Numpy
Summary
TLDRThe lecture introduces the NumPy library, emphasizing its importance for numerical operations in Python. It covers the creation and manipulation of arrays, explaining how to process multi-dimensional data efficiently. The instructor demonstrates various functions like reshaping, indexing, and mathematical operations on arrays. The tutorial also touches on nested lists and how to convert them into NumPy arrays, showcasing the library's capabilities for scientific computing.
Takeaways
- 😀 The lecture introduces the NumPy library, a fundamental package for numerical operations in Python.
- 📚 NumPy arrays are used for efficient processing of multi-dimensional data and are integral to scientific computing in Python.
- 🔢 The script explains how to create and manipulate arrays, including reshaping and accessing elements, which is crucial for data analysis.
- 💻 The tutorial demonstrates installing the NumPy library, a prerequisite for using its functionalities in any Python environment.
- 🎯 The use of specific functions like `np.array()` is highlighted for creating arrays, emphasizing the need for correct data types.
- 📊 The lecture covers how to perform mathematical operations on arrays, such as addition, subtraction, and multiplication, which are essential for data manipulation.
- 🔄 The concept of array reshaping is introduced, allowing for the transformation of array dimensions, which is useful for certain data processing tasks.
- 📈 The script also touches on more advanced topics like nested lists and the creation of multi-dimensional arrays for complex data structures.
- 🛠️ Practical examples of array operations are provided, such as calculating the sum or mean of array elements, which are common in data analysis.
- 🔍 The lecture concludes with insights on how NumPy arrays can be used for mathematical and logical operations on multi-dimensional data.
Q & A
What is the primary focus of the lecture?
-The primary focus of the lecture is to introduce the NumPy library, explain its significance, and demonstrate how to utilize it for numerical operations and processing multi-dimensional data arrays in Python.
What does the NumPy library stand for?
-NumPy stands for Numerical Python, and it is a library that provides support for large, multi-dimensional arrays and matrices, along with a large collection of high-level mathematical functions to operate on these arrays.
Why is NumPy important for scientific computing in Python?
-NumPy is important for scientific computing in Python because it provides an efficient array object and tools for working with arrays, which are fundamental for various types of numerical computations and data processing tasks.
What is the difference between a Python list and a NumPy array?
-A Python list is a built-in data structure that can hold a collection of items, which can be of different data types. A NumPy array, on the other hand, is a homogenous data type that is more compact and efficient in terms of memory usage and performance, making it suitable for large data sets.
How can one create a NumPy array?
-One can create a NumPy array by using the `np.array` function, which converts a Python list or a tuple into a NumPy array. Additionally, one can also create arrays using functions like `np.zeros`, `np.ones`, or `np.arange` for more specific use cases.
What are the benefits of using NumPy arrays over Python lists for numerical operations?
-NumPy arrays offer several benefits over Python lists for numerical operations, including faster execution due to internal optimizations, less memory consumption due to homogeneous data types, and the ability to perform vectorized operations, which allow element-wise operations without the need for explicit loops.
How can you reshape a NumPy array?
-You can reshape a NumPy array using the `reshape` method, which allows you to change the shape of the array without altering its data. The method requires a tuple specifying the new dimensions as an argument.
What is the significance of the 'ndarray' object in NumPy?
-The 'ndarray' object in NumPy is a multi-dimensional array that is the primary data structure in NumPy, providing an efficient way to store and operate on large amounts of data. It supports operations like slicing, reshaping, and broadcasting.
How can you install the NumPy library if it's not already available in your Python environment?
-You can install the NumPy library using a package manager like pip. The command to install NumPy is `pip install numpy`, which can be executed in a terminal or command prompt.
What are some common mathematical operations that can be performed using NumPy functions?
-Common mathematical operations that can be performed using NumPy functions include addition, subtraction, multiplication, division, and more advanced operations like exponentials, logarithms, and trigonometric functions. These operations can be vectorized to act on entire arrays at once.
How does NumPy handle broadcasting in array operations?
-NumPy's broadcasting feature allows operations on arrays of different shapes. During an operation, NumPy compares the dimensions of the arrays and executes the operation by 'broadcasting' the smaller array across the larger one, filling in missing dimensions with copies of the smaller array's data.
