Learn Python OOP in under 20 Minutes
Summary
TLDRIn this educational video, the concept of Python classes is explored through an analogy with blueprints for constructing a house. The class is introduced as a template for creating objects, with a focus on the 'microwave' class. Key elements such as class instantiation, initializers, and instance methods are covered. The video demonstrates how to define and use methods within a class, like 'turn on' and 'run', to simulate the functionality of a microwave. Additionally, the use of dunder methods for customizing operator behavior and string representations of classes is explained, highlighting the importance of classes in organizing code and enhancing functionality.
Takeaways
- 🏗️ Classes in Python serve as blueprints for creating objects, defining their structure and behavior.
- 🔑 The class keyword in Python is used to define a class, and class names follow the camel case convention.
- 🔧 Instantiating a class creates an object, or an instance of that class, using the constructor (parentheses).
- ✅ Type annotations are optional in Python and are used for code editor assistance, not for runtime functionality.
- 🛠️ Initializers, or the __init__ method, allow customization of each instance with specific information.
- 🔄 The 'self' keyword in class methods refers to the instance of the class and ensures data sticks to the correct object.
- 🔀 Methods within a class define the functionality of the objects, such as turning a microwave on or off.
- 🔄 Dunder (double underscore) methods are special methods in Python classes that allow customization of built-in operations.
- 🔁 The __add__ method is an example of a dunder method that can be defined to handle the addition of two objects.
- 📝 The __str__ and __repr__ dunder methods provide user-friendly and developer-friendly string representations of objects, respectively.
Q & A
What is a class in Python?
-A class in Python is a blueprint for creating objects. It defines the properties and methods that an object of that class will have.
Why are classes useful in Python programming?
-Classes are useful because they allow for the creation of custom objects with specific properties and behaviors, promoting code reusability and organization.
What is an instance in the context of classes?
-An instance is a specific object created from a class blueprint. It represents a unique object with its own set of attributes and behaviors as defined by the class.
How do you create a class in Python?
-In Python, you create a class by using the 'class' keyword followed by the class name and a colon. The class name should follow the camel case convention, starting with an uppercase letter.
What is the purpose of the __init__ method in a class?
-The __init__ method is a special method called a constructor in Python. It is used to initialize the attributes of a class when an object is created.
What does 'self' represent in a class method?
-'self' represents the instance of the class and is used to access variables and methods within the class. It ensures that the data and methods belong to the correct instance.
How can you add functionality to a class in Python?
-You can add functionality to a class by defining methods within the class. Methods are functions that belong to the class and can perform actions on the object's data.
What is a Dunder method in Python?
-A Dunder method, short for 'double underscore' method, is a special method in Python classes that starts and ends with double underscores. These methods have special meanings and are called 'magic methods'.
Why is the string representation of an object important?
-The string representation of an object is important because it provides a human-readable description of the object, which is useful for debugging and logging.
How can you customize the way an object is displayed when printed?
-You can customize the way an object is displayed when printed by defining the __str__ method in the class. This method should return a string that represents the object.
What is the difference between __str__ and __repr__ methods in Python classes?
-The __str__ method should return a user-friendly string representation of the object, while the __repr__ method should return a developer-friendly representation that could be used to recreate the object if possible.
