Introduction to Pointers in C
Summary
TLDRIn this lecture, the concept of pointers in C programming is introduced as a crucial topic. The speaker uses an example of a 20-byte memory with blocks, each storing one byte, to explain how an integer can be stored in two blocks starting from address 1002. A pointer is defined as a special variable that stores the address of another variable or object, in this case, the integer variable 'i'. The lecture aims to provide an initial understanding of pointers and sets the stage for learning how to declare pointers in future sessions.
Takeaways
- 💡 The lecture introduces a new topic: pointers in C programming, emphasizing its importance.
- 💻 The speaker uses an example of a computer memory with a capacity of 20 bytes, each block storing one byte.
- 📍 The memory is assumed to have a starting address of 1000 and an ending address of 1019.
- 🔢 The speaker plans to store an integer in this memory, which is assumed to occupy two bytes.
- 📌 A variable 'i' of integer type is introduced to demonstrate how it can store an integer using two blocks of memory.
- 👉 Pointers are defined as variables that store the initial address of another object they refer to.
- 🏷️ The pointer in the example is assumed to point to the variable 'i', storing its base address, which is 1002.
- 📚 A pointer is a special variable that can store addresses, differing from normal variables that store data types like integers or characters.
- 🔑 The pointer points to the memory location where the first byte of the variable is stored, highlighting its role in memory allocation.
- 🚀 The lecture provides an overview of pointers and sets the stage for future lessons on declaring pointers in C.
Q & A
What is the primary focus of the lecture?
-The primary focus of the lecture is to introduce the concept of pointers in C programming.
How much memory is assumed to be available in the computer for the example?
-The computer's memory is assumed to be capable of storing 20 bytes of information.
What is the starting and ending address of the memory in the example?
-The starting address of the memory is 1000 and the ending address is 1019.
What is the maximum memory an integer is assumed to take in this context?
-An integer is assumed to take up to a maximum of two bytes of memory.
What is the purpose of the variable 'i' in the example?
-The variable 'i' is of integer type and is used to store an integer, taking up two blocks of memory.
What does the term 'pointer' refer to in the context of C programming?
-A pointer is a variable that can store the initial address of an object it points to.
What is the assumed base address of the object 'i' in the example?
-The base address of the object 'i' is assumed to be 1002.
What is the definition of a pointer given in the lecture?
-A pointer is a special variable capable of storing an address, specifically the base address of an object or variable it points to.
Why is the pointer considered special in comparison to normal variables?
-Pointers are special because they do not store data types like integers, characters, or floats; instead, they store the memory addresses of these data types.
What is the next topic to be covered in the series of lectures?
-The next topic to be covered is how to declare pointers in C programming.
What is the main takeaway from the lecture regarding pointers?
-The main takeaway is that pointers are used to store the memory addresses of variables or objects, and they point to the location where the first byte of the object is stored.
