Quick Sort - Computerphile
Summary
TLDRThis video script explains the Quicksort algorithm, a popular sorting technique. It begins with an unsorted list and selects a 'pivot' element, typically the last one for convenience. Elements are partitioned into two groups, those less than or equal to the pivot and those greater. This process is recursively applied to the sublists until each contains one or no elements, ensuring they are sorted. The script also discusses the importance of pivot selection and its impact on performance, highlighting that while Quicksort averages O(n log n) time, poor pivot choices can degrade to O(n^2), as demonstrated with a sorted list example.
Takeaways
- 📚 Quicksort is an algorithm used for sorting a list of elements.
- 🔍 The process begins with an unsorted list which is then partitioned into two parts.
- 🔑 A pivot element is chosen, typically the rightmost element in the script.
- 🔄 Elements less than or equal to the pivot are placed on one side, and those greater are placed on the other.
- 🔄 The partitioning process is repeated for the sublists until each sublist has one or no elements, indicating they are sorted.
- 🔄 The pivots are then rejoined to form the sorted list, with elements on the left of the pivot and those on the right being placed accordingly.
- 🚀 The choice of pivot can affect the efficiency of the algorithm, with the middle most number being ideal in theory.
- 🚨 In practice, the first or last element is often chosen for simplicity.
- 📉 The average case for Quicksort is O(n log n), similar to merge sort.
- 📈 The best case for Quicksort is O(log n), but the worst case is O(n^2), which can occur if the pivots are consistently poor choices.
- 🚫 The script also mentions the inefficiency of bubble sort and merge sort in the worst case, highlighting the importance of algorithm choice.
Q & A
What is the Quicksort algorithm?
-Quicksort is a highly efficient sorting algorithm that operates by partitioning an array into two halves, then recursively sorting the sub-arrays. It works by selecting a 'pivot' element and rearranging the array so that all elements less than the pivot are on one side, and all elements greater are on the other side.
Why is the choice of pivot important in Quicksort?
-The choice of pivot is crucial as it affects the efficiency of the algorithm. Ideally, the pivot should be the median value to ensure balanced partitions, leading to optimal performance. Poor pivot choices can lead to unbalanced partitions, degrading the algorithm's efficiency.
What happens if the pivot is always chosen from the rightmost element?
-If the pivot is always chosen from the rightmost element, especially in an already sorted list, the algorithm can degrade to O(n^2) complexity. This is because each partition will only have one element less than the pivot, leading to a large number of recursive calls.
How does Quicksort handle sub-arrays during the sorting process?
-Quicksort recursively handles sub-arrays by selecting a pivot for each sub-array, partitioning the elements around the pivot, and then sorting the resulting sub-arrays. This process continues until each sub-array has one or no elements, at which point they are considered sorted.
What is the average case time complexity of Quicksort?
-In the average case, Quicksort has a time complexity of O(n log n), which makes it very efficient for large datasets.
What is the best case scenario for Quicksort?
-The best case scenario for Quicksort occurs when the pivot divides the array into two equal halves at each step, leading to a time complexity of O(log n).
What is the worst case scenario for Quicksort?
-The worst case scenario for Quicksort occurs when the pivot is the smallest or largest element in the array at each step, leading to a time complexity of O(n^2).
How does Quicksort compare to Merge Sort in terms of worst case performance?
-While Quicksort can degrade to O(n^2) in the worst case, Merge Sort maintains a worst case time complexity of O(n log n), making it more consistent in performance.
What is the role of the pivot in partitioning the array?
-The pivot is used to divide the array into two parts. Elements less than or equal to the pivot are placed on one side, and elements greater than the pivot are placed on the other side.
How does the script mention the handling of third-party cookies?
-The script mentions third-party cookies in the context of advertisers trying to determine if a user has seen an advertisement before, but this is likely an unrelated note or a misinterpretation in the transcript.
