2 Simple Ways To Code Linked Lists In Python
Summary
TLDRThis video tutorial demonstrates how to create a linked list in Python using object-oriented programming. It explains the concept of nodes, each with a value and a 'next' pointer to the subsequent node. The presenter introduces a 'LinkedListNode' class and shows how to manually link nodes to form a list. Further, a 'LinkedList' class is introduced with an 'insert' function to dynamically add elements. The video is aimed at viewers who are new to linked lists and object-oriented programming, providing a foundational understanding before moving on to more complex interview questions in subsequent videos.
Takeaways
- 💻 The video demonstrates how to create a linked list in Python using object-oriented programming.
- 🔗 A linked list is composed of nodes, each containing a value and a pointer to the next node.
- 📚 The script briefly explains object-oriented programming concepts to help viewers understand linked lists.
- 👨🏫 The tutorial starts by defining a 'linked list node' class with a constructor for initializing node values and setting the next node to None.
- 🔧 The video shows how to manually create and connect nodes to form a linked list, illustrating with an example of a list containing the values 3, 7, and 10.
- 🔄 A while loop is used to traverse the linked list, printing each node's value until the end of the list is reached (where the next node is None).
- 📈 The script introduces a more advanced approach by creating a 'linked list' class that includes an 'insert' function to add nodes dynamically.
- 🛠️ The 'insert' function checks if the list is empty and appropriately sets the head or appends the new node to the end (tail) of the list.
- 📊 The video concludes with a testing function that prints out the contents of the linked list to verify the insert function's correctness.
- 🔄 The script highlights the flexibility of the linked list implementation, suggesting further enhancements like tracking the tail node or the number of nodes.
Q & A
What is a linked list and how is it different from other data structures?
-A linked list is a linear data structure where each element is a separate object, called a node, which contains a value and a reference (or link) to the next node in the sequence. Unlike arrays, linked lists do not have a fixed size and can be dynamically resized during execution. Each node points to the next node in the list, and the last node points to null, indicating the end of the list.
What are the two main attributes of a node in a linked list?
-Each node in a linked list has two main attributes: the first is the 'value' which can be of any data type such as strings, integers, characters, objects, etc. The second attribute is the 'next', which is a pointer to the next node in the sequence.
How is the 'next' attribute of a node used in a linked list?
-The 'next' attribute in a node is used to point to the subsequent node in the linked list. This attribute creates a chain of nodes, allowing traversal from the head to the tail of the list. It is initialized to 'None' when a node is created and is updated to reference the next node when connecting nodes.
What is the purpose of the 'linked list node' class mentioned in the script?
-The 'linked list node' class is a blueprint for creating individual nodes in a linked list. It defines the structure for a node, including its value and the reference to the next node. This class is used to instantiate new nodes with specific values when building a linked list.
How does the script demonstrate creating a simple linked list with three nodes?
-The script demonstrates creating a linked list by first defining a 'linked list node' class with a constructor that initializes the node's value and sets the 'next' attribute to 'None'. Then, it creates three nodes with values 3, 7, and 10, and manually connects them by setting the 'next' attribute of each node to the next node in the sequence.
What is the significance of the 'head' in a linked list?
-The 'head' is the first node in a linked list and serves as the entry point for list operations. It is the starting reference from which traversal, insertion, and deletion operations begin. In the script, the head is initially set to 'None' for an empty list and is updated to reference the first node when nodes are added.
How does the script handle inserting a new node into an existing linked list?
-The script handles node insertion by first checking if the list is empty (head is 'None'). If it is, the new node becomes the head. If not, it traverses the list to find the tail (the last node where 'next' is 'None'), and then sets the 'next' attribute of the tail to point to the new node, effectively appending it to the end of the list.
What is the role of the 'insert' function in the linked list class?
-The 'insert' function in the linked list class is responsible for adding new nodes to the list. It takes a value as input, creates a new node with that value, and then appends it to the end of the list by updating the 'next' attribute of the current tail node.
Why is it important to check if the 'current node next' is 'None' in the traversal loop?
-Checking if 'current node next' is 'None' is crucial for determining whether the current node is the last node in the list. This condition is used to identify the tail node, where new nodes should be appended during the insertion process.
How does the script suggest testing the functionality of the linked list implementation?