Outlines
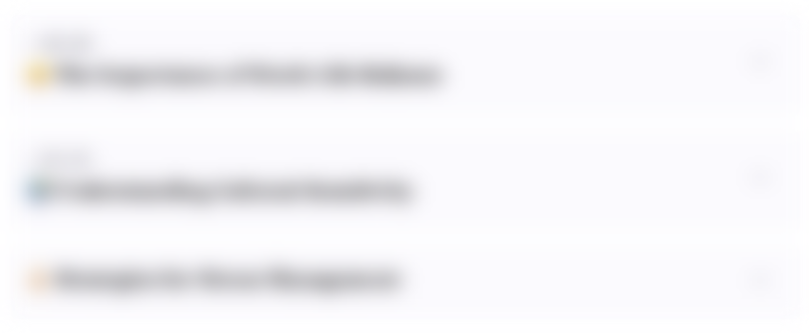
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
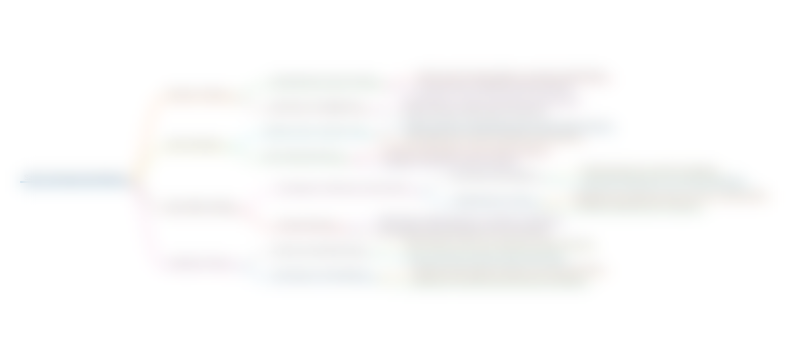
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
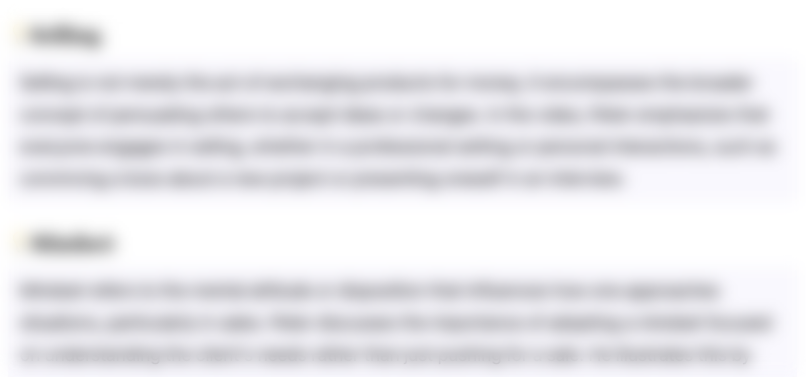