Outlines
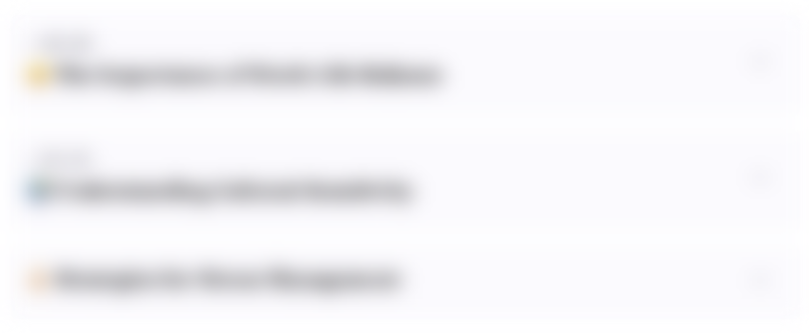
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
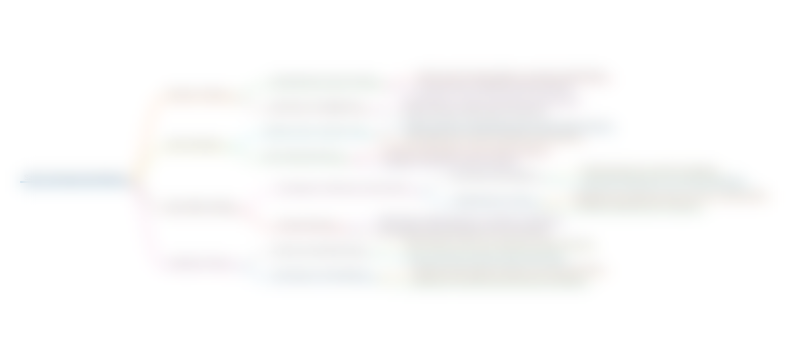
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
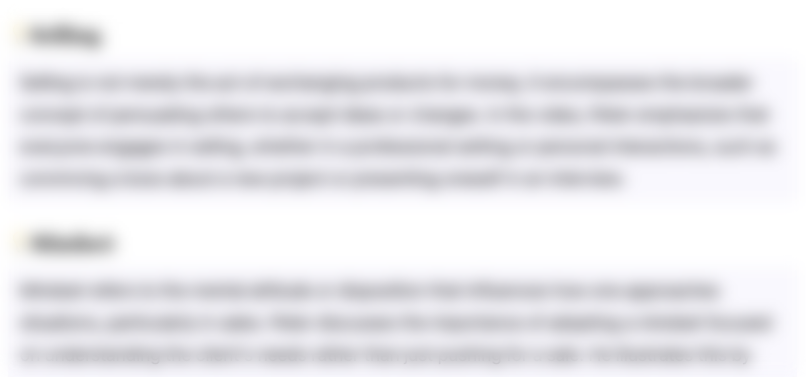
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
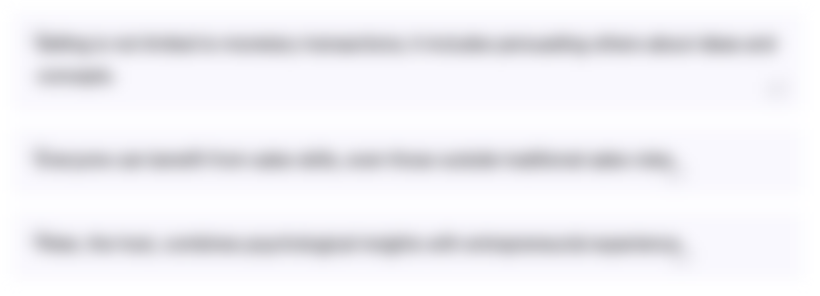
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
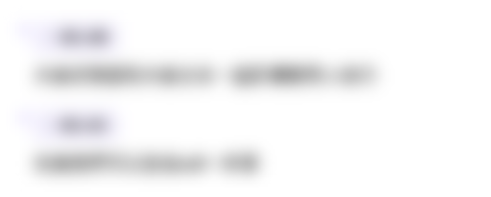
