Node.js Tutorial - 22 - Extending from EventEmitter
Summary
TLDRThis video tutorial introduces how to build a custom module in Node.js that extends the EventEmitter class. The presenter demonstrates creating a 'pizzashop.js' file with a class that handles order placements and displays the order number. By inheriting from EventEmitter, the PizzaShop class can emit custom events, which are then listened to and handled in 'index.js'. The video also shows how to integrate the event-driven architecture with other modules, such as a 'drinkmachine.js', to create a loosely coupled system. The presenter encourages viewers to learn more about class inheritance in JavaScript and hints at upcoming videos on built-in modules like FS, streams, and HTTP.
Takeaways
- 📚 The video introduces creating a custom module in Node.js that builds on the Event Emitter class.
- 🍕 A 'pizzashop.js' file is created to define a PizzaShop class with a constructor and methods for order handling.
- 🔢 The PizzaShop class initializes an order number to zero and includes methods to increment and display the order number.
- 📦 The PizzaShop class is exported for use in other modules, demonstrating modularity in Node.js applications.
- 📝 In 'index.js', the PizzaShop class is imported and an instance is created to test the order methods.
- 🔄 The video explains how to use event-driven architecture in the PizzaShop class by utilizing inheritance from the Event Emitter class.
- 📜 The 'extends' keyword is used to inherit from the Event Emitter, allowing the PizzaShop class to emit events.
- 📡 The 'this.emit' method is used within the order method to emit an 'order' event with 'size' and 'Topping' parameters.
- 📌 Event listeners are attached in 'index.js' to handle the 'order' event and log the received order details to the console.
- 🥤 A separate module, 'drinkmachine.js', is introduced to handle drink serving logic based on the order size.
- 🔗 The DrinkMachine class is used within the order event listener to serve a complimentary drink for large orders, showcasing event-based interaction between modules.
Q & A
What is the main focus of the video?
-The video focuses on demonstrating how to create a custom module in Node.js that builds on top of the event emitter class, using the example of a pizza shop.
What is the purpose of creating a 'pizzashop.js' file?
-The 'pizzashop.js' file is created to define a PizzaShop class that has its own properties and methods for handling orders in an event-driven architecture.
What are the two methods defined in the PizzaShop class?
-The two methods defined in the PizzaShop class are one to place an order, which increments the order number, and another called displayOrderNumber to view the current order number.
How is the PizzaShop class exported for use in other modules?
-The PizzaShop class is exported using the `module.exports` syntax, allowing it to be imported and used in other modules like 'index.js'.
What is the purpose of the 'extends' keyword in JavaScript?
-The 'extends' keyword in JavaScript is used for inheritance, allowing one class to inherit the properties and methods of another class.
How does the PizzaShop class inherit from the event emitter class?
-The PizzaShop class inherits from the event emitter class by using the `extends` keyword and invoking the `super` function within its constructor.
What is the significance of emitting an event in the order method?
-Emitting an event in the order method allows the PizzaShop class to trigger custom events, such as 'order', and pass parameters like size and toppings to event listeners.
What is the role of the 'DrinkMachine' class in the script?
-The 'DrinkMachine' class is a separate module that handles the logic for serving drinks based on the order size, and it is used to demonstrate how different modules can interact using events.
How does the 'DrinkMachine' class interact with the PizzaShop class?
-The 'DrinkMachine' class interacts with the PizzaShop class by being called within the order event listener, where it receives the order size and decides whether to serve a complimentary drink.
What is the advantage of using event-driven architecture in this scenario?
-The advantage of using event-driven architecture is that it allows different modules to communicate and react to events without being tightly coupled, promoting modularity and flexibility in the code.
Why is it important to understand that built-in modules like FS, streams, and HTTP extend from the event emitter class?
-Understanding that built-in modules extend from the event emitter class is important because it highlights the versatility and power of the event-driven architecture in Node.js, allowing developers to handle asynchronous events in a standardized way.
