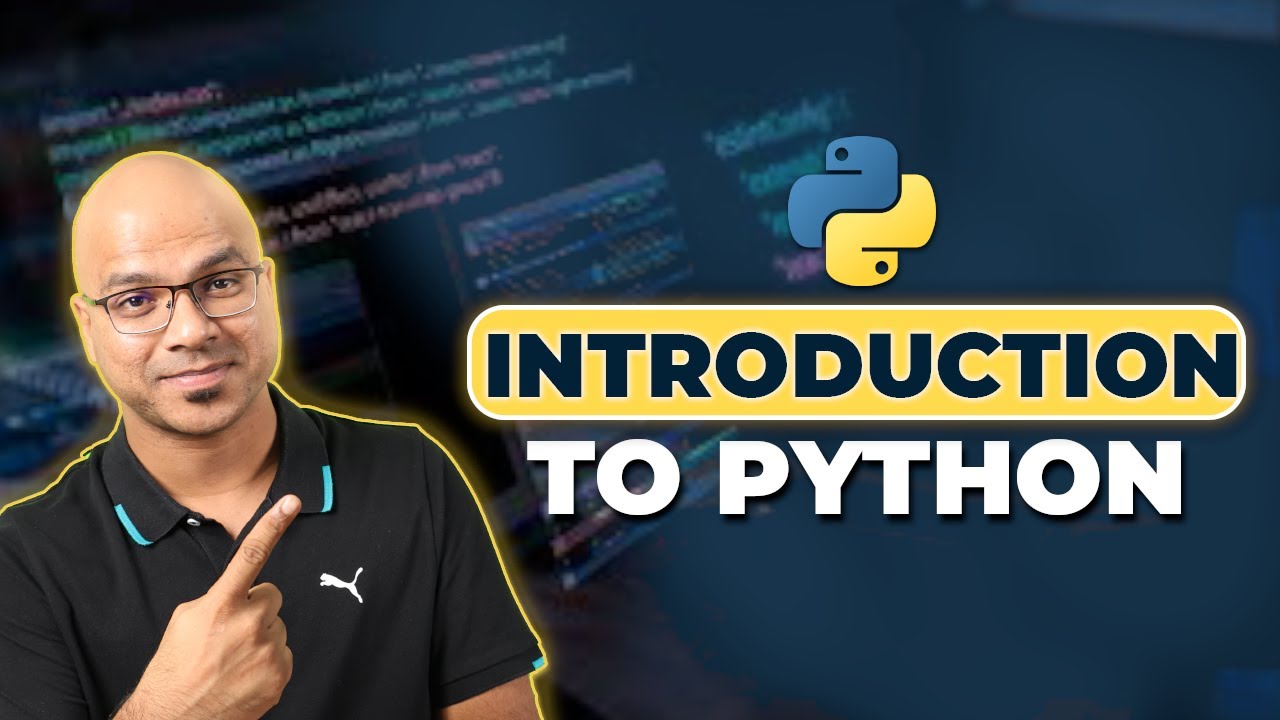
#1 Python Tutorial for Beginners | Introduction to Python

Quest 3S Hands On - Meta Quest 3 Just Got More Affordable!
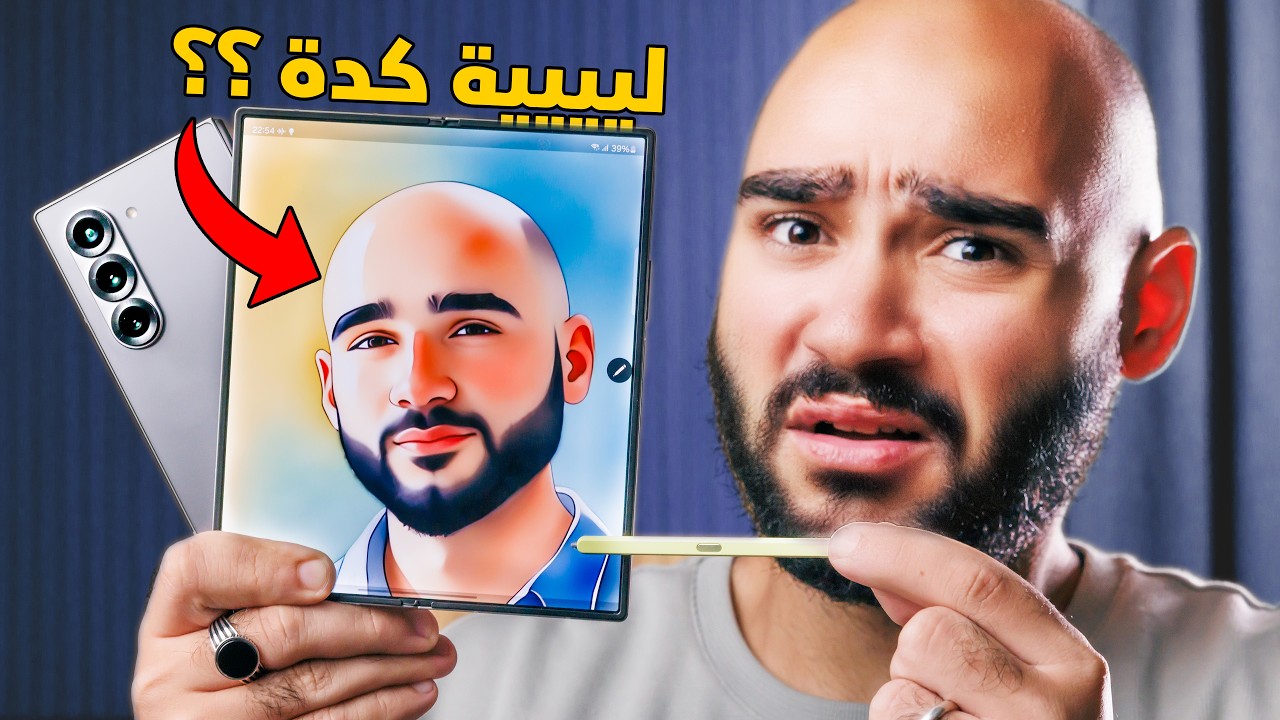