Outlines
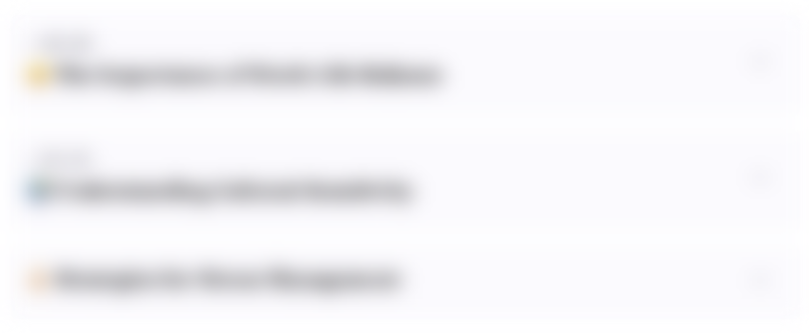
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
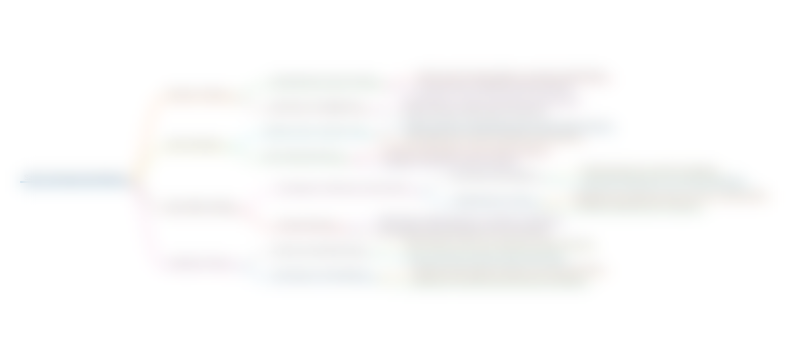
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
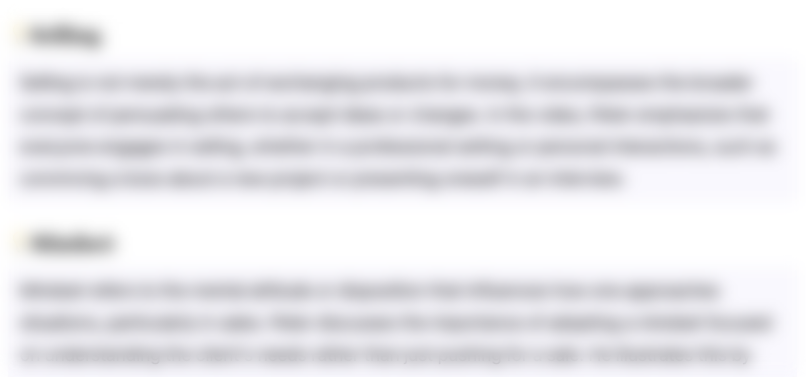
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
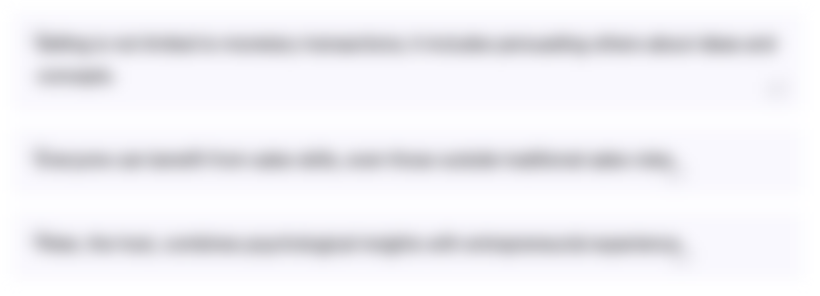
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
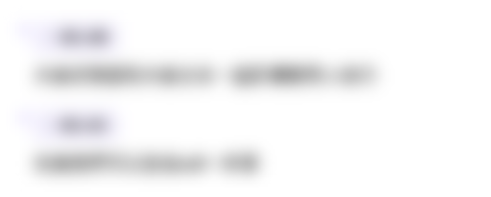
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тариф5.0 / 5 (0 votes)