Outlines
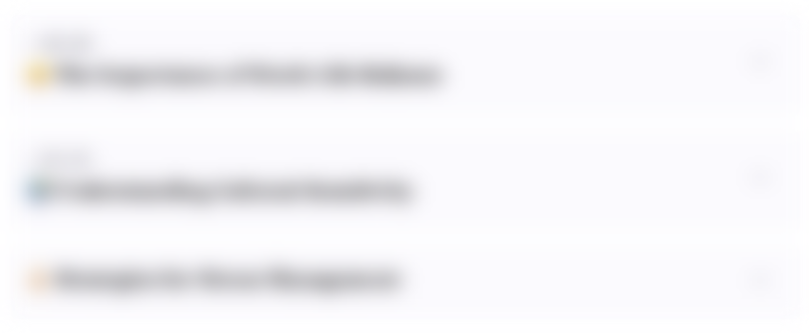
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
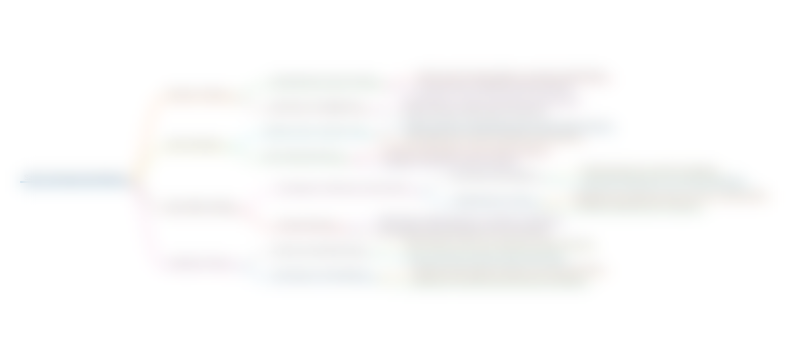
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
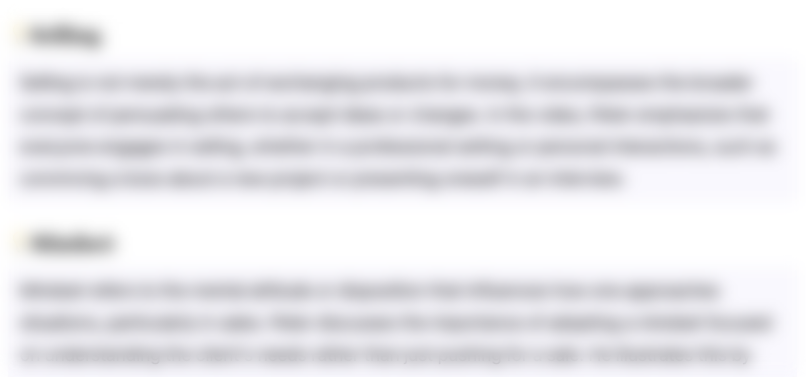