Outlines
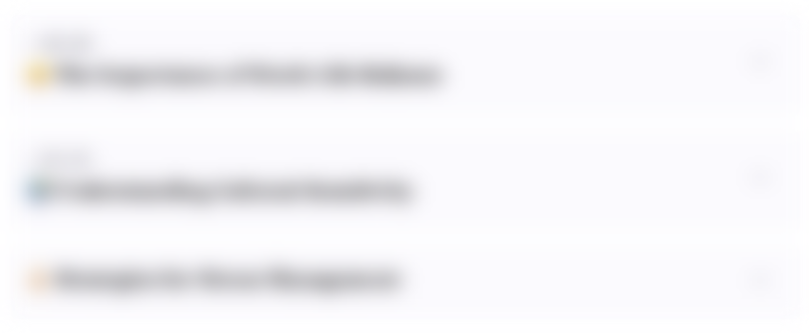
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
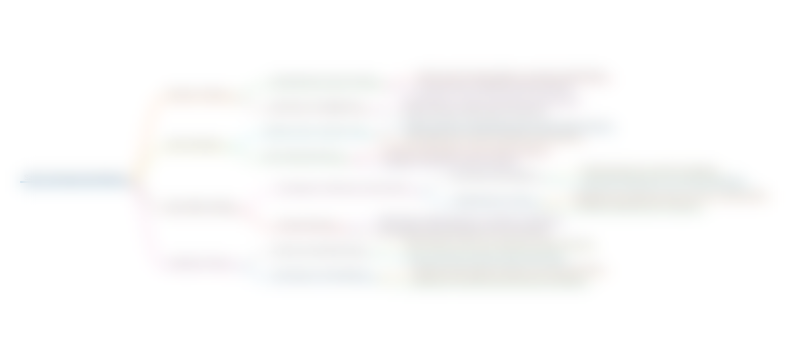
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
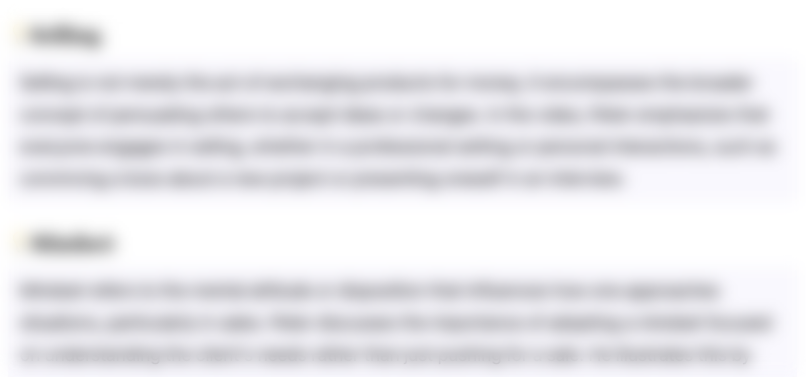
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
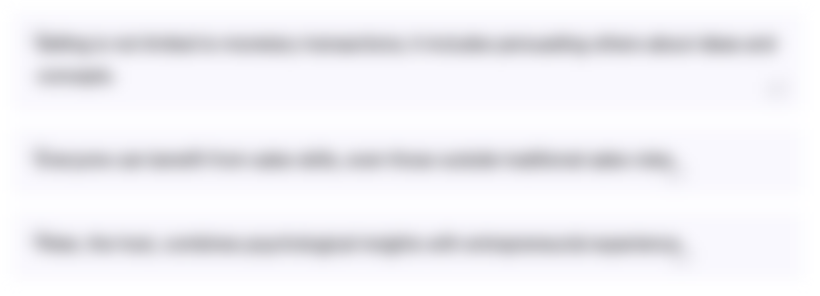
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
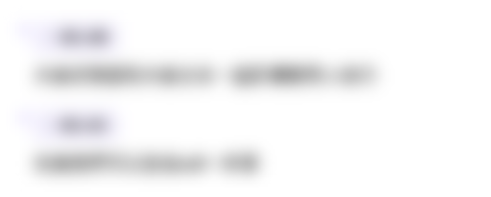
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード5.0 / 5 (0 votes)