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
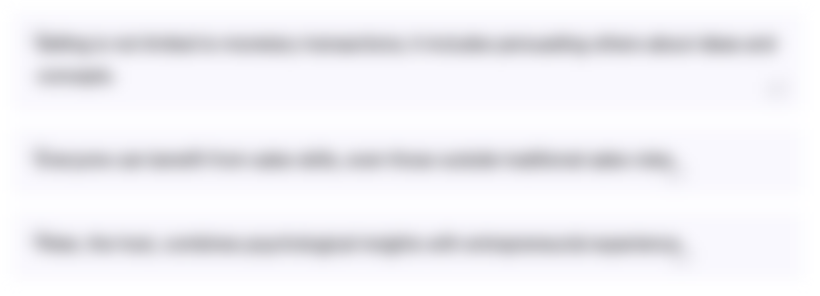
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
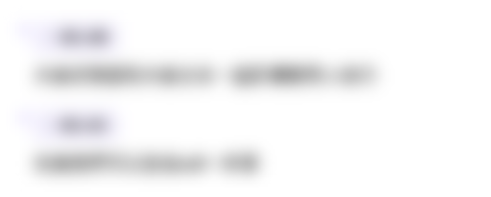
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
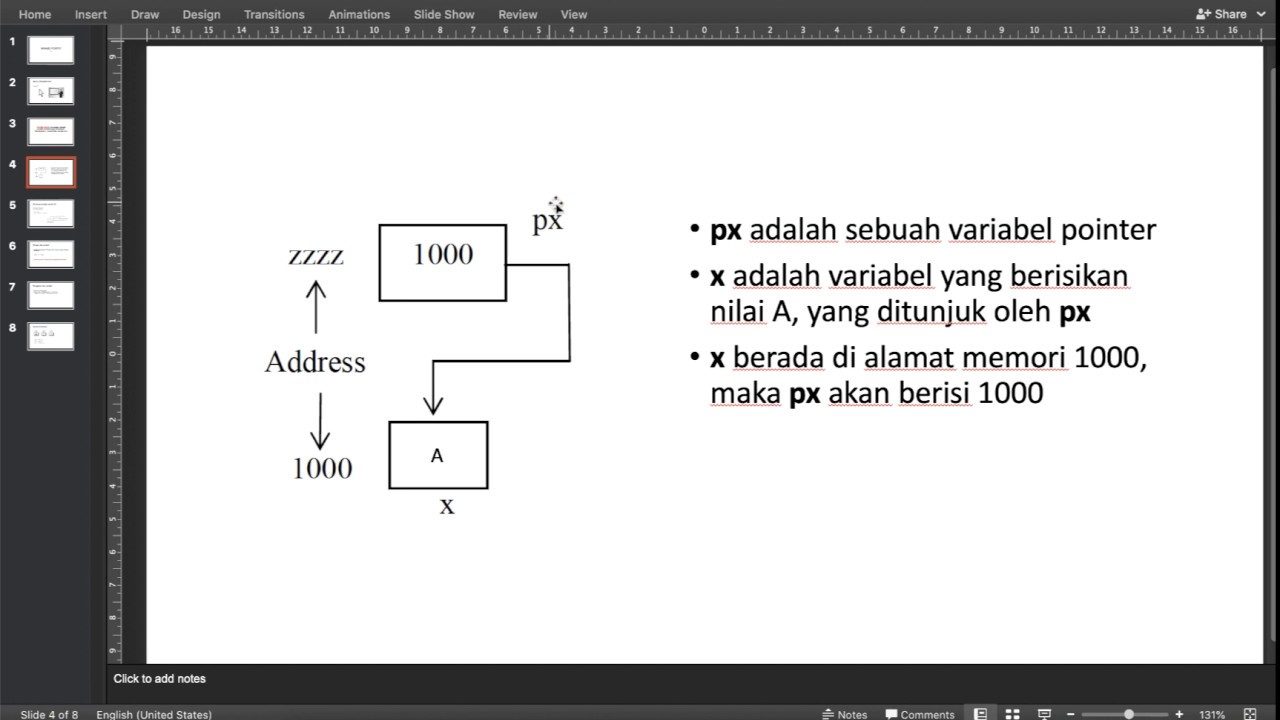
Variabel Pointer
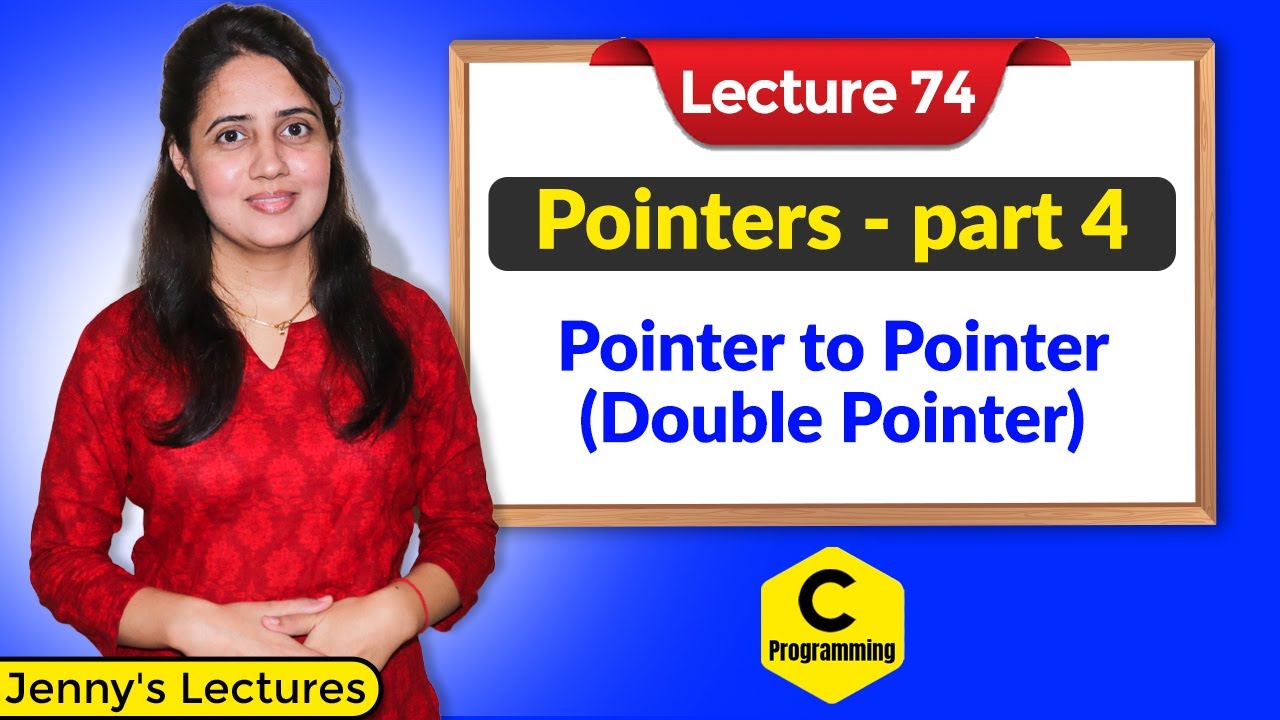
C_74 Pointers in C- part 4 | Pointer to Pointer (Double Pointer)
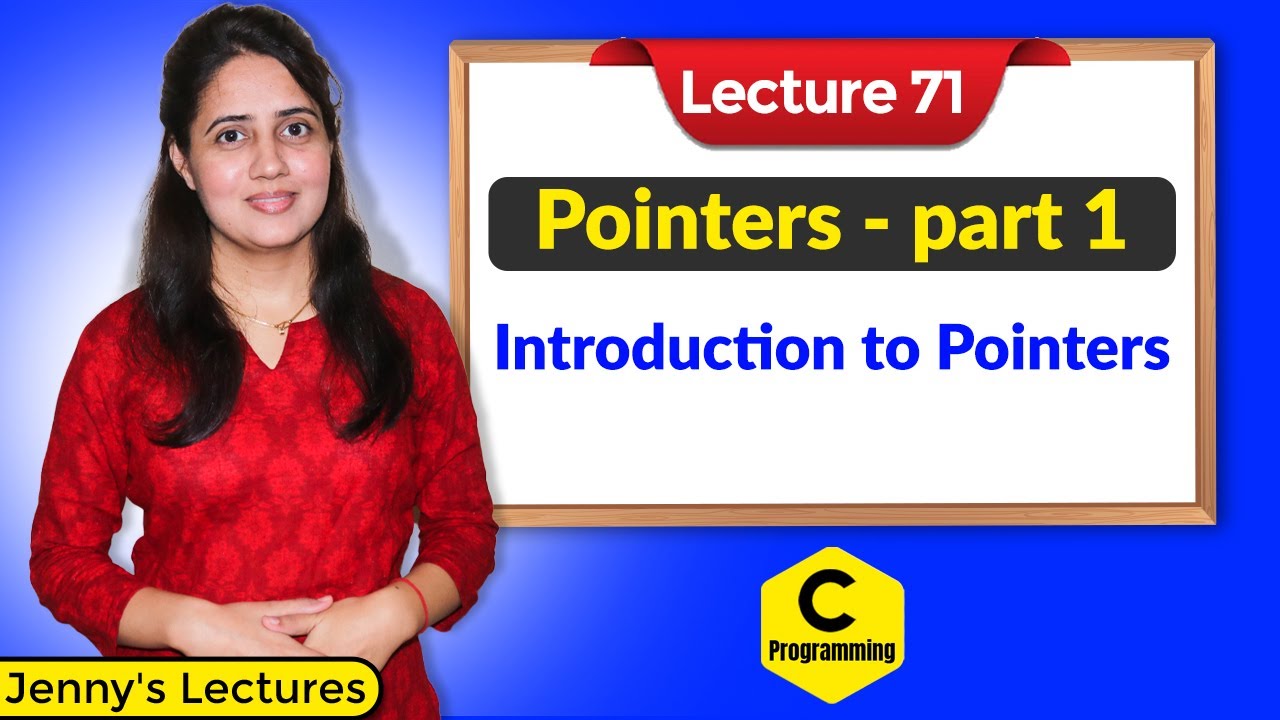
C_71 Pointers in C - part 1| Introduction to pointers in C | C Programming Tutorials