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
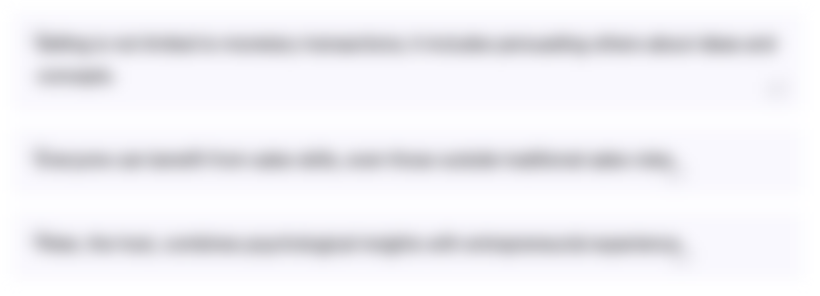
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
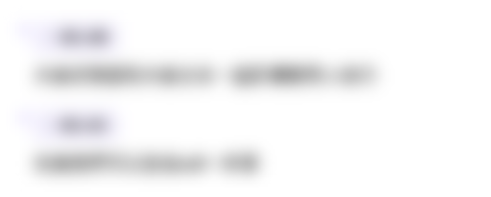
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
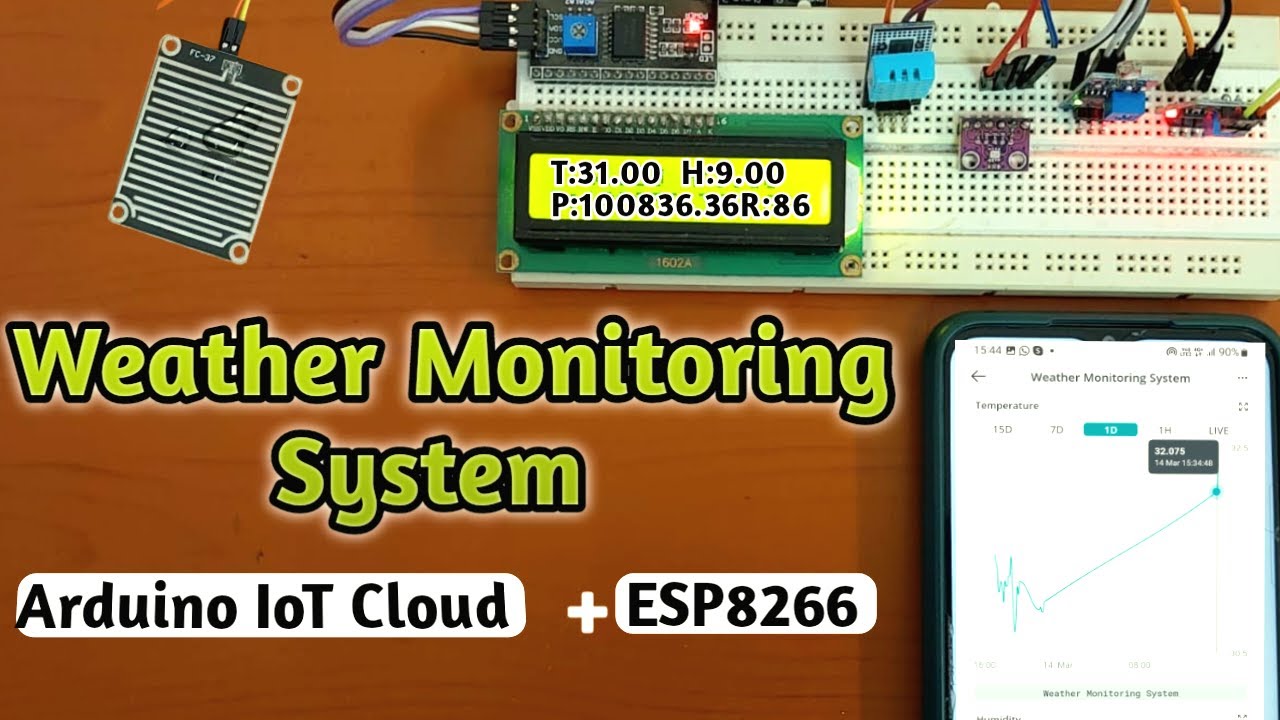