-The script suggests testing the linked list implementation by creating a linked list, inserting elements, and then using a while loop to traverse and print the values of the nodes. This helps verify that nodes are correctly connected and that the list operates as expected.
Outlines
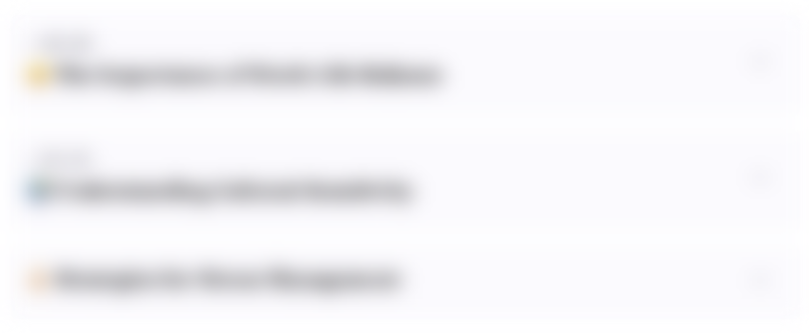
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
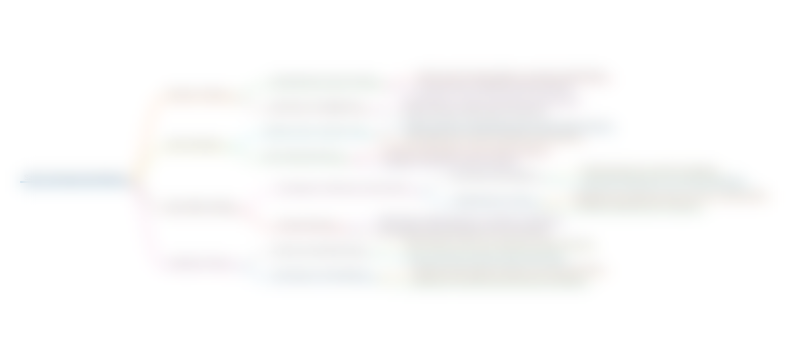
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
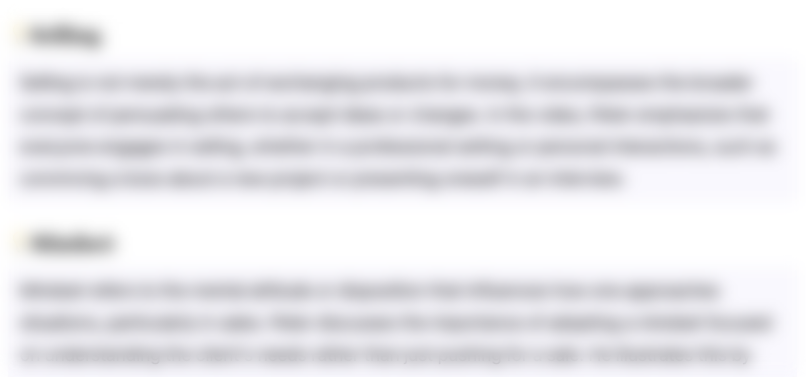
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
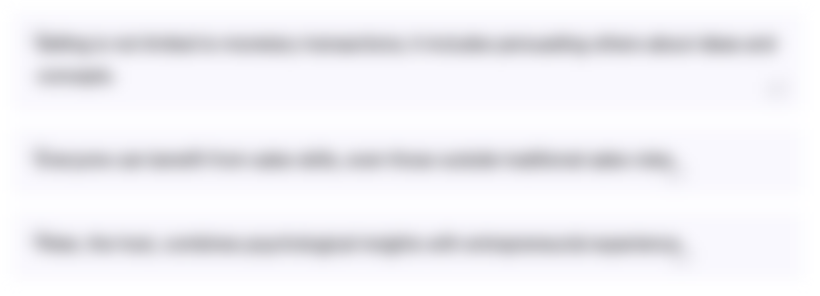
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
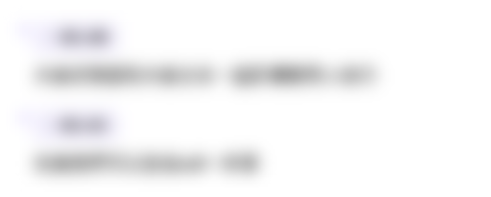
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)