DAY 30 | COMPUTER SCIENCE | II PUC | POINTERS | L1
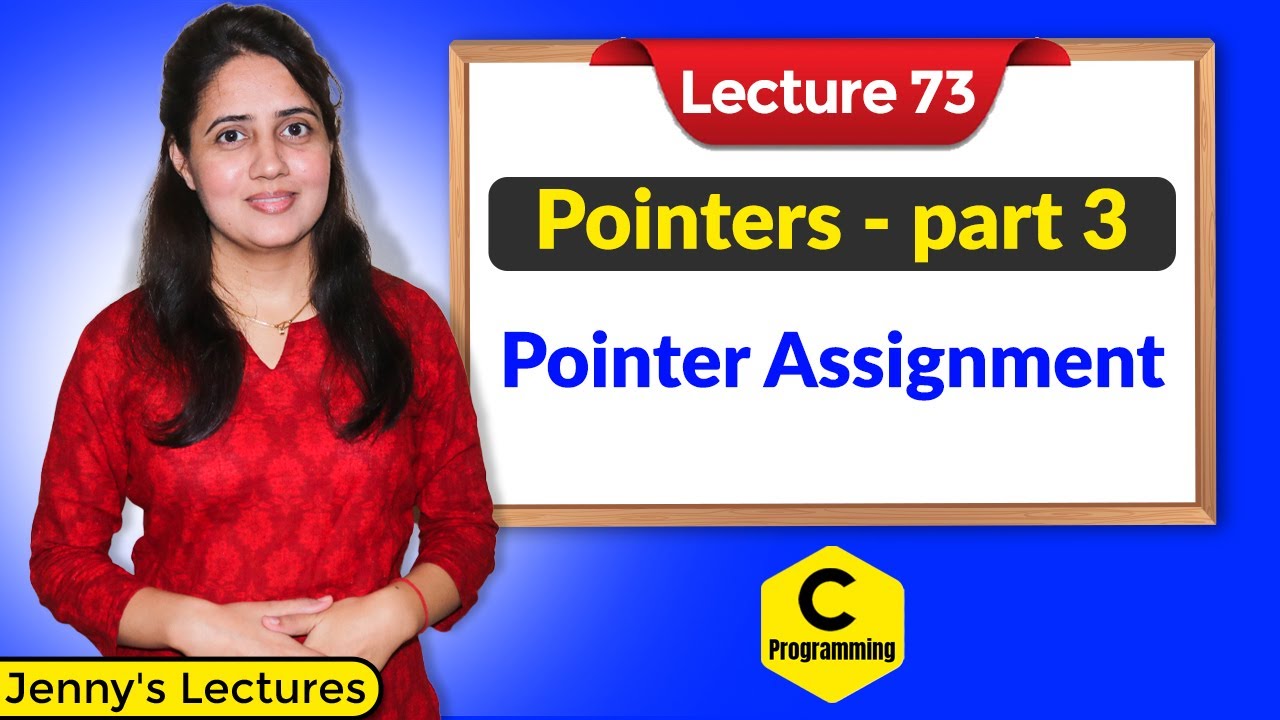
C_73 Pointers in C- part 3 | Pointer Assignment

The What, How, and Why of Void Pointers in C and C++?
5.0 / 5 (0 votes)