DIY Weather Monitoring System with Arduino IoT Cloud and ESP8266 | Arduino IoT Cloud Projects
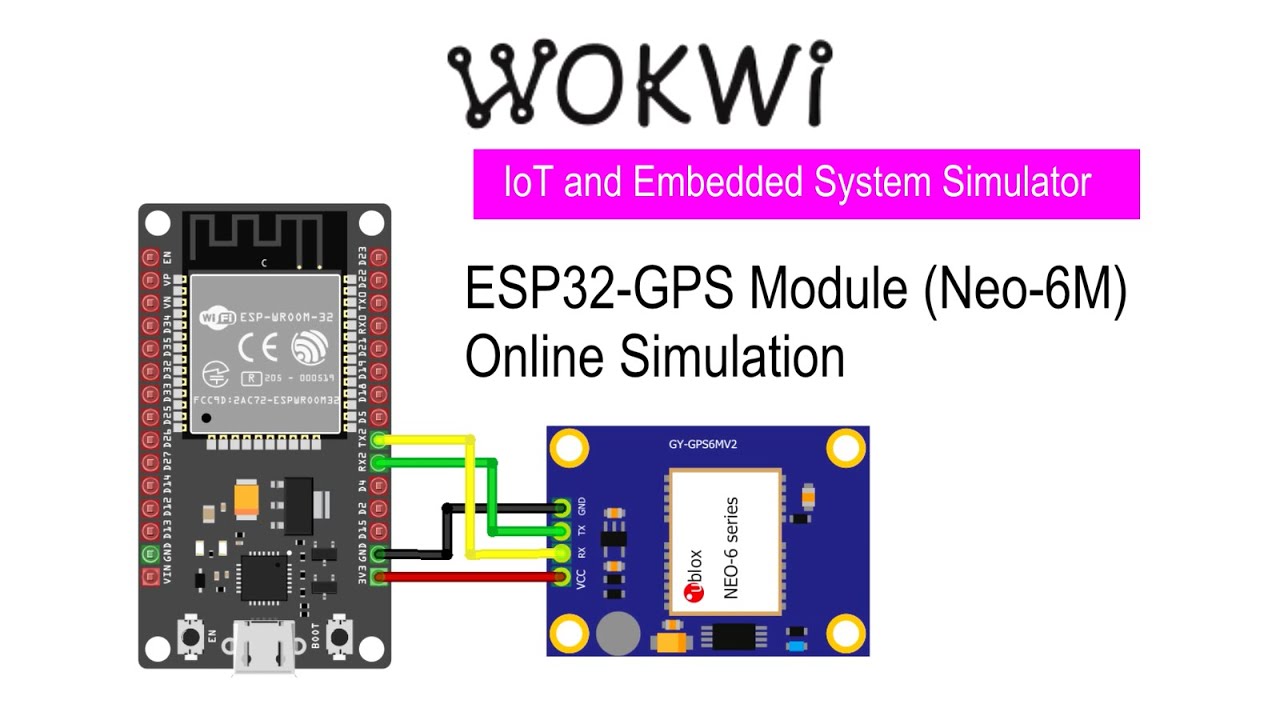
Simulasi ESP32 - modul GPS Neo 6M dengan Wokwi IoT Simulator
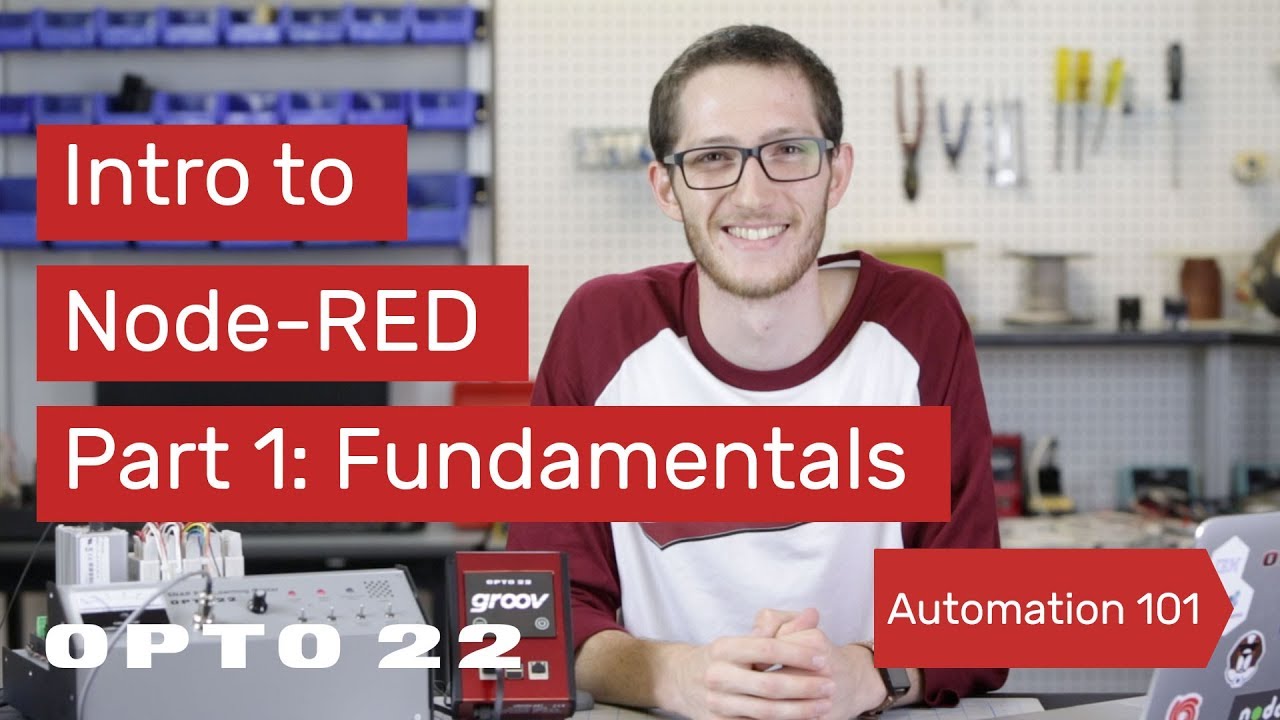
Intro to Node-RED: Part 1 Fundamentals