Samsung Z Fold 6 || غير متوقع تماماً !!
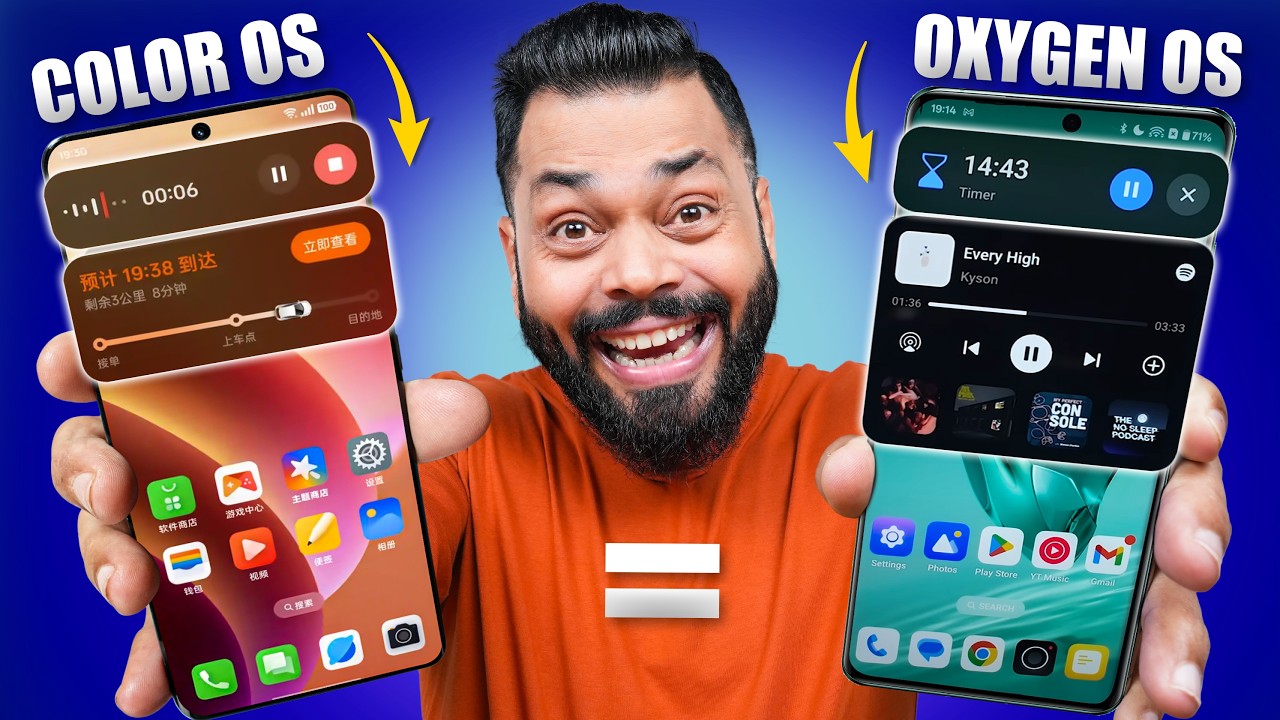
Top 15 Crazy Features Of OxygenOS 15 ⚡ ft.ColorOS 15 & realmeUI 6.0 🫣
5.0 / 5 (0 votes)