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео

Classes and Objects in Python | OOP in Python | Python for Beginners #lec85

SUPER() in Python explained! 🔴

Python OOP Tutorial 1: Classes and Instances
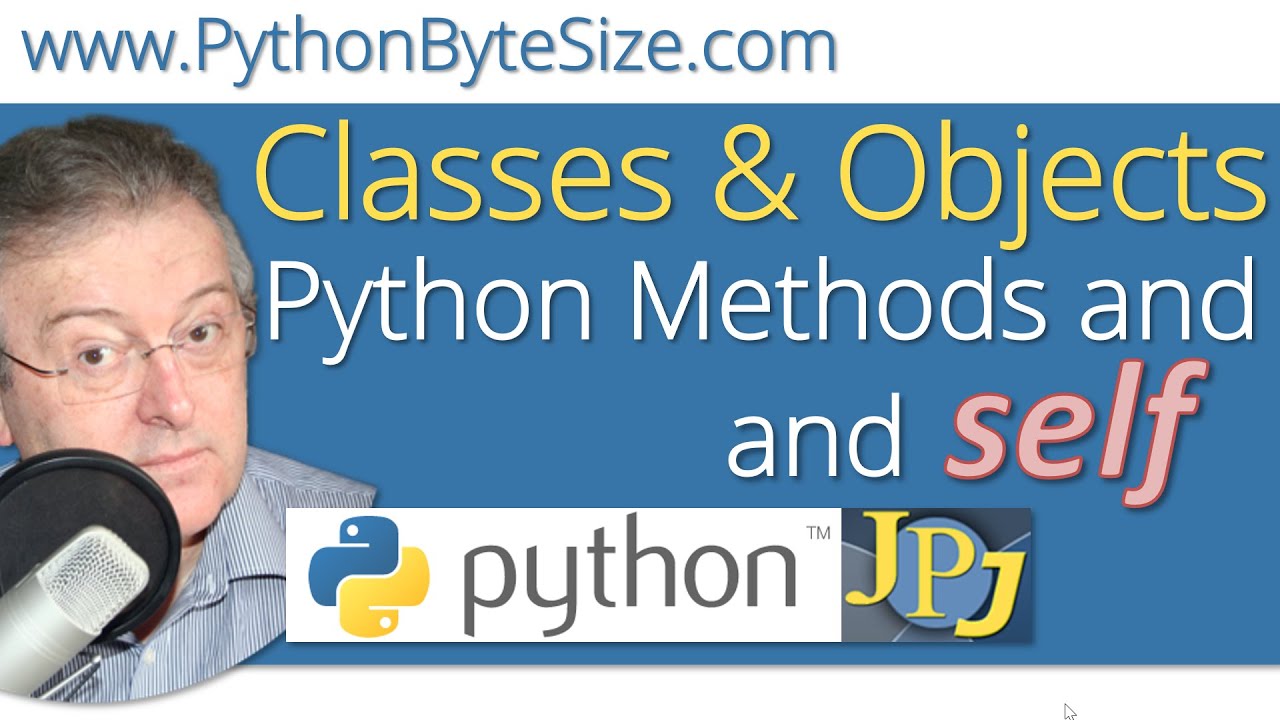
Python Methods and self
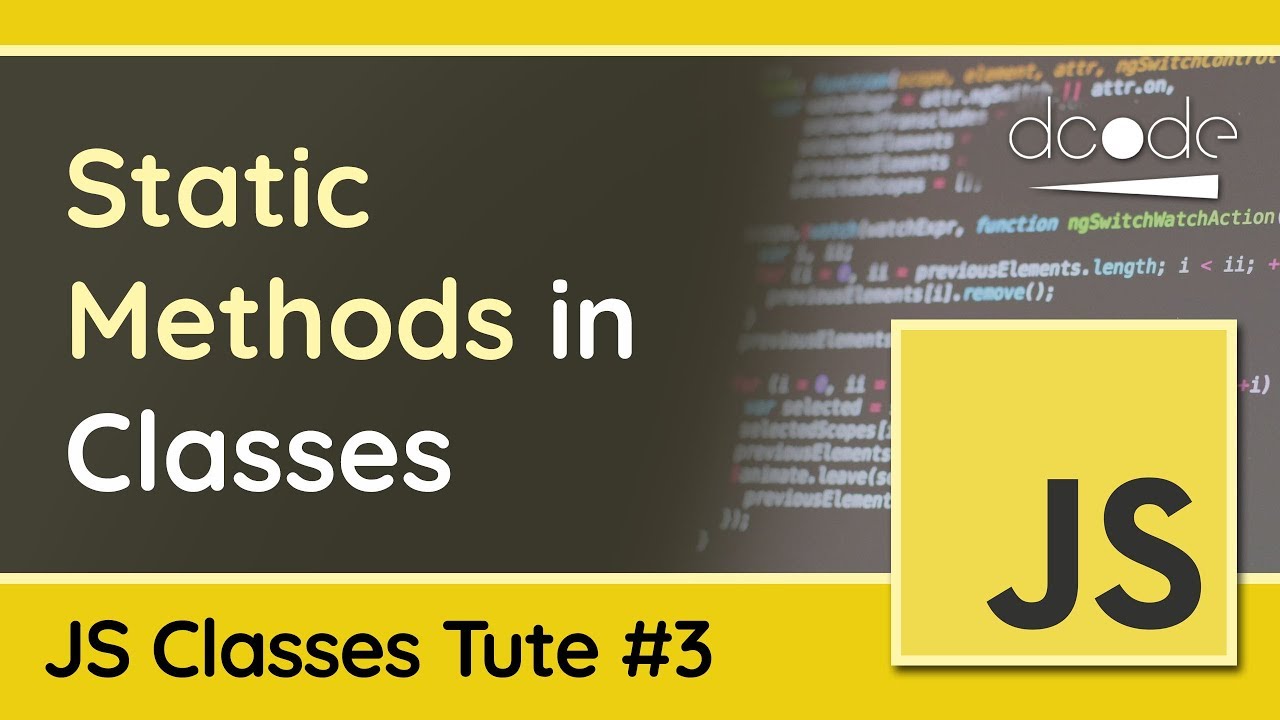
JavaScript Classes #3: Static Methods - JavaScript OOP Tutorial

Criando Métodos de Objetos - Curso Python Orientado a Objetos [Aula 03]
5.0 / 5 (0 votes)