TODO sobre EL SENSOR de PESO con MODULO HX711 , Peso EXACTO con arduno || Tutorial

Belajar NodeJS | 6. NodeJS Module System
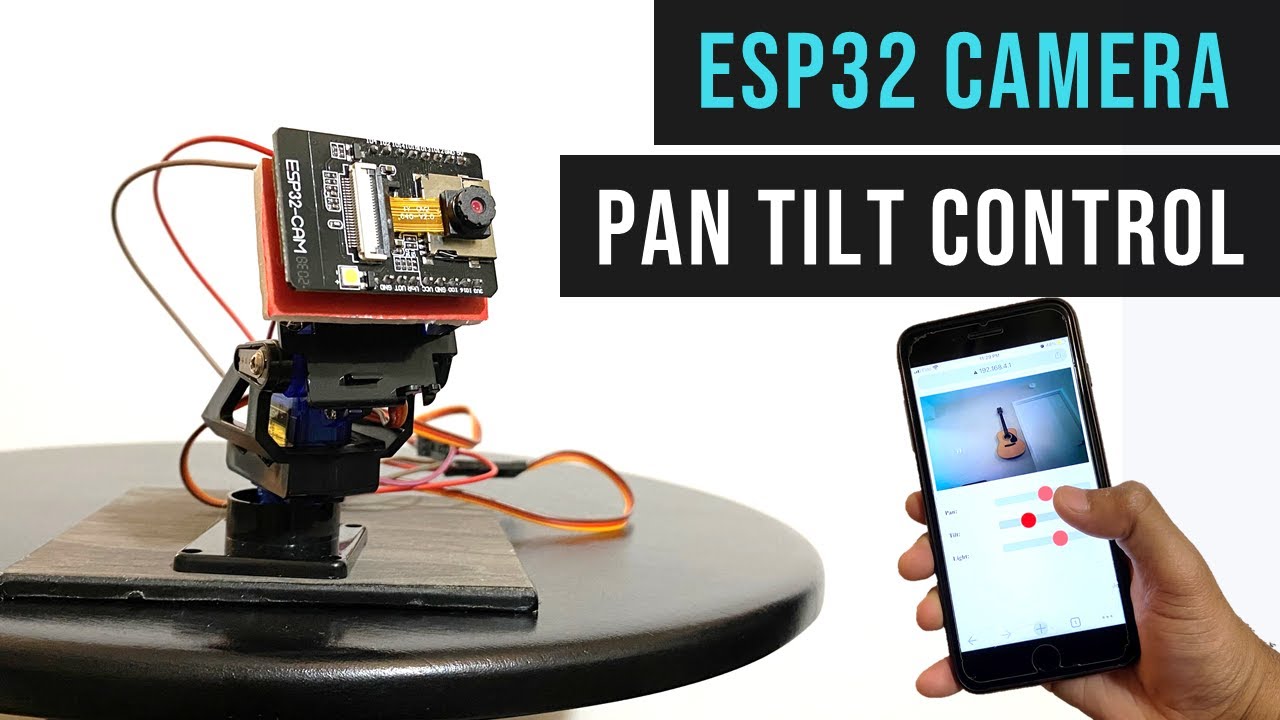
Pan Tilt Control using Servos for ESP32 Cam | WiFi Security Camera
5.0 / 5 (0 votes)